Master Microservices with Java, Spring, Docker, Kubernetes
'Master Microservices with Spring, Docker, Kubernetes' course will help in understanding about microservices architecture and how to build it using SpringBoot, Spring Cloud components, Docker and Kubernetes.
Read more about the course
By the end of this course, students will understand all the below topics:
What is microservices architecture and how it is different from monolithic and SOA architectures
How to build production ready microservices using Spring, SpringBoot and Spring Cloud
What are cloud native apps & 12 factor principles behind them
Configuration management in microservices using Spring Cloud Config Server
Service Discovery and Registration pattern inside microservices and how to implement using Spring Eureka server
Building resilient microservices using RESILIENCE4J framework
Handling Cross cutting concerns and routing inside microservices using Spring Cloud Gateway
Implementing Distributed tracing & Log aggregation in microservices using Spring Sleuth and Zipkin
Monitoring microservices using Prometheus and Grafana
Role of Docker in microservices and how to build docker images, containers
Role of Docker compose and how to use it to run all the microservices inside a application
Most commonly used Docker commands
Role of Kubernetes in microservices as a container orchestration framework.
How to setup a Kubernetes cluster inside GCP using GKE (Google Kubernetes Engine) and deploy microservices inside it
Most commonly used Kubernetes commands
Microservices Security using OAuth2
What is Helm & it's role in microservices world
The pre-requisite for the course is basic knowledge of Java, Spring and interest to learn.
Watch Online Master Microservices with Java, Spring, Docker, Kubernetes
# | Title | Duration |
---|---|---|
1 | Introduction to the course & Agenda | 05:33 |
2 | Details of Source Code, PDF Content & other instructions for the course | 07:09 |
3 | Evolution of Microservices architecture | 07:56 |
4 | Deepdive of Monolithic architecture | 10:08 |
5 | Deepdive of SOA architecture | 06:35 |
6 | Deepdive of Microservices architecture | 11:01 |
7 | Comparisons between Monolithic, SOA & Microservices architecture | 07:37 |
8 | Definition of Microservices | 03:08 |
9 | Why Spring is the best framework for building microservices | 05:17 |
10 | Using Spring Boot for microservices development | 07:48 |
11 | Creating a Hello World service using Spring Boot | 10:28 |
12 | Using Spring Cloud for microservices development | 09:53 |
13 | Approaches to identify boundaries & right sizing for building microservices | 09:52 |
14 | Sizing & identifying boundaries with a Bank App use case | 05:26 |
15 | Sizing & identifying boundaries with a Ecommerce migration use case | 06:58 |
16 | Introduction to the microservices that we are going to build | 01:40 |
17 | Creating Bank Accounts Microservices using SpringBoot | 17:04 |
18 | Creating Bank Loans Microservices using SpringBoot | 05:46 |
19 | Creating Bank Cards Microservices using SpringBoot | 05:55 |
20 | Introduction to challenges while building, deploying microservices | 04:40 |
21 | What is Containerization technology? | 08:36 |
22 | Definition of containers | 05:51 |
23 | Introduction to Docker & its architecture | 08:12 |
24 | Understanding Docker Hub & Installing Docker | 05:47 |
25 | Creating Docker image definition using a Dockerfile | 09:55 |
26 | Create Accounts microservice Docker image from the Dockerfile | 04:52 |
27 | Start and deploy Accounts microservice using Docker image & containers | 06:39 |
28 | Deep dive of important Docker commands | 10:31 |
29 | Introduction to Buildpacks | 04:34 |
