2D Game Physics Programming
This course is a gentle introduction into the world of 2D game physics! We'll review all the beautiful math that provides the foundation for most physics engines, starting with a strong review of vectors, matrices, basic trigonometry, rigid-body collision, and touching a little bit of calculus as well.
Read more about the course
The lectures are designed to teach all concepts from first principles. In our journey, we'll review several topics from physics, like velocity, acceleration, integration, mass, forces, gravity, drag, friction, rigid body dynamics, collision detection, constraints, etc.
We'll also put theory into practice by coding a very simple 2D physics engine from scratch using the C++ programming language.
We'll start by writing a simulation of particle physics, which is a good start for us to address concepts like movement, forces, displacement, and integration.
We'll then proceed to work with rigid bodies by adding shapes to our objects, like circles, rectangles, and polygons. We'll also learn how to code the collision detection and collision resolution between these rigid bodies.
We'll conclude our C++ implementation by adding constraints to our physics engine, which will help us add different types of objects to our engine, like joints and ragdolls. Ultimately, constraints will help us improve the stability of our engine, and they are a great opportunity for us to discuss some interesting ideas from calculus.
The tools you'll need
All you really need is a simple code editor and a C++ compiler. We'll use a cross-platform library called SDL to display our graphics, and since we can find a C++ compiler for virtually any operating system, you can follow along on either Windows, macOS, or Linux!
Also, make sure you have pen and paper ready for the lectures. This course will probably be a little bit different than other programming courses you took before. We will take our sweet time and make sure we understand every formula we find along the way!
Watch Online 2D Game Physics Programming
# | Title | Duration |
---|---|---|
1 | Introduction & Learning Outcomes | 08:56 |
2 | How to Take this Course | 02:59 |
3 | What is Game Physics | 04:45 |
4 | A Quick Review of Vector Math | 01:30 |
5 | Vector & Scalar Quantities | 11:02 |
6 | Using the P5js Web Editor | 06:36 |
7 | Visualizing Errors in the Web Editor | 01:08 |
8 | Coding a Vec2 Class | 06:24 |
9 | Vector Magnitude | 09:08 |
10 | Vector Addition & Subtraction | 08:37 |
11 | Methods for Vector Addition & Subtraction | 05:56 |
12 | Vector Equality | 02:15 |
13 | Scaling Vectors | 04:51 |
14 | Static Methods | 04:10 |
15 | Applications of Vector Addition & Subtraction | 08:01 |
16 | Dot Product | 09:22 |
17 | Is the Dot Product Commutative? | 04:01 |
18 | Cross Product | 10:14 |
19 | Coding the Cross Product Method | 05:20 |
20 | Exercise: Perpendicular 2D Vector | 02:39 |
21 | Perpendicular 2D Vector | 02:03 |
22 | Exercise: Vec3 Methods | 01:12 |
23 | Vec3 Methods | 05:15 |
24 | Vector Normalization | 07:23 |
25 | Coding the Normalization Method | 03:38 |
26 | Scaling, Translating, and Rotating Vectors | 08:01 |
27 | Quick Review of Sine & Cosine | 07:38 |
28 | Vector Rotation Proof (x-component) | 20:20 |
29 | Vector Rotation Proof (y-component) | 07:17 |
30 | Coding the Vec2 Rotate Method | 09:23 |
31 | Concluding our JavaScript Vector Class | 02:53 |
32 | Vec2 C++ Header File | 06:14 |
33 | A Quick Look at C++ Vec2 Syntax | 17:39 |
34 | Vec2 Operator Overloading | 09:07 |
35 | Technologies & Dependencies | 08:10 |
36 | Folder Structure | 11:18 |
37 | Initial Project Files | 23:14 |
38 | Compiling using GCC & Linux | 08:10 |
39 | Makefile | 06:05 |
40 | Configuring Visual Studio on Windows | 14:04 |
