Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)
In this course, you will learn Spring Framework Core 6, Spring Boot 3, REST API, Spring MVC, WebFlux, Spring Security, Spring Data JPA, Docker, Thymeleaf, IntelliJ IDEA, Maven, and Building Projects
No Spring framework experience is needed, I will teach you all the Spring framework core features so that you will understand Spring Boot in-depth and how it works behind the scenes.
More
What is Spring Boot?
Spring Boot is basically an extension of the Spring framework which eliminated the boilerplate configurations required for setting up a Spring application.
Spring Boot is an opinionated framework that helps developers build Spring-based applications quickly and easily. The main goal of Spring Boot is to quickly create Spring-based applications without requiring developers to write the same boilerplate configuration again and again.
What is Spring MVC?
Spring MVC is a popular module in Spring Framework and it is used to develop web applications as well as RESTful web services.
Spring MVC is called a web framework because it provides all the required components to develop a complete web application.
- The Spring MVC framework provides Model-View-Controller (MVC) architecture and ready components that can be used to develop flexible and loosely coupled web applications
What is Thymeleaf?
Thymeleaf is a modern server-side Java template engine for both web and standalone environments, capable of processing HTML, XML, JavaScript, CSS, and even plain text.
The main goal of Thymeleaf is to provide an elegant and highly-maintainable way of creating templates.
It's commonly used to generate HTML views for web applications.
Thymeleaf is a very popular choice for building UI so we will be using Thymeleaf to build the view layer in the Spring MVC web application (Blog App).
Watch Online Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)
# | Title | Duration |
---|---|---|
1 | Course Introduction | 04:27 |
2 | Quick Overview of Spring Framework | 01:31 |
3 | Create and Setup Spring Application in IntelliJ IDEA | 02:15 |
4 | Understanding Tightly Coupling and Loose Coupling | 11:48 |
5 | Spring IOC Container - Theory | 05:46 |
6 | Steps for Java Based Configuration | 06:33 |
7 | Spring IOC Container - Example | 13:09 |
8 | Summary - How Spring IOC Container Works | 02:51 |
9 | Steps for Annotation Based Configuration | 08:07 |
10 | Annotation Based Configuration Example | 10:40 |
11 | @Qualifier and @Primary Annotations | 05:45 |
12 | Stereotype Annotations - @Controller, @Service and @Repository | 09:09 |
13 | Difference Between Java Based and Annotation Based Configuration | 05:41 |
14 | What is Dependency Injection | 05:08 |
15 | Example - Without Dependency Injection | 08:53 |
16 | Example - Dependency Injection using Spring Framework | 07:34 |
17 | Constructor Based Dependency Injection | 08:10 |
18 | Setter Based Dependency Injection | 06:18 |
19 | When to Use Construction Injection and Setter Injection? | 06:00 |
20 | Why Constructor Injection is Recommended? | 04:16 |
21 | Field Based Dependency Injection | 06:59 |
22 | @Bean Annotation In-Depth | 11:17 |
23 | @Bean Annotation - initMethod and destroyMethod Attributes | 05:26 |
24 | Assignment - Sending an Email | 02:51 |
25 | Assignment Solution 1 - Using Annotation Based Configuration | 10:19 |
26 | Assignment Solution 2 - Using Java Based Configuration | 04:11 |
27 | What is Spring Boot? | 07:10 |
28 | Spring Boot Features | 08:03 |
29 | Creating Your First Spring Boot Project | 06:46 |
30 | Understanding Spring Boot Project Structure | 06:56 |
31 | Spring Boot Starters Feature | 10:24 |
32 | Spring Boot Starter Parent | 07:54 |
33 | Spring Boot Auto Configuration Feature - Theory | 04:11 |
34 | Spring Boot Auto Configuration Feature - In an Action | 10:14 |
35 | Understanding @SpringBootApplication Annotation | 10:14 |
36 | How Spring Boot Works Internally | 08:16 |
37 | Section Introduction | 01:49 |