30 | Creating docker image of Loans microservice using Buildpacks | 06:03 |
31 | Creating docker image of Cards microservice using Buildpacks | 06:46 |
32 | Pushing Docker images from your local to remote Docker hub repository | 06:11 |
33 | Deep dive on docker-compose | 14:07 |
34 | Tips & Tricks around Docker Desktop Dashboard & Logs explorer | 05:51 |
35 | Introduction to Cloud-native applications | 08:03 |
36 | Differences between cloud-native Apps & Traditional enterprise Apps | 06:58 |
37 | Twelve factor App Deepdive 1 | 08:14 |
38 | Twelve factor App Deepdive 2 | 06:56 |
39 | Twelve factor App Deepdive 3 | 08:39 |
40 | Introduction to Configurations Management challenges inside microservices | 04:51 |
41 | Configuration Management architecture inside microservices | 06:53 |
42 | Deep dive of Spring Cloud Config for Configuration management | 06:16 |
43 | Building Config Server service and load all the configurations from classpath | 16:01 |
44 | Reading configurations from a file system location | 04:00 |
45 | Reading configurations from a GitHub repository | 04:42 |
46 | Updating Accounts Microservice to read properties from Config Server | 12:21 |
47 | Updating Loans Microservice to read properties from Config Server | 03:49 |
48 | Updating Cards Microservice to read properties from Config Server | 04:25 |
49 | Generating Docker images after Config Server changes | 07:22 |
50 | Pushing all the latest Docker images with Config server changes to DockerHub | 04:28 |
51 | Updating Docker Compose file to adapt Config Server changes | 16:11 |
52 | Starting all the microservices using docker compose files based on the env | 13:13 |
53 | Refreshing properties with @RefreshScope | 12:34 |
54 | Encryption & Decryption of your properties inside Config server | 07:54 |
55 | Introduction to the Service Discovery & Registration inside microservices | 08:20 |
56 | Why not traditional load balancers for Microservices | 08:33 |
57 | Architecture of Service Discovery inside microservices | 12:51 |
58 | Client Side load balancing between microservices | 06:23 |
59 | Spring Cloud support for Service Discovery & Registration | 04:26 |
60 | Setup Service Discovery agent using Eureka server | 09:37 |
61 | Make changes for Accounts microservice to connect Eureka Server | 07:16 |
62 | Make changes for Loans & Cards microservice to connect Eureka Server | 07:40 |
63 | Degistration from Eureka server when microservices shutdown | 04:43 |
64 | Demo of heartbeats mechanism to Eureka server from clients | 02:29 |
65 | Feign Client to invoke other microservices | 12:13 |
66 | Generating Docker images after Service Discovery changes | 05:02 |
67 | Pushing all the latest Docker images with Eureka changes to Docker Hub | 02:22 |
68 | Updating Docker Compose file to adapt Service Discovery changes | 04:19 |
69 | Starting all the microservices using docker compose file | 04:29 |
70 | Running docker compose with 2 instances of Accounts microservice | 05:26 |
71 | Eureka Self-Preservation mode to avoid network trap issues | 12:40 |
72 | Introduction to the need of Resiliency inside microservices | 10:03 |
73 | Typical use case or scenario for the need of Resiliency | 07:05 |
74 | Deep dive on Circuit Breaker pattern in microservices | 13:32 |
75 | Implementing Circuit Breaker pattern - Part 1 | 07:30 |
76 | Implementing Circuit Breaker pattern - Part 2 | 07:16 |
77 | Implementing Circuit Breaker pattern - Part 3 | 05:29 |
78 | Deep dive on Retry pattern in microservices | 03:30 |
79 | Implementing Retry Pattern in microservices | 06:57 |
80 | Deep dive on Rate Limiter pattern in microservices | 03:17 |
81 | Implementing Rate Limiter Pattern in microservices | 03:33 |
