RESTful Web Services, Java, Spring Boot, Spring MVC and JPA
This video course also covers the H2 in-memory database and teaches how to build RESTful Web Service that stores data in a database without a need to install MySQL or any other database server. You will also learn how to use H2 console to preview data stored in an in-memory database tables or in a MySQL database server. When it comes to storing data in a database you will learn how to use Spring Data JPA Query Methods as well as Native SQL Queries.
Read more about the course
This is a very practical video course aimed at beginner Java developers to help them build their very first RESTful Web Service application with Java, Spring MVC, Spring Boot and Spring Data JPA to implement features like:
User sign-up and User sign-in,
Email verification,
Password Reset,
Get user details API call,
Get list of users API call and implement Pagination,
Update user details and Delete user details API calls,
Learn to secure Web Service endpoints with Spring Security,
Learn to use Amazon AWS Simple Email Service(SES) to send and request to confirm user email address,
and learn to generate and include the JSON Web Token in HTTP Request.
Students will learn how to use Postman HTTP client software to send: HTTP POST, GET, DELETE and PUT requests, as well as how to set needed HTTP Headers. We will also cover how to:
Send data to your RESTful WebService via HTTP Body and,
How to pass data via URL Query String and as a Path parameter,
How to enable your RESTful Web Service to respond to Cross Origin AJAX HTTP Requests.
You will also learn how to make your RESTful Web Service accept and respond back with JSON or XML media type.
This video course you will learn how to:
Download and install Spring Tool Suite(STS),
Download and install MySQL server and MySQL Workbench GUI,
Download and run Apache Tomcat,
Add a specific Apache Tomcat Version to your Spring Tool Suite,
User Maven to build and run your project as well as package it into a deployable WAR file,
You will also learn how to run your Web Service as a stand along Java application with build-in Tomcat,
Deploy your RESTful Web Service to Apache Tomcat as well as,
Start up your own Amazon AWS EC2 Service in Amazon Cloud, install Java, MySQL, Tomcat and deploy RESTful Web Service to your very own cloud server.
You will also learn how to use JUnit 5 to test your code and how to use one of the most popular test frameworks called Rest Assured to test RESTful Web Service endpoints of your REST Api.
- Basic knowledge of Java
- Mac computer
- Beginner Java developers
- Java developers interested in learning how to Build, Deploy RESTful Web Services
- Java developers interested in learning how to Test Rest API with Rest Assured framework
What you'll learn:
- Build a RESTful Web Service with Spring MVC and Spring Boot
- Learn how to implement User Sign-in functionality
- Learn how to implement User Sign-up functionality
- Protect RESTful Web Service with Spring Security Framework
- Learn how to implement Token-Based Authentication
- Implement Password Reset and Email Verification features
- Use Spring Data JPA Query Methods
- Use Spring Data JPA Native SQL Queries
- Download and Install MySQL Server on Mac
- Download and Install MySQL Workbench
- Download and Install Spring Tool Suite
- Create new project using Spring Tool Suite and Spring Initializer
- Download, run and stop Apache Tomcat
- Deploy RESTful Web Service application to Apache Tomcat
- Run RESTful Web Service application as a stand along Java application
- Build and run REST API with Maven
- Use Postman HTTP client to send HTTP Request to a Web Service endpoints
- Start up Amazon EC2 server
- Install Java, MySQL and Apache Tomcat on Amazon AWS EC2 server
- Deploy RESTful Web Service to Amazon AWS EC2 server
- Use H2 in-memory database
- Use H2 database console to preview data in memory database as well as in a stand along MySQL database server
- Use JUnit 5 to test code
- Use Rest Assured to test RESTful Web Service endpoint
Watch Online RESTful Web Services, Java, Spring Boot, Spring MVC and JPA
# | Title | Duration |
---|---|---|
1 | Install HTTP client Postman | 02:10 |
