Java Foundations: The Complete Course with Java 21 Updates
88h 37m 5s
English
Paid
This course is designed for absolute beginners in programming and for those who don't mind learning Java at a slower pace. If you need an accelerated course, it might not be suitable (although you can speed up playback and find useful moments). Even if you already know the basics of Java, you can skip familiar sections and focus on new ones for yourself, such as Regex, Streams/Lambdas API, Optionals, Date/Time, SQL, Database/JDBC - topics that many experienced developers often don't know as well as they should. The course sections on these topics cover more than many individual courses on similar subjects - at a comparable price.
If you want to start a career as a professional developer, this course will help you master the basics with an instructor who has extensive experience in hiring and training developers. The course focuses on real Java skills needed for a successful start and rapid growth, emphasizing practical skills rather than academic knowledge. The instructor leverages their experience of observing successful and unsuccessful candidates to create a course that will help you achieve success and secure a sought-after developer role.
Read more about the course
In this course, you will learn topics such as:
- Object-Oriented Programming (OOP)
- Many developers know the syntax of Java but lack the skills to utilize its capabilities for creating complex, maintainable, and team-friendly code.
- Regular Expressions
- Many Java developers are not well-versed in regular expressions, which makes text processing, validation, and key information extraction challenging. We will learn to use regular expressions to reduce code volume and increase its flexibility.
- Functional Programming using Lambdas and Streams API
- Despite the widespread use of functional programming, many Java developers have not mastered the capabilities of lambdas and the Streams API. We will explore these powerful tools to simplify and enhance data processing.
- Optionals
- The concept of "null" has caused numerous errors. Optionals aim to solve this problem, but many developers have yet to master their use. We will explore how to avoid issues related to null.
- Date and Time API in Java 8
- Java 8 introduced significant improvements in handling dates and times, but many developers have not mastered the new API. We will demonstrate how to work with dates and times correctly using a modern approach.
- Working with Databases and SQL
- Although many Java developers work with databases through frameworks like Spring Boot, this often leads to insufficient understanding of how these frameworks interact with the database. We will provide foundational knowledge to help you better utilize these tools.
- Introduction to Spring Framework and Spring Boot
- The Spring Framework and Spring Boot have revolutionized Java application development. These frameworks are almost always necessary for Java developers in the job market. We will introduce you to the basics of Spring and prepare you for the next step in your learning journey.
If you are ready to put in the effort and start a new stage of your career, join us!
Watch Online Java Foundations: The Complete Course with Java 21 Updates
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | 1 - Welcome to the World of Java | 03:20 |
2 | 2 - What is Java | 05:06 |