38 | Create Spring Boot Project using Spring Initializr and Import in IntelliJ IDE | 05:53 |
39 | Create Simple Spring Boot REST API | 07:22 |
40 | Spring Boot REST API That Returns Java Bean as JSON | 05:40 |
41 | Create Spring Boot REST API returns List in JSON Format | 03:53 |
42 | Spring Boot REST API with Path Variable - @PathVariable | 07:59 |
43 | Spring Boot REST API with Request Param - @RequestParam | 06:42 |
44 | Spring Boot POST REST API - @PostMapping and @RequestBody | 09:22 |
45 | Spring Boot PUT REST API - @PutMapping and @RequestBody | 09:06 |
46 | Spring Boot DELETE REST API - @DeleteMapping | 04:48 |
47 | Using Spring ResponseEntity to Manipulate the HTTP Response | 09:40 |
48 | Define Base URL for REST API’s in Spring MVC Controller - @RequestMapping | 05:32 |
49 | Section Introduction | 01:50 |
50 | Create and Setup Spring Boot Project in IntelliJ | 06:01 |
51 | Configure MySQL Database in Spring Boot App | 05:31 |
52 | Create User JPA Entity | 06:52 |
53 | Create Spring Data JPA Repository - UserRepository | 06:21 |
54 | Build Create User REST API | 13:36 |
55 | Build Get User By ID REST API | 08:15 |
56 | Build Get All Users REST API | 05:29 |
57 | Build Update User REST API | 10:13 |
58 | Build Delete User REST API | 05:05 |
59 | Understanding DTO Pattern | 04:08 |
60 | How to Use DTO in Spring Boot Project | 05:18 |
61 | Refactor Create User REST API to use DTO | 10:16 |
62 | Create and Use UserMapper Class | 05:21 |
63 | Refactor Get User By Id REST API to use DTO | 03:43 |
64 | Refactor Get All Users REST API to use DTO | 03:45 |
65 | Refactor Update User REST API to use DTO | 04:31 |
66 | ModelMapper and MapStruct Libraries Overview | 04:31 |
67 | Using ModelMapper Library to Map Entity to DTO and Vice Versa | 13:25 |
68 | Using MapStruct Library: Step 1 - Add Maven Dependencies | 04:34 |
69 | Using MapStruct Library: Step 2 - Create Mapper | 05:08 |
70 | Using MapStruct Library: Step 3 - Using Mapper and Test CRUD REST API's | 07:11 |
71 | Spring Boot REST API Exception Handling Overview 1 | 04:25 |
72 | Spring Boot REST API Exception Handling Overview 2 | 02:19 |
73 | How to Create and Use Custom Exception - ResourceNotFoundException | 13:08 |
74 | Handing Specific Custom Exception - ResourceNotFoundException | 10:35 |
75 | Handing Specific Custom Exception - EmailAlreadyExistsException | 08:39 |
76 | Spring Boot REST API Global Exception Handling | 03:58 |
77 | Validation with Spring Boot - Overview | 04:13 |
78 | Validate Create and Update User REST API Requests | 06:34 |
79 | Customizing Validation Error Response | 11:46 |
80 | Actuator Section Overview | 01:21 |
81 | Adding Actuator to Spring Boot App | 04:22 |
82 | The /info Endpoint | 03:31 |
83 | The /health Endpoint | 02:29 |
84 | The /beans Endpoint | 03:16 |
85 | The /conditions Endpoint | 02:39 |
86 | The /mappings Endpoint | 03:38 |
87 | The /configprops Endpoint | 02:35 |
88 | The /metrics Endpoint | 05:12 |
89 | The /env & /threaddump Endpoints | 03:47 |
90 | The /loggers Endpoint | 04:40 |
91 | The /shutdown Endpoint | 03:04 |
92 | Section Overview and Demo | 04:01 |
93 | Generate REST API Documentation using SpringDoc OpenAPI | 08:28 |
94 | Define General API Information using Annotations | 06:18 |
95 | Customizing Swagger API Documentation with Annotations | 11:53 |
96 | Todo Management Project - Understanding Requirements | 02:09 |
97 | Create and Setup Spring Boot Project in IntelliJ IDEA | 05:10 |
98 | Configure MySQL Database in Spring Boot Application | 05:28 |
99 | Create Todo JPA Entity | 05:55 |
100 | Create TodoRepository and TodoDto | 08:00 |
101 | Build Add Todo REST API | 13:41 |
102 | Using ModelMapper Library | 07:07 |
103 | Build Get Todo REST API | 10:40 |
104 | Build Get All Todos REST API | 06:50 |
105 | Build Update Todo REST API | 11:00 |
106 | Build Delete Todo REST API | 06:24 |
107 | Build Complete Todo API | 08:16 |
108 | Build In Complete Todo REST API | 06:34 |
109 | Section Overview | 01:08 |
110 | Authentication and Authorization | 04:11 |
