Spring Boot Microservices with Spring Cloud Beginner to Guru
Microservices are all the buzz in the industry right now. Building a microservice is not just a matter of using RESTFul APIs. Microservices are much MUCH more than that. In this course you will learn that Microservices are an architectural style. The allow companies to achieve massive scale while maintaining a high degree of flexibility. This course goes beyond simple RESTful APIs and explores microservices as an architectural style.
More
About the Course
Traditionally, large enterprise class applications were developed as large monolithic applications.
The Spring Framework started as an alternative to J2EE (now JEE) for building these large monolithic enterprise applications.
As the industry evolved to favor microservices over monoliths, the Spring Framework and Spring Boot evolved also.
The Spring Framework gives you a battle-tested enterprise grade framework for building applications.
Spring Boot and Spring Cloud are tools specifically for the development of microservices using the Spring Framework.
Microservices present a unique set of challenges over monoliths. Spring Boot and Spring Cloud help you overcome these changes.
What are these challenges that microservices have, which traditional monoliths do not?
Is it okay for microservices to share databases?
How do you coordinate business logic across a series of microservices?
How do you manage transactions across serval microservices with different databases?
To explain these questions, in this course you get to explore a traditional Spring Boot monolith type of application. (Along the style of Spring Pet Clinic).
We will then re-create this monolithic application using a set of microservices.
You get to see, step by step how to build 3 different microservices.
Microservices are much more than just having a set of RESTFul APIs. Microservices frequently use asynchronous messaging systems, which is fully covered.
While the Spring Framework and Spring Boot are the tools you use to construct Microservices, Spring Cloud provides the tools to deploy microservices.
You get to see the latest tools in Spring Cloud for deploying Spring Boot Microservices into a distributed (or cloud) environment.
In 2018, Netflix announced several core projects to Spring Cloud were entering maintenance mode. Meaning no new development would be done on these projects.
Thus, in December of 2018, the Spring Cloud Team recommended several key replacements:
Previous Replacement
Hystrix --> Resliience4J
Ribbon --> Spring Cloud Load Balancer
Zuul 1 --> Spring Cloud Gateway
Archaius 1 --> Spring Cloud Config
Therefore, recommend replacements are covered in this course.
Covered in this Course
In this course you will learn:
Develop RESTful Services using Spring MVC
Consume RESTFul Services with Spring RestTemplate
How to use Project Lombok
How to use MapStruct
Spring Data JPA with Hibernate
Configuration of Spring Boot for MySQL and H2
How to use and configure Jackson for processing JSON with Spring Boot
Documentation and testing of Spring Boot microservices using Spring RESTdocs
Standardizing dependencies using Apache Maven
Spring Application Events
Using JMS Messaging using Apache ActiveMQ Artemis
The Saga Pattern
How to use Spring State Machine for coordinating Sagas
Integration Testing using Spring Boot and JUnit 5
Using WireMock with JUnit 5
How to use Awaitily in your Integration Tests
The API Gateway pattern using Spring Cloud Gateway
Load Balanced Routes using Netflix Ribbon / Spring Cloud Loadbalancer
Service Registration using Netflix Eureka
Service Discovery with Netflix Eureka
Service Discovery using Spring Cloud OpenFeign
Circuit Breaker Pattern using Reslience4J and Hystrix / Spring Cloud OpenFeign
Manage configuration with Spring Cloud Config
Spring Boot Actuator
Use Docker to create images for your Spring Boot applications
And much much more!
