[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners
Spring is an enterprise Java framework. It was designed to simplify Java EE development and make developers more productive. Spring makes use of Inversion of Control and Dependency Injection to promote good software coding practices and speed up development time. This course covers Spring Core, Annotations, All Java Spring Configuration, Spring AOP, Spring MVC, Spring Security, Spring REST, Spring Boot and Spring Data JPA.
More
LEARN these HOT TOPICS in Spring 5:
Spring Framework 5
Spring Core
Spring Annotations
Spring Java Configuration (all Java, no xml)
Spring AOP
Spring MVC
Hibernate CRUD
JPA CRUD
Spring Security
Spring REST
Maven
SPRING BOOT
Spring Boot Starters
Spring Boot and Hibernate
Spring Boot and Spring Data JPA
Spring Boot and Spring Data REST
Spring Boot, Thymeleaf and Spring MVC
REAL-TIME PROJECTS
Spring MVC and Hibernate CRUD real-time project
Spring Security (with password encryption in the database)
Spring REST (with full database CRUD real-time project)
Spring Boot REST (with full database CRUD real-time project)
Spring Boot with JPA and Spring Data JPA (with full database CRUD real-time project)
Spring Boot with Spring Data REST (with full database CRUD real-time project)
Spring Boot with Thymeleaf (with full database CRUD real-time project)
Live Coding - I code all of the real-time projects from scratch
All source code is available for download
Responsive Instructor - All questions answered within 24 hours
PDFs of all lectures are available for download
Closed-Captions / Subtitles available for English and other languages (new!)
Professional video and audio recordings (check the free previews)
This course includes mini-courses on Maven, Spring Security, Spring REST and Spring Boot. These mini-courses are designed to help you quickly get started with Maven, Spring Security, Spring REST and Spring Boot.
Mini-Courses
Maven mini-course includes 16 videos (1 hour of video)
Spring Security mini-course includes 68 videos (5 hours of video)
Spring REST mini-course includes 55 videos (4.5 hours of video)
Spring Boot mini-course includes 39 videos (6.5 hours of video)
What Is Hibernate?
Hibernate is an Object-to-Relational-Mapping (ORM) framework. It simplifies database access for Java applications. By using the framework, you can easily store and retrieve Java objects by setting up some simple configuration mappings.
This course covers basic Hibernate CRUD. Also, advanced Hibernate mappings are covered for one-to-one, one-to-many and many-to-many.
Benefits of Taking This Spring and Hibernate Course
Knowing Spring and Hibernate can get you a job or improve the one you have. It's a skill that will put you more in demand in the enterprise Java industry, and make your software life easier, that's why it's so popular.
Nearly every job posting asks for skills in Spring and Hibernate!
This course will help you quickly get up to speed with Spring and Hibernate. I will demystify the technology and help you understand the essential concepts to build a real Spring and Hibernate application from scratch.
You Will Learn How To:
Spring Core
Build a complete Spring MVC and Hibernate CRUD Project ... all from scratch
Set up your Spring and Hibernate development environment with Tomcat and Eclipse
Wire beans together in the Spring container using Inversion of Control
Configure the Spring container for Dependency Injection
Define Spring Beans using the @Component annotation
Perform auto-scanning of Spring beans to minimize configuration
Automatically wire beans together using @Autowired annotation
Apply all Java configuration to Spring Beans (no xml)
Spring MVC
Set up your Spring MVC environment with configs and directories
Create controllers using @Controller annotation
Read HTML form data using @RequestParam
Leverage Spring MVC model to transport data between controller and view page
Define Request Mappings for GET and POST requests
Minimize coding with Spring MVC Form data binding
Apply Spring MVC form validation on user input
Create custom Spring MVC form validation rules
Hibernate
Perform object/relational mapping with Hibernate
Leverage the Hibernate API to develop CRUD apps
Develop queries using the Hibernate Query Language (HQL)
Apply advanced Hibernate mappings: one-to-one, one-to-many and many-to-many
Create a real-time project using Spring and Hibernate together in a Real-Time Project
Spring AOP
Apply Aspect-Oriented-Programming AOP for cross-cutting concerns
Examine AOP use-cases and how AOP can resolve code-tangling
Create AOP pointcut expressions to match on method invocations
Leverage AOP annotations: @Before, @After, @AfterReturning, @AfterThrowing, @Around
Create a real-time project using AOP and Spring MVC together in a Real-Time Project
Spring Security
Secure your web application with Spring Security
Set up your Maven pom.xml file with compatible Spring Security dependencies
Configure Spring Security with all Java configuration (no xml)
Create custom Spring Security login pages with Bootstrap CSS
Add logout support using default features of Spring Security
Leverage Spring Security support for Cross Site Request Forgery (CSRF)
Define users and roles for authentication
Display user login info and role using Spring Security tags
Restrict access to URLs based on user role
Hide and Display content based on user role
Add JDBC authentication, store user accounts and passwords in the database
Store encrypted passwords in the database using bcrypt
Register new users and encrypt passwords using Java code
Create a Spring Security Real-Time Project using authorization, authentication and database encryption
Spring REST Web Services - Spring REST APIs
Overview of REST Web Services - REST APIs
Investigating Spring REST support
Sending JSON data over HTTP
JSON Data Binding with the Jackson project
Converting JSON data to Java POJO with Jackson
Processing nested JSON objects and JSON arrays
Developing a Spring REST API
Setting up a Spring REST project with Maven
Creating the Spring REST Controller using @RestController
Running the Spring REST Controller with Eclipse and Tomcat
Testing Spring REST Web Services with Postman
Parameterize REST API endpoints using @PathVariable
Add Spring REST exception handling with @ExceptionHandler
Integrate global REST exception handling with @ControllerAdvice
Leverage ResponseEntity for fine-grained control of Spring REST HTTP response
Build REST API to execute CRUD actions on the Database with Hibernate
Create a Real-Time Project using REST API with full database CRUD
Spring Boot
What is Spring Boot?
