Working with React and Go (Golang)
React and Go are something of a match made in heaven. React is the world's most popular JavaScript library for building Single Page Applications, and Go is uniquely well-suited for building REST back ends. That is what this course is all about. Learn how to develop and deploy a fast, secure web application built using the most popular and in-demand JavaScript front end (React), with one of the most popular and powerful programming languages for the back end (Go).
Read more about the course
React is an open-source, front end JavaScript library for building user interfaces or UI components. React is maintained by Facebook and a community of developers and companies. React can be used as a base in the development of single-page or mobile applications. React is the most popular front-end JavaScript library in the field of web development, and is used by many well-known organizations, including Netflix, Instagram, and the New York Times. With React, we build fast, interactive user interfaces.
Go is a modern, type safe, compiled, and extremely fast programming language. It it is ideally suited for building safe, scalable, incredibly fast REST APIs and web applications, and is also used by large corporations around the wold, including Netflix, Instagram, and the New York Times.
If you were paying attention, you might have noticed some overlap there -- the same large companies using React are also using Go. There is a reason for that, and we will be exploring that reason in this course.
In this course we will go over the core fundamentals of React, including the React life cycle, components, functional components, props, state, and more. We will also cover calling a remote API (both one we build, and a 3rd party API), and much more.
In the first part of the course, we'll build an application using React class components, and then, once that is complete, we'll rebuild the entire application using functions and hooks. Although hooks and functions appear to the the future for React, there are literally millions of lines of code out there built using classes, so it's important to know how to work with React using both classes and functions & hooks.
We will also cover receiving requests on the back end, both as JSON and as GraphQL, and returning that response as JSON.
In order to secure access to authenticated users for certain parts of our site, we will also explore how to generate and use JSON Web Tokens (JWT).
This course is not intended for absolute beginners. I expect that you have some experience in JavaScript and Go, and a good knowledge of HTML.
Watch Online Working with React and Go (Golang)
# | Title | Duration |
---|---|---|
1 | Welcome | 06:13 |
2 | A bit about me | 01:02 |
3 | Asking for help | 01:15 |
4 | Mistakes: we all make them. | 01:07 |
5 | Installing Node | 01:02 |
6 | Installing Go | 01:28 |
7 | Installing Visual Studio Code | 01:57 |
8 | Installing Docker | 00:52 |
9 | How React works | 13:42 |
10 | Hello world with React using Classes | 05:00 |
11 | Hello world with React using functional components | 06:43 |
12 | Styling Components | 03:52 |
13 | Using Bootstrap CSS | 03:09 |
14 | Using props: passing data to components | 04:58 |
15 | React and State I | 09:20 |
16 | React and State II | 06:48 |
17 | React and State III | 09:24 |
18 | React and State IV | 06:19 |
19 | React and State V | 06:46 |
20 | Intercepting form submissions with onSubmit() | 03:22 |
21 | handleSubmit() continued | 05:53 |
22 | Ref: using references in React | 03:30 |
23 | References with components: forwardRef() | 03:21 |
24 | Class Lifecycle | 14:00 |
25 | Getting started with our project | 02:49 |
26 | Setting up the application layout | 11:38 |
27 | Getting started with React Router v6 | 03:58 |
28 | Configuring React Router | 07:15 |
29 | Using React Router's Link | 10:24 |
30 | Working on the Movies component | 07:49 |
31 | Routing from the Movies component to individual movies | 02:23 |
32 | Displaying an individual movie | 05:30 |
33 | Working on the Login button | 05:12 |
34 | Creating the login form | 12:46 |
35 | Giving the Login component access to setJWT | 04:27 |
36 | Adding error messages and redirects to the Login component | 09:02 |
37 | Logging out | 02:35 |
38 | Starting the back end API | 09:56 |
39 | Adding handlers and routes to our API | 06:56 |
40 | Installing a routing package | 04:12 |
41 | Adding a route to our handlers | 02:43 |
42 | Returning JSON from our API | 06:03 |
43 | Returning a list of movies as JSON | 10:52 |
44 | Connecting the front end to the back end API | 04:47 |
45 | Enabling CORS middleware | 07:05 |
46 | Enabling React's proxy to the back end API | 02:51 |
47 | Setting up our database using Docker | 06:13 |
48 | Getting started connecting our API to Postgres | 03:25 |
49 | Installing a database driver and connecting to Postgres | 09:03 |
50 | Setting up a database repository I | 16:00 |
51 | Setting up a database repository II | 05:40 |
