The Ultimate Data Structures & Algorithms: Part 2
5h 56m 46s
English
Paid
Data structures and algorithms are patterns for solving problems. Developers who know more about data structures and algorithms are better at solving problems. That’s why companies like Google, Microsoft and Amazon always include interview questions on data structures and algorithms. They want to assess your problem-solving skills. They don't care how many programming languages and frameworks you're familiar with.
Read more about the course
By the end of this course…
You’ll be able to:
- Ace your next coding interview
- Write better, faster code
- Become a better developer
- Improve your problem solving skills
- Master computer science fundamentals
- Implement all the essential data structures from scratch
- Master dozens of popular algorithms
Watch Online The Ultimate Data Structures & Algorithms: Part 2
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction | 00:51 |
2 | Introduction | 00:56 |
3 | What are Trees | 05:37 |
4 | Exercise- Building a Tree | 02:42 |
5 | Solution- insert() | 07:45 |
6 | Solution- find() | 02:01 |
7 | Traversing Trees | 05:59 |
8 | Recursion | 05:40 |
9 | Depth First Traversals | 05:24 |
10 | Depth and Height of Nodes | 07:07 |
11 | Minimum Value in a Tree | 07:38 |
12 | Exercise- Equality Checking | 00:46 |
13 | Solution- Equality Checking | 04:09 |
14 | Exercise- Validating Binary Search Trees | 04:15 |
15 | Solution- Validating Binary Search Trees | 04:19 |
16 | Exercise- Nodes at K Distance | 01:49 |
17 | Solution- Nodes at K Distance from the Root | 04:38 |
18 | Level Order Traversal | 02:56 |
19 | Summary | 01:23 |
20 | Introduction | 00:32 |
21 | Balanced and Unbalanced Trees | 03:02 |
22 | Rotations | 05:03 |
23 | AVL Trees | 04:05 |
24 | Exercise- Building an AVL Tree | 01:12 |
25 | Solution- insert() | 08:48 |
26 | Exercise- Height Calculation | 01:25 |
27 | Solution- Height Calculation | 02:44 |
28 | Exercise- Balance Factor | 02:05 |
29 | Solution- Balance Factor | 04:02 |
30 | Exercise- Detecting Rotations | 02:55 |
31 | Solution- Detecting Rotations | 03:34 |
32 | Exercise- Implementing Rotations | 03:52 |
33 | Solution- Implementing Rotations | 05:41 |
34 | Summary | 01:02 |
35 | Introduction | 00:22 |
36 | What are Heaps | 06:29 |
37 | Exercise- Building a Heap | 01:56 |
38 | Solution- insert() | 08:25 |
39 | Solution- remove() | 07:08 |
40 | Solution - Edge Cases | 06:12 |
41 | Heap Sort | 02:30 |
42 | Priority Queues | 05:05 |
43 | Exercise- Heapify | 01:27 |
44 | Solution- Heapify | 07:13 |
45 | Solution- Optimization | 02:43 |
46 | Exercise- Kth Largest Item | 00:32 |
47 | Solution- Kth Largest Item | 03:48 |
48 | Summary | 01:20 |
49 | Introduction | 00:31 |
50 | What are Tries | 03:51 |
51 | What are Tries | 03:04 |
52 | Solution- Building a Trie | 05:45 |
53 | An Implementation with a HashTable | 01:51 |
54 | A Better Abstraction | 05:29 |
55 | Exercise- Looking Up a Word | 01:13 |
56 | Solution- Looking Up a Word | 02:36 |
57 | Traversals | 03:36 |
58 | Exercise- Removing a Word | 01:54 |
59 | Solution- Removing a Word | 08:15 |
60 | Exercise- Auto Completion | 02:52 |
61 | Solution- Auto Completion | 06:00 |
62 | Summary | 00:46 |
63 | Introduction | 00:27 |