Creating a Project with Spring Boot Initializr
Develop a REST API Controller with Spring Boot
Explore the Spring Boot Project Structure
Leverage Spring Boot Starters - A Curated List of Dependencies
Inherit Defaults with Spring Boot Starter Parents
Automatically Restart with Spring Boot Dev Tools
Add DevOps functionality with Spring Boot Actuator Endpoints
Secure Spring Boot Actuator Endpoints
Run Spring Boot apps from the Command-Line
Use the Spring Boot Maven Plugin to package and run Spring Boot apps
Inject custom application properties into a Spring Boot REST Controller
Spring Boot REST API CRUD
Develop a REST API Controller with Spring Boot with full CRUD support
Configure Spring Boot Data Source for MySQL Database
Create DAO implementations using JPA Entity Manager
Apply Best Practices by integrating a Service Layer
Expose REST API endpoints in Controller code (GET, POST, PUT and DELETE)
Access the REST API using Postman
Add support for Standard JPA API
Learn the benefits of the JPA API in Spring Boot applications
Spring Boot and Spring Data JPA
Minimize boilerplate code with Spring Data JPA and the JpaRepository
Refactor existing REST API to integrate with Spring Data JPA
Leverage new features of the the Java Optional pattern with JpaRepository
Test the Spring Data JPA repository with Postman
Spring Boot and Spring Data REST
Accelerate your development process with Spring Data REST
Leverage Spring Data REST to eliminate custom code for controllers and service
Automatically expose REST endpoints for your JPA Repositories
Customize REST base path endpoints
Apply pagination and sorting to REST API endpoints
Configure default page sizes for REST APIs
Investigate HATEOAS compliant REST responses
Test Spring Data REST with Postman
Spring Boot and Thymeleaf
Develop view templates with Thymeleaf in Spring Boot projects
Compare the functionality of Thymeleaf to JSP
Examine the auto-configuration of Thymeleaf in Spring Boot projects
Create a Spring Boot project with Thymeleaf using the Spring Initializer website
Develop a Spring MVC Controller and a Thymeleaf template
Leverage Thymeleaf expressions to access data from the Spring MVC Model
Apply CSS stylesheets to your Thymeleaf templates
Spring Boot, Thymeleaf and Database CRUD
Develop a real-time project with Thymeleaf and Spring Boot with full database CRUD support
Integrate the @Repository to execute CRUD actions on the Database with Spring Data JPA
Apply best practices with the @Service layer design pattern
Create a @Controller to handle web browser requests
Develop Thymeleaf templates to render database results
Beautify your Thymeleaf templates with Bootstrap CSS
Maven
Simplify your build process with Maven
Create Maven POM files and add dependencies
Search Central Maven repository for Dependency Coordinates
Run Maven builds from the Eclipse IDE
Use Maven during the development of Real-Time Projects for Spring MVC, Spring Security, Spring REST, Spring Boot and Hibernate.
Compared to other Spring/Hibernate courses
This course is fully up to date and covers the latest versions of Spring 5 and Hibernate 5. The course also includes new content on Spring Boot, Spring Data JPA, Spring Data REST and Thymeleaf.
Beware of other Udemy Spring/Hibernate courses. Most of them are outdated and use old versions of Spring and Hibernate. Don’t waste your time or money on learning outdated technology.
Also, I create all of the code from scratch in this course. Beware of other courses, those instructors simply copy/paste from their github repo or they use pre-written code. Their approach is not ideal for real-time learning.
Take my course where I show you how to create all of the code from scratch. You can type the code along with me in the videos, which is the best way to learn.
I am a very responsive instructor and I am available to answer your questions and help you work through any problems.
Finally, all source code is provided with the course along with setup instructions.
Quality Material
You will receive a quality course, with solid technical material and excellent audio and video production. This is my fifth course at Udemy.
My first four courses on Udemy were:
Eclipse IDE for Beginners
Java Database Connection (JDBC)
JavaServer Faces (JSF) for Beginners
JSP and Servlets for Beginners
These courses have received rave 5 star reviews and over 315,000 students have taken the courses. Also, these courses are the most popular courses in their respective categories.
Similar thing for this Spring course, it is ranked as #1 best seller for Spring courses.
I also have an active YouTube channel where I post regular videos. In the past year, I’ve created over 300 video tutorials (public and private). My YouTube channel has over 5 million views and 36k subscribers. So I understand what works and what doesn’t work for creating video tutorials.
Target Audience
Java Developers with basic Java experience
- Basic Java knowledge is required
- Basic HTML knowledge is helpful
- The course is appropriate for all Java developers: beginners to advanced
What you'll learn:
- Develop a REAL-TIME project with Spring MVC, Spring REST, Spring Boot and Hibernate CRUD ... all from SCRATCH
- You will TYPE IN EVERY LINE of code with me in the videos. I EXPLAIN every line of code to help you learn!
- LEARN key Spring 5 features: Core, Annotations, Java Config, AOP, Spring MVC, Hibernate and Maven
- I am a RESPONSIVE INSTRUCTOR ... post your questions and I will RESPOND in 24 hours.
