Spring Framework 6 Beginner to Guru
This course is All Things Spring! Do you wish to master Spring Framework 6 and Spring Boot 3? Then this is the course for you. This course is for developers with no previous Spring Framework or Spring Boot experience. This course has been developed by a back end developer, for back end developers!
More
Inside this course, you will learn about:
Build a Spring Boot Web App
Use Spring for Dependency Injection
Create RESTful Web Services with Spring MVC
Create RESTful Web Services with Spring Webflux
Create RESTful Web Services with Spring Webflux.fn
Learn Best Practices using Project Lombok with Spring
Create MapStruct Mappers as Spring Components
Spring MockMVC with Mockito and JUnit 5
Spring Data JPA
Spring Data MongoDB
Spring Data R2DBC (Reactive)
Spring RestTemplate
Spring WebClient
Spring WebTestClient
Spring Security HTTP Basic Authentication
Spring Security OAuth2 Authentication w/ JWT
Spring Authorization Server
Spring WebMVC OAuth2 Resource Server
Spring WebFlux OAuth2 Resource Server
Spring Cloud Gateway
Spring Boot Maven Plugin
Spring Boot Gradle Plugin
Use Java Bean Validation with Spring
Spring Boot Auto-Configuration with MySQL
Use Spring Boot and Flyway for Database Migrations
Hibernate Database Relationship Mapping with Spring Data JPA
Watch Online Spring Framework 6 Beginner to Guru
# | Title | Duration |
---|---|---|
1 | Course Introduction | 01:23 |
2 | Instructor Introduction | 02:18 |
3 | Getting the Most out of this Course | 05:38 |
4 | Setting Up Your Development Environment | 03:45 |
5 | Is Your IDE Free Like a Puppy? | 02:46 |
6 | Getting Help with the Spring Framework | 07:32 |
7 | Using GitHub | 12:21 |
8 | Introduction | 01:17 |
9 | Introduction to Spring | 07:51 |
10 | Application Overview | 04:48 |
11 | Spring Initilizer | 05:38 |
12 | Open Project in IntelliJ | 08:44 |
13 | Using JPA Entities | 04:33 |
14 | JPA Relationships | 04:15 |
15 | Code Examples in GitHub | 03:58 |
16 | Equality in Hibernate | 03:33 |
17 | Spring Data Repositories | 03:57 |
18 | Initializing Data with Spring | 11:08 |
19 | Publisher Relationships | 04:28 |
20 | Introduction to H2 Database Console | 06:02 |
21 | Introduction to Spring MVC | 05:43 |
22 | Create Service Layer | 03:42 |
23 | Configuring Spring Controllers | 03:56 |
24 | Thymeleaf Templates | 08:19 |
25 | Project Review | 02:58 |
26 | Introduction | 01:08 |
27 | SOLID Principles of OOP | 06:37 |
28 | The Spring Context | 04:12 |
29 | Spring Test Context | 04:12 |
30 | Basics of Dependency Injection | 08:14 |
31 | Dependencies Without Injection | 03:42 |
32 | Dependency Injection without Spring | 06:12 |
33 | Dependency Injection using Spring Framework | 08:30 |
34 | Primary Beans | 03:03 |
35 | Using Qualifiers | 08:03 |
36 | Spring Profiles | 06:11 |
37 | Default Profile | 03:25 |
38 | Spring Bean Life Cycle | 05:26 |
39 | Spring Bean Life Cycle Demo | 08:42 |
40 | Introduction | 02:30 |
41 | HTTP Protocol | 07:18 |
42 | HTTP Request Methods | 05:34 |
43 | Postman Demonstration | 05:09 |
44 | Beginners Guide to REST | 06:04 |
45 | Richardson Maturity Model | 04:05 |
46 | Spring Framework and RESTFul Services | 07:11 |
47 | Introduction | 01:39 |
48 | Create New Project | 04:09 |
49 | Project Lombok Features | 07:20 |
50 | Project Lombok POJOs | 04:14 |
51 | Project Lombok Builders | 03:51 |
52 | Project Lombok Constructors | 02:30 |
53 | Project Lombok Logging | 05:07 |
54 | Delombok | 02:08 |
