Java Data Structures and Algorithms Masterclass
Welcome to the Java Data Structures and Algorithms Masterclass, the most modern, and the most complete Data Structures and Algorithms in Java course on the internet. At 45+ hours, this is the most comprehensive course online to help you ace your coding interviews and learn about Data Structures and Algorithms in Java.
Read more about the course
You will see 100+ Interview Questions done at the top technology companies such as Apple,Amazon, Google and Microsoft and how to face Interviews with comprehensive visual explanatory video materials which will bring you closer towards landing the tech job of your dreams!
Learning Java is one of the fastest ways to improve your career prospects as it is one of the most in demand tech skills! This course will help you in better understanding every detail of Data Structures and how algorithms are implemented in high level programming language.
We'll take you step-by-step through engaging video tutorials and teach you everything you need to succeed as a professional programmer.
After finishing this course, you will be able to:
- Learn basic algorithmic techniques such as greedy algorithms, binary search, sorting and dynamic programming to solve programming challenges.
- Learn the strengths and weaknesses of a variety of data structures, so you can choose the best data structure for your data and applications
- Learn many of the algorithms commonly used to sort data, so your applications will perform efficiently when sorting large datasets
- Learn how to apply graph and string algorithms to solve real-world challenges: finding shortest paths on huge maps and assembling genomes from millions of pieces.
Why this course is so special and different from any other resource available online?
- This course will take you from very beginning to a very complex and advanced topics in understanding Data Structures and Algorithms!
- You will get video lectures explaining concepts clearly with comprehensive visual explanations throughout the course.
- You will also see Interview Questions done at the top technology companies such as Apple,Amazon, Google and Microsoft.
- I cover everything you need to know about technical interview process!
So whether you are interested in learning the top programming language in the world in-depth and interested in learning the fundamental Algorithms, Data Structures and performance analysis that make up the core foundational skillset of every accomplished programmer/designer or software architect and is excited to ace your next technical interview this is the course for you!
Watch Online Java Data Structures and Algorithms Masterclass
# | Title | Duration |
---|---|---|
1 | Curriculum Walkthrough | 08:22 |
2 | What is a data structure? | 03:27 |
3 | What is an algorithm? | 03:54 |
4 | Why are Data Structures and Algorithms important? | 03:41 |
5 | Types of Data Structures | 03:23 |
6 | Types of Algorithms | 04:01 |
7 | Goals : what you will learn by the end of this section | 01:06 |
8 | What is Recursion? | 05:01 |
9 | Why do we need Recursion? | 03:22 |
10 | The Logic Behind Recursion | 09:16 |
11 | Recursive vs Iterative Solution | 04:14 |
12 | When to Use/Avoid Recursion? | 06:22 |
13 | How to Write Recursion in 3 Steps? | 13:43 |
14 | Fibonacci Series using Recursion | 09:38 |
15 | Question 1 - Sum of Digits | 08:51 |
16 | Question 2 - Power | 05:56 |
17 | Question 3 - GCD | 06:41 |
18 | Question 4 - Decimal to Binary | 08:17 |
19 | What is Big O? | 03:21 |
20 | Big O Notations (Big O, Big Omega, Big Theta) | 08:03 |