- POPULAR VIDEOS for: Spring Boot 2, Spring Security, Spring REST, Spring Data JPA, Spring Data REST and Thymeleaf
- Join an ACTIVE COMMUNITY of 140,000+ students that are already enrolled! Over 38,000+ Reviews - 5 STARS
- Students have LANDED NEW JOBS with the skills from this course. Spring and Hibernate developers are in HIGH-DEMAND!
- You can DOWNLOAD all videos, source code and PDFs. Perfect for offline LEARNING and REVIEW.
Watch Online [NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners
# | Title | Duration |
---|---|---|
1 | Introduction | 01:20 |
2 | Java Development Environment Checkpoint | 02:21 |
3 | Spring Boot Overview | 07:21 |
4 | Spring Boot Initialzr Demo | 09:58 |
5 | Spring Boot - Create a REST Controller | 05:06 |
6 | Spring Projects | 01:57 |
7 | What is Maven? | 04:58 |
8 | Maven Project Structure | 04:20 |
9 | Maven Key Concepts | 06:56 |
10 | Exploring Spring Boot Project Files - Part 1 | 05:14 |
11 | Exploring Spring Boot Project Files - Part 2 | 04:53 |
12 | Spring Boot Starters | 06:37 |
13 | Spring Boot Parents for Starters | 02:52 |
14 | Spring Boot Dev Tools - Overview | 02:33 |
15 | Spring Boot Dev Tools - Coding | 10:51 |
16 | Spring Boot Actuator - Overview | 06:41 |
17 | Spring Boot Actuator - Accessing Endpoints - Part 1 | 07:31 |
18 | Spring Boot Actuator - Accessing Endpoints - Part 2 | 06:07 |
19 | Spring Boot Actuator - Securing Endpoints - Overview | 03:25 |
20 | Spring Boot Actuator - Securing Endpoints - Coding | 08:01 |
21 | Run Spring Boot apps from the Command Line - Overview | 04:12 |
22 | Run Spring Boot apps from the Command Line - Prep | 03:21 |
23 | Run Spring Boot apps from the Command Line - Microsoft Windows | 07:33 |
24 | Run Spring Boot apps from the Command Line - macOS / Linux | 05:20 |
25 | Injecting Custom Application Properties - Overview | 03:18 |
26 | Injecting Custom Application Properties - Coding | 07:38 |
27 | Configuring the Spring Boot Server - Overview | 06:19 |
28 | Configuring the Spring Boot Server - Coding | 04:49 |
29 | What is Inversion of Control? | 02:21 |
30 | Defining Dependency Injection - Overview - Part 1 | 04:01 |
31 | Defining Dependency Injection - Overview - Part 2 | 03:52 |
32 | Constructor Injection - Coding - Part 1 | 04:00 |
33 | Constructor Injection - Coding - Part 2 | 09:11 |
34 | IDE Warning - No Usages | 01:14 |
35 | Constructor Injection - Behind the Scenes | 02:05 |
36 | Component Scanning - Overview | 04:58 |
37 | Component Scanning - Coding - Part 1 | 04:04 |
38 | Component Scanning - Coding - Part 2 | 05:26 |
39 | Setter Injection - Overview | 03:19 |
40 | Setter Injection - Coding | 05:42 |
41 | Field Injection | 02:27 |
42 | Qualifiers - Overview | 02:52 |
43 | Qualifiers - Coding - Part 1 | 04:38 |
44 | Qualifiers - Coding - Part 2 | 04:49 |
45 | Primary - Overview | 03:52 |
46 | Primary - Coding | 06:23 |
47 | Lazy Initialization - Overview | 04:57 |
48 | Lazy Initialization - Coding - Part 1 | 05:34 |
49 | Lazy Initialization - Coding - Part 2 | 03:12 |
50 | Bean Scopes - Overview | 03:39 |
51 | Bean Scopes - Coding | 07:10 |
52 | Bean Lifecycle Methods - Overview | 01:59 |
53 | Bean Lifecycle Methods - Coding | 05:58 |
54 | Java Config Bean - Overview | 07:00 |
55 | Java Config Bean - Coding - Part 1 | 04:31 |
56 | Java Config Bean - Coding - Part 2 | 04:31 |
57 | Hibernate / JPA Overview | 09:21 |
58 | Hibernate, JPA and JDBC | 01:07 |
59 | Setting Up Development Environment | 01:50 |
60 | Setting Up Database Table - Overview | 01:03 |
61 | Setting Up Database Table - Coding | 05:46 |
62 | Setting Up Spring Boot Project - Overview | 03:27 |
63 | Setting Up Spring Boot Project - Coding - Part 1 | 05:24 |
64 | Setting Up Spring Boot Project - Coding - Part 2 | 07:47 |
65 | JPA Annotations - Overview | 08:11 |
66 | JPA Annotations - Coding | 07:56 |
67 | Saving a Java Object with JPA - Overview - Part 1 | 07:34 |
68 | Saving a Java Object with JPA - Overview - Part 2 | 05:06 |
69 | Saving a Java Object with JPA - Coding - Part 1 | 05:41 |
70 | Saving a Java Object with JPA - Coding - Part 2 | 05:25 |
71 | Primary Keys | 05:26 |
72 | Changing Index of MySQL Auto Increment | 03:22 |
73 | Reading Objects with JPA - Overview | 03:03 |
74 | Reading Objects with JPA - Coding | 06:41 |
75 | Querying Objects with JPA - Overview | 08:14 |
76 | Querying Objects with JPA - Coding - Part 1 | 09:08 |
77 | Querying Objects with JPA - Coding - Part 2 | 06:47 |
78 | Updating Objects with JPA - Overview | 03:28 |
