Data Structures & Algorithms !
28h 40m 46s
English
Paid
Learn data structures and algorithms from scratch. Start with basic data structures and work your way up to intermediate. This course is for all those who want to learn data structure and algorithm from an absolute basic to an intermediate level. We don't expect you to have any prior knowledge of data structures or algorithms, but a basic prior knowledge of Java will be helpful.
Watch Online Data Structures & Algorithms !
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | S01 - L01 -- Course Breakdown | 07:09 |
2 | S01 - L02 -- What is DS and Algo | 04:37 |
3 | S01 - L03 -- Why companies ask DS | 03:28 |
4 | S01 - L04 -- DS in every day life | 03:30 |
5 | S01 - L05 -- Types of DS | 03:42 |
6 | S01.1-L01--What is Recursion | 11:59 |
7 | S01.1-L02--Why learn Recursion | 05:07 |
8 | S01.1-L03--Format of Recursion Method | 04:09 |
9 | S01.1-L04--How Recurssion works internally | 14:41 |
10 | S01.1-L05--Finding Factorial using Recursion | 08:22 |
11 | S01.1-L06--Finding Fibonacci using Recursion | 07:42 |
12 | S01.1-L07--Recursion vs Iteration | 03:19 |
13 | S01.1-L08--When to Use & Avoid Recursion | 04:43 |
14 | S01.1-L09--Practical Uses of Recursion | 03:16 |
15 | S02-L01 -- What is Algo Run Time Analysis | 03:14 |
16 | S02-L02 -- What are Notations | 10:39 |
17 | S02-L03 -- Examples of Notations | 07:55 |
18 | S02-L04 -- Examples of Time Complexity | 06:52 |
19 | S02-L05 -- Finding Time Complexity of Iterative Algo | 08:14 |
20 | S02-L06 -- Finding Time Complexity of Recursive Algo#1 | 11:10 |
21 | S02-L07 -- Finding Time Complexity of Recursive Algo#2 | 17:48 |
22 | S03 - L01 -- What and Why of Array | 07:02 |
23 | S03 - L02 -- Types of Array | 07:18 |
24 | S03 - L03 -- How is Array represented in Memory | 06:01 |
25 | S03 - L04 -- Create an Array | 11:26 |
26 | S03 - L05 -- Insert Traverse in 1D Array | 07:05 |
27 | S03 - L06 -- Access Search Delete in 1D Array | 16:27 |
28 | S03 - L07 -- Code 1D Array | 13:45 |
29 | S03 - L08 -- Time Complexity of 1D Array | 04:02 |
30 | S03 - L09 -- Create 2D Array | 09:14 |
31 | S03 - L10 -- 2D Array operations | 14:49 |
32 | S03 - L11 -- Time Complexity of 2D Array | 02:56 |
33 | S03 - L12 -- When to use Array | 06:17 |
34 | S03 - L13 -- Code 2D Array | 14:33 |
35 | S03 - L14 -- Practical uses of Array | 07:31 |
36 | S04 - L01 -- What is linked list | 08:44 |
37 | S04 - L02 -- Linked list vs Array | 03:19 |
38 | S04 - L03 -- Components of LinkedList | 06:30 |
39 | S04 - L04 -- Types of LinkedList | 06:55 |
40 | S04 - L05 -- Why so many types of LinkedList | 07:57 |
41 | S04 - L06 -- How is LinkedList stored in Memory | 05:06 |
42 | S04 - L07 -- Creation of Single LinkedList (SLL) | 05:57 |
43 | S04 - L08 -- Insertion in SLL - Dry Run | 07:24 |
44 | S04 - L09 -- Insertion in SLL - Algo | 10:57 |
45 | S04 - L10 -- Traversal of SLL | 03:50 |
46 | S04 - L11 -- Search node in SLL | 04:53 |
47 | S04 - L12 -- Deletion of node from SLL - Dry Run | 09:14 |
48 | S04 - L13 -- Deletion of node from SLL - Algo | 08:48 |
49 | S04 - L14 -- Delete entire SLL | 03:15 |
50 | S04 - L15 -- Time Complexity of SLL | 03:30 |