3 | 3 - Installing JDK 21 IntelliJ IDE on MacOS with SDKMan Homebrew | 15:57 |
4 | 4 - Installing JDK 21 IntelliJ IDE on Windows with Chocolatey | 20:00 |
5 | 5 - Legacy Lesson Install JDK 17 on Windows | 03:04 |
6 | 6 - Legacy Lesson Install JDK 17 on MacOS | 04:13 |
7 | 7 - Legacy Lesson Installing a Java Editor IntelliJ IDE | 05:44 |
8 | 8 - Hello World Our First Simple Application | 09:17 |
9 | 9 - Asking for Help | 06:33 |
10 | 10 - Coding Exercises for the Whole Course | 06:25 |
11 | 12 - Object Orientation | 34:44 |
12 | 13 - Class Basics | 17:53 |
13 | 14 - Variables Data Types | 18:51 |
14 | 15 - var Variables | 06:50 |
15 | 16 - Simple Collections with Arrays | 19:58 |
16 | 17 - Methods | 32:55 |
17 | 18 - Varargs | 11:25 |
18 | 19 - Static Methods | 09:04 |
19 | 20 - Static Variables | 06:28 |
20 | 21 - Static Initializers | 05:42 |
21 | 22 - The Object Superclass | 15:02 |
22 | 23 - Member Visibility Methods | 33:12 |
23 | 24 - Member Visibility Fields | 07:20 |
24 | 25 - Constructors | 22:51 |
25 | 26 - Getters Setters | 22:46 |
26 | 28 - Intro | 00:58 |
27 | 29 - Creating Strings | 17:09 |
28 | 30 - Upper Lower Casing | 10:00 |
29 | 31 - Strings Blank or Empty | 05:39 |
30 | 32 - Replacing Text Within Strings | 06:30 |
31 | 33 - Removing White Space strip | 19:10 |
32 | 34 - Getting Individual Characters of a String charAt | 05:28 |
33 | 35 - Comparing String for Alphabetical Order compareTo | 09:36 |
34 | 36 - Determining if Text is Contained in a String contains | 03:18 |
35 | 37 - String Concatenation concat | 29:16 |
36 | 38 - Determining the Length of a String length | 10:34 |
37 | 39 - Getting Parts of a String substring | 20:37 |
38 | 40 - Searching within a String indexOf | 32:12 |
39 | 41 - Splitting String Apart split | 23:39 |
40 | 42 - Beginning Ending of Strings startsWith endsWith | 08:25 |
41 | 43 - Comparing Strings for Equality contentEquals | 15:06 |
42 | 44 - JDK 21 String Templates | 11:05 |
43 | 46 - Intro to Regular Expressions | 02:24 |
44 | 47 - ABCs of Regular Expressions Part One | 13:09 |
45 | 48 - ABCs of Regular Expressions Part Two | 34:24 |
46 | 49 - Capture Groups Part One | 25:28 |
47 | 50 - Capture Groups Part Two | 12:58 |
48 | 51 - Named Capture Groups | 13:04 |
49 | 52 - Comments in Regular Expressions | 11:26 |
50 | 53 - Wrapping Up Phone Number Parsing | 06:44 |
51 | 54 - Additional Character Classes | 19:54 |
52 | 55 - Parsing a Real Text Document | 40:14 |
53 | 56 - Greedy Operators | 15:32 |
54 | 57 - Finding Multiple Matches | 16:07 |
55 | 60 - Bits and Bytes | 09:05 |
56 | 61 - Hexadecimal Numbers | 09:33 |
57 | 62 - Numeric Data Types | 17:26 |
58 | 63 - Bigger Numbers | 11:33 |
59 | 64 - Floating Point Numbers | 19:41 |
60 | 66 - Simple Math Operations | 37:27 |
61 | 67 - Standard Math Functions | 17:36 |
62 | 68 - More on Random Numbers | 07:02 |
63 | 69 - Coding Exercise Calculating the Area of a Circle | 06:05 |
64 | 70 - Coding Exercise Calculating Centripetal Force | 21:50 |
65 | 71 - Comparing Numbers | 05:52 |
66 | 72 - Introducing BigDecimal | 09:10 |
67 | 73 - Using BigDecimal | 12:31 |
68 | 74 - More on BigDecimal BigInteger | 11:59 |
69 | 76 - Formatting Numbers | 19:48 |
70 | 77 - Customizing Number Formatters | 13:04 |
71 | 79 - Numeric Wrapper Classes | 24:42 |
72 | 81 - Intro to Control Flow | 06:01 |
73 | 82 - Conditionals with IfElse | 27:21 |
74 | 83 - Control Flow Inequalities | 07:51 |