2 | Postman overview | 10:42 |
3 | Resource and Collection URIs | 11:13 |
4 | HTTP Methods: GET, POST, DELETE and PUT | 03:45 |
5 | HTTP Headers: Accept and Content Type | 04:14 |
6 | Introduction to Web Service Application Layers | 04:30 |
7 | A few suggestions | 03:24 |
8 | Downloading and Installing MySQL on MAC | 03:17 |
9 | Start MySQL Server and Login | 06:15 |
10 | Creating MySQL Database and a new User | 06:26 |
11 | Downloading and Installing MySQL Workbench | 03:03 |
12 | Connect to MySQL Database using MySQL WorkBench | 05:28 |
13 | MySQL WorkBench brief overview | 04:16 |
14 | Install Java Platform (JDK) | 05:49 |
15 | Download and Install Spring Tool Suite(STS) | 01:57 |
16 | Create new Spring Boot Project with Spring Tool Suite | 06:13 |
17 | Creating a new Spring project using Spring Boot Initializr | 04:43 |
18 | Create Users Rest Controller class | 02:46 |
19 | Adding Methods to Handle POST, GET, PUT, DELETE HTTP requests | 03:14 |
20 | Running Web Service Application | 04:37 |
21 | Update POM.XML file | 06:17 |
22 | Configure MySQL Database Access Details | 04:27 |
23 | Adding method to handle HTTP Post Request | 02:21 |
24 | Implementing the Create User Request Model class | 03:22 |
25 | Implementing Create User Response Model | 06:06 |
26 | Implementing the UserDto | 04:39 |
27 | Making use of UserRest and UserDto in RestController | 03:20 |
28 | Implementing Service class method | 03:58 |
29 | Implementing UserEntity class | 06:14 |
30 | Set Default Value for Email Verification Status field | 01:08 |
31 | Implementing UsersRepository class | 05:33 |
32 | Autowire UserRepository into Service class | 05:01 |
33 | Trying how the User Sign up works | 06:13 |
34 | Preventing Duplicate Entries with @Column(unique=true) | 03:47 |
35 | Check if user already exists | 06:20 |
36 | Generate User Public ID | 06:31 |
37 | Adding Spring Security to our project | 05:23 |
38 | Encrypt User Password | 02:54 |
39 | Make the Sign-up Web Service Endpoint Public | 08:52 |
40 | Migrating from WebSecurityConfigurerAdapter | 13:40 |
41 | Implementing User Sign-in Request Model | 01:57 |
42 | [Updated] Implementing loadUserByUsername() | 05:45 |
43 | [Updated] Implementing SecurityConstants class | 04:35 |
44 | [Updated]Adding Maven Dependencies to generate and validate JWT | 02:35 |
45 | [Updated] AuthenticationFilter Part 1. Implementing attemptAuthentication(). | 05:55 |
46 | [Updated] AuthenticationFilter Part 2. Implementing successfulAuthenticatio(). | 03:49 |
47 | [Updated] AuthenticationFilter Part 3. Register with HttpSecurity. | 01:40 |
48 | Trying how user Authentication/Login works | 06:59 |
49 | [Updated] Add UserId to HTTP Response Header | 11:52 |
50 | [Updated]Customize Login URL Path | 02:34 |
51 | [Updated] Implementing Authorization Filter. | 10:33 |
52 | Trying how User Authorization works | 04:18 |
53 | [Updated] Reading Token Secret from a properties file | 05:58 |
54 | Get User Details Resource Method | 05:06 |
55 | Implement Service layer method | 03:09 |
56 | Update UserRepository | 04:00 |
57 | Trying the Get User Details API Call | 05:38 |
58 | Update POM.XML | 01:15 |
59 | Responding with XML or JSON | 07:20 |
60 | Consuming XML or JSON | 04:26 |
61 | Introduction | 02:41 |
62 | Implementing ErrorMessages enum | 05:40 |
63 | Implementing UserServiceException | 03:15 |
64 | Handle a Specific Exception | 06:57 |
65 | Return Custom Error Object Representation | 04:14 |
66 | Handle All Other Exceptions | 04:57 |
67 | Update User Details Resource Method | 05:49 |
68 | Implementing Service Layer Method | 05:39 |
69 | Trying the Update User Details API Call | 02:55 |
70 | Delete User Resource Method | 06:05 |
71 | Implementing Service Layer Method | 02:45 |
72 | Trying the Delete User API Call | 04:55 |
73 | The Get Users Request URL | 03:40 |
74 | The Get Users Resource Method | 05:39 |
75 | Get Users Service Layer Method | 06:02 |
76 | Trying the Get Users API Call | 05:53 |
77 | Running Your Web Services App without STS | 02:44 |
78 | Create Context Path to Your Web Service | 04:23 |
79 | Run Your App as a Java application | 04:35 |
80 | Generating WAR file | 04:26 |
81 | Install Apache Tomcat on Windows | 13:32 |