79 | Updating Objects with JPA - Coding | 06:28 |
80 | Deleting Objects with JPA - Overview | 03:47 |
81 | Deleting Objects with JPA - Coding - Part 1 | 05:05 |
82 | Deleting Objects with JPA - Coding - Part 2 | 04:39 |
83 | Create Database Tables from Java Code - Overview | 05:46 |
84 | Create Database Tables from Java Code - Coding - Part 1 | 05:03 |
85 | Create Database Tables from Java Code - Coding - Part 2 | 05:02 |
86 | What Are REST Services - Part 1 | 05:20 |
87 | What Are REST Services - Part 2 | 04:04 |
88 | JSON Basics | 03:40 |
89 | Spring Boot REST HTTP Basics | 07:01 |
90 | Postman Demo | 04:14 |
91 | Spring Boot REST Controller - Overview - Part 1 | 03:07 |
92 | Spring Boot REST Controller - Overview - Part 2 | 01:12 |
93 | Spring Boot Rest Controller - Coding | 08:12 |
94 | JSON Jackson Data Binding | 06:41 |
95 | Spring Boot REST POJO - Overview | 06:18 |
96 | Spring Boot REST POJO - Coding - Part 1 | 02:29 |
97 | Spring Boot REST POJO - Coding - Part 2 | 06:27 |
98 | Spring Boot REST Path Variables - Overview | 04:23 |
99 | Spring Boot REST Path Variables - Coding - Part 1 | 04:13 |
100 | Spring Boot REST Path Variables - Coding - Part 2 | 04:36 |
101 | Spring Boot REST Exception Handling - Overview - Part 1 | 03:41 |
102 | Spring Boot REST Exception Handling - Overview - Part 2 | 04:32 |
103 | Spring Boot REST Exception Handling - Coding - Part 1 | 04:45 |
104 | Spring Boot REST Exception Handling - Coding - Part 2 | 07:00 |
105 | Spring Boot REST Exception Handling - Coding - Part 3 | 03:22 |
106 | Spring Boot REST Exception Handling - Coding - Part 4 | 04:28 |
107 | Spring Boot REST Global Exception Handling - Overview | 05:26 |
108 | Spring Boot REST Global Exception Handling - Coding | 03:37 |
109 | Spring Boot REST API Design - Best Practices | 04:58 |
110 | Spring Boot REST API Design - API Design of Real-Time Projects | 03:35 |
111 | Spring Boot REST Project Overview | 01:58 |
112 | Spring Boot REST Setup Sample Data | 03:49 |
113 | Spring Boot REST Create Project | 05:39 |
114 | Spring Boot REST DAO | 02:08 |
115 | Spring Boot REST DAO - Coding - Part 1 | 09:51 |
116 | Spring Boot REST DAO - Coding - Part 2 | 05:44 |
117 | Spring Boot REST DAO - Coding - Part 3 | 05:29 |
118 | Spring Boot Define Service Layer - Overview | 03:49 |
119 | Spring Boot Define Service Layer - Coding | 06:32 |
120 | Spring Boot DAO: Add, Update, Delete - Overview | 03:43 |
121 | Spring Boot DAO: Add, Update, Delete - Coding | 06:27 |
122 | Spring Boot Service: Add, Update, Delete - Coding | 03:46 |
123 | Spring Boot REST: Get Single Employee - Coding | 07:56 |
124 | Spring Boot REST: Add Employee - Coding | 05:26 |
125 | Spring Boot REST: Update Employee - Coding | 06:20 |
126 | Spring Boot REST: Delete Employee - Coding | 05:26 |
127 | Spring Boot REST: Spring Data JPA - Overview | 08:18 |
128 | Spring Boot REST: Spring Data JPA - Coding - Part 1 | 11:32 |
129 | Spring Boot REST: Spring Data JPA - Coding - Part 2 | 04:33 |
130 | Spring Boot REST: Spring Data REST - Overview | 08:29 |
131 | Spring Boot REST: Spring Data REST - Coding - Part 1 | 06:04 |
132 | Spring Boot REST: Spring Data REST - Coding - Part 2 | 07:22 |
133 | Spring Boot REST: Spring Data REST Configs and Sorting - Overview | 04:16 |
134 | Spring Boot REST: Spring Data REST Configs and Sorting - Coding | 06:06 |
135 | Spring Boot REST API Security Overview | 06:48 |
136 | Spring Boot REST API Security - Coding - Part 1 | 04:22 |
137 | Spring Boot REST API Security - Coding - Part 2 | 05:25 |
138 | Spring Boot REST API Security - Basic Configuration - Overview | 03:24 |
139 | Spring Boot REST API Security - Basic Configuration - Coding | 08:19 |
140 | Spring Boot REST API Security - Restrict URLs based on Roles - Overview | 08:32 |
141 | Spring Boot REST API Security - Restrict URLs based on Roles - Coding - Part 1 | 06:22 |
142 | Spring Boot REST API Security - Restrict URLs based on Roles - Coding - Part 2 | 06:47 |
143 | Spring Boot REST API Security - Restrict URLs based on Roles - Coding - Part 3 | 05:11 |
144 | 403 ERROR with PUT REQUEST - Spring Data REST | 01:24 |
145 | Spring Boot REST API Security - JDBC Authentication - Plain Text - Overview | 06:58 |
146 | Spring Boot REST API Security - JDBC Authentication - Plain Text - Coding Part 1 | 05:29 |
147 | Spring Boot REST API Security - JDBC Authentication - Plain Text - Coding Part 2 | 04:29 |
148 | Spring Boot REST API Security - JDBC Authentication - Plain Text - Coding Part 3 | 04:46 |