55 | Introduction | 01:31 |
56 | Introducing SFG Beer Works | 01:24 |
57 | HTTP GET with Spring MVC List Operation | 04:41 |
58 | HTTP Client | 02:57 |
59 | Using Path Parameters - Get By Id | 06:19 |
60 | Spring Boot Development Tools | 04:07 |
61 | HTTP POST with Spring MVC | 08:23 |
62 | Set Header on HTTP Response | 04:33 |
63 | HTTP PUT with Spring MVC | 06:33 |
64 | HTTP DELETE with Spring MVC | 04:08 |
65 | HTTP PATCH with Spring MVC | 05:25 |
66 | Introduction | 02:25 |
67 | Introduction to Testing with MockMVC | 09:21 |
68 | MockMVC Configuration | 06:49 |
69 | Return Data With Mockito | 05:15 |
70 | Using JSON Matchers | 05:50 |
71 | MockMVC Test List Beers | 03:13 |
72 | Create JSON Using Jackson | 04:59 |
73 | MockMVC Test Create Beer | 05:46 |
74 | MockMVC Test Update Beer | 04:03 |
75 | MockMVC Test Delete Beer | 03:56 |
76 | MockMVC Test Patch Beer | 04:53 |
77 | DRY - Don't Repeat Yourself | 02:48 |
78 | DRY - Refactoring | 03:57 |
79 | URI Builder | 03:01 |
80 | Introduction | 01:31 |
81 | Exception Handling Overview | 06:34 |
82 | Throw Custom Exception with Mockito | 02:49 |
83 | Using Exception Handler | 02:44 |
84 | Controller Advice | 02:42 |
85 | Using ResponseStatus Annotation | 02:42 |
86 | Using Java Optional | 04:00 |
87 | Introduction | 02:52 |
88 | Data Transfer Objects | 04:27 |
89 | Refactoring to DTOs | 02:32 |
90 | Spring Data JPA Dependencies | 03:23 |
91 | Creating JPA Entities | 03:38 |
92 | Hibernate UUID Id Generation | 03:26 |
93 | Spring Data JPA Repositories | 02:42 |
94 | Spring Boot JPA Test Splice | 04:31 |
95 | MapStruct Dependencies and Configuration | 04:36 |
96 | MapStruct Mappers | 03:28 |
97 | JPA Services | 03:08 |
98 | JPA Get Operations | 03:05 |
99 | Controller Integration Test | 06:11 |
100 | Testing for expected Exceptions | 03:36 |
101 | JPA Save New Beer Operation | 06:44 |
102 | JPA Update Beer by Id Operation | 04:27 |
103 | JPA Update Beer Not Found | 06:34 |
104 | JPA Delete Beer by Id | 03:35 |
105 | JPA Delete by Id Not Found | 05:02 |
106 | Introduction | 02:24 |
107 | Data Validation Overview | 07:55 |
108 | Java Bean Validation Maven Dependencies | 01:49 |
109 | Controller Binding Validation | 03:11 |
110 | Custom Validation Handler | 04:11 |
111 | Custom Error Body | 03:52 |
112 | JPA Validation | 03:30 |
113 | Database Constraint Validation | 04:08 |
114 | Controller Testing with JPA | 03:41 |
115 | JPA Validation Error Handler | 02:01 |
116 | JPA Validation Error Message | 05:01 |
117 | Introduction | 02:30 |
118 | Overview of MySQL | 07:49 |
119 | Create MySQL Schema and User Accounts | 05:49 |
120 | Adding MySQL Dependencies | 03:15 |
121 | Spring Boot MySQL Profile | 05:03 |
122 | Console Logging of SQL Statements | 04:40 |
123 | JPA Updates for MySQL | 05:25 |
124 | Hikari Datasource Pool | 05:24 |
125 | Schema Script Generation | 04:33 |
126 | Spring Boot Database Initialization | 03:21 |
127 | Introduction | 02:34 |
128 | Overview of Flyway | 05:44 |
129 | Flyway Dependencies | 02:14 |
130 | Flyway Migration Script Configuration | 08:40 |
131 | Add Database Column | 05:08 |
132 | Flyway Advanced Spring Boot Configuration | 03:04 |
133 | Fixing Integration Tests using H2 | 03:16 |
134 | Introduction | 01:55 |
135 | Beer CSV Data | 02:58 |
136 | Beer CSV POJO | 03:13 |
137 | Mapping with OpenCSV | 06:02 |
138 | CSV Parse Service | 05:32 |
139 | Save CSV Data to Database | 08:27 |
140 | Hibernate Create and Update Timestamp | 03:13 |
