Java Data Structures & Algorithms + LEETCODE Exercises
9h 47m 55s
English
Paid
Welcome to the course "Data Structures and Algorithms in Java"!
This course makes learning programming exciting and simplifies complex concepts for easy understanding.
How did I manage to achieve this? With the help of animation!
Animation of data structures and algorithms makes the material visually accessible and engaging, allowing you to learn more in less time with a higher degree of retention (a great combination).
I will use these visualizations to guide you step by step through the entire course.
The course includes dozens of practical programming exercises so you can immediately apply what you've learned in practice (a very important step).
All of this will help you feel more confident and prepared when you go to a programming interview.
Watch Online Java Data Structures & Algorithms + LEETCODE Exercises
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction | 03:27 |
2 | Overview (Please Watch) | 06:18 |
3 | Code Editor | 09:22 |
4 | Big O: Intro | 02:57 |
5 | Big O: Worst Case | 01:37 |
6 | Big O: O(n) | 01:51 |
7 | Big O: Drop Constants | 01:35 |
8 | Big O: O(n^2) | 02:01 |
9 | Big O: Drop Non-Dominants | 02:09 |
10 | Big O: O(1) | 01:48 |
11 | Big O: O(log n) | 03:45 |
12 | Big O: Different Terms for Inputs | 02:24 |
13 | Big O: Array Lists | 04:55 |
14 | Big O: Wrap Up | 07:03 |
15 | Classes | 06:42 |
16 | Pointers | 05:52 |
17 | Linked List: Intro | 02:43 |
18 | LL: Big O | 06:01 |
19 | LL: Under the Hood | 02:19 |
20 | LL: Constructor | 09:19 |
21 | LL: Print List | 03:02 |
22 | LL: Append | 03:54 |
23 | LL: Remove Last (Intro) | 04:52 |
24 | LL: Remove Last (Code) | 09:33 |
25 | LL: Prepend | 03:44 |
26 | LL: Remove First | 06:28 |
27 | LL: Get | 04:22 |
28 | LL: Set | 04:31 |
29 | LL: Insert | 05:37 |
30 | LL: Remove | 07:17 |
31 | LL: Reverse | 05:50 |
32 | DLL: Constructor | 03:57 |
33 | DLL: Append | 04:13 |
34 | DLL: Remove Last | 07:56 |
35 | DLL: Prepend | 03:57 |
36 | DLL: Remove First | 04:56 |
37 | DLL: Get | 06:34 |
38 | DLL: Set | 03:53 |
39 | DLL: Insert | 05:46 |
40 | DLL: Remove | 06:15 |
41 | Stack: Intro | 05:21 |
42 | Stack: Constructor | 03:48 |
43 | Stack: Push | 03:10 |
44 | Stack: Pop | 02:49 |
45 | Queue: Intro | 02:37 |
46 | Queue: Constructor | 04:31 |
47 | Queue: Enqueue | 03:30 |
48 | Queue: Dequeue | 04:18 |
49 | Trees: Intro & Terminology | 04:24 |
50 | Binary Search Trees: Example | 02:34 |
51 | BST: Big O | 08:03 |
52 | BST: Constructor | 05:55 |
53 | BST: Insert - Intro | 05:33 |
54 | BST: Insert - Code | 09:53 |
55 | BST: Contains - Intro | 03:15 |
56 | BST: Contains - Code | 07:17 |
57 | Hash Table: Intro | 04:41 |
58 | HT: Collisions | 01:49 |
59 | HT: Constructor | 05:19 |
60 | HT: Hash Method | 04:14 |
61 | HT: Set | 05:42 |
62 | HT: Get | 06:10 |
63 | HT: Keys | 03:23 |
64 | HT: Big O | 02:15 |
65 | HT: Interview Question | 07:30 |
66 | Graph: Intro | 03:46 |
67 | Graph: Adjacency Matrix | 02:59 |
68 | Graph: Adjacency List | 01:26 |
69 | Graph: Big O | 07:10 |
70 | Graph: Add Vertex | 05:20 |
71 | Graph: Add Edge | 03:36 |
72 | Graph: Remove Edge | 03:38 |
73 | Graph: Remove Vertex | 06:59 |
74 | Heap: Intro | 06:04 |
75 | Heap: Insert (Intro) | 04:41 |
76 | Heap: Helper Methods | 02:42 |