149 | Spring Boot REST API Security - BCrypt Encryption - Overview - Part 1 | 05:05 |
150 | Spring Boot REST API Security - BCrypt Encryption - Overview - Part 2 | 04:00 |
151 | Spring Boot REST API Security - BCrypt Encryption - Coding | 07:13 |
152 | Spring Boot REST API Security - JDBC Authentication - Custom Tables - Overview | 03:38 |
153 | Spring Boot REST API Security -Custom Tables - Coding - Part 1 | 04:05 |
154 | Spring Boot REST API Security -Custom Tables - Coding - Part 2 | 05:32 |
155 | Spring Boot REST API Security -Custom Tables - Coding - Part 3 | 06:22 |
156 | Spring Boot - Spring MVC with Thymeleaf - Overview | 05:23 |
157 | Spring Boot - Spring MVC with Thymeleaf - Coding - Part 1 | 03:15 |
158 | Spring Boot - Spring MVC with Thymeleaf - Coding - Part 2 | 06:22 |
159 | Spring Boot - Spring MVC with Thymeleaf and CSS - Overview | 04:24 |
160 | Spring Boot - Spring MVC with Thymeleaf and CSS - Coding | 03:14 |
161 | Spring Boot - Spring MVC Behind the Scenes | 05:05 |
162 | Spring Boot - Hello World Form and Model Overview | 02:51 |
163 | Spring Boot - Hello World Form and Model - Coding - Part 1 | 05:51 |
164 | Spring Boot - Hello World Form and Model - Coding - Part 2 | 06:35 |
165 | Spring Boot - Adding Data to Spring MVC Model - Overview | 05:36 |
166 | Spring Boot - Adding Data to Spring MVC Model - Coding - Part 1 | 05:05 |
167 | Spring Boot - Adding Data to Spring MVC Model - Coding - Part 2 | 03:16 |
168 | Spring Boot - Spring MVC Binding Request Params - Overview | 01:36 |
169 | Spring Boot - Spring MVC Binding Request Params - Coding | 05:25 |
170 | Spring Boot - GetMapping and PostMapping - Overview | 04:33 |
171 | Spring Boot - GetMapping and PostMapping - Coding - Part 1 | 04:05 |
172 | Spring Boot - GetMapping and PostMapping - Coding - Part 2 | 07:01 |
173 | Spring Boot - Spring MVC Form Data Binding - Text Fields - Overview | 08:03 |
174 | Spring Boot - Spring MVC Form Data Binding - Text Fields - Coding - Part 1 | 05:29 |
175 | Spring Boot - Spring MVC Form Data Binding - Text Fields - Coding - Part 2 | 07:37 |
176 | Spring Boot - Spring MVC Form Data Binding - Text Fields - Coding - Part 3 | 03:33 |
177 | Spring Boot - Spring MVC Form Data Binding - Drop-Down Lists - Overview | 02:27 |
178 | Spring Boot - Spring MVC Form Data Binding - Drop-Down Lists - Coding - Part 1 | 05:42 |
179 | Spring Boot - Spring MVC Form Data Binding - Drop-Down Lists - Coding - Part 2 | 08:18 |
180 | Spring Boot - Spring MVC Form Data Binding - Radio Buttons - Overview | 01:47 |
181 | Spring Boot - Spring MVC Form Data Binding - Radio Buttons - Coding - Part 1 | 05:31 |
182 | Spring Boot - Spring MVC Form Data Binding - Radio Buttons - Coding - Part 2 | 06:34 |
183 | Spring Boot - Spring MVC Form Data Binding - Check Boxes - Overview | 01:30 |
184 | Spring Boot - Spring MVC Form Data Binding - Check Boxes - Coding - Part 1 | 09:23 |
185 | Spring Boot - Spring MVC Form Data Binding - Check Boxes - Coding - Part 2 | 05:40 |
186 | Spring Boot - Spring MVC Validation - Overview | 02:24 |
187 | Spring Boot - Spring MVC Validation - Setup Dev Environment | 04:34 |
188 | Spring Boot - Spring MVC Validation - Required Fields - Overview | 06:21 |
189 | Spring Boot - Spring MVC Validation - Required Fields - Coding - Part 1 | 03:44 |
190 | Spring Boot - Spring MVC Validation - Required Fields - Coding - Part 2 | 02:57 |
191 | Spring Boot - Spring MVC Validation - Required Fields - Coding - Part 3 | 08:37 |
192 | Spring Boot - Spring MVC Validation - Required Fields - Coding - Part 4 | 04:01 |
193 | Spring Boot - Spring MVC Validation - Required Fields - Coding - Part 5 | 07:40 |
194 | Spring Boot - Spring MVC Validation - @InitBinder - Overview | 03:56 |
195 | Spring Boot - Spring MVC Validation - @InitBinder - Coding | 06:38 |
196 | Spring Boot - Spring MVC Validation - Validate a Number Range - Overview | 02:37 |
197 | Spring Boot - Spring MVC Validation - Validate a Number Range - Coding | 06:58 |
198 | Spring Boot - Spring MVC Validation - Applying Regular Expressions - Overview | 02:21 |
199 | Spring Boot - Spring MVC Validation - Applying Regular Expressions - Coding | 06:38 |
200 | Spring Boot - Spring MVC Validation - Make Integer Fields Required | 05:34 |
201 | Spring Boot - Spring MVC Validation - Strings for Int Fields and Custom Messages | 04:48 |
202 | Spring Boot - Spring MVC Validation - Debugging Tips for Custom Error Names | 05:05 |
203 | Spring Boot - Spring MVC Validation - Custom Validation - Overview - Part 1 | 04:02 |
