Data Structures in Java
8h 3m 54s
English
Paid
May 1, 2024
Data Structures in Java (Late 2017 Edition) is an action-packed 8 hours of tips and tricks that professional Java programmers have used for the past 20 years to produce code that is robust and fast. Every lecture is followed by a short quiz to test your learning. Sometimes the questions are easy, others require some research on your side. Over 130 quiz questions in total will help you gauge how well you understood the various data structures.
Watch Online Data Structures in Java
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Welcome | 03:09 |
2 | Computational Time Complexity | 23:00 |
3 | Space Complexity | 04:13 |
4 | Arrays | 15:21 |
5 | Lists | 13:23 |
6 | ArrayList | 14:08 |
7 | Iteration | 07:56 |
8 | CopyOnWriteArrayList | 07:04 |
9 | LinkedList | 08:40 |
10 | Vector | 04:11 |
11 | Stack | 05:35 |
12 | Sorting lists | 49:42 |
13 | Sets | 10:07 |
14 | TreeSet | 35:20 |
15 | ConcurrentSkipListSet | 09:44 |
16 | CopyOnWriteArraySet | 18:03 |
17 | Hashing | 16:26 |
18 | HashSet | 13:44 |
19 | ConcurrentHashMap.newKeySet() | 03:29 |
20 | Maps | 02:12 |
21 | HashMap | 28:35 |
22 | ConcurrentHashMap | 06:29 |
23 | TreeMap | 18:43 |
24 | ConcurrentSkipListMap | 09:03 |
25 | Hashtable | 18:16 |
26 | LinkedHashMap and LinkedHashSet | 13:17 |
27 | Highly Specialized Collections: EnumSet, EnumMap, IdentityHashMap, Properties, WeakHashMap | 19:54 |
28 | Queues and Deques | 04:34 |
29 | ConcurrentLinkedQueue and ConcurrentLinkedDeque | 11:30 |
30 | ArrayDeque | 01:51 |
31 | BlockingQueues | 14:13 |
32 | LinkedBlockingQueue and LinkedBlockingDeque | 06:27 |
33 | ArrayBlockingQueue | 16:01 |
34 | Highly specialized queues: DelayQueue, SynchronousQueue, LinkedTransferQueue | 18:05 |
35 | PriorityQueue and PriorityBlockingQueue | 17:36 |
36 | java.util.Collections | 11:03 |
37 | java.util.Arrays | 01:25 |
38 | Conclusion And Where To Next? | 01:25 |
Similar courses to Data Structures in Java
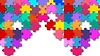
Java Design Patterns & SOLID Design Principles
Duration 17 hours 5 minutes 6 seconds
Course
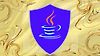
Java Programming Bootcamp: Zero to Mastery
Duration 9 hours 15 minutes 36 seconds
Course
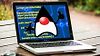
Java Programming Masterclass covering Java 11 & Java 17
Duration 80 hours 13 minutes 14 seconds
Course
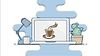
The complete guide to running Java in Docker and Kubernetes
Duration 4 hours 39 minutes 16 seconds
Course
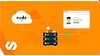
Machine Learning with Javascript
Duration 17 hours 42 minutes 20 seconds
Course
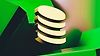
RESTful Web Services, Java, Spring Boot, Spring MVC and JPA
Duration 25 hours 11 minutes 7 seconds
Course
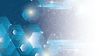
Java Multithreading, Concurrency & Performance Optimization
Duration 5 hours 16 minutes 23 seconds
Course
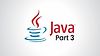
Ultimate Java Part 3: Advanced Topics
Duration 6 hours 28 minutes 19 seconds
Course
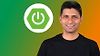
Master Spring Boot 3 & Spring Framework 6 with Java
Duration 37 hours 34 minutes 14 seconds
Course
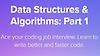
Data Structures & Algorithms: Part 1
Duration 4 hours 39 minutes 17 seconds
Course