141 | Fix Integration Tests | 02:16 |
142 | Introduction | 01:33 |
143 | Overview of Query Parameters | 03:23 |
144 | List Beers Spring MVC Test | 03:14 |
145 | Capture Query Parameters with Spring MVC | 04:57 |
146 | Update Service to Accept Query Parameter | 03:39 |
147 | Refactor Service with Conditional Logic | 03:20 |
148 | Find By Name with Spring Data JPA | 05:01 |
149 | Complete Implementation | 02:59 |
150 | Complete Search Functionality | 08:24 |
151 | Introduction | 01:50 |
152 | What is Paging and Sorting? | 03:55 |
153 | Paging and Sorting with Spring | 03:14 |
154 | Add Paging Parameters | 04:46 |
155 | Create Page Request Object | 05:44 |
156 | Refactor Spring Data JPA Repositories | 06:53 |
157 | Add Sort Parameter | 03:13 |
158 | Introduction | 02:32 |
159 | Overview of Database Relationships | 06:56 |
160 | Review of Database Changes | 03:43 |
161 | One to Many Bidirectional | 04:49 |
162 | Create Beer Order Repository | 07:17 |
163 | Persisting Beer Order Relationships | 06:43 |
164 | Association Helper Methods | 05:59 |
165 | Many to Many | 06:57 |
166 | Many to Many Persistence | 07:18 |
167 | One to One Bi-Directional | 03:57 |
168 | Cascade on Persist | 04:43 |
169 | Hibernate Cascade Types | 02:33 |
170 | Introduction | 02:48 |
171 | Overview of Database Transactions | 18:48 |
172 | Database Locking Demo | 07:44 |
173 | Spring Data JPA Transactions | 07:32 |
174 | Optimistic Locking Demo | 05:02 |
175 | Introduction | 02:01 |
176 | Project Code Review | 04:58 |
177 | Add Spring Data REST Dependency | 02:39 |
178 | List All With Spring Data REST | 04:26 |
179 | Set Base Path | 01:54 |
180 | Customize URL Path | 04:57 |
181 | Version Property - ETag Header | 02:47 |
182 | API Profile | 03:46 |
183 | Create with Spring Data REST | 02:40 |
184 | Update Data with Spring Data REST | 02:14 |
185 | Delete Data with Spring Data REST | 01:52 |
186 | Use Repository Methods | 02:47 |
187 | Introduction | 02:15 |
188 | Project Code Review | 03:31 |
189 | Create Client Service | 03:46 |
190 | Get List as JSON String | 05:09 |
191 | Get List as Java Map | 04:55 |
192 | Get List as Jackson Object | 03:46 |
193 | Spring Pageable with Jackson | 07:10 |
194 | RestTemplateBuilder Configuration | 04:01 |
195 | Externalize Root URL | 03:34 |
196 | Uri Components Builder | 02:53 |
197 | Query Parameters | 03:35 |
198 | URL Parameters | 04:43 |
199 | HTTP Post with RestTemplate | 03:50 |
200 | Get Response Header | 03:16 |
201 | HTTP Put with RestTemplate | 04:04 |
202 | HTTP Delete with RestTemplate | 02:37 |
203 | Introduction | 01:28 |
204 | Create Mock Test Class | 05:33 |
205 | Using Mockito | 04:48 |
206 | Mock Test Create Beer | 04:50 |
207 | Refactor Setup Method | 02:44 |
208 | Mock Test Update Beer | 05:15 |
209 | Mock Test Delete Beer | 02:11 |
210 | Mock Test Not Found | 02:40 |
211 | Mock Test Query Param | 04:20 |
212 | Introduction | 01:54 |
213 | Adding Spring Security Dependencies | 04:10 |
214 | Calling Rest API with Postman and HTTP Basic | 03:18 |
215 | Customizing User Name and Password | 02:50 |
216 | Testing Spring Security with JUnit 5 | 03:31 |
217 | Spring Security Config - Disable CSRF | 05:27 |
218 | Spring Security with Web Application Context | 04:37 |
219 | HTTP Basic with RestTemplate | 05:51 |
220 | Refactor of RestTemplate Builder Config | 02:07 |
221 | HTTP Basic with RestTemplate Mock Context | 01:56 |
222 | Introduction | 01:48 |