82 | Downloading Apache Tomcat on Mac | 02:14 |
83 | Starting and Stopping Apache Tomcat | 02:52 |
84 | Creating a new Apache Tomcat User | 02:40 |
85 | Deploying Your Web Service to Apache Tomcat | 04:42 |
86 | Introduction | 02:50 |
87 | [Updated] Startup your own Linux virtual server in Amazon EC2 | 12:46 |
88 | Configure Virtual Server Firewall Rules(Security Group) | 03:48 |
89 | Connect To Your Server via SSH | 04:41 |
90 | Update Server Software Packages and Update Java | 03:35 |
91 | Download & Install Apache Tomcat on AWS EC2 Linux Server | 10:28 |
92 | Configure remote access to Manager app | 02:33 |
93 | Configure Apache Tomcat Users | 03:13 |
94 | Download and Install MySQL Server on EC2 Linux Server | 03:19 |
95 | Installing MySQL on AWS Linux 2 AMI | 05:50 |
96 | Install MariaDb Server on EC2 Linux 2 AMI | 04:44 |
97 | Create Database and Add Database User | 04:03 |
98 | Deploy Our Web Service App on Apache Tomcat | 03:53 |
99 | Sending HTTP Request to a WebService Deployed on a Remote Server | 03:28 |
100 | Introduction | 03:10 |
101 | Amazon RDS - Creating MySQL Database | 06:14 |
102 | Security Group Configuration | 02:31 |
103 | Configure application to use MySQL deployed in RDS | 03:32 |
104 | Deploying WAR to AWS Elastic Beanstalk | 02:31 |
105 | Trying how it works | 01:53 |
106 | Introduction: @OneToOne, @OneToMany, @ManyToOne | 08:27 |
107 | Add List of Addresses to JSON Payload | 03:37 |
108 | Add List of Addresses to a UserDetailsRequestModel | 03:47 |
109 | Creating AddressDTO | 03:02 |
110 | A Better Way of Mapping DTO to an Entity and Entity to a DTO | 03:23 |
111 | Trying if Deep Objects Mapping Works | 03:36 |
112 | Create AddressEntity class | 07:28 |
113 | Add @OneToMany to UserEntity class | 03:01 |
114 | Generate Public Address Id | 02:21 |
115 | Updating Service class Java code | 05:16 |
116 | Trying How it Works: Creating a new User record | 05:30 |
117 | Include List of Addresses Into Response | 03:56 |
118 | Get List of Addresses Web Service Endpoint | 08:06 |
119 | Get List of Addresses Service Interface | 02:09 |
120 | Get List of Addresses Service Interface Implementation | 03:48 |
121 | Get List of Addresses Spring Data JPA Interface | 07:47 |
122 | Trying How the Get List of Addresses Works | 03:40 |
123 | API Call to Get a Single Address Details | 06:40 |
124 | Important house keeping message | 01:07 |
125 | Introduction | 03:03 |
126 | Adding HATEOAS Support to Our Project | 03:06 |
127 | Adding Links to the AddressRest Model | 09:24 |
128 | Using the methodOn() | 05:25 |
129 | Adding Links to a Get Addresses API Call | 04:57 |
130 | Applying HAL Format | 08:13 |
131 | Introduction | 03:02 |
132 | Adding HATEOAS support to our project | 02:04 |
133 | Adding Links. Representation Model. | 10:03 |
134 | Adding Links. Entity Model. | 03:23 |
135 | Building links with methodOn() | 05:16 |
136 | Returning a collection of resources with CollectionModel. | 04:57 |
137 | Adding links to embedded list of addresses | 03:34 |
138 | Introduction | 04:58 |
139 | Verify Email Address with Amazon SES | 05:02 |
140 | Moving Out of AWS SES Sandbox | 05:05 |
141 | Submit Support Ticket to Increase Sending Limits | 02:10 |
142 | Create AWS IAM Access Credentials | 04:27 |
143 | Creating Shared Credentials File | 00:43 |
144 | Add AWS Java SDK SES Maven Dependency | 01:17 |
145 | Spring Security. Make Email Verification a Public Web Service Endpoint. | 02:32 |
146 | The verifyEmailToken() RestController Method | 04:49 |
147 | The verifyEmailToken() Service Layer Function | 04:12 |
148 | The findUserByEmailVerificationToken() Data Layer Function | 01:35 |
149 | Checking if Email Verification Token Has Expired | 03:52 |
150 | Generate and Save the Email Verification Token | 05:00 |
151 | Prevent Users with Unverified Email Address to Login | 04:50 |
152 | Trying How it works | 05:14 |
153 | Create a new Web Project | 03:02 |
154 | Download Apache Tomcat and Add to Spring STS | 03:46 |
155 | Creating Email Verification Service Web Page | 05:23 |
156 | Reading JavaScript URL Request Parameters | 02:54 |
157 | The verifyToken() AJAX HTTP Get Request | 04:11 |
