C++ Game Engine Programming
This course provides a gentle and comprehensive introduction to the basics of 2D game engine architecture. We will cover several popular programming patterns used in game development and try to apply all the theory in practice by writing a small 2D game engine using modern C++, SDL, and Lua.
We will write a small ECS framework from scratch to manage entities, components, and systems. We will discuss how engine developers design their code and how to organize game objects in memory with performance in mind.
Read more about the course
Tools You Will Need
Tools for a C++ Game Engine:
- C++: A powerful compiled programming language.
- SDL2: A library for cross-platform rendering and input.
- GLM: A library for mathematical calculations.
- Dear ImGui: A library for creating engine tool interfaces.
- Sol: A library for binding modern C++ and Lua.
- Lua: A fast and easy-to-use scripting language.
We will try to write most of our engine code from scratch. All these libraries and tools are cross-platform, so you will be able to write code on Windows, macOS, or Linux!
Is This Course Right for You?
Target Audience: Beginner programmers looking to learn C++ in the context of game development. Students should be able to write basic code (if-else, loops, functions, classes) and be familiar with OOP.
Required Knowledge: You do not need to know C++ before starting the course. Many successful students have had experience developing web, mobile, and game applications in languages like Java, Python, Ruby, Go, Swift, JavaScript, and others.
Course Differences
The course does not just teach how to create a game in C++. It allows you to understand the abstraction of the game and write a small engine from scratch in C++, covering ECS, data-oriented design, C++ templates, the game loop, SDL rendering, event systems, resource management, memory, and performance. It also includes integrating Lua into C++ code to add scripting capabilities.
Although there are other resources on game engine development, they are either too theoretical or excessively long. If you are looking for a gentle introduction to the world of game engine programming and want to understand how games work from the inside out, this course is for you!
Watch Online C++ Game Engine Programming
# | Title | Duration |
---|---|---|
1 | Motivations & Learning Outcomes | 16:29 |
2 | How to Take this Course | 02:59 |
3 | Project Technologies & Libraries | 22:06 |
4 | Linux Dependencies | 06:24 |
5 | macOS Dependencies | 02:36 |
6 | A Small Note for Windows Programmers | 03:40 |
7 | Compilation | 11:11 |
8 | Header & Implementation Files | 06:14 |
9 | Linking | 11:39 |
10 | Makefile | 08:36 |
11 | Lua Version & Lua Header Files | 04:48 |
12 | Compiling & Testing All Dependencies | 15:43 |
13 | Configuring Visual Studio on Windows | 34:22 |
14 | Library Binaries | 09:33 |
15 | Game Loop | 08:49 |
16 | Game Class | 18:06 |
17 | Creating an SDL Window | 15:46 |
18 | Polling SDL Events | 13:41 |
19 | Rendering our SDL Window | 06:51 |
20 | Fullscreen Window | 08:31 |
21 | Fake Fullscreen vs. Real Fullscreen | 03:54 |
22 | Drawing an SDL Rectangle | 06:41 |
23 | Double-Buffered Renderer | 02:33 |
24 | Loading PNG Textures | 10:15 |
25 | Object Movement & Velocity Vectors | 11:06 |
26 | Capping the Game Framerate | 15:20 |
27 | SDL Delay | 07:39 |
28 | Delta Time | 13:29 |
29 | Uncapped Framerate | 01:44 |
30 | Logger Class | 12:25 |
31 | Logger Class Implementation | 18:33 |
32 | Popular Logging Libraries | 04:41 |
33 | Source Subfolders | 05:13 |
34 | Makefile Variables | 12:27 |
35 | Creating C++ Objects | 15:24 |
36 | Examples of C++ Object Creation | 05:37 |
37 | Organizing Game Objects | 07:51 |
38 | Object Inheritance Design | 05:19 |
39 | Component-Based Design | 16:27 |
40 | Entity-Component-System Design | 22:10 |
41 | ECS Folder Structure | 21:03 |
42 | System Component Signature | 17:27 |
43 | Working with C++ Templates | 07:59 |
44 | Component Type Template | 10:42 |
45 | Exercise: System Functions | 06:16 |
46 | Adding & Removing Entities from Systems | 08:14 |
47 | Operator Overloading for Entities | 10:41 |
48 | Component Pool | 11:31 |
49 | The Pool Class | 07:18 |
50 | Implementing the Pool Class | 13:46 |
51 | Registry Systems & Entity Signatures | 10:55 |
52 | Entity Creation & Management | 17:55 |