64 | What are Graphs | 02:10 |
65 | Adjacency Matrix | 04:15 |
66 | Adjacency List | 06:33 |
67 | Exercise- Building a Graph | 01:51 |
68 | Solution- Adding Nodes and Edges | 07:35 |
69 | Solution- Removing Nodes and Edges | 04:49 |
70 | Traversal Algorithms | 03:59 |
71 | Exercise- Depth-first Traversal (Recursive) | 01:30 |
72 | Solution- Depth-first Traversal (Recursive) | 03:45 |
73 | Exercise- Depth-first Traversal (Iterative) | 02:45 |
74 | Solution- Depth-first Traversal (Iterative) | 04:00 |
75 | Exercise- Breadth-first Traversal (Iterative) | 01:19 |
76 | Solution- Breadth-first Traversal | 02:42 |
77 | Exercise- Topological Sorting | 05:07 |
78 | Solution- Topological Sort | 04:06 |
79 | Exercise- Cycle Detection (Directed Graphs) | 03:43 |
80 | Solution- Cycle Detection (Directed Graphs) | 06:27 |
81 | Graphs Summary | 01:03 |
82 | Introduction | 00:27 |
83 | Exercise- Weighted Graphs | 01:31 |
84 | Solution- Weighted Graphs | 05:21 |
85 | An Object-oriented Solution | 06:14 |
86 | Dijkstra's Shortest Path Algorithm | 04:36 |
87 | Exercise- Getting the Shortest Distance | 06:09 |
88 | Solution- The Shortest Distance | 05:28 |
89 | Solution- Shortest Path | 07:54 |
90 | Exercise- Cycle Detection (Undirected Graphs) | 02:04 |
91 | Solution- Cycle Detection (Undirected Graphs) | 04:43 |
92 | Minimum Spanning Tree | 01:57 |
93 | Exercise- Prim's Algorithm | 02:46 |
94 | Solution- Prim's Algorithm | 10:40 |
Similar courses to The Ultimate Data Structures & Algorithms: Part 2
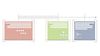
Building an Interpreter from scratchudemyDmitry Soshnikov
Category: Others
Duration 2 hours 59 minutes 53 seconds
Course
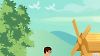
Clean Code Zero to OneShahan Chowdhury
Category: Others
Duration
Book
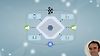
Microservices: Clean Architecture, DDD, SAGA, Outbox & Kafkaudemy
Category: Spring Boot, Others, Java
Duration 18 hours 2 minutes 34 seconds
Course
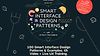
Smart Interface Design Patterns Vitaly Friedmansmashingmagazine.com
Category: Others
Duration 13 hours 18 minutes 5 seconds
Course
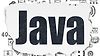
Java Puzzles to Eliminate Code Fearudemy
Category: Preparing for an interview, Java
Duration 7 hours 33 minutes 44 seconds
Course
![Complete Guide to Protocol Buffers 3 [Java, Golang, Python]](https://cdn.courseflix.net/courses/100x56/complete-guide-to-protocol-buffers-3-java-golang-python.jpg?d=1745210617746)
Complete Guide to Protocol Buffers 3 [Java, Golang, Python]udemy
Category: Others
Duration 3 hours 53 minutes 59 seconds
Course
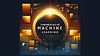
Machine Learning FundamentalsLunarTech
Category: Others
Duration 4 hours 5 minutes 9 seconds
Course
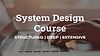
System Design Courseget.interviewready.io (Gaurav Sen)
Category: Others, Preparing for an interview
Duration 92 hours 26 minutes 21 seconds
Course
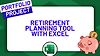
Create a Retirement Planning Tool with Excelzerotomastery.io
Category: Others
Duration 2 hours 51 minutes 33 seconds
Course
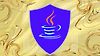
Java Programming Bootcamp: Zero to Masteryzerotomastery.io
Category: Java
Duration 9 hours 15 minutes 36 seconds
Course