158 | Deploying REST API and Email Verification Service to a Local Tomcat | 09:52 |
159 | Trying Email Verification Feature on Local Server | 08:18 |
160 | Adding Code to Send Email | 10:46 |
161 | Deploying Email Verification to a Remote Amazon EC2 Linux Server | 05:19 |
162 | Trying Email Verification on Remote Server | 08:19 |
163 | Introduction | 04:40 |
164 | Password Reset Request RestController Method | 05:03 |
165 | Password Reset Request Service Layer Method | 04:31 |
166 | Generating Password Reset Token | 03:32 |
167 | Create PasswordResetTokenEntity & Password Reset Repository | 06:21 |
168 | Update AmazonSES Class with Code that Sends Email | 07:45 |
169 | Make the /password-reset-request Public | 02:32 |
170 | Trying How Password Reset Request Works | 07:27 |
171 | Password Reset HTML Page: Add Input fields | 06:03 |
172 | Password Reset HTML Page: Add jQuery | 02:09 |
173 | Password Reset HTML Page: Add the saveNewPassword() function | 10:33 |
174 | Add Content Type HTTP Header | 03:06 |
175 | Password Reset RestController Method | 03:52 |
176 | Password Reset Service Layer Method | 07:59 |
177 | Making Password Rest URL Public | 01:54 |
178 | Deploying RESTful Web Service and the Verification Service App | 04:58 |
179 | Trying How Password Reset Works | 07:40 |
180 | Introduction to Testing with JUnit & Mockito | 04:20 |
181 | Test Cases Source Code and Test Libraries Dependency | 04:18 |
182 | Creating a new JUnit 5 Test Case | 04:39 |
183 | Mocking Objects with Mockito @Mock | 08:57 |
184 | JUnit 5 Assertions. Asserting Successful Method Execution. | 07:50 |
185 | JUnit 5. Expect an Exception with assertThrows() | 05:33 |
186 | The testCreateUser() method. Mocking Objects. | 10:13 |
187 | Testing the createUser() Service Method | 07:56 |
188 | The testCreateUser() method. Adding more code. | 14:47 |
189 | doNothing(). Exclude Integration Code from Unit Test. | 07:52 |
190 | Assert an Exception is thrown in the createUser() method | 04:03 |
191 | Create a New Test Case | 02:40 |
192 | Create Mock Objects and Configure Methods Behaviour | 06:39 |
193 | Asserting with assertNotNull, assertEquals and assertTrue | 03:37 |
194 | Create a new JUnit Integration Test Case | 06:13 |
195 | Test the Generate UserId Method | 04:14 |
196 | Test If JWT Token Has Not Expired | 06:10 |
197 | Test the Expired JWT Token | 05:43 |
198 | What is H2 In-memory Database and Why Using It | 03:20 |
199 | H2 Database Console Preview | 07:54 |
200 | Adding Support for the H2 Database | 08:00 |
201 | Sign in to H2 In-Memory Database | 05:01 |
202 | Protect the H2 In-Memory Database with a Password | 02:04 |
203 | API Call to Create a New User and Preview User Details in an In-Memory Database | 03:45 |
204 | Introduction | 03:56 |
205 | Creating a new maven project | 04:41 |
206 | Add support for Rest Assured and JUnit 5 to your project | 07:39 |
207 | Create User API Call: Creating a Test Method | 02:20 |
208 | Create User API Call: Setting Request URL, Context Path and a Port number | 03:18 |
209 | Create User API Call: Create HTTP Post Request and Validate Response | 08:40 |
210 | Create User API Call: Running a Test Case | 06:14 |
211 | Create User API Call: Verify JSON Array with a list of Addresses | 09:06 |
212 | User Login API Call: Create Test Class | 02:56 |
213 | User Login API Call: Create Test Method | 08:49 |
214 | User Login API Call: Run Test Method | 05:17 |
215 | JUnit Test Methods ordering with @FixMethodOrder | 04:31 |
216 | Get User Details API Call: Create Test method | 05:41 |
217 | Get User Details API Call: Validating List of Addresses | 04:12 |
218 | Get User Details API Call: Use the pathParam() | 01:21 |
219 | Get User Details API Call: Running Test Method | 03:36 |
220 | Update User Details API Call: Create Test Method & HTTP Request | 08:46 |
221 | Update User Details API Call: Validating HTTP Response | 07:05 |
222 | Delete User Details API Call: Create Test Method | 05:19 |
223 | Delete User Details API Call: Run Test Method | 02:40 |
224 | Introduction. What If You Need to Run SQL Query? | 02:29 |
225 | Native SELECT SQL Query Example | 04:47 |
226 | JUnit Test to test Native SELECT SQL Query | 10:40 |