52 | Improving the allMovies handler to use our database | 03:07 |
53 | Setting up utils.go and a writeJSON helper function | 07:11 |
54 | Adding a readJSON helper function | 06:20 |
55 | Adding an errorJSON helper function | 02:23 |
56 | Using our errorJSON helper function | 01:32 |
57 | Installing a JWT package | 08:10 |
58 | Generating tokens | 13:12 |
59 | Setting default values for JWT tokens | 06:10 |
60 | Creating a handler to generate a JWT | 03:43 |
61 | Trying out our handler | 02:14 |
62 | Generate refresh token cookie | 05:47 |
63 | Reading a JSON payload in the authenticate handler | 02:08 |
64 | Looking up a user by email | 07:46 |
65 | Validating a password | 06:42 |
66 | Updating the Login component on the front end | 08:46 |
67 | Refresh tokens I | 10:12 |
68 | Refresh tokens II | 04:19 |
69 | Refresh tokens III | 05:32 |
70 | Refresh tokens IV | 09:00 |
71 | Refresh tokens V | 09:34 |
72 | Protecting routes using JWT tokens | 13:43 |
73 | Authentication middleware | 02:44 |
74 | Adding our middleware to routes | 03:10 |
75 | Trying our our protected routes | 09:13 |
76 | Starting on the Add/Edit movie component | 04:13 |
77 | Creating a select component | 05:31 |
78 | Creating a checkbox component | 03:04 |
79 | Using our input controls to create the edit movie form | 13:52 |
80 | Continuing to work on the EditMovie component | 09:01 |
81 | Getting a movie and Genres from the database | 19:34 |
82 | Creating handlers to get movies for public and admin | 08:37 |
83 | Displaying a movie to the public on the front end | 08:52 |
84 | Getting started with showing genres on the Add/Edit movie component | 07:30 |
85 | Adding checkboxes for genres to the front end form | 18:32 |
86 | Enabling checkbox clicks on the EditMovie component | 04:59 |
87 | Validation I | 05:48 |
88 | Validation II | 06:17 |
89 | Creating a database method to insert a movie | 04:18 |
90 | Creating a handler to insert a movie | 03:04 |
91 | Getting a movie image from a remote API I | 12:35 |
92 | Getting a movie image from a remote API II | 02:36 |
93 | Handling genres when inserting a movie | 05:52 |
94 | Inserting a movie from the front end | 07:38 |
95 | Trying out adding a movie | 02:36 |
96 | Editing an existing movie I | 10:02 |
97 | Editing an existing movie II | 01:52 |
98 | Editing an existing movie III | 03:19 |
99 | Writing the handler to update a movie | 05:26 |
100 | Trying out editing a movie from the front end | 03:32 |
101 | Getting started with deleting a movie | 06:20 |
102 | Deleting a movie II | 02:02 |
103 | Deleting a movie III | 02:37 |
104 | Deleting a movie IV | 01:44 |
105 | Getting started with listing movies by Genre | 04:25 |
106 | Creating a handler to list movies by Genre | 03:15 |
107 | Modifying the front end to list movies by Genre | 08:41 |
108 | Creating the OneGenre component | 10:50 |
109 | Getting started with GraphQL | 09:29 |
110 | GraphQL II | 11:43 |
111 | GraphQL III | 04:36 |
112 | Creating a handler for GraphQL | 05:38 |
113 | Connecting to our GraphQL endpoint from the front end | 15:59 |
114 | Adding search functionality | 07:14 |
115 | Configuring environment variables with .env | 07:11 |
116 | Preparing the back end for deployment | 03:36 |
117 | Dumping our database for the live server | 02:16 |
118 | Copying files to the server for deployment | 05:46 |
119 | Populating the database on the live server | 06:28 |
120 | Starting our back end with Supervisor | 07:44 |
121 | Configuring Caddy | 07:46 |
122 | Trying out our live application | 02:27 |
123 | Fixing the Genres component | 03:23 |
124 | Introduction | 04:03 |
125 | A bit about me | 00:53 |
126 | How to ask for help | 01:45 |
127 | Installing node.js and an IDE | 01:30 |
128 | Installing Postgres | 02:53 |
129 | Installing Go | 00:51 |
130 | How React Works | 03:36 |
131 | How to use the downloadable code | 01:09 |
132 | Our first React app | 02:34 |
133 | The obligatory "Hello, world" app | 10:53 |
134 | Working with components | 07:10 |
135 | Styling components | 05:03 |
136 | Using a CSS Framework | 04:38 |
137 | More about the CSS Framework | 03:18 |
138 | Components and props | 04:16 |
139 | More about props | 02:40 |
140 | React Events | 06:32 |
141 | More events | 04:31 |
142 | Refs | 05:19 |
143 | Simplifying things with state | 08:25 |
144 | More about state: lifting state to share data between components | 09:39 |
145 | Functional components | 06:08 |
146 | Cleaning things up | 02:09 |
147 | What are we going to create? | 02:24 |
148 | Creating our front end application and introducting the React Router | 15:14 |
149 | Routing to a component | 02:25 |
150 | Challenge: Route to components | 01:14 |
151 | Solution to Challenge | 02:53 |
152 | More about routing (and a bit about the React lifecycle) | 07:41 |
153 | More about routing Part II | 05:15 |
154 | More about routing Part III | 10:57 |
155 | Displaying one movie | 06:29 |
156 | Installing the necessary software | 02:07 |
