Java Programming Bootcamp: Zero to Mastery
9h 15m 36s
English
Paid
May 17, 2024
Learn Java from scratch with an industry expert. You'll learn Java programming fundamentals all the way to advanced skills and reinforce your learning with over 80 exercises and 18 quizzes. The only course you need to go from complete programming beginner to being able to get hired as a Backend Developer!
Watch Online Java Programming Bootcamp: Zero to Mastery
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Java Programming Bootcamp: Zero to Mastery | 02:12 |
2 | What is Java? | 04:17 |
3 | When Do We Need Java? Backend vs. Frontend | 03:12 |
4 | Time To Get Your Hands Dirty - Your First Java Program | 04:51 |
5 | Creating Classes | 06:41 |
6 | Using Classes to Create Objects | 03:32 |
7 | Structuring the Application with Packages | 05:32 |
8 | Adding Class Members to Classes | 07:07 |
9 | Understanding the Application Structure | 01:49 |
10 | Understanding Variables | 02:31 |
11 | Working with Variables in Java | 02:35 |
12 | Working with Primitives | 05:54 |
13 | Sizes of Primitives | 02:44 |
14 | Performing Operations with Operators | 01:31 |
15 | Arithmetic Operators | 04:31 |
16 | Assignment Operators | 02:08 |
17 | Unary Operators | 04:22 |
18 | Relational Operators | 02:13 |
19 | Storing Text Variables in Strings | 07:16 |
20 | Understanding Casting | 02:44 |
21 | Casting in Java | 05:09 |
22 | Storing Multiple Values in One Variable | 03:23 |
23 | Working with Arrays | 02:05 |
24 | Primitives vs. Objects | 03:52 |
25 | Objects Containing Primitives | 01:10 |
26 | Objects Containing Objects | 02:24 |
27 | Understanding the Stack and Heap | 04:48 |
28 | The Meaning of Pass by Value | 04:59 |
29 | If Statement | 03:17 |
30 | Demo: If Statement | 06:28 |
31 | Switch Statement | 03:52 |
32 | Demo: Switch Statement | 06:46 |
33 | While Loop | 02:54 |
34 | Demo: While Loop | 04:00 |
35 | Do While Loop | 02:11 |
36 | Demo: Do While Loop | 02:13 |
37 | For Loop | 03:10 |
38 | Demo: For Loop | 04:13 |
39 | Foreach Loop | 01:59 |
40 | Demo: Foreach Loop | 01:50 |
41 | Controlling Loops with Break and Continue | 05:49 |
42 | The Syntax of a Basic Method | 02:37 |
43 | Adding Input Parameters to Methods | 02:44 |
44 | Adding the Return Type and the Return Statement | 02:20 |
45 | Invoking Different Methods | 03:41 |
46 | Exercise: Imposter Syndrome | 02:57 |
47 | Understanding Access Modifiers | 02:07 |
48 | Public Access Modifier | 01:43 |
49 | Demo: Public | 02:15 |
50 | Private Access Modifier | 03:07 |
51 | Demo: Private | 02:18 |
52 | Default Access (No Modifier) | 02:18 |
53 | Demo: Default | 01:26 |
54 | Protected Access Modifier | 01:55 |
55 | Demo: Protected | 01:52 |
56 | Static Modifier | 04:37 |
57 | Demo: Static | 03:15 |
58 | String Class | 01:06 |
59 | Methods on the String Class | 05:42 |
60 | Understanding String Immutability | 06:20 |
61 | Comparing Strings | 05:05 |
62 | Working with StringBuilder | 04:42 |
63 | LocalDate | 05:07 |
64 | LocalTime | 03:14 |
65 | LocalDateTime | 04:32 |
66 | ZonedDateTime | 05:35 |
67 | Duration and Period | 05:02 |
68 | Calculating with Dates and Times | 04:14 |
69 | Formatting and Parsing Dates | 10:02 |
70 | Introduction to OOP | 03:37 |
71 | OOP Pillar 1: Inheritance | 05:48 |
72 | OOP Pillar 2: Encapsulation | 04:23 |
73 | OOP Pillar 3: Abstraction | 01:54 |
74 | OOP Pillar 4: Polymorphism | 07:17 |
75 | Overriding, Overloading and Hiding | 06:56 |
76 | Constructors Explained | 02:41 |
77 | Default Constructor | 02:19 |
78 | Custom Constructor | 03:07 |
79 | Using super() and this() | 05:36 |
80 | Constructors and Inheritance | 05:35 |
81 | What Are Enums? | 01:46 |
82 | Creating and Using Basic Enums | 03:23 |
83 | Enums with Members | 03:53 |