204 | Spring Boot - Spring MVC Validation - Custom Validation - Overview - Part 2 | 05:54 |
205 | Spring Boot - Spring MVC Validation - Custom Validation - Coding - Part 1 | 03:22 |
206 | Spring Boot - Spring MVC Validation - Custom Validation - Coding - Part 2 | 04:15 |
207 | Spring Boot - Spring MVC Validation - Custom Validation - Coding - Part 3 | 05:29 |
208 | Spring Boot - Spring MVC Validation - Custom Validation - Coding - Part 4 | 04:24 |
209 | Spring Boot - Spring MVC Validation - Custom Validation - Coding - Part 5 | 05:40 |
210 | CRUD Database Project - Overview | 02:25 |
211 | CRUD Database Project - Set up | 04:32 |
212 | CRUD Database Project - Get Employees - Coding - Part 1 | 05:24 |
213 | CRUD Database Project - Get Employees - Coding - Part 2 | 03:36 |
214 | CRUD Database Project - Get Employees - Coding - Part 3 | 06:40 |
215 | CRUD Database Project - Get Employees - Coding - Part 4 | 04:46 |
216 | CRUD Database Project - Add Employees - Overview | 08:28 |
217 | CRUD Database Project - Add Employee - Coding - Part 1 | 05:21 |
218 | CRUD Database Project - Add Employee - Coding - Part 2 | 08:56 |
219 | CRUD Database Project - Add Employee - Coding - Part 3 | 08:36 |
220 | CRUD Database Project - Update Employee - Overview | 04:13 |
221 | CRUD Database Project - Update Employee - Coding | 08:54 |
222 | CRUD Database Project - Delete Employee - Overview | 02:51 |
223 | CRUD Database Project - Delete Employee - Coding | 04:49 |
224 | Spring MVC Security - Overview | 07:56 |
225 | Spring MVC Security - Demo | 02:46 |
226 | Spring MVC Security - Project Set Up - Part 1 | 06:35 |
227 | Spring MVC Security - Project Set Up - Part 2 | 06:23 |
228 | Spring MVC Security - Dev Testing with Private/Incognito Windows | 06:14 |
229 | Spring MVC Security - Basic Configuration - Overview | 03:24 |
230 | Spring MVC Security - Basic Configuration - Coding | 06:14 |
231 | Spring MVC Security - Custom Login Form - Overview - Part 1 | 04:47 |
232 | Spring MVC Security - Custom Login Form - Overview - Part 2 | 04:35 |
233 | Spring MVC Security - Custom Login Form - Coding - Part 1 | 04:40 |
234 | Spring MVC Security - Custom Login Form - Coding - Part 2 | 08:16 |
235 | Spring MVC Security - Custom Login Form - Coding - Part 3 | 02:16 |
236 | Spring MVC Security - Login Form Error Message - Overview | 02:41 |
237 | Spring MVC Security - Login Form Error Message - Coding | 06:06 |
238 | Spring MVC Security - Custom Login Form with Bootstrap - Overview | 01:54 |
239 | Spring MVC Security - Custom Login Form with Bootstrap - Coding | 07:10 |
240 | Spring MVC Security - Logout - Overview | 02:53 |
241 | Spring MVC Security - Logout - Coding | 07:01 |
242 | Spring MVC Security - Display User ID and Roles - Overview | 01:32 |
243 | Spring MVC Security - Display User ID and Roles - Coding | 05:51 |
244 | Spring MVC Security - Restrict URLs Based on Roles - Overview | 04:38 |
245 | Spring MVC Security - Restrict URLs Based on Roles - Coding - Part 1 | 03:32 |
246 | Spring MVC Security - Restrict URLs Based on Roles - Coding - Part 2 | 07:22 |
247 | Spring MVC Security - Restrict URLs Based on Roles - Coding - Part 3 | 06:11 |
248 | Spring MVC Security - Restrict URLs Based on Roles - Coding - Part 4 | 07:38 |
249 | Spring MVC Security - Custom Access Denied Page - Overview | 01:46 |
250 | Spring MVC Security - Custom Access Denied Page - Coding - Part 1 | 03:19 |
251 | Spring MVC Security - Custom Access Denied Page - Coding - Part 2 | 03:29 |
252 | Spring MVC Security - Display Content Based on Roles - Overview | 02:24 |
253 | Spring MVC Security - Display Content Based on Roles - Coding - Part 1 | 04:18 |
254 | Spring MVC Security - Display Content Based on Roles - Coding - Part 2 | 04:50 |
255 | Spring MVC Security - JDBC Authentication - Plain Text - Overview | 06:58 |
256 | Spring MVC Security - JDBC Authentication - Plain Text - Coding - Part 1 | 06:57 |
257 | Spring MVC Security - JDBC Authentication - Plain Text - Coding - Part 2 | 04:38 |
258 | Spring MVC Security - JDBC Authentication - Plain Text - Coding - Part 3 | 03:14 |
259 | Spring MVC Security - JDBC Authentication - Plain Text - Coding - Part 4 | 06:06 |
260 | Spring MVC Security - JDBC Authentication - BCrypt Encryption - Overview Part 1 | 05:05 |
261 | Spring MVC Security - JDBC Authentication - BCrypt Encryption - Overview Part 2 | 04:00 |
262 | Spring MVC Security - JDBC Authentication - BCrypt Encryption - Coding | 03:49 |