157 | Setting up the Go project | 12:17 |
158 | Installing a router and creating better handlers | 14:14 |
159 | Models | 05:19 |
160 | Setting up a simple API route | 11:44 |
161 | Improved error handling | 03:32 |
162 | Creating the database | 01:41 |
163 | Creating our connection pool and connecting to the database | 08:01 |
164 | Database functions & a challenge | 12:13 |
165 | Solution to challenge | 08:50 |
166 | An aside: cleaning up our JSON feed | 02:33 |
167 | Getting all movies as JSON | 09:27 |
168 | Next Steps | 02:00 |
169 | Setting up CORS middleware | 03:47 |
170 | Getting the list of movies | 05:05 |
171 | Checking for errors | 05:55 |
172 | Displaying one movie | 08:11 |
173 | Getting started with Movies by Genre | 07:40 |
174 | Getting Genres from back end | 05:52 |
175 | Displaying the list of Genres | 03:19 |
176 | Getting movies by Genre | 06:11 |
177 | Displaying movies by Genre | 06:24 |
178 | Showing Genre name - an alternative to lifting state | 03:50 |
179 | Code clean up | 02:57 |
180 | Building a form in React | 11:59 |
181 | Making our form a controlled component, and binding it to state | 10:57 |
182 | Making form inputs reusable components and a Challenge | 07:33 |
183 | Solution to Challenge | 01:09 |
184 | Creating a reusable select component | 07:24 |
185 | Prepopulating the form with an existing movie | 08:09 |
186 | Sending data to the REST back end | 07:49 |
187 | Client side form validation | 07:06 |
188 | Receiving data on the REST back end | 16:49 |
189 | Providing feedback with a reusable alert | 09:10 |
190 | Editing an existing movie | 06:12 |
191 | Deleting a movie | 05:02 |
192 | Adding a confirmation step when deleting movies | 06:01 |
193 | Implementing delete on the back end | 03:31 |
194 | Connecting our delete button to the REST back end | 06:11 |
195 | Challenge: displaying list of movies to edit | 02:29 |
196 | Solution to challenge | 01:12 |
197 | Generating JSON Web Tokens on the back end | 15:34 |
198 | Changing App to a component, and setting up state | 19:25 |
199 | Getting the JSON Web Token from the back end | 06:15 |
200 | Handling a successful login | 04:01 |
201 | Adding middleware to check for a valid token | 18:14 |
202 | Protecting the route on our front end | 07:30 |
203 | Adding redirects for protected components | 02:59 |
204 | Challenge | 01:39 |
205 | Solution to Challenge | 02:27 |
206 | Saving our token when the user leaves the site | 06:28 |
207 | Making better error responses from our back end | 04:37 |
208 | Adding images | 05:07 |
209 | What is GraphQL? | 01:33 |
210 | Setting up a schema and REST endpoint for GraphQL | 15:33 |
211 | Handling the GraphQL request | 05:58 |
212 | Implementing GraphQL requests for all movies | 11:38 |
213 | Adding a search endpoint | 05:33 |
214 | Implementing GraphQL requests for search on front end | 08:58 |
215 | Displaying one movie using GraphQL | 02:57 |
216 | Updating the front end | 06:56 |
217 | Modifying the back end to handle poster images | 12:53 |
218 | Updating the front end to display the poster image | 04:56 |
219 | Cleaning things up | 02:53 |
220 | Getting the React application ready for deployment | 04:05 |
221 | Building the production ready React application | 02:45 |
222 | Getting the Go project ready for deployment | 03:04 |
223 | Building the Go back end for our remote server | 01:30 |
224 | Copying files to the server | 02:21 |
225 | Setting up the production database | 03:35 |
226 | Setting up the web server | 10:03 |
227 | Running the Go back end with supervisor | 04:53 |
228 | About this section | 08:59 |
229 | Converting the Movies.js component to a function with hooks | 10:28 |
230 | Coverting the Genres.js component to a function with hooks | 04:47 |
231 | Converting the OneMovie.js component to a function | 05:25 |
232 | Converting the OneGenre.js component to a function | 07:15 |
233 | Converting the EditMovie.js component to a function | 20:16 |
234 | Challenge: convert Admin.js to a function | 01:45 |
235 | Solution to challenge | 02:17 |
236 | Convert Login.js to a function | 10:07 |
237 | Convert App.js to a function | 07:42 |
238 | React and Redux | 01:58 |
Similar courses to Working with React and Go (Golang)
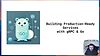
Building Production-Ready Services with gRPC and GoByteSizeGo
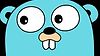
How to design & develop REST microservices in Golang (Go)udemy
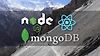
MERN Stack - React Node from Scratch Building Social Networkudemy
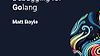
Foundations of Debugging for GolangByteSizeGoMatt Boyle
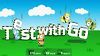
Testing with Gousegolang.com
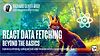
React Data Fetching: Beyond the Basicsfullstack.io

For the Love of Go: Book/Video BundleJohn Arundel
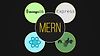
React, NodeJS, Express & MongoDB - The MERN Fullstack Guideudemy
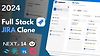