21 | Most Common Time Complexities | 10:08 |
22 | Space Complexity | 02:42 |
23 | Drop Constants and Non Dominant Terms | 03:56 |
24 | Addition vs Multiplication | 02:28 |
25 | How to Measure the Code using Big O? | 04:49 |
26 | How to Measure Recursive Algorithm? | 07:20 |
27 | How to Measure Recursive Algorithm with Multiple Calls? | 04:08 |
28 | Question 1 - Time Complexity of Method that returns Sum and Product of Array | 05:47 |
29 | Question 2 - Time Complexity of Print Pairs Method | 05:51 |
30 | Question 3 - Time Complexity of Print Unordered Pairs Method | 04:32 |
31 | Question 4 - Find Time Complexity for Given Method | 02:15 |
32 | Question 5 - Find Time Complexity for Given Method | 01:36 |
33 | Question 6 - Time Complexity of Reverse Array Function | 06:05 |
34 | Question 7 - Equivalent to O(N) | 03:24 |
35 | Question 8 - Time Complexity of Factorial | 02:52 |
36 | Question 9 - Time Complexity of Fibonacci | 02:56 |
37 | Question 10 - Time Complexity Powers Of 2 | 03:00 |
38 | What is an Array? | 07:58 |
39 | Types of Arrays | 06:52 |
40 | Arrays in Memory | 03:53 |
41 | Create an Array | 09:06 |
42 | Insertion in Array | 11:37 |
43 | Accessing Elements in Array | 05:13 |
44 | Array Traversal | 07:28 |
45 | Search for Array Element | 07:53 |
46 | Delete Array Element | 05:58 |
47 | Time and Space Complexity of 1D Arrays | 04:21 |
48 | Create Two Dimensional Array | 11:06 |
49 | Insertion - Two Dimensional Array | 10:39 |
50 | Accessing Two Dimensional Array Element | 07:17 |
51 | Traverse Two Dimensional Array | 07:22 |
52 | Searching Two Dimensional Array | 08:15 |
53 | Delete Two Dimensional Array Element | 06:38 |
54 | Time and Space Complexity of 2D Arrays | 04:07 |
55 | When to Use/Avoid Arrays | 02:38 |
56 | Goals - what you will make by the end of this section | 02:52 |
57 | Calculate Average Temperature | 07:09 |
58 | Find the Days Above Average Temperature | 06:44 |
59 | Section Goals | 00:43 |
60 | Solution - Missing Number | 06:57 |
61 | Solution - Pairs | 09:12 |
62 | Solution - Finding a Number in an Array | 05:27 |
63 | Solution - Max Product of Two Integers | 06:06 |
64 | Solution - IsUnique / Contains Duplicate - LeetCode 217 | 05:16 |
65 | Solution - Permutation | 08:35 |
66 | Rotate Matrix / Image - LeetCode 48 | 12:30 |
67 | What is a Linked List? | 05:58 |
68 | Linked List vs Array | 03:50 |
69 | Types of Linked List | 10:54 |
70 | Linked List in the Memory | 04:02 |
71 | Creation of Singly Linked List | 09:54 |
72 | Insertion in Singly Linked List in Memory | 03:54 |
73 | Insertion Algorithm in Singly Linked List | 08:03 |
74 | Insertion Method in Singly Linked List | 14:56 |
75 | Traversal of Singly Linked List | 09:49 |
76 | Searching in Singly Linked List | 08:50 |
77 | Deletion of a Node from Singly Linked List | 09:38 |
78 | Deletion Method in Singly Linked List | 15:39 |
79 | Deletion of Entire Singly Linked List | 05:57 |
80 | Time and Space Complexity of Singly Linked List | 04:06 |
81 | Create Circular Singly Linked List | 12:59 |
82 | Insertion in Circular Singly Linked List | 07:31 |
83 | Insertion Algorithm in Circular Singly Linked List | 04:49 |
84 | Insertion Method Circular Singly Linked List | 12:00 |
85 | Traversal of Circular Singly Linked List | 09:58 |
86 | Searching a Node in Circular Singly Linked List | 09:49 |
87 | Deletion of a Node from Circular Singly List | 08:39 |
88 | Deletion Algorithm in Circular Singly Linked List | 05:20 |
89 | Deletion Method in Circular Singly Linked List | 11:21 |
90 | Delete Entire Circular Singly Linked List | 06:32 |
