Algorithms in Java: Live problem solving & Design Techniques
Algorithm Design Techniques: Live problem-solving in Java. Algorithms are everywhere. One great algorithm applied sensibly can result in a System like GOOGLE! Completer scientists have worked from 100s of years and derived some of the techniques that can be applied to write and design algorithms. So Why to reinvent the wheel ?? Let’s go through some of the most famous algorithm design techniques in this course.
Read more about the course
Once you will come to know these design techniques It will become very easy for you to approach a problem by identifying which technique to apply to solve that correctly and efficiently.
0. Complexity analysis
1. Recursion is the base of any algorithm design
2. Backtracking
3. Divide and Conquer
4. Greedy algorithms
5. Dynamic programming
And WE WILL WRITE THE CODE LINE BY LINE IN JAVA !!
By the end of this course -
1. You will understand how to design algorithms
2. A lot of coding practice and design live problems in Java
3. Algorithm Complexity analysis
Watch Online Algorithms in Java: Live problem solving & Design Techniques
# | Title | Duration |
---|---|---|
1 | Course Introduction | 04:08 |
2 | Introduction to Algorithms | 03:50 |
3 | Section Introduction | 01:15 |
4 | Complexity Analysis 1 | 07:42 |
5 | Complexity Analysis 2 | 14:04 |
6 | Section Summary | 01:14 |
7 | Section Introduction | 00:38 |
8 | Recurrence Relation | 05:39 |
9 | Solving Recurrence Relation | 11:19 |
10 | Master's Theorem | 05:36 |
11 | Section Summary | 00:31 |
12 | Section Introduction | 01:57 |
13 | Recursion | 07:01 |
14 | Identification | 06:10 |
15 | Approaching | 04:36 |
16 | Problem 01 : FindingSubsequences - Logic | 04:07 |
17 | Problem 01 : FindingSubsequences - Live Code Java | 05:40 |
18 | Problem 01 : FindingSubsequences - Complexity Analysis | 02:21 |
19 | Problem 02: Tower of Hanoi - Logic | 05:39 |
20 | Problem 02 : Tower of Hanoi - Live Code Java | 04:49 |
21 | Problem 02 : Tower of Hanoi - Complexity Analysis | 11:11 |
22 | Problem 03 : 7.Array Product Sum - Logic | 04:32 |
23 | Problem 03 : 7.Array Product Sum - Live Code Java | 05:00 |
24 | Problem 03 : Array Product Sum - Complexity Analysis | 01:08 |
25 | Problem 04 : Binary Subtree - Logic | 06:23 |
26 | Problem 04 : Binary Subtree - Live Code Java | 08:25 |
27 | Problem 04 : Binary Subtree - Complexity Analysis | 00:58 |
28 | Why and Why not Recursion | 01:19 |
29 | Types Of Recursion | 04:02 |
30 | Tail Recursion | 05:19 |
31 | Section Summary | 01:01 |
32 | Section Introduction | 01:28 |
33 | Introduction to Backtracking | 01:45 |
34 | Identify Backtracking | 03:04 |
35 | Approching the Solution | 09:37 |
36 | Problem 01 : Rat In Maze - Logic | 08:41 |
37 | Problem 01 : Rat In Maze - Code | 08:45 |
38 | Problem 01 : Rat In Maze - Complexity Analysis | 01:20 |
39 | Problem 02 : NQueen - Logic | 08:44 |
40 | Problem 02 : NQueen - Live Code in Java | 08:10 |
41 | Problem 02 : NQueen - Complexity Analysis | 02:09 |
42 | Problem 03 : Knights Tour Problem - Logic | 05:02 |
43 | Problem 03 : Knights Tour Problem - Live Code in Java | 07:01 |
44 | Problem 03 : Knight Tour Problem - Complexity Analysis | 00:52 |
45 | Problem 04 : Boggle | Word Search - Logic | 05:49 |
46 | Problem 04 : Boggle | Word Search - Live Code in Java | 07:44 |