51 | S04 - L16 -- Creation of Circular Single LinkedList (CSLL) | 04:07 |
52 | S04 - L17 -- Insertion of data in CSLL - Dry Run | 06:16 |
53 | S04 - L18 -- Insertion of data in CSLL - Algo | 07:45 |
54 | S04 - L19 -- Traverse CSLL | 02:31 |
55 | S04 - L20 -- Search a node in CSLL | 04:54 |
56 | S04 - L21 -- Delete a node from CSLL - Dry Run | 05:35 |
57 | S04 - L22 -- Deletion of node from CSLL - Algo | 07:05 |
58 | S04 - L23 -- Deletion of entire CSLL | 04:02 |
59 | S04 - L24 -- Time Complexity of CSLL | 04:17 |
60 | S04 - L25 -- Create Double Linked List (DLL) | 05:59 |
61 | S04 - L26 -- Insert node in DLL - Dry Run | 08:00 |
62 | S04 - L27 -- Insert node in DLL - Algo | 13:35 |
63 | S04 - L28 -- Traverse DLL | 02:32 |
64 | S04 - L29 -- Reverse Traversal of DLL | 03:21 |
65 | S04 - L30 -- Search a node in DLL | 03:13 |
66 | S04 - L31 -- Delete a node from DLL - Dry Run | 07:21 |
67 | S04 - L32 -- Delete a node from DLL - Algo | 09:40 |
68 | S04 - L33 -- Delete entire DLL | 04:47 |
69 | S04 - L34 -- Time Complexity of DLL | 04:13 |
70 | S04 - L35 -- Create Double Circular LinkedList (CDLL) | 07:37 |
71 | S04 - L36 -- Insert node in CDLL - Dry Run | 11:06 |
72 | S04 - L37 -- Insert node in CDLL - Algo | 09:46 |
73 | S04 - L38 -- Traverse CDLL | 03:36 |
74 | S04 - L39 -- Reverse traverse CDLL | 03:28 |
75 | S04 - L40 -- Search a node in CDLL | 04:31 |
76 | S04 - L41 -- Delete a node from CDLL - Dry Run | 08:45 |
77 | S04 - L42 -- Delete a node from CDLL - Algo | 10:38 |
78 | S04 - L43 -- Delete entire CDLL | 06:29 |
79 | S04 - L44 -- Time Complexity of CDLL | 04:27 |
80 | S04 - L45 -- SLL vs CSLL vs DLL vs DLL | 06:43 |
81 | S04 - L46 -- Practical uses of LinkedList | 05:10 |
82 | L01 -- What and Why of Stack | 04:55 |
83 | L02 -- ARRAY - Create & Push | 06:19 |
84 | L03 -- ARRAY - Pop, Peek, isEmpty, isFull, Delete | 07:52 |
85 | L04 -- LinkedList - Create, Push, Pop | 06:36 |
86 | L05 -- LinkedList - Peek, Delete | 05:06 |
87 | L06 -- When to use or avoid Stack | 03:36 |
88 | S06 - L01 -- What and Why of Queue | 03:53 |
89 | S06 - L02 -- LINEAR QUEUE(Array) - Create, Enqueue | 06:59 |
90 | S06 - L03 -- LINEAR QUEUE((Array)) - deQueue, isEmpty, isFull, Delete | 07:23 |
91 | S06 - L04 -- Why Circular Queue | 04:45 |
92 | S06 - L05 -- CIRCULAR QUEUE(Array) - Enqueue | 05:57 |
93 | S06 - L06 -- CIRCULAR QUEUE(Array) - Dequeue | 03:19 |
94 | S06 - L07 -- CIRCULAR QUEUE(Array) - Peek, isEmpty, isFull, Delete | 05:22 |
95 | S06 - L08 -- LINEAR QUEUE(LL) - Enqueue | 05:31 |
96 | S06 - L09 -- LINEAR QUEUE(LL) - DeQueue | 03:11 |
97 | S06 - L10 -- LINEAR QUEUE(LL) - Peek, isEmpty, Delete | 04:04 |
98 | S06 - L11 -- Array vs LinkedList Implementation | 04:29 |
99 | S08.01 - L01 -- What is Tree | 09:30 |
100 | S08.01 - L02 -- Why learn Tree | 02:08 |
101 | S08.01 - L03 -- Tree Terminologies - Part#1 | 10:01 |
102 | S08.01 - L03 -- Tree Terminologies - Part#2 | 04:29 |
103 | S08.01 - L04 -- What & Why of Binary Ttree | 04:15 |
104 | S08.01 - L05 -- Types of Binary Tree | 05:29 |
105 | S08.01 - L06 -- How is Tree Represented in Code | 08:54 |