91 | Time and Space Complexity of Circular Singly Linked List | 05:33 |
92 | Create Doubly Linked List | 13:46 |
93 | Insertion Doubly Linked List | 09:55 |
94 | Insertion Algorithm Doubly Linked List | 06:30 |
95 | Insertion Method Doubly Linked List | 14:55 |
96 | Traversal of Doubly Linked List | 08:36 |
97 | Reverse Traversal of Doubly Linked List | 08:22 |
98 | Searching for a Node in Doubly Linked List | 08:56 |
99 | Deletion of a Node in Doubly Linked List | 08:34 |
100 | Deletion Algorithm Doubly Linked List | 06:09 |
101 | Deletion Method in Doubly Linked List | 13:45 |
102 | Delete Entire Doubly Linked List | 07:25 |
103 | Time and Space Complexity of Doubly Linked List | 05:28 |
104 | Create Doubly Linked List | 12:22 |
105 | Insertion Circular Doubly Linked List | 10:30 |
106 | Insertion Algorithm Circular Doubly Linked List | 06:59 |
107 | Insertion Method Circular Doubly Linked List | 13:55 |
108 | Traversal of Circular Doubly Linked List | 08:32 |
109 | Reverse Traversal of Circular Doubly Linked List | 08:18 |
110 | Search for a Node in Circular Doubly Linked List | 09:00 |
111 | Deletion in Circular Doubly Linked List | 10:54 |
112 | Deletion Algorithm in Circular Doubly Linked List | 06:48 |
113 | Deletion Method in Circular Doubly Linked List | 11:52 |
114 | Delete Entire Circular Doubly Linked List | 07:41 |
115 | Time and Space Complexity of Circular Doubly Linked List | 04:35 |
116 | Time Complexity of Array vs Linked List | 07:13 |
117 | Linked List Class | 10:19 |
118 | Solution - Remove Dups | 10:52 |
119 | Solution - Return Nth to Last | 09:20 |
120 | Solution - Partition | 09:37 |
121 | Solution - Sum Lists | 10:52 |
122 | Solution - Intersection | 13:21 |
123 | What and Why of Stack? | 05:28 |
124 | Stack Operations | 06:33 |
125 | Stack using Array vs Linked List | 01:33 |
126 | Stack Operations using Array (Create, isEmpty, isFull) | 08:23 |
127 | Stack Operations using Array (Push, Pop, Peek, Delete) | 12:41 |
128 | Time and Space Complexity of Stack using Array | 02:49 |
129 | Stack Operations using Linked List | 06:47 |
130 | Stack methods - Push , Pop, Peek, Delete and isEmpty using Linked List | 18:12 |
131 | Time and Space Complexity of Stack using Linked List | 03:22 |
132 | When to Use/Avoid Stack | 02:15 |
133 | What is a Queue? | 06:33 |
134 | Linear Queue Operations using Array | 00:00 |
135 | Create, isFull, isEmpty and enQueue methods using Linear Queue Array | 14:45 |
136 | Dequeue, Peek and Delete Methods using Linear Queue Array | 10:46 |
137 | Time and Space Complexity of Linear Queue using Array | 03:49 |
138 | Why Circular Queue? | 04:37 |
139 | Circular Queue Operations using Array | 08:00 |
140 | Create, Enqueue, isFull and isEmpty Methods in Circular Queue using Array | 18:20 |
141 | Dequeue, Peek and Delete Methods in Circular Queue using Array | 12:22 |
142 | Time and Space Complexity of Circular Queue using Array | 03:56 |
143 | Queue Operations using Linked List | 07:54 |
144 | Create, Enqueue and isEmpty Methods in Queue using Linked List | 10:45 |
145 | Dequeue, Peek and Delete Methods in Queue using Linked List | 10:28 |
146 | Time and Space Complexity of Queue using Linked List | 03:04 |
147 | Array vs Linked List Implementation | 02:28 |
148 | When to Use/Avoid Queue? | 01:57 |
149 | Goals | 01:04 |
150 | Solution - Three in One | 23:49 |
151 | Solution - Stack Minimum | 12:48 |
152 | Solution Part 1 - Stack of Plates | 14:29 |
153 | Solution Part 2 - Stack of Plates | 10:57 |
154 | Solution Part 3 - Follow UP | 08:11 |
155 | Solution - Queue via Stacks | 11:50 |