223 | Overview of OAuth 2 and JWT | 08:10 |
224 | Introduction to Spring Authorization Server | 03:38 |
225 | Create New Project | 03:19 |
226 | Add Authorization Server Filter Chain | 06:25 |
227 | Add Default Security Filter Chain | 01:45 |
228 | Create User Details Service | 01:59 |
229 | Add Registered Client Repository | 03:40 |
230 | Create JWK Source | 03:34 |
231 | Create JwtDecoder | 01:13 |
232 | Set Authorization Server Settings | 01:41 |
233 | Get Authorization Token Using Postman | 07:22 |
234 | Introduction | 01:49 |
235 | Maven Dependencies | 02:08 |
236 | Spring Security Configuration | 04:46 |
237 | Testing with Postman | 03:02 |
238 | Spring MockMVC Testing with JWT | 03:35 |
239 | Refactor JWT Tests | 05:04 |
240 | Introduction | 01:49 |
241 | Maven Dependencies and Spring Configuration | 04:01 |
242 | Spring Authorized Client Manager | 03:57 |
243 | Http Request Interceptor | 04:41 |
244 | Add Interceptor to RestTemplate Builder | 05:38 |
245 | Mock OAuth2 Manager | 09:35 |
246 | Introduction | 02:03 |
247 | Reactive Manifesto | 04:31 |
248 | What is Reactive Programming? | 05:54 |
249 | Reactive Streams API | 04:45 |
250 | Create Spring Boot Project | 03:17 |
251 | Implement Repository | 04:47 |
252 | Mono Operations | 06:59 |
253 | Flux Operations | 05:49 |
254 | Filter Flux Objects | 03:36 |
255 | Error Handling | 06:05 |
256 | Step Verifier | 02:47 |
257 | Introduction | 01:56 |
258 | Overview of Spring Data R2DBC | 04:56 |
259 | Create Spring Boot Project | 02:29 |
260 | Initializing Database | 05:11 |
261 | Create Database Entity | 02:43 |
262 | Create Spring Data R2DBC Repository | 02:47 |
263 | Test Save New Entity | 02:37 |
264 | Add Create and Update Date with Auditing | 01:55 |
265 | Initializing Database | 05:42 |
266 | Introduction | 02:12 |
267 | Create WebFlux Controller | 06:15 |
268 | List Flux from Database | 05:10 |
269 | Using Path Variables | 05:49 |
270 | Create New Beer Entity | 05:20 |
271 | Testing Create with Postman | 03:49 |
272 | Update Beer Entity | 06:26 |
273 | Test Update with Postman | 01:39 |
274 | Delete Beer Entity | 03:26 |
275 | Add Validation | 04:28 |
276 | Introduction | 01:34 |
277 | Test List Beers | 03:34 |
278 | Test Get By ID | 02:01 |
279 | Test Create Beer Entity | 03:30 |
280 | Test Update Beer Entity | 02:00 |
281 | Test Delete Beer Entity | 02:02 |
282 | Test Method Order | 04:18 |
283 | Introduction | 02:05 |
284 | Test Validation Failure On Create | 02:02 |
285 | Test Validation Failure On Update | 01:36 |
286 | Test Get By ID Not Found | 02:41 |
287 | Test Update Not Found | 02:06 |
288 | Test Delete Not Found | 03:12 |
289 | Verify MongoDB installation | 02:48 |
290 | Create New Spring Boot Project | 02:34 |
291 | Add Entity, Model, and Mapstruct | 05:01 |
292 | Add Mongo Database and Client Configuration | 01:49 |
293 | Mongo Database Authentication | 03:26 |
294 | Add Spring Data Repository | 03:02 |
295 | Test Save New Document | 05:33 |
296 | Using Awaitility | 03:26 |
297 | Implement CRUD Operations | 07:25 |
298 | Find By Name with Spring Data Mongo | 05:52 |
299 | Find By Beer Style with Spring Data Mongo | 06:12 |
300 | Initializing Database | 02:59 |
301 | WebFlux.fn Overview | 02:35 |
302 | WebFlux Handler | 03:52 |
303 | WebFlux Router | 08:33 |
304 | Get by Id Endpoint | 03:34 |
305 | Create Endpoint | 06:29 |
306 | Update Endpoint | 03:24 |
307 | Patch Endpoint | 02:41 |
308 | Delete Endpoint | 02:09 |