- Must know Java and Spring Framework
- Basic Docker Skills
- SQL Skills - MySQL is used in course
- Linux skills helpful
Who this course is for:
- Java Developers who wish to learn how to develop Spring Boot Microservices with Spring Cloud
What you'll learn:
- Learn how to develop Microservices with Spring Boot
- Learn how to deploy and manage Spring Boot Microservices with Spring Cloud
- How to deconstruct a monolith into Spring Boot Microservices
- Best Practices for Developing RESTFul APIs with Spring MVC
- How to consume RESTFul APIs using Spring RestTemplate
- Using Java Bean Validation with Spring Boot
- How to use Project Lombok and MapStruct to reduce boiler plate code
- Generate API documentation with Spring REST Doc
- Configure Jackson for JSON processing with Spring Boot
- Use JMS for messaging between Spring Boot Microservices
- Use Spring State Machine to Apply the Saga Pattern with Microservices
Watch Online Spring Boot Microservices with Spring Cloud Beginner to Guru
# | Title | Duration |
---|---|---|
1 | Welcome to Spring Boot Microservices! | 05:39 |
2 | What you will build in the Spring Boot Microservices Course | 07:49 |
3 | Getting the Most out of this Course | 07:24 |
4 | Setting up your Development Environment | 03:22 |
5 | Is your IDE Free Like A Puppy? | 04:33 |
6 | Introduction to Intro to Microservices Section | 12:21 |
7 | The Traditional Monolith Application | 01:15 |
8 | What are Microservices? | 08:20 |
9 | What is the Cloud? | 07:18 |
10 | Adopting Microservices in Your Organization | 07:20 |
11 | Microservice Architecture and Design | 06:26 |
12 | 12 Factor Applications | 08:49 |
13 | Introduction to Microservices Conclusion | 10:00 |
14 | Introduction | 03:03 |
15 | HTTP Protocol | 01:27 |
16 | HTTP Request Methods | 07:18 |
17 | Beginners Guide to REST | 06:44 |
18 | Richardson Maturity Model | 06:07 |
19 | Spring Framework and RESTFul Services | 07:27 |
20 | Conclusion | 07:11 |
21 | Introduction | 01:43 |
22 | Introducing SFG Beer Works | 01:16 |
23 | HTTP GET with Spring MVC | 07:50 |
24 | Introduction to Postman | 22:06 |
25 | Axis TCPMon | 05:42 |
26 | Assignment - Create GET Endpoint with Spring MVC | 04:16 |
27 | Assignment Review | 01:11 |
28 | HTTP POST with SpringMVC | 08:47 |
29 | Spring Boot Development Tools | 05:38 |
30 | HTTP PUT with Spring MVC | 07:40 |
31 | Spring MVC @RequestBody Annotation | 04:22 |
32 | HTTP DELETE with Spring MVC | 08:15 |
33 | Assignment - HTTP Operations with Spring MVC | 03:58 |
34 | Assignment Review | 01:15 |
35 | API Versioning | 08:19 |
36 | API Versioning Example | 05:19 |
37 | API Versioning and Source Control | 09:03 |
38 | LC Beer Service - Initial Spring Boot Project Creation | 05:15 |
39 | LC Beer Service - Data Model | 09:13 |
40 | LC Beer Service - Beer Controller | 08:32 |
41 | LC Beer Service - JUnit 5 Controller Tests | 06:08 |
42 | Conclusion to Spring MVC Rest Services | 08:55 |
43 | Introduction to Spring Boot RestTemplate | 00:57 |
44 | HTTP GET with Spring RestTemplate | 02:07 |
45 | HTTP POST with Spring RestTemplate | 19:50 |
46 | HTTP PUT with Spring RestTemplate | 04:01 |
47 | HTTP DELETE with Spring RestTemplate | 04:01 |
48 | Assignment - Create Spring RestTemplate clients | 04:38 |
49 | Assignment Review | 01:40 |
50 | HTTP Clients | 06:08 |
51 | Apache HTTP Client Configuration | 09:24 |
52 | Apache Client Request Logging | 09:26 |
53 | Assignment - Externalize Properties | 05:15 |
54 | Assignment Review | 02:59 |
55 | LC - JPA Entities | 08:16 |
56 | LC - Spring Data JPA Repositories | 07:10 |
57 | LC - Bootstrap Data | 03:34 |
58 | Conclusion to Spring Boot RestTemplate | 07:19 |
59 | Introduction | 01:12 |
60 | Java Bean Validation | 01:39 |
