Mastering Maintainable React
"Supported by React" is a comprehensive course designed to teach you the best practices for writing clean and maintainable code in React. Whether you are a beginner or an experienced developer, this course will provide you with the tools and knowledge to write high-quality, maintainable code in React. Join me and take your React skills to the next level!
Course Highlights:
Clean Code and Refactoring
The course covers refactoring, a technique for improving the design and structure of existing code. This is an important skill for developers, allowing them to maintain and improve their code over time.
Test-Driven Development (TDD)
The course covers TDD — an approach to software development that emphasizes writing automated tests before writing code. This is a highly sought-after skill in the industry, which helps improve the quality and maintainability of the code.
Real-World Project Features
The course includes examples from real-world projects to help students understand how to apply the concepts being taught in practice and build confidence in mastering the material.
Course Benefits:
The main benefit of this course is that the discussed "code smells" (indicators of poor code) and refactorings are taken from real React projects. Since these techniques address pertinent issues, participants can immediately apply the acquired knowledge to their own projects and see the results in practice.
Practical Learning:
The "Supported by React" course focuses on practical programming. In addition to watching video demonstrations, participants will complete exercises to reinforce the material. It is important that the exercises are done as the course progresses, and participants are encouraged to apply the learned techniques in their projects to personally experience their benefits.
Join the course and learn to create clean and maintainable code in React, improving your professional skills and enhancing the quality of your projects.
Watch Online Mastering Maintainable React
# | Title | Duration |
---|---|---|
1 | Introduction to Maintainable React | 09:30 |
2 | Introduction to basic ES6 features | 02:18 |
3 | ES6 - variable declarations: var, let and const | 02:36 |
4 | ES6 - play with objects and arrays | 10:32 |
5 | Introduction to the collection API (filter, map, reduce) | 11:38 |
6 | A minimal guide to Typescript | 09:16 |
7 | React in 5 minutes | 09:15 |
8 | Introduction to code smells | 06:50 |
9 | Code smell - raw JS loop and collection APIs | 07:33 |
10 | Code smells - a real-world scenario | 05:50 |
11 | Introduction of refactorings | 09:11 |
12 | Refactoring - Extract Function | 04:59 |
13 | Refactoring - Boolean Parameter | 02:42 |
14 | Refactoring - ES6 destructuring assignment | 03:25 |
15 | Refactoring - Extract Component | 05:11 |
16 | Refactoring - Move Component | 03:22 |
17 | Demonstration of common Refactoring usages | 14:08 |
18 | Benefits of having tests | 06:08 |
19 | How to write a test? | 04:20 |
20 | Test runner + test cases + code structure | 05:35 |
21 | What is Test-Driven Development | 04:52 |
22 | The first TDD journey - A project effort tracker | 08:48 |
23 | The first TDD journey - Refactoring a bit further | 05:18 |
24 | TDD with React component - implement a simple Header Component | 10:11 |
25 | TDD with React Component - implement user interaction | 10:41 |
26 | Single Responsibility Principle | 06:24 |
27 | Composable Design | 05:59 |
28 | Using create-react-app to create your application | 05:16 |
29 | Walk through the project structure | 05:50 |
30 | Feature - Add a Todo to a list | 18:05 |
31 | Refactoring - Extract sub-components | 10:23 |
32 | Feature - Complete an item when clicked | 14:34 |
33 | Refactoring - Custom Hooks for Managing states | 12:15 |
34 | Feature - Add summary information to Todo List | 26:15 |
35 | Refactoring - Reduce duplication and extract more sub-components | 15:45 |
36 | Feature - Search by keyword | 13:44 |
37 | Feature - Enhance accessibility | 11:31 |
38 | Direct to boot - Feature introduction | 02:52 |
39 | Network-relate status statechart | 05:06 |
40 | Feature - the I'm here button - happy path | 11:38 |
41 | Introduce mirage.js | 15:50 |
42 | Feature - Error handling | 10:05 |
43 | Refactoring - extract hooks | 05:33 |
44 | Feature - I'm Here - retry | 07:21 |
45 | Use react-query to simplify the network statuses check | 12:18 |
46 | Fix all the tests with React-Query | 06:43 |
47 | Feature - Notify the store | 14:05 |
48 | Final refactoring | 11:47 |
49 | Summarise what we've covered in the course | 05:24 |
Similar courses to Mastering Maintainable React
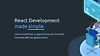
React Formula - Learn Frontend DevelopmentAlvin Zablan
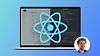
The Ultimate React Course 2024: React, Redux & Moreudemy
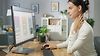
Complete DApp - Solidity & React - Blockchain Developmentudemy
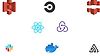
Build a React & Redux App w CircleCI CICD, AWS & Terraformudemy
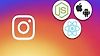
Instagram Clone Coding 3.0Nomad Coders
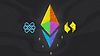
NFT Marketplace in React, Typescript & Solidity - Full Guideudemy
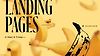
Build fancy landing pages with React and ThreejsPaul Henschel (@0xca0a)
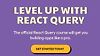
React Queryui.dev (ex. Tyler McGinnis)
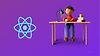
Advanced React For Enterprise: React for senior engineersudemy
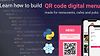