111 | Adding Spring Security to Spring Boot Project (Todo Management) | 04:13 |
112 | Spring Boot Auto Configuration for Spring Security | 09:22 |
113 | Customizing the Default User | 03:59 |
114 | Configure and Understand Basic Authentication | 09:07 |
115 | In-Memory Authentication | 06:36 |
116 | Understanding Role-Based Autherization | 15:30 |
117 | Method Level Security | 11:42 |
118 | Creating User and Role JPA Entities | 10:48 |
119 | Creating UserRepository and RoleRepository | 04:29 |
120 | Creating CustomUserDetailsService Class Implements UserDetailsService | 08:05 |
121 | How Database Authentication Works in Spring Security | 09:11 |
122 | Spring Security Database Authentication | 12:49 |
123 | Module Introduction - What you will learn in this section? | 01:14 |
124 | Transaction Management with Spring Data JPA | 07:01 |
125 | Use Case - Placing Order on eCommerce Shopping Website | 03:18 |
126 | Create and Setup Spring Boot Project in IntelliJ | 06:44 |
127 | Configure MySQL Database | 05:20 |
128 | Create Order and Payment JPA Entities | 08:11 |
129 | Create OrderRepository and PaymentRepository Interfaces | 02:28 |
130 | Create OrderRequest and OrderResponse DTO Classes | 02:28 |
131 | Create Service Layer | 09:35 |
132 | Create Place Order REST API and Test using Postman | 06:37 |
133 | Handle Transaction using Spring @Transactional Annotation | 06:33 |
134 | Create Spring Boot Project | 06:12 |
135 | Configure MySQL Database | 05:21 |
136 | Create Product JPA Entity | 04:45 |
137 | Create ProductRepository Interface | 02:31 |
138 | Create JPQL and Native SQL Queries to Search Products | 05:25 |
139 | Create Service Layer | 03:03 |
140 | Build Search REST API and Test using Postman | 10:10 |
141 | What is ORM? | 03:25 |
142 | What is JPA? | 05:39 |
143 | What is Hibernate? | 04:04 |
144 | JPA vs Hibernate | 02:47 |
145 | What is Spring Data JPA? | 03:46 |
146 | Hibernate vs Spring Data JPA | 04:47 |
147 | Basic Flow of Spring Data JPA | 03:49 |
148 | Create and Setup Spring Boot Project in IntelliJ IDEA | 06:35 |
149 | Understanding Spring Boot Starter Data JPA Dependency | 04:18 |
150 | Connect Spring Boot Project with MySQL Database | 11:17 |
151 | Create Product Entity with @Entity and @Id | 08:04 |
152 | Using JPA Annotations - @Table, @Column, @GeneratedValue and @UniqueConstraint | 12:00 |
153 | Primary key generation strategies - AUTO, IDENTITY, SEQUENCE and TABLE | 14:24 |
154 | Adding Hibernate annotations - @CreationTimestampВ andВ @UpdateTimestamp | 02:36 |
155 | Using Lombok Library to Reduce Boilerplate Code | 04:46 |
156 | Understanding Spring Data JPA Repository Interfaces and it’s Hierarchy | 08:16 |
157 | Decompile Spring Data JPA Library to Understand Repository Interfaces | 07:32 |
158 | Steps to Create Spring Data JPA Repository - ProductRepository | 06:03 |
159 | Overview of Spring Data JPA Repository Methods | 03:51 |
160 | save() - Save an Entity to the Database Table | 12:16 |
161 | save() - Update an Entity to the Database Table | 06:24 |
162 | findById() - Retrieve a Single Entity from the Database | 03:55 |
163 | saveAll() - Save Multiple Entities to the Database Table | 06:19 |
164 | findAll() - Retrieve All the Entities From the Database Table | 04:15 |
165 | deleteById() - Delete a Single Entity from the Database | 03:44 |
166 | delete() - Delete an Entity From the Database Table | 07:06 |
167 | deleteAll() - Delete All the Entities From the Database Table | 10:01 |
168 | count() - Get the Number of Records in the Database Table | 03:32 |
169 | existsById() - Check if Entity Exists With Given ID in the Database Table | 05:46 |
170 | Overview of Creating Query Methods From Method Names Strategy | 02:44 |
171 | How Query Generation From Method Names Works Behind the Scene | 05:09 |
172 | Understanding Rules and Supported Keywords to Create Query Methods | 12:44 |
173 | Spring Data JPA Query Method - Find by Single Field Name | 13:14 |
174 | Spring Data JPA Query Method - Find by Multiple Field Names | 12:40 |
