Learn Java Unit Testing with Junit & Mockito in 30 Steps
Read more about the course
You take 12 steps with JUnit and 18 steps with Mockito into unit testing proficiency.
Mocking with Mockito
Step 01 : Set up an Eclipse Project with JUnit and Mockito frameworks. First Green Bar.
Step 02 : Example to start understanding why we need mocks.
Step 03 : What is a stub? Create an unit test using Stub? Disadvantages of Stubs.
Step 04 : Your first Mockito code! Hurrah!!! Lets use Mockito to mock TodoService.
Step 05 : Stubbing variations with Mockito. A few mockito examples mocking List class : Multiple return values, Argument Matchers and throwing exceptions.
Step 06 : Introduction to BDD. Given When Then. BDD Mockito Syntax.
Step 07 : How to verify calls on a mock? Verify how many times a method is called. We will add deleteTodo method to the TodoService.
Step 08 : How to capture an argument which is passed to a mock?
Step 09 : Hamcrest Matchers.
Step 10 : Let's simplify things with Mockito Annotations. @Mock, @InjectMocks, @RunWith(MockitoJUnitRunner.class), @Captor
Step 11 : JUnit Rules. Using MockitoJUnit.rule() instead of @RunWith(MockitoJUnitRunner.class).
Step 12 : Real world Example with Spring
Step 13 : What is a spy? How to spy with Mockito?
Step 14 : Some Theory : Why does Mockito not allow stubbing final and private methods?
Step 15 : Using PowerMock and Mockito to mock a Static Method.
Step 16 : Using PowerMock and Mockito to invoke a private Method.
Step 17 : Using PowerMock and Mockito to mock a constructor.
Step 18 : Good Unit Tests.
JUnit Framework
Step 01 : Need for Unit Testing
Step 02 : Setting up your First JUnit
Step 03 : First Successful JUnit. Green Bar and assertEquals
Step 04 : Refactoring Your First JUnit Test
Step 05 : Second JUnit Example assertTrue and assertFalse
Step 06 : @Before @After
Step 07 : @BeforeClass @AfterClass
Step 08 : Comparing Arrays in JUnit Tests
Step 09 : Testing Exceptions in JUnit Tests
Step 10 : Testing Performance in JUnit Tests
Step 11 : Parameterized Tests
Step 12 : Organize JUnits into Suites
Watch Online Learn Java Unit Testing with Junit & Mockito in 30 Steps
# | Title | Duration |
---|---|---|
1 | Introduction | 02:19 |
2 | Three thing you need to know | 01:46 |
3 | JUnit Step 1 : Why is Unit Testing Important? | 03:42 |
4 | JUnit Step 2 : Setting up your first JUnit | 08:06 |
5 | Step 03 : First Successful JUnit. Green Bar and assertEquals | 09:40 |
6 | Step 04 : Refactoring Your First JUnit Test | 07:45 |
7 | Step 05 : Second JUnit Example assertTrue and assertFalse | 14:46 |
8 | Step 06 : @Before @After | 07:03 |
9 | Step 07 : @BeforeClass @AfterClass | 03:31 |
10 | Step 08 : Comparing Arrays in JUnit Tests | 05:20 |
11 | Step 09 : Testing Exceptions in JUnit Tests | 03:34 |
12 | Step 10 : Testing Performance in JUnit Tests | 04:04 |
13 | Step 11 : Parameterized Tests | 11:12 |
14 | Step 12 : Organize JUnits into Suites | 03:25 |
15 | An Overview | 01:54 |
16 | Mockito Step 01 : Setting up a Maven Project | 07:32 |
17 | Overview of this Section | 02:11 |
18 | Mockito Step 02 : Setting up SUT (System Under Test) | 11:03 |
19 | Step 03 : Stubbing Example - with Disadvantages of Stubbing | 11:44 |
20 | Step 04 : Your first Mockito code! Hurrah!!! | 18:55 |
21 | Basics of Mockito - Section Overview | 01:06 |
22 | Step 05 : Stubbing variations with Mockito - Argument Matchers & More... | 13:05 |
23 | Step 06 : BDD Style - Given When Then | 13:29 |
24 | Step 07 : Verify calls on Mocks | 08:53 |
25 | Step 08 : Capturing arguments passed to a Mock | 08:44 |
26 | Step 09 : Hamcrest Matchers | 11:42 |
27 | Step 10 : Mockito Annotations - @Mock, @InjectMocks, @RunWith, @Captor.. | 10:01 |
28 | Step 11 : Mockito Junit Rule | 03:41 |
29 | Step 12 : Real world Mockito Example with Spring | 16:09 |
30 | Step 13 : Mockito Spy | 10:04 |
31 | Step 14 : Theory : Why does Mockito not allow stubbing final & private methods? | 04:26 |
32 | Step 15 : Setting up PowerMock and SystemUnderTest | 08:38 |
33 | Step 15 : Continued. Mocking Static Method | 12:32 |
34 | Step 16 : Invoking Private Methods | 05:44 |
35 | Step 17 : Mocking a Constructor | 09:26 |
36 | Step 18 : Writing Good Unit Tests | 07:23 |
Similar courses to Learn Java Unit Testing with Junit & Mockito in 30 Steps
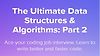
The Ultimate Data Structures & Algorithms: Part 2codewithmosh (Mosh Hamedani)
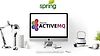
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
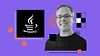
Java Data Structures & Algorithms + LEETCODE Exercisesudemy
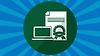
Oracle Java Certification - Pass the Associate 1Z0-808 Exam.udemy
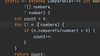
Java Genericsamigoscode (Nelson Djalo)
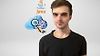
Java Test Automation Engineer - from Zero to Heroudemy
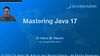
Mastering Java 17javaspecialists.eu
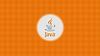
Experience Design Patterns In Javaudemy
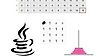
Algorithms in Java: Live problem solving & Design Techniquesudemy
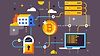