47 | Problem 04 : Boggle | Word Search - Complexity Analysis | 00:53 |
48 | Section Summary | 01:10 |
49 | Section Introduction | 00:45 |
50 | Introduction To Divide And Conquer | 03:16 |
51 | Identification and Approaching | 03:33 |
52 | Problem 01 : MergeSort - Logic | 09:34 |
53 | Problem 01 : MergeSort - Live Java Code | 08:43 |
54 | Problem 01 : MergeSort - Complexity Analysis | 04:22 |
55 | Problem 02 : QuickSort - Logic | 11:06 |
56 | Problem 02 : QuickSort - Live Java Code | 06:13 |
57 | Problem 02 : QuickSort - Complexity Analysis | 02:30 |
58 | Problem 03 : Median Of Medians - Logic | 09:01 |
59 | Problem 03 : Median Of Medians - Live Java Code | 14:53 |
60 | Section Summary | 00:43 |
61 | Section Introduction | 00:57 |
62 | Introduction to Greedy | 05:23 |
63 | Identification & Approaching the Solution | 03:08 |
64 | Problem 01 : Fractional Knapsack - Logic | 06:24 |
65 | Problem 01 : Fractional Knapsack - Live Code Java | 09:37 |
66 | Problem 01 : Fractional Knapsack - Complexity Analysis | 00:58 |
67 | Problem 02 : IntervalScheduling - Logic | 03:12 |
68 | Problem 02 : IntervalScheduling - Live Code Java | 07:48 |
69 | Problem 02 : IntervalScheduling - Complexity Analysis | 01:39 |
70 | Problem 03 : Huffman Code - Logic | 12:27 |
71 | Problem 03 : Huffman Code - Live Code Java | 19:04 |
72 | Problem 03 : Huffman Code - Complexity Analysis | 01:50 |
73 | Problem 04 : Dijkstra - Logic | 09:22 |
74 | Problem 04 : Dijkstra Logic - Live Code | 08:59 |
75 | Problem 04 : Dijkstra - Complexity Analysis | 01:02 |
76 | Summary | 00:43 |
77 | Section Introduction | 02:16 |
78 | Introduction to Dynamic Programming | 05:25 |
79 | Identification | 01:20 |
80 | Compare DP D&C and Greedy | 03:15 |
81 | Approaching the Solution | 03:44 |
82 | Example 01 : Staircase Problem Theory & Live Code | 15:42 |
83 | Example 01 : Staircase Problem Complexity Analysis | 03:05 |
84 | Example 02 - 0/1 Knapsack Theory & Live code | 26:36 |
85 | Example 02 - 0/1 Knapsack Complexity Analysis | 02:46 |
86 | Example 03 - Coin Change Problem Theory and Code | 25:35 |
87 | Example 03 - Coin Change Problem Complexity Analysis | 03:57 |
88 | Example 04 : Longest Decreasing Subsequence Explanation And Code | 27:55 |
89 | Example 04 : Longest Decreasing Subsequence | Complexity Analysis | 01:37 |
90 | Example 05 : Levenshtein problem | 23:29 |
91 | Example 05 : Levenshtein Complexity Analysis | 01:54 |
92 | Example 06 : Rod Cutting | 18:16 |
93 | Example 06 : Rod Cutting - Complexity Analysis | 01:42 |
94 | Example 07 : Matrix Chain Multiplication | 26:10 |
95 | Example 07 : Matrix Chain Multiplication | Complexity Analysis | 02:31 |
96 | Summary | 00:44 |
97 | Kadane's Algo | 07:40 |
98 | Kadane's Algo Live Code Java | 03:35 |
99 | Kadane's Algo Complexity Analysis | 00:34 |
100 | BellmanFord's Algo | 06:47 |
101 | BellmanFord's Algo Live Code Java | 11:20 |
102 | BellmanFord's Algo Complexity Analysis | 00:52 |
103 | Topological Sort : Kahn's Algo | 06:32 |
104 | Topological Sort Live Code Java | 13:54 |
105 | Topological Sort Complexity Analysis | 01:48 |
106 | Edmond's Karp/Ford-Fulkerson Algorithm | 26:35 |
107 | Ford-Fulkerson Algorithm - Edmond's Karp Implementation | 20:16 |
108 | Tree Overview | 23:28 |
109 | Identification | 02:02 |
110 | Approaching Tree Problems | 04:36 |
111 | Problem 1: Sum Tree - Logic | 09:29 |
