Getting Started With Golang
Learn all the key fundamentals of Go - one of the most in-demand and popular programming languages you can learn these days! Go (or Golang) is a very modern, performant and popular programming language which you can use to build applications, scripts, automations, web servers and APIs and much more!
Read more about the course
Go was originally invented and developed by a team at Google and whilst Google still heavily uses Go, it's now also very popular outside of Google. Golang regularly scores top placements in Stackoverflow surveys: In 2020, Go was the 5th most loved language according to the Stackoverflow survey.
Hence there was never a better time to get started with go and in this course, you'll learn all the key fundamentals of Go in great depth, step by step and from the ground up.
NO prior Go knowledge is assumed and you will of course learn all key concepts with both theory and practical examples, demo projects and exercises. After finishing this course, you will understand how Go works, how to write Go code and which features Go has to offer. Of course we're also going to build multiple demo projects throughout this course!
In detail, this course will teach you:
- What Go is and how it works
- The general Go syntax and rules
- Key basics like working with values & variables
- All about Go's value types, how they differ and how you use them
- How to write functions with Go
- "Special features" like multiple return values in functions
- How to organize your code into packages and modules
- How to work with third-party modules
- How to run and build Go programs
- All about controlling code flow with conditionals and loops
- More complex data types like structs
- Collection value types like arrays, slices and maps (+ how and when to use them)
- A highly understandable explanation of "Pointers"
- And so much more!
This course doesn't assume any prior Go knowledge and will turn you into a Go developer in a matter of hours!
I'd love to start this journey together with you! :)
Watch Online Getting Started With Golang
# | Title | Duration |
---|---|---|
1 | Welcome To The Course | 01:01 |
2 | What Is Go? | 06:13 |
3 | Why Would You Use Go? | 03:53 |
4 | Installing Go | 03:56 |
5 | Code Editor Setup (VS Code) | 02:41 |
6 | Finishing Editor Config & First Go Code | 06:54 |
7 | Basic Programming Knowledge Helps! | 01:56 |
8 | About This Course | 02:22 |
9 | How To Get The Most Out Of This Course | 03:51 |
10 | Module Introduction | 01:27 |
11 | Base Syntax & Language Features | 09:09 |
12 | Getting Started With Values & Value Types | 05:26 |
13 | Exploring Variables | 09:35 |
14 | The "int" Type & Math Operations | 08:21 |
15 | Time To Practice: Problem | 05:39 |
16 | Time To Practice: Solution | 10:25 |
17 | Working With Floats & Type Conversions | 07:48 |
18 | float64 vs float32 | 03:31 |
19 | A Brief Look At "bool", "rune" and "byte" Types | 07:11 |
20 | String Operations & Type Clashes | 06:27 |
21 | Formatting Strings | 11:06 |
22 | Time To Practice: Problem | 02:42 |
23 | Time To Practice: Solution | 07:37 |
24 | Go Packages & Modules: The Theory | 05:34 |
25 | Creating & Using A First Module | 05:00 |
26 | Working With Packages & Exports + Imports | 09:02 |
27 | Using Constant Values (Constants) | 03:55 |
28 | Module Summary | 02:53 |
29 | Module Introduction | 01:50 |
30 | Creating Our Go Module | 04:24 |
31 | Printing Output | 03:41 |
32 | Fetching User Input | 08:20 |
33 | Cleaning & Parsing User Input | 10:16 |
34 | BMI Calculation & Outputting Formatted Strings | 03:41 |
35 | Using Constant Values | 04:21 |
36 | Splitting Our Code Into Files & Packages | 07:39 |
37 | Module Introduction | 00:50 |
38 | What Is A "Function"? | 03:50 |
39 | Creating A Function & Working With Parameters + Return Values | 10:11 |
40 | Practicing Functions | 03:44 |
41 | Go Special Feature: Multiple Return Values | 07:57 |
42 | Using Named Return Values | 03:40 |
43 | Module Summary | 01:39 |
44 | Module Introduction | 02:53 |
45 | Creating a First Function | 03:45 |
46 | Outsourcing The User Input Logic | 05:22 |
47 | Finishing the "main" Function Refactoring | 05:23 |
48 | Avoiding Code Duplication | 06:25 |
49 | Module Summary | 01:12 |
50 | Module Introduction | 00:47 |
51 | What Are Pointers & Why Do We Have Them? | 08:08 |
52 | Creating a First Pointer | 05:07 |
53 | Working With Pointers | 05:36 |
54 | Advantages Of Pointers (& Disadvantages) | 08:48 |
55 | Module Introduction | 00:55 |
56 | What & Why + Defining a First Struct | 09:26 |
57 | Creating Struct Instances | 06:22 |
58 | Creating Structs With "Creation Functions" | 03:40 |
59 | Structs & Pointers | 04:32 |
60 | Accessing Struct Values | 08:43 |
61 | Adding Methods To Structs | 07:20 |
62 | Module Summary | 04:18 |
63 | Module Introduction | 03:07 |
64 | Defining a Struct | 03:30 |
65 | Creating Struct Instances | 09:45 |