75 | 84 - The Switch Statement | 19:42 |
76 | 85 - Switch Statement New Features | 22:39 |
77 | 86 - While Loops Part One | 26:46 |
78 | 87 - Switch Statement Pattern Matching in JDK 21 | 14:58 |
79 | 88 - Legacy Lesson see JDK 21 version Switch Statement Pattern Matching JDK 17 | 11:17 |
80 | 89 - While Loops Part Two | 22:04 |
81 | 90 - DoWhile Loops Part One | 32:15 |
82 | 91 - DoWhile Loops Part Two | 23:35 |
83 | 92 - For Loops | 26:19 |
84 | 93 - A Simpler For Loop | 09:40 |
85 | 94 - The Enhanced For Loop | 04:40 |
86 | 95 - Applying Loops to Regex | 06:21 |
87 | 97 - Intro to Testing | 02:54 |
88 | 98 - Setting Up | 18:59 |
89 | 99 - Our First Test | 28:36 |
90 | 100 - Writing the Second Test | 23:33 |
91 | 101 - Testing Edge Cases | 12:19 |
92 | 102 - Testing Annuity Calculation | 19:22 |
93 | 103 - Reimplementing the Guessing Game with TDD | 19:51 |
94 | 104 - Guessing Game TDD Part Two | 37:23 |
95 | 105 - Implementing Randomness | 19:51 |
96 | 106 - Testing for Randomness A Deeper Explanation | 25:26 |
97 | 107 - Tracking the Number of Guesses | 34:40 |
98 | 108 - Handling More than Four Guesses | 11:39 |
99 | 109 - Wrapping up Testing | 24:58 |
100 | 110 - Reimplementing the Guessing Game User Interface | 13:21 |
101 | 111 - Debugging Code Part One | 35:46 |
102 | 112 - Debugging Code Part Two | 17:05 |
103 | 114 - Intro | 01:32 |
104 | 115 - Enums | 31:01 |
105 | 116 - Enum Ordinals | 22:00 |
106 | 117 - Enum Methods | 20:57 |
107 | 118 - Enum Fields | 22:39 |
108 | 119 - Enum ValueOf | 12:22 |
109 | 120 - The this Keyword | 12:54 |
110 | 121 - Setup for More Advanced OOP Topics | 13:18 |
111 | 122 - Employee Salaries Continued | 29:02 |
112 | 123 - Completing Other Employee Cases | 24:28 |
113 | 124 - Introducing a Programmer Class | 34:12 |
114 | 125 - Implementing the Other Employee Classes | 26:24 |
115 | 126 - Introducing Interfaces | 29:30 |
116 | 127 - Revisiting Class Hierarchies | 20:55 |
117 | 128 - Completing the Employee Class Hierarchy | 19:03 |
118 | 129 - Dealing with the Null Case | 07:35 |
119 | 130 - Introducing Abstract Classes | 22:17 |
120 | 131 - Factory Methods | 27:38 |
121 | 132 - Nested Classes | 09:49 |
122 | 133 - Other Types of Nested Classes | 17:44 |
123 | 134 - Records | 20:36 |
124 | 135 - Lambdas Versus Anonymous Classes | 18:43 |
125 | 136 - Composition Versus Inheritance | 24:54 |
126 | 137 - Default Methods | 22:00 |
127 | 138 - Comparing Classes with instanceof | 19:23 |
128 | 139 - Unnamed Patterns | 11:53 |
129 | 140 - OOP Recap | 20:47 |
130 | 142 - Solutions Explanations to Exercises 81 | 36:12 |
131 | 143 - Solutions Explanations to Exercises 82 83 | 42:05 |
132 | 144 - Solutions Explanations to Exercises 84 89 | 20:39 |
133 | 145 - Solutions Explanations to Exercises 810 819 | 20:05 |
134 | 146 - Solutions Explanations to Exercises 820 822 | 20:50 |
135 | 147 - Intro to Collections | 04:52 |
136 | 148 - List Basics | 22:28 |
137 | 149 - Linked Lists | 26:52 |
138 | 150 - Looping with Iterators | 20:33 |
139 | 151 - Loose Ends of Lists | 07:22 |
140 | 152 - Additional List Methods | 27:03 |
141 | 153 - Listcontains Objectequals | 43:51 |
142 | 154 - Implementing Comparator to Sort Lists | 31:05 |
143 | 155 - Implementing Comparable to Sort Lists | 38:11 |
144 | 156 - Intro to Sets | 19:54 |
145 | 157 - Sets Hashcode | 22:46 |
146 | 158 - LinkedHashSet | 12:34 |
147 | 159 - TreeSet | 25:41 |
