Ultimate Java Part 2: Object-oriented Programming
3h 41m 47s
English
Paid
April 19, 2024
Heard about object-oriented programming, but not sure what it is? Object-oriented programming (OOP) is a popular style of programming. It comes up in technical interviews often and it's an essential skill for every developer. The problem is that there’s lots of bad info out there about OOP. Lots of myths, misconceptions, bad advice and downright poor tutorials and books. It took me a long time to really get it!
Watch Online Ultimate Java Part 2: Object-oriented Programming
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | 1- Introduction | 00:35 |
2 | 2- Programming Paradigms | 03:41 |
3 | 3- Benefits of Object-oriented Programming | 02:13 |
4 | 4- Course Structure | 01:49 |
5 | 1- Introduction | 00:43 |
6 | 2- Classes and Objects | 03:14 |
7 | 3- Creating Classes | 04:25 |
8 | 4- Creating Objects | 04:31 |
9 | 5- Memory Allocation | 03:54 |
10 | 6- Procedural Programming | 05:38 |
11 | 7- Encapsulation | 04:38 |
12 | 8- Getters and Setters - Title | 07:28 |
13 | 9- Abstraction | 02:25 |
14 | 10- Coupling | 04:19 |
15 | 11- Reducing Coupling | 07:18 |
16 | 12- Constructors | 05:14 |
17 | 13- Method Overloading | 02:53 |
18 | 14- Constructor Overloading | 02:45 |
19 | 15- Static Members | 04:23 |
20 | 17- Summary | 00:51 |
21 | 1- Introduction | 00:55 |
22 | 2- The Problem | 02:41 |
23 | 3- What Classes Do We Need? | 03:42 |
24 | 4- Extracting the Console Class | 02:59 |
25 | 5- Overloading Methods | 02:40 |
26 | 6- Extracting the MortgageReport Class | 01:26 |
27 | 7- Extracting the MortgageCalculator Class | 06:18 |
28 | 8- Moving Away from Static Members | 05:56 |
29 | 9- Moving Static Fields | 02:19 |
30 | 10- Extracting Duplicate Logic | 04:57 |
31 | 11- Extracting getRemainingBalances - Title | 06:14 |
32 | 12- One Last Touch | 02:02 |
33 | 1- Introduction | 00:26 |
34 | 2- Inheritance | 03:50 |
35 | 3- The Object Class | 05:00 |
36 | 4- Constructors and Inheritance | 02:42 |
37 | 5- Access Modifiers | 03:57 |
38 | 6- Overriding Methods | 02:51 |
39 | 7- Upcasting and Downcasting | 05:21 |
40 | 8- Comparing Objects | 09:49 |
41 | 9- Polymorphism | 05:24 |
42 | 10- Abstract Classes and Methods | 02:23 |
43 | 11- Final Classes and Methods | 02:49 |
44 | 12- Deep Inheritance Hierarchies | 02:49 |
45 | 13- Multiple Inheritance | 02:04 |
46 | 15- Summary | 01:11 |
47 | 1- Introduction | 00:37 |
48 | 2- What are Interfaces | 06:05 |
49 | 3- Tightly-coupled Code | 03:57 |
50 | 4- Creating an Interface | 05:24 |
51 | 5- Dependency Injection | 01:30 |
52 | 6- Constructor Injection | 03:21 |
53 | 7- Setter Injection | 02:40 |
54 | 8- Method Injection | 02:28 |
55 | 9- Interface Segregation Principle | 07:11 |
56 | 10- Project- MyTube Video Platform | 03:34 |
57 | 11- Solution | 05:09 |
58 | 12- Fields | 03:14 |
59 | 13- Static Methods | 02:53 |
60 | 14- Private Methods | 02:04 |
61 | 15- Interfaces and Abstract Classes | 02:11 |
62 | 16- When to Use Interfaces | 03:14 |
63 | 18- Course Wrap Up | 00:33 |
Similar courses to Ultimate Java Part 2: Object-oriented Programming
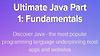
Ultimate Java Part 1: Fundamentals
Duration 3 hours 21 minutes 58 seconds
Course
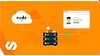
Machine Learning with Javascript
Duration 17 hours 42 minutes 20 seconds
Course
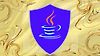
Java Programming Bootcamp: Zero to Mastery
Duration 9 hours 15 minutes 36 seconds
Course
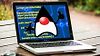
Java Programming Masterclass covering Java 11 & Java 17
Duration 80 hours 13 minutes 14 seconds
Course
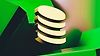
RESTful Web Services, Java, Spring Boot, Spring MVC and JPA
Duration 25 hours 11 minutes 7 seconds
Course
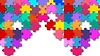
Java Design Patterns & SOLID Design Principles
Duration 17 hours 5 minutes 6 seconds
Course
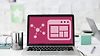
Data Structures and Algorithms: Deep Dive Using Java
Duration 15 hours 53 minutes 4 seconds
Course
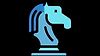
Master Spring 6 Spring Boot 3 REST JPA Hibernate
Duration 36 hours 15 minutes 18 seconds
Course
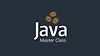
Java Master Class
Duration 24 hours 40 minutes 37 seconds
Course
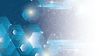
Java Multithreading, Concurrency & Performance Optimization
Duration 5 hours 16 minutes 23 seconds
Course