227 | Native SQL Query with Positional Parameters | 08:27 |
228 | Native SQL Query with Named Parameters | 04:50 |
229 | Log SQL Queries and Their Values in the Console | 03:51 |
230 | Using Advanced LIKE Expressions | 05:59 |
231 | Select Specific Columns from a Table | 06:53 |
232 | UPDATE SQL Query Example | 08:32 |
233 | JPQL Introduction | 02:01 |
234 | JPQL Select SQL Query | 06:56 |
235 | JPQL Query to Select Specific Fields Only | 04:11 |
236 | JPQL Update SQL Query | 06:28 |
237 | Do I Need To Enable CORS? | 05:40 |
238 | Send HTTP Request to Reproduce Cross Origin Issue | 07:37 |
239 | Enable Cross Origin Requests in Rest Controller | 08:08 |
240 | Global CORS configuration | 05:17 |
241 | Spring Security Configuration for CORS | 13:27 |
242 | Introduction | 02:13 |
243 | Add Swagger Dependencies | 03:40 |
244 | Create Swagger Configuration File | 02:48 |
245 | Enable Swagger URLs and View JSON Documentation | 05:56 |
246 | View API Documentation In Swagger UI | 05:46 |
247 | Add Authorization Header | 07:03 |
248 | Add /login endpoint | 16:57 |
249 | Upading API Documentation Information | 06:57 |
250 | Web Service Endpoints Additional Information | 04:53 |
251 | Share Your API with Others | 01:26 |
252 | Share Swagger UI URL | 04:39 |
253 | Introduction to Roles and Authorities | 05:24 |
254 | Authentication vs Authorization | 07:32 |
255 | Database Tables Design | 02:56 |
256 | User Roles @ManyToMany Mapping for User Entity | 06:23 |
257 | Create RoleEntity | 04:48 |
258 | Role Authority @ManyToMany Mapping for the RoleEntity | 03:28 |
259 | Create AuthorityEntity | 05:52 |
260 | Create Role Repository | 03:02 |
261 | Create Authority Repository | 01:44 |
262 | Creating Initial Roles, Authorities and Admin User | 02:58 |
263 | Create InitialUsersSetup Class | 03:03 |
264 | Creating Authorities: READ, WRITE, DELETE | 05:40 |
265 | Creating Roles: ROLE_ADMIN, ROLE_USER | 07:09 |
266 | Create User with Admin Role | 07:31 |
267 | Create User Principal Class | 12:06 |
268 | Update Authentication and Authorization Filters | 06:26 |
269 | Configure HttpSecurity to Use the ADMIN Role | 10:00 |
270 | Configure HttpSecurity to Use the DELETE_AUTHORITY | 01:07 |
271 | hasAnyRole() and hasAnyAuthority() | 01:52 |
272 | Method Level Security Introduction | 04:22 |
273 | Enable Global Method Security | 01:41 |
274 | @Secured Annotation Example | 04:48 |
275 | @PreAuthorize Annotation Example | 03:22 |
276 | Accessing Principal Object and Method Argument | 05:50 |
277 | Trying How It Works | 03:36 |
278 | @PostAuthorize Annotation Example | 06:06 |
279 | Assign ROLE_USER to a Regular User | 11:39 |
280 | Spring Web MVC - Overview | 03:28 |
281 | Creating Spring Web MVC project | 06:42 |
282 | Configure JSP & JSTL support | 07:53 |
283 | Configure Thymeleaf support | 06:11 |
284 | The Model object | 03:39 |
285 | The ModelMap object | 02:53 |
286 | The ModelAndView object | 03:11 |
287 | Read URI path variables | 05:50 |
288 | Read query string parameters | 04:32 |
289 | Read Form Data with @ModelAttribute | 08:12 |
290 | Read JSON request body | 04:10 |
291 | Return JSON in response body | 04:57 |
292 | Bonus lecture | 03:05 |
Similar courses to RESTful Web Services, Java, Spring Boot, Spring MVC and JPA
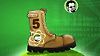
Spring Framework 5: Beginner to Guruudemy
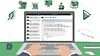
Learn Spring 5 and Spring Boot 2baeldung
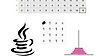
Algorithms in Java: Live problem solving & Design Techniquesudemy
![[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners](https://cdn.courseflix.net/courses/100x56/new-spring-boot-3-spring-6-hibernate-for-beginners.jpg?d=1743455628751)
[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginnersudemy
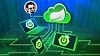
Spring Boot Microservices with Spring Cloud Beginner to Guruudemy
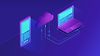
Spring Boot Microservices and Spring Cloududemy
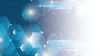
Java Multithreading, Concurrency & Performance Optimizationudemy
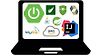
Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog Appudemy
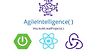