61 | Bean Validation Implementation | 08:13 |
62 | Validation Error Handling | 07:23 |
63 | Spring Boot Method Validation | 04:39 |
64 | Assignment - Add Validation and Error Handling | 02:26 |
65 | Assignment Review | 01:30 |
66 | Spring MVC Controller Advice | 04:58 |
67 | LC - Bean Validation | 05:25 |
68 | LC - Validation and Error Handling | 03:52 |
69 | Conclusion to Spring MVC Validation | 05:29 |
70 | Introduction | 01:17 |
71 | Overview of Project Lombok | 02:33 |
72 | Project Lombok Configuration | 09:31 |
73 | Project Lombok Examples | 05:51 |
74 | Overview of MapStruct | 05:40 |
75 | MapStruct Configuration | 05:36 |
76 | Example of using Project Lombok and MapStruct | 12:14 |
77 | Assignment - Use MapStruct | 06:21 |
78 | Assignment Review | 01:19 |
79 | Date Conversion with MapStruct | 03:48 |
80 | LC - Implementing MapStruct | 04:29 |
81 | LC - Fixing Broken Tests | 06:32 |
82 | LC - Adding CI Builds with CircleCI | 03:21 |
83 | Conclusion | 06:44 |
84 | Introduction | 01:35 |
85 | Spring REST Docs Introduction | 02:12 |
86 | Project Code Review | 05:07 |
87 | Maven Configuration | 05:56 |
88 | Spring Mock MVC Configuration | 06:21 |
89 | Documenting Path Parameters | 04:33 |
90 | Documenting Query Parameters | 06:09 |
91 | Documenting Responses | 02:27 |
92 | Documenting Requests | 03:13 |
93 | Documenting Validation Constraints | 04:13 |
94 | URI Customization | 10:42 |
95 | Documentation Generation | 03:18 |
96 | Serving Docs with Spring Boot | 08:54 |
97 | Assignment - Add Spring REST Doc to Project | 04:04 |
98 | Assignment Review | 02:26 |
99 | Conclusion | 07:25 |
100 | Introduction | 01:54 |
101 | JSON with Spring Boot Overview | 02:08 |
102 | Overview of Jackson | 05:47 |
103 | Json Testing with Spring Boot | 09:08 |
104 | Jackson Property Naming Strategies | 06:21 |
105 | Assignment - Configure Property Naming Strategy | 05:07 |
106 | Assignment Review | 01:16 |
107 | Setting Property Names with Jackson | 04:15 |
108 | Using @JsonFormat with Jackson | 04:15 |
109 | Custom Serializer with Jackson | 04:13 |
110 | Custom Deserializer with Jackson | 05:33 |
111 | LC - Jackson Configuration | 06:43 |
112 | LC - Jackson JSON Creator | 02:28 |
113 | LC - Beer Service - Implement Controller Methods | 03:29 |
114 | LC - Beer Service - Adding standard UPCs | 12:21 |
115 | Conclusion | 07:40 |
116 | Introduction | 01:28 |
117 | SFG Brewery Monolith | 03:25 |
118 | Monolith Project Code Review | 05:14 |
119 | Deconstruction Strategies | 10:48 |
120 | Monolith Deconstruction Plan | 09:27 |
121 | Beer Service Code Review | 05:32 |
122 | Beer Order Service Code Review | 02:49 |
123 | Beer Inventory Service Code Review | 06:33 |
124 | Setting Default Ports for Services | 08:09 |
125 | Data Initialization | 05:21 |
126 | Enhance Get Beer with Inventory Information | 05:22 |
127 | Assignment - Add Show Inventory as Parameter | 14:32 |
128 | Assignment Review | 01:36 |
129 | Add Caching to Get Beer API | 07:34 |
130 | Assignment - Create Get Beer by UPC Endpoint | 11:00 |
131 | Assignment Review | 01:31 |
132 | Save UPC on Beer Order | 07:20 |
133 | Assignment - Enhance Order Response with Beer Information | 02:33 |
134 | Assignment Review | 01:46 |
135 | Deconstruction Next Steps | 08:30 |
136 | Conclusion | 05:11 |
137 | Introduction | 01:05 |
138 | Maven Bill of Materials | 03:16 |
139 | Maven BOM Creation | 04:34 |
140 | Maven BOM Setting Common Properties | 06:03 |
141 | Maven BOM Dependency Management | 06:57 |
142 | Maven BOM Common Dependencies | 05:15 |
143 | Maven BOM Common Build Plugins | 06:16 |
144 | Maven Enforcer Build Plugin | 03:46 |
145 | Beer Service Parent POM Configuration | 05:49 |
146 | Assignment - Update Services to use BOM | 11:32 |