175 | Spring Data JPA Query Method - Find by Distinct | 04:42 |
176 | Spring Data JPA Query Method - Find by GreaterThan | 05:49 |
177 | Spring Data JPA Query Method - Find by LessThan | 05:02 |
178 | Spring Data JPA Query Method - Find by Containing | 05:12 |
179 | Spring Data JPA Query Method - Find by Like | 05:44 |
180 | Spring Data JPA Query Method - Find by Between (Price Range Example) | 06:17 |
181 | Spring Data JPA Query Method - Find by Between (Date Range Example) | 08:49 |
182 | Spring Data JPA Query Method - Find by In | 05:28 |
183 | Spring Data JPA Query Method - Limiting Query Results | 07:03 |
184 | What is Spring MVC? | 03:33 |
185 | What is DispatcherServlet? | 03:23 |
186 | Spring MVC Components | 08:31 |
187 | How Spring MVC Works Internally | 03:18 |
188 | Spring Boot Web MVC Architecture (Three - Layer Architecture) | 01:48 |
189 | What is Thymeleaf? | 04:05 |
190 | How Thymeleaf Engine Works? | 02:34 |
191 | Create Spring Boot Project and Integrate Thymeleaf | 05:34 |
192 | Spring Boot Auto Configuration for Thymeleaf | 03:48 |
193 | Thymeleaf Hello World Example | 08:02 |
194 | Thymeleaf Variable Expressions | 12:03 |
195 | Thymeleaf Selection Expressions | 08:20 |
196 | Thymeleaf Message Expressions | 06:58 |
197 | Thymeleaf Link (URL) Expressions | 10:21 |
198 | Thymeleaf Fragment Expressions | 11:45 |
199 | Thymeleaf Basic Attribute - th:text | 02:55 |
200 | Thymeleaf Loop or Iteration - th:each | 11:49 |
201 | Thymeleaf Looping or Iteration - th:each Attribute Status Variable | 05:21 |
202 | Thymeleaf Attribute - th:if and th:unless | 09:56 |
203 | Thymeleaf Attribute - th:switch and th:case | 08:37 |
204 | Form Handling in Thymeleaf Overview | 03:49 |
205 | Form Handling in Thymeleaf - Create Handler Method to Return Register Page | 06:35 |
206 | Form Handling in Thymeleaf Template - Design User Registration Form | 15:48 |
207 | Form Handling in Thymeleaf Template - Display User Registration Form Data | 08:37 |
208 | Create and Setup Spring Boot Project in IntelliJ | 06:22 |
209 | Understanding spring-boot-starter-thymeleaf Dependency | 04:46 |
210 | Configure MySQL Database in Spring Boot App | 06:42 |
211 | Create Student JPA Entity | 06:23 |
212 | Create StudentRepository Interface | 06:45 |
213 | Create StudentDto and StudentMapper | 05:49 |
214 | List Students Feature Backend | 10:09 |
215 | List Students Feature Frontend | 15:43 |
216 | Create Student Feature - Create Handler Method for Student Form | 04:15 |
217 | Create Student Feature - Create Student Form Handling | 12:07 |
218 | Create Student Feature - Create Handler Method for Save Student | 07:48 |
219 | Create Student Feature - Create Student Form Validation | 10:34 |
220 | Update Student Feature Backend | 08:37 |
221 | Update Student Feature Frontend | 11:44 |
222 | Delete Student Feature Implementation | 07:14 |
223 | View Student Feature Backend | 05:23 |
224 | View Student Feature Frontend | 08:23 |
225 | Create and Setup Spring Boot Project in IntelliJ | 05:42 |
226 | Understanding spring-boot-starter-thymeleaf Dependency | 04:46 |
227 | Configure MySQL Database in Spring Boot App | 04:46 |
228 | Create User and Role Entities (Many to Many Mapping) | 14:52 |
229 | Create UserRepository and RoleRepository | 05:05 |
230 | Create Thymeleaf Template for Home Page | 07:32 |
231 | Create Handler Method to Handle Registration Form Request | 05:20 |
232 | User Registration Form Handling | 14:47 |
233 | Create Handler Method to Save User Registered Data | 13:34 |
234 | Adding Validation to User Registration Form | 12:27 |
235 | Display List Registered Users - Backend | 06:05 |
236 | Display List Registered Users - Frontend | 08:18 |
237 | Add Spring Security & Use Spring Security’s Default Login and Logout Features | 09:19 |
238 | Create Custom Login Form and Configure Spring Security | 16:28 |
239 | Logout Feature Implementation | 06:18 |
240 | Configure URL’s in Spring Security | 04:35 |
241 | Database Authentication Implementation | 12:57 |
242 | Introduction to Reactive Programming | 09:23 |