106 | S08.01 - L07 -- Create blank Binary Tree(LL) | 03:17 |
107 | S08.01 - L08 -- Pre-order traversal Binary Tree(LL) | 14:45 |
108 | S08.01 - L09 -- In-order traversal Binary Tree(LL) | 06:18 |
109 | S08.01 - L10 -- Post-order traversal Binary Tree(LL) | 06:33 |
110 | S08.01 - L11 -- Level-order traversal Binary Tree(LL) | 07:26 |
111 | S08.01 - L12 -- Search for value in Binary Tree(LL) | 08:10 |
112 | S08.01 - L13 -- Insert value in Binary Tree(LL) | 05:54 |
113 | S08.01 - L14 -- Delete value from Binary Tree(LL) | 06:55 |
114 | S08.01 - L15 -- Delete Binary Tree(LL) | 03:59 |
115 | S08.01 - L16 -- Create Binary Tree(Array) | 05:14 |
116 | S08.01 - L17 -- Insert value in Binary Tree(Array) | 05:55 |
117 | S08.01 - L18 -- Search for value in Binary Tree(Array) | 03:39 |
118 | S08.01 - L19 -- Inorder traversal of Binary Tree(Array) | 06:18 |
119 | S08.01 - L20 -- Pre-order traversal of Binary Tree(Array) | 03:48 |
120 | S08.01 - L21 -- Post-order traversal of Binary Tree(Array) | 04:18 |
121 | S08.01 - L22 -- Level-order traversal of Binary Tree(Array) | 02:46 |
122 | S08.01 - L23 -- Delete node from Binary Tree(Array) | 05:34 |
123 | S08.01 - L24 -- Delete Binary Tree(Array) | 03:26 |
124 | S08.01 - L25 -- Binary Tree (Array vs Linked List) | 04:44 |
125 | S08.02 - L01 -- What & Why of BST | 04:45 |
126 | S08.02 - L02 -- Creation & Searching in BST | 10:00 |
127 | S08.02 - L03 -- Traversing a BST | 07:37 |
128 | S08.02 - L04 -- Inserting a node in BST | 13:58 |
129 | S08.02 - L05 -- Deleting a node from BST | 15:55 |
130 | S08.02 - L06 -- Deleting a BST | 03:43 |
131 | S08.03 - L01 -- Why learn AVL Tree | 06:49 |
132 | S08.03 - L02 -- What is AVL Tree | 10:21 |
133 | S08.03 - L03 -- Create Search Traverse AVL Tree | 06:28 |
134 | S08.03 - L04 -- Insert in AVL_LL Theory | 12:02 |
135 | S08.03 - L05 -- Insert in AVL_LL Algorithm | 04:48 |
136 | S08.03 - L06 -- Insert in AVL LR | 08:13 |
137 | S08.03 - L07 -- Insert in AVL RR | 08:24 |
138 | S08.03 - L08 -- Insert in AVL RL | 06:23 |
139 | S08.03 - L09 -- Insert End to End Case | 14:20 |
140 | S08.03 - L10 -- Delete LL LR RR RL | 11:27 |
141 | S08.03 - L11 -- Delete End to End Case | 11:57 |
142 | S08.03 - L12 -- Delete AVL Tree & Tree Comparison | 07:37 |
143 | S08.04 - L01 -- What Why and Type of Heap | 12:27 |
144 | S08.04 - L02 -- Create, Peek, Size of Heap | 04:45 |
145 | S08.04 - L03 -- Insert element in Heap | 05:12 |
146 | S08.04 - L04 -- Extract and Delete from Heap | 06:04 |
147 | S08.04 - L05 -- Why avoid Reference based implementation ? | 03:50 |
148 | S08.05 - L01 -- What and Why of Trie | 05:57 |
149 | S08.05 - L02 -- Create Insert in Trie | 06:53 |
150 | S08.05 - L03 -- Search a String in Trie | 02:36 |
151 | S08.05 - L04 -- Delete a String from Trie | 07:38 |
152 | S08.05 - L05 -- Practical Uses of Trie | 02:33 |
153 | S09 - L01 -- Introduction to Hashing | 03:39 |
154 | S09 - L02 -- Why learn Hashing ? | 05:13 |
155 | S09 - L03 -- Introduction to Hash Functions | 08:53 |
156 | S09 - L04 -- Types of Collision Resolution Techniques | 14:55 |
157 | S09 - L05 -- What happens when Hash Table is full ? | 04:31 |