41 | Introduction to Particle Physics | 11:58 |
42 | Particle Class | 10:15 |
43 | Particle Velocity | 05:57 |
44 | Using the + Operator to Add Vectors | 01:47 |
45 | Controlling our Framerate | 13:07 |
46 | Framerate Independent Movement | 11:48 |
47 | Clamping Invalid DeltaTime Values | 02:51 |
48 | Moving in a Constant Velocity | 06:20 |
49 | Changing the Particle's Velocity | 12:21 |
50 | Keeping the Particle Inside the Window | 08:35 |
51 | Constant Acceleration | 05:26 |
52 | Discrete vs. Continuous | 12:54 |
53 | Integration & Movement Simulation | 16:02 |
54 | Different Integration Methods | 10:00 |
55 | Particle Integrate Function | 04:27 |
56 | Applying Forces to Particles | 11:42 |
57 | Function to Add Force | 09:25 |
58 | Particles with Different Mass | 13:08 |
59 | The Weight Force | 08:21 |
60 | Inverse of the Mass | 04:18 |
61 | Applying Forces with the Keyboard | 05:57 |
62 | Drag Force | 15:21 |
63 | Drag Force Function | 14:51 |
64 | Handling Mouse Clicks with SDL | 04:38 |
65 | Unexpected Drag Behavior | 02:15 |
66 | Friction Force | 08:47 |
67 | Friction Force Function | 07:56 |
68 | Gravitational Attraction Force | 13:16 |
69 | Gravitational Attraction Force Function | 15:59 |
70 | Spring Force | 14:35 |
71 | Spring Force Function | 17:49 |
72 | Exercise: Spring Forces | 09:04 |
73 | Multiple Particles: Chain | 14:15 |
74 | Multiple Particles: Soft body | 08:20 |
75 | Soft Bodies & Verlet Integration | 08:58 |
76 | Rigid-Bodies | 14:04 |
77 | Shapes | 07:39 |
78 | Shape Class | 07:51 |
79 | Shape Class Implementation | 17:36 |
80 | Circle Shape | 10:06 |
81 | Angular Velocity & Angular Acceleration | 11:32 |
82 | Torque & Moment of Inertia | 18:25 |
83 | Circle Shape Angular Motion | 22:48 |
84 | Box Vertices | 19:13 |
85 | Local Space vs. World Space | 17:04 |
86 | Body Update Function | 03:48 |
87 | Why Not a Shape Draw Function? | 02:29 |
88 | No Draw Method in the Shape Class | 02:01 |
89 | Circle-Circle Collision Detection | 10:11 |
90 | Circle-Circle Collision Class | 12:20 |
91 | Circle-Circle Collision Implementation | 09:57 |
92 | Collision Contact Information | 09:46 |
93 | Collision Information Code | 29:06 |
94 | Broad Phase & Narrow Phase | 10:52 |
95 | The Projection Method | 15:17 |
96 | Objects with Infinite Mass | 14:41 |
97 | Impulse Method & Momentum | 10:02 |
98 | Impulse | 10:09 |
99 | Deriving the Linear Impulse Formula | 21:39 |
100 | Simplifying the Impulse Method Formula | 09:42 |
101 | Coding the Linear Impulse Method | 13:07 |
102 | Is Linear Collision Response Enough? | 06:10 |
103 | AABB Collision Detection | 12:27 |
104 | SAT: Separating Axis Theorem | 20:02 |
105 | Finding Minimum Separation with SAT | 11:41 |
106 | Polygon-Polygon Collision Code | 12:53 |
107 | Code to Find SAT Minimum Separation | 28:35 |
108 | Refactoring the SAT Separation Function | 07:45 |
109 | Finding Extra Collision Information with SAT | 14:57 |
110 | Polygon-Polygon Collision Information | 16:42 |
111 | Linear & Angular Velocity At Point | 11:53 |
112 | Post-Collision Velocity At Point | 14:24 |
113 | Computing Linear & Angular Impulse | 12:06 |
114 | Collision Distance Vectors Ra-Rb | 18:21 |
115 | 2D Cross Product Simplification | 19:58 |
116 | Coding the Impulse Along Normal | 15:11 |
117 | Exercise: Impulse Along Tangent | 12:57 |
118 | Friction Impulse Along Tangent | 11:41 |
119 | Removing Window Boundaries Check | 04:16 |
120 | Circle-Polygon Collision Detection | 10:13 |
121 | Finding Polygon's Nearest Edge with Circle | 07:11 |
122 | Exercise: Circle-Polygon Edge Regions | 05:41 |
123 | Circle-Polygon Collision Information | 26:25 |
124 | Circle-Polygon Collision Resolution | 07:57 |
125 | Exercise: Polygons with Multiple Vertices | 16:08 |
126 | Polygon with Multiple Vertices | 04:55 |