82 | Deep dive on Bulk head pattern in microservices | 07:23 |
83 | Introduction to the challenges with Routing & Cross cutting concerns | 05:14 |
84 | Introduction to Spring Cloud Gateway | 11:03 |
85 | Deep dive on Spring Cloud Gateway internal architecture | 06:19 |
86 | Building Spring Cloud Gateway service | 15:26 |
87 | Implementing Custom Routing using Spring Cloud Gateway | 10:08 |
88 | Implementing Cross cutting concern Tracing & Logging using Gateway Server | 15:24 |
89 | Generating and pushing Docker images with Spring Cloud Gateway changes | 08:42 |
90 | Updating Docker Compose file to adapt Spring Cloud Gateway changes | 07:32 |
91 | Introduction to the challenges related to Distributed tracing & Log aggregation | 08:42 |
92 | Introduction to Spring Cloud Sleuth & Zipkin | 04:32 |
93 | Deep dive on Spring Cloud Sleuth & it's tracing format | 07:06 |
94 | Deep dive on Zipkin internal architecture | 07:27 |
95 | Implementing Distributed tracing with Spring Cloud Sleuth | 10:05 |
96 | Implementing Log aggregation with Zipkin Server | 14:28 |
97 | Pushing Sleuth message into RabbitMQ | 10:25 |
98 | Generate, Push Docker images with Sleuth & Zipkin changes | 12:51 |
99 | Introduction to the challenges related to monitoring microservices | 02:52 |
100 | Different approaches to monitor microservices | 10:27 |
101 | Setup of micrometer inside microservices | 10:19 |
102 | Setup of Prometheus to monitor microservices | 15:31 |
103 | Setup of Grafana to monitor microservices with inbuilt dashboards | 10:07 |
104 | Building custom dashboards inside Grafana | 05:44 |
105 | Sending alerts using Grafana when service is down | 08:46 |
106 | Introduction to the challenges related to container orchestration | 05:28 |
107 | Introduction to Kubernetes | 08:22 |
108 | Dee dive of Kubernetes internal architecture | 17:10 |
109 | Cloud providers support for Kubernetes | 07:40 |
110 | GCP Account Setup and creating a K8s cluster | 04:46 |
111 | Exploring K8S cluster and establish connection with it | 11:26 |
112 | Deep dive on Kubernetes YAML configurations | 09:03 |
113 | Kubernetes YAML configurations for applicable microservices | 13:18 |
114 | Create environment variables inside K8S cluster using ConfigMap | 09:17 |
115 | Deploying our microservices to Kubernetes cluster | 12:14 |
116 | Validating our microservices deployed into K8s cluster | 04:08 |
117 | Automatic Self healing inside Kubernetes cluster | 06:34 |
118 | Automatic Rollout & Rollback inside Kubernetes cluster | 10:50 |
119 | Logging & Monitoring inside Kubernetes cluster | 05:12 |
120 | Autoscaling inside Kubernetes cluster using HPA | 04:06 |
121 | Deleting Kubernetes cluster inside GCP | 01:27 |
122 | Deploying all the microservices into K8s cluster - Theory | 05:13 |
123 | Creating the K8s yaml config files for all microservices | 10:23 |
124 | How Deployment and Service are tied together inside K8s | 05:41 |
125 | Deploying all the microservices into K8s cluster | 08:05 |
126 | Validating microservices deployed into K8s cluster | 03:18 |
127 | Problems with manually created Kubernetes manifest files | 07:41 |
128 | Introduction to Helm | 05:50 |
129 | Problems that Helm solves | 09:24 |
130 | Installing Helm | 06:18 |
131 | Creating our first Helm Chart | 06:16 |
132 | Installing the Default Helm chart into K8s cluster | 14:48 |
133 | Exploring the default Helm chart content | 09:12 |
134 | Creating our own Helm template files | 15:47 |
135 | Creating Helm chart for Accounts microservice | 11:12 |
136 | Creating Helm chart for other microservice | 13:07 |
137 | Creating Helm chart for Dev and Prod environment | 13:06 |
138 | Demo of helm template command | 06:01 |