158 | S09 - L06 -- Collision Resolution Techniques Compared | 08:27 |
159 | S09 - L07 -- Practical Use of Hashing | 06:41 |
160 | S09 - L08 -- Hashing vs Other DS | 04:37 |
161 | S10 - L01 -- What and Why of Sorting | 03:55 |
162 | S10 - L02 -- Types of Sorting Techniques | 07:15 |
163 | S10 - L03 -- Sorting Terminologies | 05:02 |
164 | S10 - L04 -- Bubble Sort | 07:47 |
165 | S10 - L05 -- Selection Sort | 06:08 |
166 | S10 - L06 -- Insertion Sort | 08:52 |
167 | S10 - L07 -- Bucket Sort | 08:35 |
168 | S10 - L08 -- Merge Sort | 11:12 |
169 | S10 - L09 -- Quick Sort Part#1 | 12:37 |
170 | S10 - L09 -- Quick Sort Part#2 | 06:01 |
171 | S10 - L10 -- HeapSort | 12:42 |
172 | S10 - L11 -- Sorting Techniques compared | 02:15 |
173 | S11 - L01 -- What and Why of Graphs | 08:57 |
174 | S11 - L02 -- Graph Terminologies | 05:12 |
175 | S11 - L03 -- Types of Graphs | 04:00 |
176 | S11 - L04 -- Graph Representation in Code | 07:09 |
177 | S11 - L05 -- BFS Algorithm | 08:30 |
178 | S11 - L06 -- BFS Time Complexity | 06:34 |
179 | S11 - L07 -- DFS Algorithm | 08:08 |
180 | S11 - L08 -- DFS Time Complexity | 05:59 |
181 | S11 - L09 -- BFS vs DFS | 04:27 |
182 | S11 - L10 -- What is Topological Sort | 03:45 |
183 | S11 - L11 -- Topological Sort Dry Run | 06:33 |
184 | S11 - L12 -- Why Topological Sort Works | 07:12 |
185 | S11 - L13 -- Topological Sort Algorithm | 06:39 |
186 | S11 - L14 -- What is Single Source Shortest Path Problem(SSSP) | 06:30 |
187 | S11 - L15 -- BFS for SSSP | 08:08 |
188 | S11 - L16 -- Why BFS does not works for Weighted Graph SSSP | 04:04 |
189 | S11 - L17 -- Why DFS does not works for SSSP | 03:02 |
190 | S11 - L18 -- Dijkstra for SSSP | 11:17 |
191 | S11 - L19 -- Why Dijkstra does not work for Negative Cycle | 06:07 |
192 | S11 - L20 -- BellmanFord Dry run for SSSP | 09:48 |
193 | S11 - L21 -- BellanFord Algorithm for SSSP | 04:28 |
194 | S11 - L22 -- How Bellman Ford works for Negative Cycle | 09:09 |
195 | S11 - L23 -- Why BellmanFord runs for V-1 times | 10:09 |
196 | S11 - L24 -- BFS vs Dijkstra vs BellmanFord | 04:33 |
197 | S11 - L25 -- What is All Pair Shortest Path Problem(APSP) | 05:35 |
198 | S11 - L26 -- Dry run of BFS Dijkstra Bellman for APSP | 05:18 |
199 | S11 - L27 -- Floyd Warshall Algorithm for APSP | 10:09 |
200 | S11 - L28 -- Why Floyd Warshall Algorithm always works | 08:13 |
201 | S11 - L29 -- Why Floyd does not works for Negative Cycle | 03:50 |
202 | S11 - L30 -- BFS vs Dijkstra vs Bellman vs Floys | 04:25 |
203 | S11 - L31 -- What is Minimum Spanning Tree (MST) | 04:39 |
204 | S11 - L32.1 -- DisjointSet | 08:49 |
205 | S11 - L32.2 -- Kruskals Algorithm | 12:40 |
206 | S11 - L33 -- Prims Algorithm Dry Run | 06:34 |
207 | S11 - L34 -- Prims Algorithm Explained | 05:23 |
208 | S11 - L35 -- Prims vs Kruskal | 02:34 |
209 | S12.1 - L01 -- Magic Framework | 04:46 |
210 | S12.2 - L01 -- Greedy Algo Introduction | 06:18 |
211 | S12.2 - L02 -- Known Algos | 10:51 |
212 | S12.2 - L03 -- Activity Selection Problem | 08:05 |
213 | S12.2 - L04 -- Coin Change Problem | 09:07 |
214 | S12.2 - L05 -- Fractional Knapsack Problem | 11:56 |
215 | S12.3 - L01 -- What and Why of Divide&Conquer | 07:35 |