148 | 160 - Intro to Maps | 04:12 |
149 | 161 - A Map Scenario | 25:43 |
150 | 162 - Using Map Implementations | 29:47 |
151 | 163 - Additional Map Methods | 24:50 |
152 | 164 - JDK 21 Collections Changes | 08:07 |
153 | 165 - Wrapping Up | 03:56 |
154 | 167 - Intro to Streams Lambdas | 01:48 |
155 | 168 - First Steps into Streams API | 22:17 |
156 | 169 - Streams Explained | 11:10 |
157 | 170 - Creating Streams | 42:41 |
158 | 171 - Summing with Streams | 28:58 |
159 | 172 - Sorting with Streams | 25:10 |
160 | 173 - Filtering with Streams | 24:10 |
161 | 174 - Additional Filtering Techniques | 20:43 |
162 | 175 - Flattening Streams of Streams | 31:35 |
163 | 176 - Alternatives to Filter | 30:09 |
164 | 177 - The Map Reduce Pattern | 45:33 |
165 | 178 - Intro to More Advanced Streams | 23:46 |
166 | 179 - Big Data Summing | 46:07 |
167 | 180 - Domain Models with Streams API | 18:50 |
168 | 181 - Grouping Records | 24:16 |
169 | 182 - Summing by Groups | 22:19 |
170 | 183 - Nested Groupings | 29:01 |
171 | 184 - Reducing with Collect | 18:13 |
172 | 185 - Partitioning vs Grouping | 12:57 |
173 | 186 - Functional Interfaces | 11:46 |
174 | 187 - Functional Methods of Collections | 38:30 |
175 | 189 - Exceptions | 36:20 |
176 | 190 - Checked Exceptions | 19:10 |
177 | 191 - Generics Part One | 30:20 |
178 | 192 - Generics Part Two | 34:28 |
179 | 193 - Optionals Part One | 31:02 |
180 | 194 - Optionals Part Two | 42:24 |
181 | 195 - Dates Times Intro | 28:46 |
182 | 196 - Dates Times Periods Durations | 08:03 |
183 | 197 - Dates Times Time Zones | 15:44 |
184 | 198 - Dates Times Parsing Formatting | 31:43 |
185 | 199 - Dates Times Temporal Adjustors Immutability | 13:00 |
186 | 200 - Getting Started with Databases | 12:26 |
187 | 201 - Installing DBeaver SQL Client | 09:17 |
188 | 202 - Installing Squirrel SQL Client | 14:54 |
189 | 203 - Lets Learn Some SQL | 20:43 |
190 | 204 - Creating Retrieving Records | 20:55 |
191 | 205 - Database Functions | 12:29 |
192 | 206 - The UD in CRUD | 12:47 |
193 | 207 - Creating a New Database Project | 29:13 |
194 | 208 - TDD for Saving a Person | 19:22 |
195 | 209 - Writing the JDBC Code to Save a Person | 28:58 |
196 | 210 - Tidying Up Explaining Our Save Code | 37:33 |
197 | 211 - Creating a Custom Save Exception | 08:32 |
198 | 212 - Finding a Person By ID | 41:33 |
199 | 213 - FindById A Negative Case | 10:59 |
200 | 214 - Deleting a Person | 09:44 |
201 | 215 - Deleting Multiple People at Once | 18:50 |
202 | 216 - Updating a Person | 34:26 |
203 | 217 - Creating a Reusable CRUD Repository | 21:15 |
204 | 218 - CRUD Repository FindById | 09:34 |
205 | 219 - CRUD Repository Completing the Remaining Methods | 22:53 |
206 | 220 - CRUD Repository Implementing a Custom Annotation | 31:42 |
207 | 221 - CRUD Repository Allowing Multiple SQL Annotations | 38:33 |
208 | 222 - CRUD Repository Custom ID Annotation | 28:54 |
209 | 223 - Loading Five Million People with PeopleRepository | 32:31 |
210 | 224 - Speeding up the Queries with Indexes | 22:51 |
211 | 225 - Creating an Address Table | 30:24 |
212 | 226 - Saving One Address | 39:40 |
213 | 227 - Saving a Person without an Address | 20:01 |
214 | 228 - Fetching a Person with Their Address | 20:09 |
215 | 229 - Fetching Address in One Go with Join Queries | 29:00 |
216 | 230 - Joins with Missing Addresses | 40:03 |
217 | 231 - Adding a Second Address Field | 41:30 |
