Concurrency, Multithreading and Parallel Computing in Java
This course is about the basics of multithreading and concurrent programming with some parallel concepts. In the 21st century this topic is becoming more and more popular with the advent of Big Data and Machine Learning. We will consider the low level concepts such as threads, synchronization and locks. The second chapter will be about concurrent library: of course there are built in classes and interfaces that we can use when implementing multithreaded applications.
Read more about the course
Then we develope little programs as show-cases for multithreading: the dining-philosopher problem or the students in library simulation. Last chapter is about parallel computing and MapReduce.
Section 1 - Multithreading Theory:
theory behind multithreading
pros and cons of multithreading
life cycle of a thead
Section 2 - Threads Manipulation:
starting threads (Runnable interface and Thread class)
join keyword
daemon threads
Section 3 - Inter-Thread Communication:
memory management of threads
synchronization and synchronized blocks
locks
wait and notify
producer-consumer problem and solution
concurrent collections
latch, cyclic barrier and blocking queues
delay queue, priority queue and concurrent maps
Section 4 - Multithreading Concepts:
volatile keywords
deadlocks and livelocks
semaphores and mutexes
dining philosophers problem
library application
miner game
Section 6 - Executors and ExecutorServices:
executors
executor services
Section 6 - Concurrent Collections:
latches
cyclic barriers
delay and priority queues
concurrent HashMaps
Section 7 - Simulations:
dining philosophers problem
library problem
Section 8 - Parallel Algorithms:
what is parallel computing
parallel merge sort
parallel algorithms
Section 9 - Fork-Join Framework
Fork-Join framework
maximum finding in parallel manner
Section 10 - Stream API
the Stream API explained with examples
sequential streams and parallel streams
Section 11 - BigData and MapReduce:
what is MapReduce
MapReduce and Fork-Join framework
Watch Online Concurrency, Multithreading and Parallel Computing in Java
# | Title | Duration |
---|---|---|
1 | Introduction | 01:37 |
2 | Processes and threads introduction | 06:13 |
3 | What is time-slicing algorithm? | 02:42 |
4 | Benefits of multithreading | 02:21 |
5 | Downside of multithreading | 02:47 |
6 | Thread life cycle in Java | 02:45 |
7 | Sequential processing | 04:23 |
8 | Starting threads - Runnable | 06:29 |
9 | Starting threads - Thread class | 05:28 |
10 | Wait for threads to finish - join | 04:39 |
11 | Daemon threads and user threads | 08:59 |
12 | Memory management of threads | 03:39 |
13 | Synchronization | 07:38 |
14 | Problems with synchronization | 09:44 |
15 | Locking with custom objects | 03:21 |
16 | Wait and notify | 08:39 |
17 | Producer and consumer | 11:10 |
18 | Locks | 06:15 |
19 | Producer consumer with locks | 06:24 |
20 | Locks and synchronization | 01:35 |
21 | Volatile | 06:04 |
22 | Stopping a thread | 01:19 |
23 | Deadlock and livelock | 04:38 |
24 | Deadlock example | 06:21 |
25 | Livelock example | 06:25 |
26 | Atomic variables | 05:56 |
27 | What are semaphores? | 03:52 |
28 | Mutexes and semaphores | 05:29 |
29 | Semaphores example | 07:20 |
30 | Why to use thread pools? | 04:49 |
31 | Executors example - SingleThreadExecutor | 05:22 |
32 | Executors example - FixedThreadPools | 04:12 |
33 | Executors example - ScheduledExecutor | 03:25 |
34 | Stopping executors | 04:56 |
35 | What is a Callable interface and Future object? | 03:25 |
36 | Callable and future example | 07:41 |
37 | Latch | 09:07 |
38 | Cyclic barrier | 09:17 |
39 | Blocking queue | 08:53 |
40 | Delay queue | 09:10 |
41 | Priority queue | 10:30 |
42 | Concurrent maps | 07:26 |
43 | Exchanger | 09:33 |
44 | Dining philosophers problem I - the problem | 02:31 |
45 | Dining philosophers problems II - constants | 03:56 |
46 | Dining philosophers problems III - chopstick | 08:04 |
47 | Dining philosophers problems IV - philosopher | 11:06 |
48 | Dining philosophers problems V - starting the threads | 10:21 |
49 | Dining philosophers problems VI - running the simulation | 03:30 |
50 | Student library simulation I - the problem | 01:25 |
51 | Student library simulation II - constants | 01:51 |
52 | Student library simulation III - book | 03:17 |
53 | Student library simulation VI - student | 02:58 |
54 | Locking: locks () and tryLock() | 02:41 |
55 | Student library simulation V - running the simulation | 04:13 |
56 | Miner game implementation I | 01:44 |
57 | Miner game implementation II | 03:56 |
58 | Miner game implementation III | 02:16 |
59 | Miner game implementation IV | 03:36 |
60 | Miner game implementation V | 05:57 |
61 | Parallel methods versus multithreading | 08:07 |
62 | Merge sort introduction I | 06:51 |
63 | Merge sort introduction II | 08:55 |
64 | Sequential merge sort I | 06:27 |
65 | Parallel merge sort | 06:48 |
66 | Comparing sorting implementations | 04:46 |
67 | Sum problem introduction | 02:45 |
68 | Sum problem - sequential approach | 04:29 |
69 | Sum problem - parallel implementation | 08:27 |
70 | Comparing sum implementations | 03:59 |
71 | Fork-join framework introduction | 05:45 |
72 | Fork-join framework simple example - RecursiveAction | 09:15 |
73 | Fork-join framework simple example - RecursiveTask<T> | 07:57 |
74 | Maximum finding - the algorithm | 08:18 |
75 | Maximum finding - running the application | 06:55 |
76 | Fork-join merge sort I | 08:32 |
77 | Fork-join merge sort II | 05:01 |
78 | What is the Stream API? | 02:46 |
79 | Streams with numbers | 08:55 |
80 | Streams with strings | 03:44 |
81 | Processing files with streams | 04:53 |
82 | Streams with custom objects | 09:41 |
83 | Serial and parallel streams - counting prime numbers | 07:59 |
84 | MapReduce introduction - basics | 06:02 |
85 | MapReduce introduction - example | 06:08 |
86 | MapReduce and Fork-Join | 01:49 |
Similar courses to Concurrency, Multithreading and Parallel Computing in Java
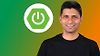
Master Spring Boot 3 & Spring Framework 6 with Javaudemy
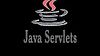
Java Servlets and JSPs developer courseudemy
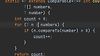
Java Genericsamigoscode (Nelson Djalo)
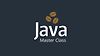
Java Master Classamigoscode (Nelson Djalo)
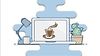
The complete guide to running Java in Docker and Kubernetesudemy
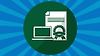
Oracle Java Certification - Pass the Associate 1Z0-808 Exam.udemy
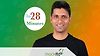
Learn Java Unit Testing with Junit & Mockito in 30 Stepsudemy
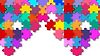
Java Design Patterns & SOLID Design Principlesudemy
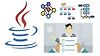
Java Data Structures and Algorithms Masterclassudemy
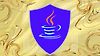