216 | S12.3 - L02 -- Binary Search, Quick, Merge Sortt | 05:48 |
217 | S12.3 - L03 -- Fibonacci Series | 03:32 |
218 | S12.3 - L04 -- Number Factor | 09:38 |
219 | S12.3 - L05 -- House thief | 08:49 |
220 | S12.3 - L06 -- Convert One String to Another | 12:58 |
221 | S12.3 - L07 -- Zero-One Knapsack | 07:49 |
222 | S12.3 - L08 -- Longest Common Subsequence | 08:52 |
223 | S12.3 - L09 -- Longest Palindromic Subsequence | 09:47 |
224 | S12.3 - L10 -- Longest Palindromic Substring | 09:11 |
225 | S12.3 - L11 -- Min Cost to Reach End of Array | 07:13 |
226 | S12.3 - L12 -- Number of Paths to reach last cell with given Cost | 10:30 |
227 | S12.4 - L01 -- What and Why of Dynamic Programming | 04:46 |
228 | S12.4 - L02 -- Top Down Approach | 06:41 |
229 | S12.4 - L03 -- Bottom Up Approach | 07:45 |
230 | S12.4 - L04 -- Is Merge Sort Dynamic Programming ? | 03:57 |
231 | S12.4 - L05 -- Number Factor Problem | 15:26 |
232 | S12.4 - L06 -- HouseThief Problem | 12:15 |
233 | S12.4 - L07 -- Convert One String to Another | 11:20 |
234 | S12.4 - L08 -- Zero One Knapsack Problem | 13:16 |
235 | S12.4 - L09 -- Longest Common Subsequence | 10:20 |
236 | S12.4 - L10 -- Longest Palindromic Subsequence | 10:53 |
237 | S12.4 - L11 -- Longest Palindromic Substring | 12:34 |
238 | S12.4 - L12 -- Min Cost to Reach End of Array | 12:35 |
239 | S12.4 - L13 -- Ways to Reach last cell | 24:53 |
Similar courses to Data Structures & Algorithms !
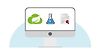
Spring Professional Certification Exam Tutorial - Module 08udemy
Category: Java, Spring
Duration 1 hour 54 minutes 51 seconds
Course
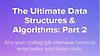
The Ultimate Data Structures & Algorithms: Part 2codewithmosh (Mosh Hamedani)
Category: Others, Java
Duration 5 hours 56 minutes 46 seconds
Course
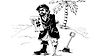
Data Structures in Javajavaspecialists.eu
Category: Java
Duration 8 hours 3 minutes 54 seconds
Course
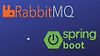
RabbitMQ : Messaging with Java, Spring Boot And Spring MVCudemy
Category: Spring Boot, Java, Spring Cloud, Spring MVC
Duration 4 hours 3 minutes 11 seconds
Course
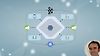
Microservices: Clean Architecture, DDD, SAGA, Outbox & Kafkaudemy
Category: Spring Boot, Others, Java
Duration 18 hours 2 minutes 34 seconds
Course
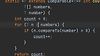
Java Genericsamigoscode (Nelson Djalo)
Category: Java
Duration 1 hour 8 minutes 39 seconds
Course
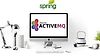
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
Category: Spring Boot, Java
Duration 1 hour 47 minutes 44 seconds
Course
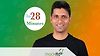
Learn Java Unit Testing with Junit & Mockito in 30 Stepsudemy
Category: Java
Duration 4 hours 44 minutes 35 seconds
Course
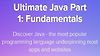
Ultimate Java Part 1: Fundamentalscodewithmosh (Mosh Hamedani)
Category: Java
Duration 3 hours 21 minutes 58 seconds
Course
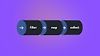
Java Streams APIamigoscode (Nelson Djalo)
Category: Java
Duration 2 hours 33 minutes 12 seconds
Course