139 | Installing Helm charts into K8s cluster | 11:44 |
140 | Demo of helm upgrade command | 06:03 |
141 | Demo of helm history and rollback commands | 03:41 |
142 | Demo of helm uninstall command | 02:33 |
143 | Revision of important helm commands | 06:50 |
144 | Problem with Kubernetes LoadBalancer Service | 05:02 |
145 | Problem with Kubernetes LoadBalancer Service-Demo | 05:33 |
146 | Introduction to types of K8s Services | 04:21 |
147 | Deep dive on ClusterIP Service - Theory | 04:27 |
148 | Deep dive on ClusterIP Service - Demo | 11:43 |
149 | Deep dive on NodePort Service - Theory | 04:38 |
150 | Deep dive on NodePort Service - Demo | 12:04 |
151 | Deep dive on LoadBalancer Service - Theory | 04:28 |
152 | Deep dive on LoadBalancer Service - Demo | 07:06 |
153 | Introduction to securing Spring Cloud Gateway with OAuth2 | 02:59 |
154 | Quick intro to OAuth2 framework | 11:04 |
155 | Deep dive on OAuth2 Client Credentials grant flow | 10:42 |
156 | KeyCloak Auth Server installation and setup using Docker command | 07:55 |
157 | Register Client details inside KeyCloak Auth server | 05:24 |
158 | Getting Access token from Auth Server using Client details | 08:32 |
159 | Making code changes inside Spring Cloud Gateway to secure the APIs | 13:10 |
160 | Demo of Spring Cloud Gateway security inside local system | 09:03 |
161 | Generating and Pushing latest docker image of Gateway into Docker Hub | 03:11 |
162 | Installation of KeyCloak into K8s cluster using Helm chart | 12:43 |
163 | Updating Helm charts of microservices | 07:46 |
164 | Deploying all microservices into K8s and validating security changes | 05:53 |
165 | Introduction to Authorization | 04:38 |
166 | Demo of Authorization changes using Spring Security - Part 1 | 10:42 |
167 | Demo of Authorization changes using Spring Security - Part 2 | 06:36 |
168 | Introduction to OAuth2 Authorization code grant flow | 06:02 |
169 | Deep dive of OAuth2 Authorization code grant flow | 07:36 |
170 | Making code changes inside Accounts microservice to secure the APIs | 12:25 |
171 | Register Client details inside KeyCloak Auth server for Spring Cloud Gateway | 05:57 |
172 | Making code changes inside Spring Cloud Gateway | 12:42 |
173 | Demo of OAuth2 Authorization code grant flow inside local system | 11:37 |
174 | Updating Helm charts of microservices | 14:03 |
175 | Deploy all microservices into K8s cluster and demo of Authorization code flow | 13:15 |
176 | Introduction to Kubernetes Ingress | 09:51 |
177 | Introduction to Service mesh | 07:03 |
178 | Deep dive on Service mesh and Istio | 10:52 |
179 | Thank You & Congratulations | 01:58 |
Similar courses to Master Microservices with Java, Spring, Docker, Kubernetes
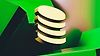
RESTful Web Services, Java, Spring Boot, Spring MVC and JPAudemy
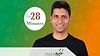
Learn Java Unit Testing with Junit & Mockito in 30 Stepsudemy
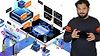
Kubernetes Certified Application Developer (CKAD) with Testsudemy
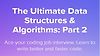
The Ultimate Data Structures & Algorithms: Part 2codewithmosh (Mosh Hamedani)
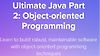
Ultimate Java Part 2: Object-oriented Programmingcodewithmosh (Mosh Hamedani)
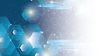
Java Multithreading, Concurrency & Performance Optimizationudemy
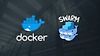
Docker Swarm Mastery: DevOps Style Cluster Orchestrationudemy
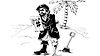
Data Structures in Javajavaspecialists.eu
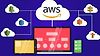
AWS Serverless REST APIs for Java Developers. CI/CD includedudemy
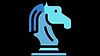