53 | Function Templates to Manage Components | 12:00 |
54 | Adding Components | 17:55 |
55 | Function to Add Components | 18:24 |
56 | Function to Remove Components | 07:11 |
57 | Templates as Placeholders | 07:55 |
58 | Implementing System Functions | 29:41 |
59 | Creating our First Entity | 11:22 |
60 | Smart Pointers | 14:58 |
61 | Converting ECS Code to Smart Pointers | 11:45 |
62 | SDL Raw Pointers | 03:34 |
63 | Adding our First Components | 10:06 |
64 | Exercise: Entity Class Managing Components | 17:50 |
65 | Entity Class Managing Components | 07:26 |
66 | A Warning About Cyclic Dependencies | 02:20 |
67 | Movement System | 16:03 |
68 | Movement System & Delta Time | 03:30 |
69 | Render System | 17:28 |
70 | Managing Game Assets | 13:56 |
71 | The Asset Store | 23:42 |
72 | Displaying Textures in our Render System | 20:29 |
73 | Exercise: Displaying the Tilemap | 08:19 |
74 | Displaying the Tilemap | 15:07 |
75 | Rendering Order | 17:49 |
76 | Sorting Sprites by Z-Index | 16:22 |
77 | Animated Sprites | 06:35 |
78 | Animation System | 27:43 |
79 | Entity Collision Check | 18:15 |
80 | Implementing the Collision System | 27:56 |
81 | Exercise: Render Collider Rectangle | 02:22 |
82 | Render Collider Rectangle | 06:09 |
83 | Killing Entities & Re-Using IDs | 10:00 |
84 | Implementing Entity Removal | 20:01 |
85 | Introduction to Event Systems | 11:27 |
86 | Event System Design Options | 12:41 |
87 | Starting to Code the Event System | 10:51 |
88 | Event Handlers | 30:38 |
89 | Emitting Events & Subscribing to Events | 19:24 |
90 | Exercise: Key Pressed Event | 03:32 |
91 | Implementing the Key Pressed Event | 07:33 |
92 | Event System Design Patterns | 06:28 |
93 | Keyboard Control System | 21:18 |
94 | Camera Follow System | 24:00 |
95 | Sprites with Fixed Position | 06:09 |
96 | Camera Movement for Colliders | 03:05 |
97 | Projectile Emitter Component | 05:21 |
98 | Health Component | 32:04 |
99 | Projectile Duration | 14:22 |
100 | Exercise: Shooting Projectiles | 03:07 |
101 | Shooting Projectiles | 13:28 |
102 | Tags & Groups | 11:20 |
103 | Optimizing Access of Tags & Groups | 05:49 |
104 | Implementing Tags & Groups | 22:50 |
105 | Projectiles Colliding with Player | 18:59 |
106 | Projectiles Colliding with Enemies | 04:30 |
107 | Error Checking and Validation | 05:21 |
108 | Data-Oriented Design | 18:21 |
109 | Avoiding Data Gaps | 07:30 |
110 | Packed Pool of Components | 20:59 |
111 | Coding Packed Pools of Data | 31:04 |
112 | Checking for Null Pool | 01:55 |
113 | Array of Structs vs. Struct of Arrays | 14:22 |
114 | Cache Profiling with Valgrind | 10:18 |
115 | Popular ECS Libraries | 15:30 |
116 | Adding Fonts to the Asset Store | 13:05 |
117 | Render Text System | 27:01 |
118 | Exercise: Display Health Values | 03:12 |
119 | Rendering Health Values | 20:26 |
120 | Introduction to Dear ImGui | 18:22 |
121 | Dear ImGui Demo Window | 24:19 |
122 | Immediate-Mode GUI Paradigm | 19:41 |
123 | Render GUI System | 17:59 |
124 | Button to Spawn Enemies | 15:19 |
125 | Exercise: Customizing New Enemy | 07:15 |
126 | Custom Enemy Values with ImGui | 33:37 |
127 | Killing Entities Outside Map Limits | 08:27 |
128 | Flipping Sprites on Collision | 17:26 |
129 | Exercise: Keep Player Inside the Map | 01:45 |
130 | Keeping the Player Inside the Map | 03:38 |
131 | Culling Sprites Outside Camera View | 10:41 |
132 | Do Not Cull Fixed Sprites | 01:43 |
133 | Game Scripting | 15:12 |
134 | The Lua Scripting Language | 08:41 |
135 | Lua State | 22:45 |
136 | Reading Lua Tables | 13:09 |
137 | Lua Functions | 16:34 |
138 | Level Loader Class | 19:45 |
139 | Reading Assets from a Lua Table | 27:13 |
140 | Reading Entities from a Lua Table | 21:22 |
141 | Handling Multiple Objects in a Lua Level | 08:33 |
142 | Scripting Night-Day Tilemap | 12:38 |
143 | Scripting Entity Behavior with Lua | 09:55 |
144 | Script System | 13:59 |
145 | Lua Bindings | 18:23 |
146 | Binding Multiple Lua Functions | 12:16 |
147 | Loading Different Lua Levels | 03:54 |
148 | Division of C++ & Lua Code | 04:50 |
149 | Next Steps | 06:26 |
Similar courses to C++ Game Engine Programming
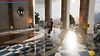
Unreal Engine C++ The Ultimate Game Developer Courseudemy
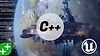
Unreal Engine C++ Developer: Learn C++ and Make Video Gamesudemy
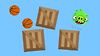