66 | Adding a Method | 05:03 |
67 | Reading User Input | 14:07 |
68 | Writing To Files | 07:54 |
69 | Module Introduction | 01:09 |
70 | Introducing Arrays For Storing Lists Of Data | 08:03 |
71 | Working With Arrays | 06:19 |
72 | Selecting Parts Of Arrays With Slices | 03:27 |
73 | More Ways Of Using Slices | 02:24 |
74 | Slices - Deep Dive | 09:44 |
75 | Creating Dynamic Lists With Slices | 09:17 |
76 | Time To Practice - Problem | 04:18 |
77 | Time To Practice - Solution | 20:11 |
78 | Unpacking List Values | 03:30 |
79 | Introducing Maps | 06:49 |
80 | Mutating Maps | 03:42 |
81 | Maps vs Structs | 04:01 |
82 | Module Introduction | 01:15 |
83 | Working on the Project Setup | 05:35 |
84 | Introducing "if" Statements | 03:47 |
85 | More about "if" Statements & Boolean Values (Booleans) | 04:41 |
86 | "else" & "else if" | 05:09 |
87 | Combining Conditions | 04:29 |
88 | Handling Expected Errors | 08:11 |
89 | Returning Errors In Functions | 03:34 |
90 | Practicing What We Learned | 13:51 |
91 | Onwards To "Loops" (and why do we need them?) | 05:45 |
92 | Introducing a Basic "for" Loop | 05:12 |
93 | A More Useful Loop | 02:56 |
94 | Practicing Basic "for" Loops | 04:23 |
95 | Go's "while" Loop | 09:44 |
96 | Preparing Another Scenario | 05:30 |
97 | Looping Through Collections (Arrays, Slices, Maps) | 05:23 |
98 | "continue" and "break" | 02:34 |
99 | Module Summary | 02:48 |
100 | Module Introduction | 01:47 |
101 | Planning the App | 05:21 |
102 | Initializing The Project | 02:13 |
103 | Adding The Core Game Steps & Logic | 05:25 |
104 | Outputting Text & Adding a First Package | 04:06 |
105 | Keeping Track Of The Active Round | 05:00 |
106 | Displaying Available Player Actions | 03:39 |
107 | Getting Started With Fetching User Input | 06:55 |
108 | Validating & Using User Input | 11:06 |
109 | Generating (True) Random Numbers | 07:18 |
110 | Adding Logic For Different Actions | 07:03 |
111 | Utilizing Constants | 05:46 |
112 | Checking For A Winner | 11:13 |
113 | Adding the "End Game" Logic | 03:51 |
114 | Outputting Round Data (with a Struct) | 18:06 |
115 | Managing Multiple Rounds (with a Slice) | 05:46 |
116 | Writing To A Log File | 10:12 |
117 | Module Summary | 01:13 |
118 | Module Introduction | 01:18 |
119 | Using Third-Party Modules | 10:47 |
120 | Building Go Projects (Standalone Executables) | 03:51 |
121 | A Gotcha: Executables & File Paths | 07:39 |
122 | Module Introduction | 01:31 |
123 | Variables, Scope & Variable Shadowing | 07:37 |
124 | "make"ing Values | 10:27 |
125 | The "new" Function | 07:02 |
126 | new vs make | 01:22 |
127 | Working With Custom Types | 10:36 |
128 | More On Constants | 07:56 |
129 | Module Summary | 03:19 |
130 | Module Introduction | 01:00 |
131 | Using Functions As Values & Function Types | 15:11 |
132 | Returning Functions In Functions | 06:22 |
133 | Introducing Anonymous Functions | 06:35 |
134 | Working with Closures | 06:43 |
135 | Using Recursion | 11:21 |
136 | Introducing Variadic Functions | 06:48 |
137 | Splitting Slices Into Parameter Lists | 03:06 |
138 | Deferring Function Execution with "defer" | 09:05 |
139 | Panic! | 04:12 |
140 | Module Summary | 02:43 |
141 | Module Introduction | 00:56 |
142 | What's the Problem? | 06:32 |
143 | Interfaces As a Solution (i.e. What are Interfaces?) | 06:18 |
144 | Example Time: Built-in Interfaces We Already Used | 04:55 |
145 | Interfaces: Rules & Conventions | 05:07 |
146 | Empty Interfaces | 04:37 |
147 | Flexible Code With Empty Interfaces & Type Switches | 11:04 |
148 | Module Summary | 01:31 |
149 | Module Introduction | 00:45 |
150 | Embedding Interfaces | 08:16 |
151 | Embedding Structs | 05:59 |
152 | Module Introduction | 00:54 |
153 | What Is Concurrency? | 01:44 |
154 | Introducing Goroutines | 07:46 |
155 | Working With Channels | 09:00 |
156 | Reading From A Channel Multiple Times | 02:00 |
157 | Transmitting Values Via Channels | 08:26 |
158 | Looping Through Channel Values | 05:27 |
159 | Using Buffered Channels | 07:05 |
160 | Using The "select" Statement | 04:10 |
161 | Module Summary | 02:44 |
Similar courses to Getting Started With Golang
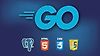
Building Modern Web Applications with Go (Golang)udemy
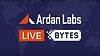
Ardan Labs Live Bytes (Ultimate Go Syntax LIVE)ardanlabs.com
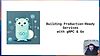
Building Production-Ready Services with gRPC and GoByteSizeGo
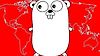
Web Development with Google’s Go (golang) Programming Languagegreatercommons.com
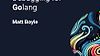
Foundations of Debugging for GolangByteSizeGoMatt Boyle
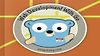
Learn to Create Web Applications using Gousegolang.com
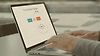
Advanced Branching and Looping in GOpluralsight
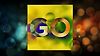