112 | Problem 1: Sum Tree - Live Code | 04:55 |
113 | Problem 2: Invert Binary Tree - Logic | 02:20 |
114 | Problem 2: Invert Binary Tree - Live Code | 03:53 |
115 | Problem 3: Binary Search Tree - Logic | 11:46 |
116 | Problem 3: Binary Search Tree - Live Code | 16:19 |
117 | Problem 4: Binary Tree from InOrder & LevelOrder - Logic | 03:47 |
118 | Problem 4: Binary Tree from InOrder & LevelOrder - Live Code | 07:50 |
119 | Graphs - In Real World | 04:57 |
120 | Graphs - Overview | 11:54 |
121 | Identification of Problem | 03:54 |
122 | Approaching the Problem | 09:13 |
123 | Mind-Map | 02:36 |
124 | Type - Graph Traversal | 02:29 |
125 | Depth First Search Traversal | 06:18 |
126 | DFS - Implementation (Recursive) | 07:07 |
127 | DFS - Implementation (Iterative) | 04:50 |
128 | Breadth First Search Traversal | 03:27 |
129 | BFS - Implementation | 04:59 |
130 | Type - Minimum Spanning Tree | 05:30 |
131 | Prim's Algorithm | 09:29 |
132 | Prim's Algorithm - Implementation | 07:34 |
133 | Kruskal's Algorithm | 03:56 |
134 | Union-Find Algorithm | 08:10 |
135 | Kruskal's Algorithm - Implementation | 13:15 |
136 | Type - Shortest Path | 01:17 |
137 | Dijkstra's Algorithm | 09:22 |
138 | Dijkstra's Algorithm - Implementation | 08:59 |
139 | BellmanFord's Algo | 06:47 |
140 | BellmanFord's Algo Live Code | 11:20 |
141 | Floyd Warshall Algorithm | 06:46 |
142 | Floyd-Warshall Algorithm - Implementation | 05:47 |
143 | Johnson's Algorithm | 15:41 |
144 | Johnson's Algorithm - Implementation | 20:23 |
145 | Type - Network Flow | 04:40 |
146 | Ford-Fulkerson Algorithm | 26:35 |
147 | Ford-Fulkerson Algorithm - Edmond's Karp Implementation | 20:16 |
148 | Max-Flow Min-Cut Theorem | 05:46 |
149 | Type - Strongly Connected Components | 02:50 |
150 | Tarjan's Algorithm | 09:55 |
151 | Tarjan's Algorithm - Implementation | 12:25 |
152 | Kosaraju's Algorithm | 06:07 |
153 | Kosaraju's Algorithm - Implementation | 11:01 |
154 | Section Introduction | 01:20 |
155 | Single Dimension Arrays | 13:14 |
156 | Multi Dimension Arrays | 12:43 |
157 | Declaration , Initialisation & Creation | 19:04 |
158 | Playing With Arrays Syntax Java | 10:49 |
159 | Traversing Arrays,Length of Array Java | 13:35 |
160 | Types of Array - based on elements it holds | 11:53 |
161 | Reassigning Array Objects to Array References | 13:17 |
162 | Anonymous Arrays | 03:50 |
163 | Arrays-Summay | 00:49 |
Similar courses to Algorithms in Java: Live problem solving & Design Techniques
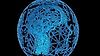
Master Microservices with Java, Spring, Docker, Kubernetesudemy
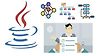
Java Data Structures and Algorithms Masterclassudemy
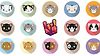
Catsrockthejvm.com
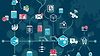
Building Modern Distributed Systems with Javaudemy
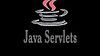
Java Servlets and JSPs developer courseudemy
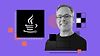
Java Data Structures & Algorithms + LEETCODE Exercisesudemy
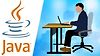
The Complete Java Developerudemy
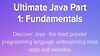
Ultimate Java Part 1: Fundamentalscodewithmosh (Mosh Hamedani)
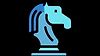
Master Spring 6 Spring Boot 3 REST JPA Hibernateudemy
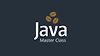