309 | Return HTTP 404 on not found | 04:12 |
310 | Add Validation | 06:32 |
311 | Using Query Parameters with WebFlux.fn | 04:39 |
312 | Create New Spring Boot Project | 01:37 |
313 | Get List as JSON String | 05:23 |
314 | Get List as Java Map | 04:35 |
315 | Get List as Jackson Object | 02:51 |
316 | Get List as Java POJOs | 03:13 |
317 | Webclient Global Configuration | 04:34 |
318 | Uri Components Builder | 03:18 |
319 | Query Parameters | 02:39 |
320 | HTTP Post with WebClient | 05:36 |
321 | HTTP Put with WebClient | 02:34 |
322 | Maven Dependencies | 01:53 |
323 | Spring Security Configuration | 04:41 |
324 | Testing API Using Postman | 01:52 |
325 | Spring webTestClient Testing with JWT | 04:48 |
326 | Maven Dependencies | 02:44 |
327 | Spring Security Configuration | 06:40 |
328 | Testing with Postman | 02:33 |
329 | Spring webTestClient Testing with JWT | 04:58 |
330 | Maven Dependencies and Spring Configuration | 02:02 |
331 | Spring Security Configuration | 03:45 |
332 | WebClient Filter Configuration | 05:34 |
333 | Overview of Spring Cloud Gateway | 03:24 |
334 | Create Spring Cloud Gateway Project | 03:03 |
335 | Server Port Mapping | 04:27 |
336 | v1 Route Mapping | 05:22 |
337 | Troubleshooting Spring Cloud Gateway | 06:05 |
338 | Gateway Resource Server Configuration | 08:56 |
339 | The Maven Build Lifecycle | 05:38 |
340 | Maven from the Command Line | 05:13 |
341 | Spring Boot Executable Jars | 03:18 |
342 | Running Spring Boot Executable Jars | 03:13 |
343 | Spring Boot Repackage to Executable Jar | 02:54 |
344 | Running Spring Boot With Maven | 03:42 |
345 | Resource Filtering | 04:15 |
346 | Maven Build Info | 03:11 |
347 | Introduction to the Spring Boot Gradle Plugin | 06:32 |
348 | The Gradle Build LIfecycle | 04:17 |
349 | Gradle from the Command Line | 03:35 |
350 | Running Spring Boot with Gradle | 01:55 |
351 | Spring Boot Build Info | 02:03 |
352 | Introduction to OpenAPI | 06:44 |
353 | OpenAPI for Spring Boot Development | 04:59 |
354 | Springdoc Maven Dependencies | 02:59 |
355 | Springdoc Spring Security Configuration | 07:07 |
356 | Save OpenAPI Specification in Maven Build | 06:34 |
357 | Atlassian Swagger Request Validator | 04:41 |
358 | RestAssured Test | 03:47 |
359 | Spring Security Configuration | 04:22 |
360 | Configure Swagger Request Validator | 04:12 |
361 | Whitelist Validation Rules | 03:48 |
362 | Types of Artificial Intelligence | 06:13 |
363 | Introduction to Large Language Models (LLMs) | 06:50 |
364 | Overview of OpenAI | 08:33 |
365 | Setting Up Your Development Environment for Spring AI | 04:11 |
366 | Create New Spring Boot Project | 03:14 |
367 | Configure OpenAI API Keys | 04:09 |
368 | Add OpenAI Service | 05:05 |
369 | Exploring LLM Capabilities | 16:20 |
370 | Create Spring MVC Controller | 03:33 |
371 | Test Controller with Postman | 03:17 |
372 | Using Prompt Templates | 05:20 |
373 | Directing OpenAI to format the Response | 06:07 |
374 | Directing OpenAI to Respond with JSON | 03:23 |
375 | Binding OpenAI Responses With JSON Schema | 06:45 |
376 | OpenAI Response Meta Data | 02:55 |
377 | Project Review | 06:00 |
378 | Implement Create Beer Entity | 06:15 |
379 | Implement Get By ID | 02:24 |
380 | Implement Update By ID | 03:00 |
381 | Implement Delete By Id | 02:23 |
382 | Implement List Beers | 03:11 |
383 | Test Operations Against Real Server | 04:40 |
384 | Java Virtual Threads | 04:41 |
385 | Introduction to Actuator | 04:04 |
