Data Structures & Algorithms: Part 1
4h 39m 17s
English
Paid
Studied Computer Science - but never really understood the complex topic of data structures and algorithms? Or maybe you’re a self-taught programmer, with little to no knowledge of this important topic. Or perhaps you failed a job interview because you couldn't answer basic data structure and algorithm questions. Data structures and algorithms are patterns for solving problems. Developers who know more about data structures and algorithms are better at solving problems.
Read more about the course
That’s why companies like Google, Microsoft and Amazon, always include interview questions on data structures and algorithms. They want to assess your problem-solving skills. They don't care how many programming languages and frameworks you're familiar with
Watch Online Data Structures & Algorithms: Part 1
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Course Outline | 02:53 |
2 | Introduction | 00:25 |
3 | What is Big O | 01:59 |
4 | O(1) | 01:30 |
5 | O(n) | 03:45 |
6 | O(n^2) | 02:25 |
7 | O(log n) | 02:40 |
8 | O(2^n) | 00:51 |
9 | Space Complexity | 02:33 |
10 | Introduction | 00:45 |
11 | Understanding Arrays | 03:09 |
12 | Working with Arrays in Java | 03:30 |
13 | Exercise- Array Class | 02:52 |
14 | Solution- Creating the Class | 03:20 |
15 | Solution- insert() | 04:20 |
16 | Solution- removeAt() | 04:52 |
17 | Solution- indexOf() | 02:29 |
18 | Dynamic Arrays | 03:49 |
19 | Summary | 01:10 |
20 | Introduction | 00:37 |
21 | What are Linked Lists | 04:34 |
22 | Working with Linked Lists | 03:25 |
23 | Exercise- Building a Linked List | 01:26 |
24 | Solution- addLast | 06:10 |
25 | Solution- addFirst | 02:14 |
26 | Solution- indexOf | 01:55 |
27 | Solution- contains | 01:06 |
28 | Solution- removeFirst | 04:24 |
29 | Solution- removeLast | 04:41 |
30 | Implementing size() | 04:16 |
31 | Converting Linked Lists to Arrays | 02:11 |
32 | Cheat Sheets | 01:13 |
33 | Arrays vs Linked Lists | 03:22 |
34 | Types of Linked Lists | 03:15 |
35 | Exercise- Reversing a Linked List | 01:34 |
36 | Solution- Reversing a Linked List | 09:01 |
37 | Exercise- Kth Node from the End | 03:21 |
38 | Solution- Kth Node from the End | 05:23 |
39 | Summary | 01:13 |
40 | Introduction | 00:32 |
41 | What are Stacks | 02:48 |
42 | Working with Stacks | 02:22 |
43 | Exercise- Reversing a String | 00:42 |
44 | Solution - Reversing a String | 05:02 |
45 | Exercise- Balanced Expressions | 02:55 |
46 | Solution- A Basic Implementation | 05:18 |
47 | Solution- Supporting Other Brackets | 03:37 |
48 | Solution- First Refactoring | 04:10 |
49 | Solution- Second Refactoring | 05:51 |
50 | Exercise- Building a Stack Using an Array | 00:48 |
51 | Solution- Implementing a Stack Using an Array | 08:19 |
52 | Summary | 00:34 |
53 | Introduction | 00:27 |
54 | What are Queues | 02:05 |
55 | Queues in Java | 05:13 |
56 | Exercise- Reversing a Queue | 01:07 |
57 | Solution- Reversing a Queue | 02:18 |
58 | Exercise- Building a Queue Using an Array | 02:04 |
59 | Solution- A Basic Implementation | 06:33 |
60 | Solution- Circular Arrays | 05:55 |
61 | Exercise- Implementing a Queue Using a Stack | 00:56 |
62 | Solution- Building a Queue Using a Stack | 07:44 |
63 | Priority Queues | 01:54 |
64 | Exercise- Building a Priority Queue | 03:58 |
65 | Solution- Building a Priority Queue | 08:52 |
66 | Solution- Refactoring | 03:03 |
67 | Summary | 00:58 |
68 | Introduction | 00:27 |
69 | What are Hash Tables | 02:45 |
70 | Working with Hash Tables | 06:07 |
71 | Exercise- Find the First Non-repeated Character | 00:55 |
72 | Solution- First Non-repeating Character | 07:40 |
73 | Sets | 02:24 |
74 | Exercise- First Repeated Character | 00:33 |
75 | Solution- First Repeated Character | 02:37 |
76 | Hash Functions | 05:56 |
77 | Collisions | 01:07 |
78 | Chaining | 01:41 |
79 | Open Addressing- Linear Probing | 02:42 |
80 | Open Addressing- Quadratic Probing | 01:30 |
81 | Open Addressing- Double Hashing | 03:20 |
82 | Exercise- Build a HashTable | 02:37 |
83 | Solution- put() | 06:08 |
84 | Solution- get() | 04:30 |
85 | Solution- remove() | 02:31 |
86 | Solution- Refactoring | 11:05 |
87 | Summary | 01:26 |
88 | Course Wrap Up | 00:33 |
Similar courses to Data Structures & Algorithms: Part 1
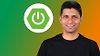
Master Spring Boot 3 & Spring Framework 6 with Javaudemy
Category: React.js, Spring Boot, Java, Hibernate ORM, Maven, Spring, Spring Security
Duration 37 hours 34 minutes 14 seconds
Course
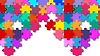
Java Design Patterns & SOLID Design Principlesudemy
Category: Java
Duration 17 hours 5 minutes 6 seconds
Course
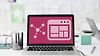
Data Structures and Algorithms: Deep Dive Using Javaudemy
Category: Java
Duration 15 hours 53 minutes 4 seconds
Course
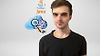
Java Test Automation Engineer - from Zero to Heroudemy
Category: Java, Other (QA)
Duration 18 hours 40 minutes 6 seconds
Course
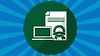
Oracle Java Certification - Pass the Associate 1Z0-808 Exam.udemy
Category: Java
Duration 20 hours 8 minutes 36 seconds
Course
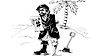
Data Structures in Javajavaspecialists.eu
Category: Java
Duration 8 hours 3 minutes 54 seconds
Course
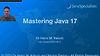
Mastering Java 17javaspecialists.eu
Category: Java
Duration 8 hours 20 minutes 27 seconds
Course
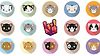
Catsrockthejvm.com
Category: Java
Duration 10 hours 39 minutes 36 seconds
Course
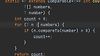
Java Genericsamigoscode (Nelson Djalo)
Category: Java
Duration 1 hour 8 minutes 39 seconds
Course