218 | 232 - Brief Word of Encouragement | 04:15 |
219 | 233 - Challenge Adding a Spouse | 04:38 |
220 | 234 - Adding Children | 28:54 |
221 | 235 - Fetching Children in One Join | 21:25 |
222 | 236 - Writing Code to Fetch Children in One Join | 32:41 |
223 | 237 - Troubleshooting Techniques for Broken Tests | 17:01 |
224 | 238 - Fixing FindAll for JoinFetched Child Code | 29:05 |
225 | 239 - Join Tables | 13:57 |
226 | 240 - Optimizing with Caching | 27:12 |
227 | 241 - Optimizing Prepared Statements | 17:36 |
228 | 242 - Conclusion | 34:33 |
229 | 243 - Introduction | 09:33 |
230 | 244 - Creating the PeopleDBWeb Project | 14:51 |
231 | 245 - Implementing Web Hello World | 09:06 |
232 | 246 - Web HTTP Basics | 22:05 |
233 | 247 - Displaying a Simple List of People | 22:53 |
234 | 248 - Introducing a Bootstrap Table for People | 25:20 |
235 | 249 - Formatting Dates Salaries | 30:03 |
236 | 250 - Introducing Spring Data | 44:03 |
237 | 251 - Saving People | 42:17 |
238 | 252 - UI Database Tweaks | 31:16 |
239 | 253 - Validating Data | 29:52 |
240 | 254 - Deleting People | 27:27 |
241 | 255 - Updating People | 37:59 |
242 | 256 - Internationalization | 40:00 |
243 | 257 - Uploading a File | 33:13 |
244 | 258 - Saving Uploaded Files | 38:14 |
245 | 259 - Retrieving Uploaded Files | 35:23 |
246 | 260 - Handling Exceptions in UI | 35:11 |
247 | 261 - Introducing a Service Layer for Coordination Logic | 28:44 |
248 | 262 - Coordinating Deletes with a Service | 18:16 |
249 | 263 - Custom Query Methods | 17:21 |
250 | 264 - Pagination | 39:02 |
251 | 265 - Importing a CSV File | 37:44 |
252 | 266 - Fixing Deletes UI Loose Ends | 26:19 |
253 | 267 - Items Not Covered Thoroughly | 35:12 |
254 | 268 - Where to Go from Here | 32:08 |
Similar courses to Java Foundations: The Complete Course with Java 21 Updates
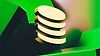
RESTful Web Services, Java, Spring Boot, Spring MVC and JPAudemy
Category: Spring Boot, Spring Data, Java, Spring, Spring MVC, Spring Security
Duration 25 hours 8 minutes 11 seconds
Course
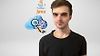
Java Test Automation Engineer - from Zero to Heroudemy
Category: Java, Other (QA)
Duration 18 hours 40 minutes 6 seconds
Course
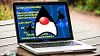
Java Programming Masterclass covering Java 11 & Java 17udemy
Category: Java
Duration 80 hours 13 minutes 14 seconds
Course
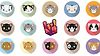
Catsrockthejvm.com
Category: Java
Duration 10 hours 39 minutes 36 seconds
Course
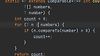
Java Genericsamigoscode (Nelson Djalo)
Category: Java
Duration 1 hour 8 minutes 39 seconds
Course
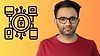
Spring Security 6 Zero to Master along with JWT,OAUTH2udemy
Category: Java, Spring Security
Duration 14 hours 50 minutes 3 seconds
Course
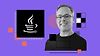
Java Data Structures & Algorithms + LEETCODE Exercisesudemy
Category: Java
Duration 9 hours 47 minutes 55 seconds
Course
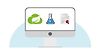
Spring Professional Certification Exam Tutorial - Module 08udemy
Category: Java, Spring
Duration 1 hour 54 minutes 51 seconds
Course
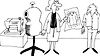
Java Design Patternsjavaspecialists.eu
Category: Java
Duration 16 hours 20 minutes 37 seconds
Course
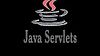
Java Servlets and JSPs developer courseudemy
Category: Java
Duration 2 hours 12 seconds
Course