243 | Reactive Stream Specifications | 04:31 |
244 | Reactive Stream Workflow | 04:03 |
245 | Understanding Project Reactor Mono and Flux | 13:08 |
246 | Spring WebFlux Overview | 04:02 |
247 | Spring Boot WebFlux Application Architecture | 02:26 |
248 | Deploy MongoDB in Docker Container | 06:04 |
249 | Create and Set up Spring Boot Project in IntelliJ IDE | 06:38 |
250 | Create Employee Entity | 04:48 |
251 | Create EmployeeRepository and Understanding Internals | 05:14 |
252 | Create EmployeeDto and EmployeeMapper | 04:04 |
253 | Build Reactive Add Employee REST API | 13:55 |
254 | Build Reactive Get Employee REST API | 06:35 |
255 | Build Reactive Get All Employees REST API | 05:52 |
256 | Build Reactive Update Employee REST API | 09:30 |
257 | Build Reactive Delete Employee REST API | 05:57 |
258 | Write a Integration Test for Add Employee REST API | 11:05 |
259 | Write a Integration Test for Get Employee REST API | 06:49 |
260 | Write a Integration Test for Get All Employees REST API | 06:14 |
261 | Refactor the Code to Use @BeforeEach Annotation | 04:03 |
262 | Write a Integration Test for Update Employee REST API | 07:30 |
263 | Write a Integration Test for Delete Employee REST API | 03:43 |
264 | General Docker Workflow | 03:34 |
265 | Create Spring Boot Project and Build Simple REST API | 05:27 |
266 | Create Dockerfile to Build Docker Image | 06:14 |
267 | Build Docker Image from Dockerfile | 06:09 |
268 | Run Docker Image in a Docker Container | 07:23 |
269 | Push Docker Image to DockerHub | 07:47 |
270 | Pulll Docker Image from DockerHub | 07:55 |
271 | Dockerizing Spring Boot MySQL Application Overview | 01:23 |
272 | Pull and Run MySQL Image in a Docker Container | 07:42 |
273 | Create a Dockerfile to Build the Image | 06:12 |
274 | Implement Profile and Build Docker image | 08:23 |
275 | Run Spring Boot App Docker Image in a Container and Test CRUD REST APIs | 08:10 |
276 | Docker Compose Overview | 04:02 |
277 | Configure and Run MySQL Image in a Container using Docker Compose | 08:27 |
278 | Configure and Run Spring Boot in a Container using Docker Compose | 05:35 |
279 | @Component Annotation | 09:07 |
280 | @Autowired Annotation | 05:06 |
281 | @Qualifier Annotation | 05:29 |
282 | @Primary Annotation | 03:25 |
283 | @Bean and @Configuration Annotations | 12:57 |
284 | @Controller, @Service and @Repository | 09:03 |
285 | @Lazy Annotation | 06:21 |
286 | @Scope Annotation | 10:40 |
287 | @Value Annotation | 09:48 |
288 | @PropertySource and PropertySources Annotations | 14:22 |
289 | @ConfigurationProperties Annotation | 16:33 |
290 | @Controller and @ResponseBody Annotations | 09:48 |
291 | @RestController Annotation | 04:53 |
292 | @RequestMapping Annotation | 08:35 |
293 | @GetMapping Annotation | 04:53 |
294 | @PostMapping and @RequestBody Annotations | 10:27 |
295 | @PutMapping Annotation | 07:32 |
296 | @DeleteMapping Annotation | 05:06 |
297 | @PathVariable Annotation | 09:29 |
298 | @RequestParam Annotation | 07:29 |
Similar courses to Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)
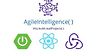
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Redux
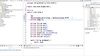
Building Your First App with Spring Boot and Angular
![[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners](https://cdn.courseflix.net/courses/100x56/new-spring-boot-3-spring-6-hibernate-for-beginners.jpg)
[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners
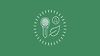
Learn Spring Security OAuth: The Master Class
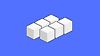
Microservices and Distributed Systems
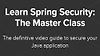
Learn Spring Security: The Master Class
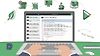
Learn Spring 5 and Spring Boot 2
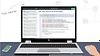
Build Your REST API with Spring 5
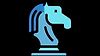
Master Spring 6 Spring Boot 3 REST JPA Hibernate
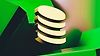