156 | Solution - Animal Shelter | 24:49 |
157 | What is a Tree? | 07:26 |
158 | Why Tree? | 02:41 |
159 | Tree Terminology | 05:17 |
160 | Creating a Basic Tree in Java | 09:44 |
161 | What is A Binary Tree? | 03:10 |
162 | Types of Binary Tree | 03:50 |
163 | Binary Tree Representation | 07:35 |
164 | Create Binary Tree using Linked List | 06:10 |
165 | PreOrder Traversal in Binary Tree using Linked List | 12:02 |
166 | InOrder Traversal in Binary Tree using Linked List | 08:49 |
167 | PostOrder Traversal in Binary Tree using Linked List | 07:03 |
168 | LevelOrder Traversal in Binary Tree using Linked List | 08:48 |
169 | Search Method in Binary Tree (Linked List) | 09:31 |
170 | Insert Method in Binary Tree (Linked List) | 12:37 |
171 | Delete a Node in Binary Tree (Linked List) | 20:30 |
172 | Delet Entire Binary Tree (Linked List) | 02:40 |
173 | Create Binary Tree (Array) | 07:28 |
174 | Insert Method Binary Tree (Array) | 07:57 |
175 | PreOrder Traversal Binary Tree (Array) | 07:26 |
176 | InOrder Traversal Binary Tree (Array) | 06:25 |
177 | PostOrder Traversal Binary Tree (Array) | 05:24 |
178 | levelOrder Traversal Binary Tree (Array) | 05:46 |
179 | Search Method Binary Tree (Array) | 07:07 |
180 | Delete a Node Binary Tree (Array) | 06:33 |
181 | Delete Binary Tree (Array) | 03:07 |
182 | Array vs Linked List in Binary Tree Implementation | 06:01 |
183 | What is a Binary Search Tree? Why do we need it? | 03:29 |
184 | Create Binary Search Tree | 04:38 |
185 | Insert a Node to Binary Search Tree | 12:07 |
186 | PreOrder Traversal Binary Search Tree | 04:45 |
187 | InOrder Traversal Binary Search Tree | 04:10 |
188 | PostOrder Traversal Binary Search Tree | 03:41 |
189 | Level Order Traversal Binary Search Tree | 05:12 |
190 | Search in Binary Search Tree | 07:42 |
191 | Delete a Node in Binary Search Tree | 17:19 |
192 | Delete BST | 02:51 |
193 | Time and Space Complexity of BST | 03:37 |
194 | What is an AVL Tree? | 07:24 |
195 | Why Do We Need AVL Tree? | 04:00 |
196 | Common Operations on AVL Tree | 12:19 |
197 | Insert a Node in AVL (Left Left Condition) | 13:33 |
198 | Insert a Node in AVL (Left Right Condition) | 08:31 |
199 | Insert a Node in AVL (Right Right Condition) | 08:01 |
200 | Insert a Node in AVL (Right Left Condition) | 06:37 |
201 | Insert a Node in AVL (All Together) | 14:27 |
202 | Insert a Node in AVL (Method in Practice) | 21:06 |
203 | Delete a Node from AVL (LL, LR, RR, RR) | 10:05 |
204 | Delete a Node from ALL (All Together) | 07:05 |
205 | Delete a Node from AVL (Method in practice) | 13:42 |
206 | Delete Entire AVL Tree | 02:11 |
207 | Time and Space Complexity of AVL | 03:03 |
208 | Binary Search Tree vs AVL | 02:57 |
209 | What is Binary Heap? Why do we need Binary Heap? | 07:40 |
210 | Common Operations on Binary Heap | 18:48 |
211 | Insert a Node in Binary Heap | 14:46 |
212 | Extract a Node from Binary Heap | 19:20 |
213 | Delete Entire Binary Heap | 02:46 |
214 | Time and Space Complexity of Binary Heap | 05:31 |
215 | What is a Trie? Why we need Trie? | 10:58 |
216 | Common Operations on a Trie (Creation) | 06:24 |
217 | Insert a String in Trie | 14:42 |
218 | Search for a String in Trie | 13:01 |
219 | Delete a String from Trie | 16:58 |
220 | Practical Uses of Trie | 02:15 |
221 | What is Hashing? Why we need it? | 05:38 |
222 | Hashing Terminology | 04:46 |
223 | Hash Functions | 09:47 |
224 | Types of Collision Resolution Techniques - Direct Chaining (Insert) | 16:52 |
225 | Direct Chaining Implementation - (Search, Delete) | 07:29 |
226 | Hash Table is Full | 04:41 |