263 | Spring MVC Security - JDBC Authentication - Custom Tables - Overview | 03:38 |
264 | Spring MVC Security - JDBC Authentication - Custom Tables - Coding - Part 1 | 04:06 |
265 | Spring MVC Security - JDBC Authentication - Custom Tables - Coding - Part 2 | 05:39 |
266 | JPA / Hibernate Advanced Mappings Overview - Part 1 | 02:58 |
267 | JPA / Hibernate Advanced Mappings Overview - Part 2 | 05:16 |
268 | @OneToOne Mapping Overview - Part 1 | 05:10 |
269 | @OneToOne Mapping Overview - Part 2 | 06:21 |
270 | @OneToOne Mapping Overview - Part 3 | 05:19 |
271 | @OneToOne Mapping - Database Setup | 09:13 |
272 | @OneToOne Mapping - Set up Spring Boot Project - Part 1 | 04:55 |
273 | @OneToOne Mapping - Set up Spring Boot Project - Part 2 | 05:10 |
274 | @OneToOneMapping - Coding - Create InstructorDetail entity | 06:56 |
275 | @OneToOneMapping - Coding - Create Instructor entity | 07:39 |
276 | @OneToOneMapping - Coding - Create the DAO | 04:46 |
277 | @OneToOneMapping - Coding - Develop the Main App | 05:56 |
278 | @OneToOneMapping - Coding - Run the Main App | 05:27 |
279 | @OneToOneMapping - Find Instructor by ID | 06:42 |
280 | @OneToOneMapping - Delete Instructor by ID | 05:52 |
281 | @OneToOneMapping - Bi-Directional - Overview | 06:54 |
282 | @OneToOneMapping - Bi-Directional - Coding - Part 1 | 07:08 |
283 | @OneToOneMapping - Bi-Directional - Coding - Part 2 | 07:44 |
284 | @OneToOneMapping - Bi-Directional - Cascade Delete - Coding | 06:14 |
285 | @OneToOneMapping - Bi-Directional - Only Delete Instructor Details - Coding | 08:01 |
286 | @OneToMany - Overview - Part 1 | 04:14 |
287 | @OneToMany - Overview - Part 2 | 02:57 |
288 | @OneToMany - Coding - Set Up Database Tables | 04:50 |
289 | @OneToMany - Coding - Create Course entity | 06:00 |
290 | @OneToMany - Coding - Annotate Course entity | 03:36 |
291 | @OneToMany - Coding - Annotate Instructor entity | 05:27 |
292 | @OneToMany - Coding - Create Main App | 03:40 |
293 | @OneToMany - Coding - Finish Main App and Run It! | 07:38 |
294 | @OneToMany - Fetch Types: Eager vs Lazy - Overview - Part 1 | 03:18 |
295 | @OneToMany - Fetch Types: Eager vs Lazy - Overview - Part 2 | 04:26 |
296 | @OneToMany: Fetch Types - Eager vs Lazy - Coding | 06:05 |
297 | @OneToMany: Lazy Find Courses - Overview | 03:13 |
298 | @OneToMany: Lazy Find Courses - Coding - Part 1 | 05:22 |
299 | @OneToMany: Lazy Find Courses - Coding - Part 2 | 06:16 |
300 | @OneToMany - JOIN FETCH Courses - Overview | 03:07 |
301 | @OneToMany - JOIN FETCH Courses - Coding - Part 1 | 04:18 |
302 | @OneToMany - JOIN FETCH Courses - Coding - Part 2 | 06:22 |
303 | @OneToMany - Update Instructor | 08:26 |
304 | @OneToMany - Update Course | 08:32 |
305 | @OneToMany - Delete Instructor | 09:46 |
306 | @OneToMany - Delete Course | 06:21 |
307 | @OneToMany - Uni-Directional - Overview | 06:46 |
308 | @OneToMany - Uni-Directional - Coding - Database Set Up | 03:16 |
309 | @OneToMany - Uni-Directional - Coding - Create Review Entity | 08:15 |
310 | @OneToMany - Uni-Directional - Coding - Refactor Course Entity | 04:08 |
311 | @OneToMany - Uni-Directional - Coding - Update DAO and Main App | 08:13 |
312 | @OneToMany - Uni-Directional - Coding - Retrieve Course and Reviews | 06:37 |
313 | @OneToMany - Uni-Directional - Coding - Delete Course and Reviews | 03:01 |
314 | @ManyToMany - Overview - Part 1 | 04:56 |
315 | @ManyToMany - Overview - Part 2 | 05:41 |
316 | @ManyToMany - Coding - Set up Database Tables | 05:03 |
317 | @ManyToMany - Coding - Set up Spring Boot Project | 02:36 |
318 | @ManyToMany - Coding - Create Student entity | 04:43 |
319 | @ManyToMany - Coding - Map Course to Student | 06:47 |
320 | @ManyToMany - Coding - Map Student to Course | 05:39 |
321 | @ManyToMany - Coding - Create Course and Students | 07:33 |
322 | @ManyToMany - Coding - Find Course and Students | 05:39 |
323 | @ManyToMany - Coding - Find Student and Courses | 06:46 |
324 | @ManyToMany - Coding - Add More Courses to Students | 08:36 |
325 | @ManyToMany - Coding - Delete Course | 03:41 |
326 | @ManyToMany - Coding - Delete Student | 05:31 |
327 | AOP - The Business Problem | 04:22 |
328 | AOP Solution and AOP Use Cases | 04:11 |
329 | AOP Concepts and Terminology | 05:13 |
330 | Comparing Spring AOP and AspectJ - Part 1 | 03:29 |
331 | Comparing Spring AOP and AspectJ - Part 2 | 02:50 |
332 | AOP - @Before Advice - Overview - Part 1 | 03:48 |
333 | AOP - @Before Advice - Overview - Part 2 | 05:15 |
334 | AOP - @Before Advice - Coding - AOP Project Set Up | 07:50 |