386 | Actuator Configuration | 03:27 |
387 | Alternate Security Configuration | 01:27 |
388 | Spring Boot Actuator Kubernetes Integration | 03:07 |
389 | Add Actuator to WebFlux | 03:04 |
390 | Introduction to Logbook | 03:22 |
391 | Logbook with Spring MVC | 04:10 |
392 | Logstash Configuration for JSON Log Output | 06:44 |
393 | Logbook with Webflux | 04:09 |
394 | Logbook with Spring RestTemplate | 03:51 |
395 | Logbook with Spring WebClient | 03:45 |
396 | Introduction to Caching | 07:08 |
397 | Add Cache Dependencies and Config | 01:52 |
398 | Configure Method Cache for Get By Id | 05:25 |
399 | Configure Cache for List Beers | 04:34 |
400 | Cache Eviction | 04:52 |
401 | Using Spring Cache Manager | 03:49 |
402 | Introduction to Spring Events | 04:25 |
403 | Create Application Event Pojos | 02:45 |
404 | Create Application Event Listener | 02:41 |
405 | Publish Spring Event | 04:12 |
406 | Test Application Event | 02:42 |
407 | Asynchronous Event Processing | 04:17 |
408 | Add Audit Entity | 04:38 |
409 | Add Audit Mapper | 02:32 |
410 | Persist Audit Event | 03:55 |
411 | Your Assignment - Add Order Functionality | 03:09 |
412 | Create DTOs | 04:26 |
413 | Create Mappers | 03:35 |
414 | Add Validation | 03:12 |
415 | Initialize Order Data | 11:38 |
416 | TDD - Get Operations | 08:17 |
417 | Implement Get Operations | 07:53 |
418 | Add Create Order DTO | 04:44 |
419 | TDD - Create Operation | 03:57 |
420 | Implement Create Operation | 05:08 |
421 | Add Order Update DTO | 05:10 |
422 | TDD - Update Operations | 03:51 |
423 | Implement Update Operations | 07:37 |
424 | TDD Delete Operations | 02:23 |
425 | Implement Delete Operations | 03:18 |
426 | Fix Failing Tests | 03:33 |
427 | Introduction to Docker | 06:06 |
428 | Creating a Docker Image with Maven | 03:42 |
429 | Running Images with Docker | 05:58 |
430 | Running Auth Server | 03:24 |
431 | Spring Configuration for Docker | 06:04 |
432 | Update Gateway Routes and Security | 03:47 |
433 | Running Gateway in Docker | 03:17 |
434 | Running RestMVC in Docker | 04:10 |
435 | Running MySQL in Docker | 02:52 |
436 | Running RestMVC Container with MySQL | 03:34 |
437 | Running Reactive Container | 03:15 |
438 | Running Reactive Mongo | 11:03 |
439 | Environment Clean Up | 02:16 |
440 | Introduction to Docker Compose | 03:31 |
441 | Create Docker Compose File | 06:16 |
442 | Add Auth Server and RestMVC to Compose File | 06:16 |
443 | Add Gateway to Compose and Test | 06:47 |
444 | Using Docker Compose with IntelliJ | 02:35 |
445 | Introduction to Kubernetes | 04:45 |
446 | Enable Kubernetes in Docker Desktop | 02:53 |
447 | Overview of Kubernetes Deployments | 05:02 |
448 | Deploy Mongo Pod and Service | 04:59 |
449 | Set Mongo Environment Variables | 04:51 |
450 | Debug CrashLoop on RestMVC | 05:14 |
451 | Kubernetes Port Forwarding and Testing in Postman | 05:48 |
452 | Introduction to Microservices | 06:12 |
453 | Twelve Factor Applications | 10:00 |
454 | The Reactive Manifesto | 05:38 |
455 | Enterprise Integration Patterns | 06:31 |
456 | Starbucks Does Not Use a Two-Phase Commit | 06:57 |
457 | Microservice Project Overview | 06:26 |
458 | Create Shared Message Library | 04:57 |
459 | Refactor Spring MVC Project to use Message Library | 04:20 |
460 | Send Order Placed Application Event | 05:46 |
461 | Interview with Marcin Grzejszczak - Lead for Spring Cloud Contract | 21:16 |
462 | Interview with James Labocki of Red Hat | 16:04 |