77 | Heap: Insert (Code) | 06:09 |
78 | Heap: Remove | 05:00 |
79 | Heap: Sink Down | 08:53 |
80 | Heap: Priority Queues & Big O | 04:31 |
81 | Recursion: Intro | 06:59 |
82 | Call Stack | 07:16 |
83 | Factorial | 09:21 |
84 | rBST: Contains | 07:23 |
85 | rBST: Insert | 08:18 |
86 | rBST: Delete Intro | 02:21 |
87 | rBST: Delete Code (1 of 3) | 05:18 |
88 | rBST: Delete Code (2 of 3) | 04:50 |
89 | rBST: Minimum Value | 04:25 |
90 | rBST: Delete Code (3 of 3) | 05:24 |
91 | Tree Traversal: Intro | 01:28 |
92 | BFS (Breadth First Search): Intro | 03:09 |
93 | BFS: Code | 06:16 |
94 | DFS (Depth First Search): PreOrder - Intro | 01:11 |
95 | DFS: PreOrder - Code | 07:01 |
96 | DFS: PostOrder - Intro | 02:14 |
97 | DFS: PostOrder - Code | 05:32 |
98 | DFS: InOrder - Intro | 02:00 |
99 | DFS: InOrder - Code | 05:17 |
100 | Bubble Sort: Intro | 02:38 |
101 | Bubble Sort: Code | 05:38 |
102 | Selection Sort: Intro | 03:39 |
103 | Selection Sort: Code | 05:22 |
104 | Insertion Sort: Intro | 01:44 |
105 | Insertion Sort: Code | 04:39 |
106 | Insertion Sort: Big O | 01:21 |
107 | Merge Sort: Overview | 01:37 |
108 | Merge: Intro | 01:36 |
109 | Merge: Code | 05:37 |
110 | Merge Sort: Intro | 01:59 |
111 | Merge Sort: Code | 11:40 |
112 | Merge Sort: Big O | 03:24 |
113 | Quick Sort: Intro | 02:44 |
114 | Pivot: Intro | 03:31 |
115 | Pivot: Code | 05:36 |
116 | Quick Sort: Code | 06:13 |
117 | Quick Sort: Big O | 03:00 |
118 | Overlapping Subproblems | 04:53 |
119 | Optimized Substructure | 03:28 |
120 | Fibonacci Sequence | 09:58 |
121 | Memoization | 13:05 |
122 | Bottom Up | 07:10 |
Similar courses to Java Data Structures & Algorithms + LEETCODE Exercises
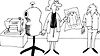
Java Design Patternsjavaspecialists.eu
Category: Java
Duration 16 hours 20 minutes 37 seconds
Course
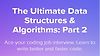
The Ultimate Data Structures & Algorithms: Part 2codewithmosh (Mosh Hamedani)
Category: Others, Java
Duration 5 hours 56 minutes 46 seconds
Course
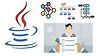
Java Data Structures and Algorithms Masterclassudemy
Category: Java
Duration 44 hours 58 minutes 57 seconds
Course
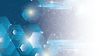
Java Multithreading, Concurrency & Performance Optimizationudemy
Category: Java
Duration 5 hours 16 minutes 23 seconds
Course
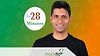
Learn Java Unit Testing with Junit & Mockito in 30 Stepsudemy
Category: Java
Duration 4 hours 44 minutes 35 seconds
Course
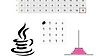
Algorithms in Java: Live problem solving & Design Techniquesudemy
Category: Java
Duration 19 hours 41 minutes 26 seconds
Course
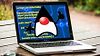
Java Programming Masterclass covering Java 11 & Java 17udemy
Category: Java
Duration 80 hours 13 minutes 14 seconds
Course
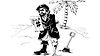
Data Structures in Javajavaspecialists.eu
Category: Java
Duration 8 hours 3 minutes 54 seconds
Course
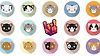
Catsrockthejvm.com
Category: Java
Duration 10 hours 39 minutes 36 seconds
Course
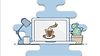
The complete guide to running Java in Docker and Kubernetesudemy
Category: Docker, Java, Kubernetes
Duration 4 hours 39 minutes 16 seconds
Course