127 | Loading SDL Textures | 12:58 |
128 | Rendering Circle Texture | 08:58 |
129 | World Class | 14:27 |
130 | Implementing World Functions | 15:53 |
131 | Refactoring Function to Update Vertices | 08:32 |
132 | Local Solvers vs. Global Solvers | 08:09 |
133 | A Naive Iterative Positional Correction | 12:08 |
134 | Constrained Rigid-Body Physics | 18:41 |
135 | Position vs. Velocity Constraints | 15:15 |
136 | Example Velocity Constraint & Bias Factor | 10:50 |
137 | Example Distance Constraint & Bias Factor | 08:22 |
138 | Constraint Forces & Constrained Movement | 13:58 |
139 | Force-Based vs. Impulse-Based Constraints | 15:59 |
140 | The Constraint Class | 07:04 |
141 | VecN Class | 15:02 |
142 | Implementing VecN Functions | 09:15 |
143 | VecN Operator Overloading | 07:31 |
144 | Matrices | 18:44 |
145 | MatMN Class | 08:07 |
146 | Matrix Transpose | 05:28 |
147 | Matrix Multiplication | 10:32 |
148 | Matrix Multiplication Function | 04:49 |
149 | Seeing Beyond the Matrix | 03:54 |
150 | Generalized Velocity Constraint | 18:41 |
151 | Solving Violated Velocity Constraints | 15:55 |
152 | Constraint Class Inheritance | 10:08 |
153 | Distance Constraint | 16:26 |
154 | Joint Constraint Class | 07:20 |
155 | Converting World Space to Local Space | 05:46 |
156 | World List of Constraints | 10:37 |
157 | Refactoring Body Update | 21:17 |
158 | Deriving the Distance Jacobian | 22:34 |
159 | Populating the Distance Jacobian | 13:19 |
160 | Solving System of Equations (Ax=b) | 20:21 |
161 | Gauss-Seidel Method | 05:23 |
162 | Constrained Pendulum | 12:55 |
163 | Solving System of Constraints Iteratively | 14:28 |
164 | Warm Starting | 17:20 |
165 | Adding the Bias Term | 08:13 |
166 | Ragdoll with Joint Constraints | 12:04 |
167 | Preventing NaN Errors | 03:31 |
168 | Penetration Constraint | 13:47 |
169 | Deriving the Penetration Jacobian | 09:52 |
170 | Penetration Constraint Class | 27:10 |
171 | Solving Penetration Constraints | 05:56 |
172 | Penetration Warm Starting | 05:15 |
173 | Penetration Constraint Friction | 14:17 |
174 | Clamping Friction Magnitude Values | 08:02 |
175 | Penetration Constraint Bounciness | 09:06 |
176 | Unstable Stack of Boxes | 12:51 |
177 | Allowing for Multiple Contact Points | 12:42 |
178 | Reference & Incident Edges | 10:38 |
179 | Finding Incident Edge | 21:52 |
180 | Getting Ready for Clipping | 18:40 |
181 | Clipping Function | 17:08 |
182 | Testing Multi-Contact Boxes | 04:09 |
183 | Testing Multiple Objects & Constraints | 10:05 |
184 | Contact Caching | 14:07 |
185 | Continuous Collision Detection | 06:50 |
186 | Broad & Narrow Split | 04:16 |
187 | Euler Integration Review | 23:58 |
188 | MidPoint & RK4 Integrators | 10:25 |
189 | Verlet Integration | 20:43 |
190 | Stick Constraints | 20:15 |
191 | Conclusion & Next Steps | 12:35 |
Similar courses to 2D Game Physics Programming
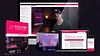
Full Time Game DevThomas Brush
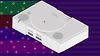
PS1 Programming with MIPS Assembly & CGustavo Pezzi
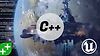
Unreal Engine C++ Developer: Learn C++ and Make Video Gamesudemy
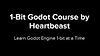
1-Bit Godot Course by Heartbeastheartgamedev.com (Benjamin Anderson)
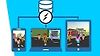
Create a 3D multi-player game using THREE.js and Socket.IOudemy
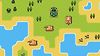
C++ Game Engine ProgrammingGustavo Pezzi
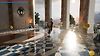
Unreal Engine C++ The Ultimate Game Developer Courseudemy
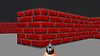
Raycasting Engine ProgrammingGustavo Pezzi
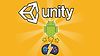
Unity Android : Build 8 Mobile Games with Unity & C#udemy
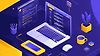