84 | How Enums Work Beneath the Surface | 04:35 |
85 | Understanding Interfaces | 04:20 |
86 | Creating Interfaces | 03:07 |
87 | Implementing Interfaces | 03:19 |
88 | Interfaces with Default Methods | 03:15 |
89 | Interfaces with Static and Private Methods | 02:51 |
90 | Implementing Interfaces with Conflicting Method Signatures | 02:46 |
91 | Abstract Classes and Abstract Methods | 06:08 |
92 | Final Keyword | 02:39 |
93 | Understanding Generics | 04:51 |
94 | Using Classes with Generics | 03:38 |
95 | Collection Framework: List | 06:17 |
96 | Collection Framework: Set | 03:13 |
97 | Collection Framework: Queue | 05:18 |
98 | Collection Framework: Map | 06:54 |
99 | Understanding Exceptions | 01:42 |
100 | Throwing Exceptions | 04:09 |
101 | Checked and Unchecked Exceptions | 04:47 |
102 | Handling Exceptions: Throws | 04:31 |
103 | Handling Exceptions: Try/Catch | 03:13 |
104 | Handling Exceptions: Try with Resources | 05:28 |
105 | Creating Custom Exceptions | 06:30 |
106 | Reading and Writing Files | 02:11 |
107 | Reading Files with FileReader | 04:51 |
108 | Writing to Files with FileWriter | 04:06 |
109 | Getting Started with Lambda Expressions | 01:54 |
110 | Functional Interfaces | 03:11 |
111 | Understanding Lambda Expressions | 02:46 |
112 | Writing Lambda Expression | 09:06 |
113 | Lambda Expressions as Arguments | 08:30 |
114 | Scope and Lambda Expressions | 04:31 |
115 | Built-in Functional Interfaces | 08:39 |
116 | Shorthand Lambda Expression: Method Reference | 08:54 |
117 | Understanding Streams | 02:46 |
118 | Stream API: Source Operations | 04:54 |
119 | Stream API: Terminal Operations | 10:40 |
120 | Stream API: Intermediate Operations | 04:20 |
121 | Using the Stream API: Practical Examples | 03:19 |
122 | Different Parts of the Memory: Heap, Stack, Metaspace | 06:27 |
123 | The Automatic Garbage Collection Process | 03:19 |
124 | Understanding StackOverflowError and OutOfMemoryError | 02:22 |
125 | Understanding Concurrency and Multithreading | 03:55 |
126 | Working with Threads | 07:22 |
127 | Atomic Classes | 04:11 |
128 | Synchronized | 04:37 |
129 | Lock Interface | 05:14 |
130 | Concurrent Collections | 04:55 |
131 | ExecutorService and Thread Pools | 05:35 |
132 | Common Problems in Multithreading | 09:34 |
133 | Using Scanner for Interactive Console Apps | 04:16 |
134 | Thank You | 01:18 |
Similar courses to Java Programming Bootcamp: Zero to Mastery
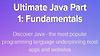
Ultimate Java Part 1: Fundamentals
Duration 3 hours 21 minutes 58 seconds
Course
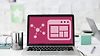
Data Structures and Algorithms: Deep Dive Using Java
Duration 15 hours 53 minutes 4 seconds
Course
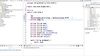
Building Your First App with Spring Boot and Angular
Duration 2 hours 22 minutes 15 seconds
Course
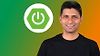
Master Spring Boot 3 & Spring Framework 6 with Java
Duration 37 hours 34 minutes 14 seconds
Course
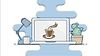
The complete guide to running Java in Docker and Kubernetes
Duration 4 hours 39 minutes 16 seconds
Course
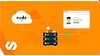
Machine Learning with Javascript
Duration 17 hours 42 minutes 20 seconds
Course
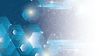
Java Multithreading, Concurrency & Performance Optimization
Duration 5 hours 16 minutes 23 seconds
Course
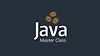
Java Master Class
Duration 24 hours 40 minutes 37 seconds
Course
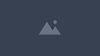
The Complete Java Developer
Duration 40 hours 41 minutes 12 seconds
Course
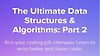
The Ultimate Data Structures & Algorithms: Part 2
Duration 5 hours 56 minutes 46 seconds
Course