463 | KBE - Course Introduction | 00:53 |
464 | KBE - Setting Up Your Development Environment | 02:49 |
465 | KBE - Introduction | 01:01 |
466 | KBE - Spring Boot Project Code Review | 07:44 |
467 | KBE - Creating Docker File | 06:14 |
468 | KBE - Building and Running Docker Image | 04:45 |
469 | KEB - Introduction - Building Layered Image | 01:39 |
470 | KBE - Overview and Maven Configuration | 05:19 |
471 | KBE - Multi-Stage Docker File | 07:44 |
472 | KBE - Introduction - Building Docker Images with Maven | 04:42 |
473 | KBE - Introducing Docker Maven | 09:07 |
474 | KBE - Using Properties in Builds | 04:42 |
475 | KBE - Pushing to Docker Hub | 03:59 |
476 | KBE - Course Introduction | 01:20 |
477 | KBE - Setting Up Your Development Environment | 03:16 |
478 | KBE - Enable Kubernetes in Docker Desktop | 03:32 |
479 | KBE - Introduction to Deploying on Kubernetes | 00:52 |
480 | KBE - Create Deployment | 04:51 |
481 | KBE - Create Service | 02:56 |
482 | KBE - Port Forwarding | 04:19 |
483 | KBE - Terminating Services and Deployments | 02:13 |
484 | KBE - Exposing Services | 03:48 |
485 | KBE - Accessing Logs | 02:52 |
486 | KBE - Setting Environment Variables | 04:53 |
487 | KBE - Readiness Probe | 05:10 |
488 | KBE - Liveness Probe | 04:29 |
489 | KBE - Graceful Shutdown | 03:50 |
490 | KBE - Course Introduction | 01:43 |
491 | KBE - Setting Up Your Development Environment | 03:16 |
492 | KBE - Enable Kubernetes in Docker Desktop | 03:32 |
493 | KBE - Introduction to Spring Boot Microservices on Kubernetes | 01:54 |
494 | KBE - Overview of Microservices Used in This Course | 06:33 |
495 | KBE - Source Code Review | 04:10 |
496 | KBE - Running Services via Docker Compose | 11:11 |
497 | KBE - Introduction - Infrastructure Services | 01:21 |
498 | KBE - MySQL Service | 06:00 |
499 | KBE - JMS Service | 03:21 |
500 | KBE - Introduction to Spring Boot Microservices | 02:00 |
501 | KBE - Inventory Service | 04:10 |
502 | KBE - Inventory Failover Service | 02:41 |
503 | KBE - Beer Service | 04:25 |
504 | KBE - Order Service | 04:36 |
505 | KBE - Add Readiness and Liveness Probe Configuration | 06:50 |
506 | KBE - Add Graceful Shutdown | 05:23 |
507 | KBE - Kubernetes Ingress Controllers | 05:48 |
508 | KBE - Spring Cloud Gateway Service | 07:36 |
509 | KBE - Deleting Services and Deployments | 02:20 |
510 | KBE - Introduction to Consolidated Logging | 01:47 |
511 | KBE - Logging Configuration Code Review | 03:25 |
512 | KBE - Elasticsearch | 03:33 |
513 | KBE - Kibana | 03:29 |
514 | KBE - Filebeat | 10:40 |
Similar courses to Spring Framework 6 Beginner to Guru
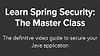
Learn Spring Security: The Master Class
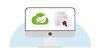
Spring Professional Certification Exam Tutorial - Module 01
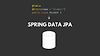
Spring Data JPA Master Class
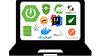
Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)
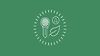
Learn Spring Security OAuth: The Master Class
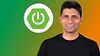
Master Spring Boot 3 & Spring Framework 6 with Java
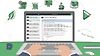
Learn Spring 5 and Spring Boot 2
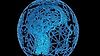
Master Microservices with Java, Spring, Docker, Kubernetes
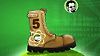
Spring Framework 5: Beginner to Guru
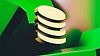