335 | AOP - @Before Advice - Coding - Create Target Object - AccountDAO | 06:23 |
336 | AOP - @Before Advice - Coding - Create Aspect | 05:07 |
337 | AOP - @Before Advice - Test the AOP Aspect | 04:02 |
338 | AOP - Pointcut Expressions - Overview | 06:46 |
339 | AOP - Pointcut Expressions - Coding - Match any addAccount Method - Part 1 | 04:13 |
340 | AOP - Pointcut Expressions - Coding - Match any addAccount Method - Part 2 | 05:03 |
341 | AOP - Pointcut Expressions - Coding - Match only AccountDAO addAccount | 03:32 |
342 | AOP - Pointcut Expressions - Coding - Match any add* Method | 02:33 |
343 | AOP - Pointcut Expressions - Coding - Match any Return Type | 04:09 |
344 | AOP - Pointcut Expressions - Overview - Match on Method Parameters | 04:18 |
345 | AOP - Pointcut Expressions - Coding - Match Method Parameter Types | 05:15 |
346 | AOP - Pointcut Expressions - Coding - Match Method with Account and more Params | 03:34 |
347 | AOP - Pointcut Expressions - Coding - Match Method with Any Params | 05:05 |
348 | AOP - Pointcut Expressions - Coding - Match Any Method in a Package | 06:17 |
349 | AOP: Pointcut Declarations - Overview | 04:05 |
350 | AOP: Pointcut Declarations - Coding - Part 1 | 05:38 |
351 | AOP: Pointcut Declarations - Coding - Part 2 | 03:13 |
352 | AOP: Combining Pointcuts - Overview | 04:41 |
353 | AOP: Combining Pointcuts - Coding - Part 1 | 08:59 |
354 | AOP: Combining Pointcuts - Coding - Part 2 | 08:33 |
355 | AOP: Ordering Aspects - Overview | 06:50 |
356 | AOP: Ordering Aspects - Coding - Part 1 | 05:13 |
357 | AOP: Ordering Aspects - Coding - Part 2 | 05:15 |
358 | AOP: Ordering Aspects - Coding - Part 3 | 06:47 |
359 | AOP: Read Method Arguments with JoinPoints - Overview | 02:41 |
360 | AOP: Read Method Arguments with JoinPoints - Coding - Part 1 | 04:30 |
361 | AOP: Read Method Arguments with JoinPoints - Coding - Part 2 | 06:26 |
362 | AOP: Progress Check | 00:45 |
363 | AOP: @AfterReturning Advice - Overview | 06:18 |
364 | AOP: @AfterReturning Advice - Coding - Part 1 | 07:31 |
365 | AOP: @AfterReturning Advice - Coding - Part 2 | 04:04 |
366 | AOP: @AfterReturning Advice - Coding - Part 3 | 08:33 |
367 | AOP: @AfterReturning Advice - Modify Return Value - Overview | 04:15 |
368 | AOP: @AfterReturning Advice - Modify Return Value - Coding | 05:51 |
369 | AOP: @AfterThrowing Advice - Overview | 06:52 |
370 | AOP: @AfterThrowing Advice - Coding - Part 1 | 08:24 |
371 | AOP: @AfterThrowing Advice - Coding - Part 2 | 05:32 |
372 | AOP: @After Advice - Overview | 03:21 |
373 | AOP: @After Advice - Coding | 05:57 |
374 | AOP: @Around Advice - Overview | 05:34 |
375 | AOP: @Around Advice - Coding - Part 1 | 04:45 |
376 | AOP: @Around Advice - Coding - Part 2 | 06:05 |
377 | AOP: @Around Advice - Coding - Part 3 | 03:25 |
378 | @Around Advice - Handle Exception - Overview | 04:42 |
379 | @Around Advice - Handle Exception - Coding - Part 1 | 05:34 |
380 | @Around Advice - Handle Exception - Coding - Part 2 | 04:12 |
381 | AOP: @Around Advice - Rethrow Exception | 05:55 |
382 | AOP: Integrating AOP with Spring MVC CRUD App - Overview | 03:10 |
383 | AOP: Integrating AOP with Spring MVC CRUD App - Coding - Part 1 | 04:54 |
384 | AOP: Integrating AOP with Spring MVC CRUD App - Coding - Part 2 | 03:28 |
385 | AOP: Integrating AOP with Spring MVC CRUD App - Coding - Part 3 | 07:02 |
386 | AOP: Integrating AOP with Spring MVC CRUD App - Coding - Part 4 | 04:20 |
387 | AOP: Integrating AOP with Spring MVC CRUD App - Coding - Part 5 | 05:14 |
388 | Thank You and Please Leave a Rating for the course | 01:32 |
Similar courses to [NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners
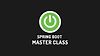
Spring Boot Master Class
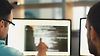
Go Full Stack with Spring Boot and React
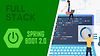
Full Stack Spring Boot & React
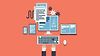
Hibernate and Java Persistence API (JPA) Fundamentals
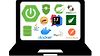
Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)
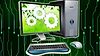
Java Spring Tutorial Masterclass - Learn Spring Framework 5
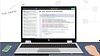
Build Your REST API with Spring 5
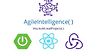
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Redux
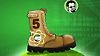
Spring Framework 5: Beginner to Guru
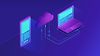