227 | Collision Resolution Technique - Linear Probing (Insert) | 17:45 |
228 | Collision Resolution Technique - Linear Probing (Search, Delete) | 08:39 |
229 | Collision Resolution Technique - Open Addressing : Quadratic Probing | 09:12 |
230 | Collision Resolution Technique - Open Addressing : Double Hashing | 13:50 |
231 | Pros and Cons of Resolution Techniques | 05:11 |
232 | Practical Use of Hashing | 04:18 |
233 | Hashing vs Other DS | 02:10 |
234 | What is Sorting? | 03:00 |
235 | Types of Sorting | 06:52 |
236 | Sorting Terminology | 05:10 |
237 | Bubble Sort | 15:45 |
238 | Selection Sort | 11:35 |
239 | Insertion Sort | 13:51 |
240 | Bucket Sort | 21:11 |
241 | Merge Sort | 15:49 |
242 | Quick Sort | 22:11 |
243 | Heap Sort | 18:21 |
244 | Comparison of Sorting Algorithms | 01:30 |
245 | Introduction To Search Algorithms | 01:45 |
246 | Linear Search | 04:01 |
247 | Linear Search in Java | 07:12 |
248 | Binary Search | 03:04 |
249 | Binary Search in Java | 13:04 |
250 | Time Complexity of Binary Search | 05:15 |
251 | What you will learn | 01:41 |
252 | What is a Graph? Why do we need Graph | 04:50 |
253 | Graph Terminology | 05:06 |
254 | Types of Graph | 05:47 |
255 | Graph Representation | 09:55 |
256 | Graph in Java using Adjacency Matrix | 12:57 |
257 | Graph in Java using Adjacency List | 09:51 |
258 | Breadth First Search Algorithm (BFS) | 10:37 |
259 | Breadth First Search Algorithm (BFS) in Java - Adjacency Matrix | 11:22 |
260 | Breadth First Search Algorithm (BFS) in Java - Adjacency List | 06:30 |
261 | Time Complexity of Breadth First Search (BFS) Algorithm | 03:25 |
262 | Depth First Search (DFS) Algorithm | 07:23 |
263 | Depth First Search (DFS) Algorithm in Java - Adjacency List | 06:35 |
264 | Depth First Search (DFS) Algorithm in Java - Adjacency Matrix | 06:35 |
265 | Time Complexity of Depth First Search (DFS) Algorithm | 03:08 |
266 | BFS Traversal vs DFS Traversal | 04:33 |
267 | What is Topological Sort? | 02:57 |
268 | Topological Sort Algorithm | 09:14 |
269 | Topological Sort using Adjacency List | 10:56 |
270 | Topological Sort using Adjacency Matrix | 07:24 |
271 | Time and Space Complexity of Topological Sort | 03:14 |
272 | What is Single Source Shortest Path Problem? | 04:46 |
273 | Breadth First Search (BFS) for Single Source Shortest Path Problem (SSSPP) | 07:04 |
274 | BFS for SSSPP in Java using Adjacency List | 11:09 |
275 | BFS for SSSPP in Java using Adjacency Matrix | 07:25 |
276 | Time and Space Complexity of BFS for SSSPP | 02:51 |
277 | Why does BFS not work with Weighted Graph? | 03:38 |
278 | Why does DFS not work for SSSP? | 02:16 |
279 | Dijkstra's Algorithm for SSSPP | 06:23 |
280 | Dijkstra's Algorithm in Java - 1 | 12:36 |
281 | Dijkstra's Algorithm in Java - 2 | 08:47 |
282 | Dijkstra's Algorithm with Negative Cycle | 03:47 |
283 | Bellman Ford Algorithm for SSSPP | 10:56 |
284 | Bellman Ford Algorithm with Negative Cycle | 05:19 |
285 | Why Bellman Ford runs V-1 times? | 04:21 |
286 | Bellman Ford Algorithm Implementation in Java | 10:46 |
287 | BFS vs Dijkstra vs Bellman Ford | 03:38 |
288 | What is All Pairs Shortest Path Problem? | 04:24 |
289 | Dry Run for All Pairs Shortest Path Problem | 04:25 |
290 | Floyd Warshall Algorithm | 09:11 |
291 | Why Floyd Warshall Algorithm? | 04:50 |
292 | Floyd Warshall with Negative Cycle | 02:34 |
293 | Floyd Warshall in Java | 12:28 |
294 | BFS vs Dijkstra vs Bellman Ford vs Floyd Warshall Algorithms | 02:43 |
295 | What is Minimum Spanning Tree? | 04:34 |
296 | What is Disjoint Set? | 02:56 |
297 | Disjoint Set in Java | 11:51 |
298 | Kruskal Algorithm | 09:12 |
299 | Kruskal Algorithm in Java | 15:46 |
300 | Prim's Algorithm | 05:42 |
301 | Prim's Algorithm in Java | 09:59 |
302 | Kruskal vs Prim's Algorithms | 03:35 |
303 | Introduction | 00:40 |
304 | Solution to Route Between Nodes | 10:52 |
305 | What is Greedy Algorithm? | 05:37 |
306 | Known Greedy Algorithms | 08:31 |
307 | Activity Selection Problem | 05:37 |
308 | Activity Selection Problem in Java | 17:07 |
309 | Coin Change Problem | 04:27 |
310 | Coin Change Problem in Java | 08:11 |
311 | Fractional Knapsack Problem | 05:23 |
312 | Fractional Knapsack Problem in Java | 17:09 |
313 | What is a Divide and Conquer Algorithm? | 06:59 |
314 | Common Divide and Conquer Algorithms | 06:52 |
315 | How to solve Fibonacci series using Divide and Conquer approach? | 05:00 |
316 | Number Factor | 06:27 |
317 | Number Factor in Java | 05:45 |
318 | House Robber | 07:11 |
319 | House Robber in Java | 06:29 |
320 | Convert One String to Another | 06:16 |
321 | Convert One String to Another in Java | 07:56 |
322 | Zero One Knapsack Problem | 05:11 |
323 | Zero One Knapsack Problem in Java | 07:51 |
324 | Longest Common Subsequence Problem | 06:23 |
325 | Longest Common Subsequence Problem in Java | 07:04 |
326 | Longest Palindromic Subsequence Problem | 05:48 |
327 | Longest Palindromic Subsequence Problem in Java | 06:20 |
328 | Minimum Cost to Reach Last Cell | 05:03 |
329 | Minimum Cost to Reach Last Cell in Java | 05:07 |
330 | Number of Paths To Reach The Last Cell with Given Cost | 05:17 |
331 | Number of Paths To Reach The Last Cell with Given Cost in Java | 06:10 |
332 | What is Dynamic Programming? (Overlapping property) | 04:58 |
333 | Where Does the Name of DP Come From? | 02:09 |
334 | Top Down with Memoization | 11:28 |
335 | Bottom Up with Tabulation | 07:44 |
336 | Top Down vs Bottom Up | 04:18 |
337 | Is Merge Sort Dynamic Programming? | 04:31 |
338 | Number Factor Problem using Dynamic Programming | 12:27 |
339 | Number Factor : Top Down and Bottom Up | 08:34 |
340 | House Robber Problem using Dynamic Programming | 10:24 |
341 | Convert one string to another using Dynamic Programming | 05:30 |
342 | Introduction | 02:58 |
343 | Step 1 - Understand the Problem | 06:02 |
344 | Step 2 - Examples | 06:41 |
345 | Step 3 - Break it Down | 07:44 |
346 | Step 4 - Solve or Simplify | 10:56 |
347 | Step 5 - Look Back and Refactor | 07:34 |
348 | What is Backtracking? | 10:02 |
349 | Backtracking vs Brute Force | 04:35 |
350 | N - Queens Problem | 09:39 |
Similar courses to Java Data Structures and Algorithms Masterclass
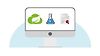
Spring Professional Certification Exam Tutorial - Module 08udemy
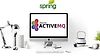
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
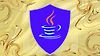
Java Programming Bootcamp: Zero to Masteryzerotomastery.io
![gRPC [Java] Master Class: Build Modern API & Micro services](https://cdn.courseflix.net/courses/100x56/grpc-java-master-class-build-modern-api-micro-services.jpg?d=1747071339854)
gRPC [Java] Master Class: Build Modern API & Micro servicesudemy
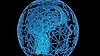
Master Microservices with Java, Spring, Docker, Kubernetesudemy
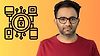
Spring Security 6 Zero to Master along with JWT,OAUTH2udemy
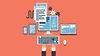
Hibernate and Java Persistence API (JPA) Fundamentalsudemy
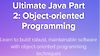
Ultimate Java Part 2: Object-oriented Programmingcodewithmosh (Mosh Hamedani)
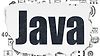