147 | Assignment Review | 02:02 |
148 | Using Released BOMs | 06:42 |
149 | Assignment - Update Services to use Release BOM | 10:25 |
150 | Assignment Review | 01:55 |
151 | IntelliJ Workspace Tips and Tricks | 06:53 |
152 | Conclusion | 10:08 |
153 | Introduction | 01:44 |
154 | Overview of Datasource Configuration with Spring Boot | 02:35 |
155 | Configure H2 MySQL Compatibility Mode | 07:16 |
156 | MySQL Requirements and Setup | 06:17 |
157 | MySQL Beer Service Configuration | 06:17 |
158 | Configure Beer Service for Local MySQL | 06:08 |
159 | Correcting Hibernate Error with MySQL | 06:11 |
160 | Assignment - Configure Services for MySQL | 05:13 |
161 | Assignment Review | 01:29 |
162 | Assignment - Database Initialization | 10:05 |
163 | Assignment Review | 08:37 |
164 | Correction - Hibernate Dialog | 04:43 |
165 | Data Source Connection Pooling | 01:27 |
166 | HikariCP Configuration for MySQL | 10:12 |
167 | Conclusion | 08:23 |
168 | Introduction | 01:14 |
169 | Introduction to JMS | 01:29 |
170 | Initial Project and Maven Dependencies | 12:30 |
171 | Java Message Object | 07:31 |
172 | Embedded Server Configuration | 04:45 |
173 | Task Configuration | 04:59 |
174 | Message Converter Configuration | 03:15 |
175 | Sending JMS Messages | 05:50 |
176 | Receiving JMS Messages | 05:43 |
177 | Send and Receive of JMS Messages | 11:03 |
178 | Running Active MQ in Docker | 12:18 |
179 | Using Local ActiveMQ Broker with Spring Boot | 06:40 |
180 | JMS and Spring Message Data Types | 06:40 |
181 | Conclusion | 07:15 |
182 | Introduction | 01:50 |
183 | Assignment - Configure JMS for Services | 02:07 |
184 | Assignment Review | 01:48 |
185 | IntelliJ Tips and Tricks - Auto Import on Paste | 03:44 |
186 | Brewing Beer Process Overview | 03:25 |
187 | Create Beer Event Objects | 02:54 |
188 | Create Brewing Service | 05:23 |
189 | Fixing the Broken Build! | 10:24 |
190 | Create Brew Beer Listener | 03:18 |
191 | Jackson JMS Configuration | 06:59 |
192 | Tips and Tricks - Logging Level | 06:42 |
193 | Challenge - Create New Inventory Listener | 03:32 |
194 | Challenge Review | 02:37 |
195 | Conclusion | 13:38 |
196 | Introduction | 02:59 |
197 | Introduction to Spring State Machine | 01:26 |
198 | Credit Card Payment State Machine Overview | 08:07 |
199 | Spring Boot Project Creation and Dependencies | 03:44 |
200 | State Machine Enumerations | 07:27 |
201 | Spring Data JPA Configuration | 03:05 |
202 | State Configuration | 03:54 |
203 | Transition Configuration | 05:37 |
204 | Logging Configuration Using State Change Listeners | 08:26 |
205 | Payment Service Creation | 04:59 |
206 | Initializing State Machine from Database | 04:25 |
207 | Sending Events to the State Machine | 05:45 |
208 | State Change Interceptor | 04:54 |
209 | Progressing State from New to Pre-Auth | 06:13 |
210 | State Machine Actions | 10:20 |
211 | State Machine Guards | 07:25 |
212 | Microservice Design Pattern - Event Sourcing | 05:30 |
213 | Conclusion | 05:23 |
214 | Introduction | 01:07 |
215 | The Problem with Transactions | 01:53 |
216 | The Need for Sagas | 09:29 |
217 | Saga Coordination | 11:30 |
218 | Order Allocation Saga | 07:21 |
219 | Update Spring Boot in BOM | 05:47 |
220 | State Machine Enumerations | 07:24 |
221 | Configure State Machine States | 04:44 |
222 | Fix CI Build Failure | 03:45 |
223 | Refactor Model to Common Package | 05:29 |
224 | Beer Order Manager Service | 12:24 |
225 | Refactor Order States | 08:41 |
226 | Fix CI Build Failure | 03:26 |
227 | Initiate Allocate Order | 02:27 |
228 | Add Allocation Service | 10:35 |
229 | Fix Failed CI Build | 04:34 |
230 | Retrospective | 03:13 |
231 | Introduction to Integration Testing | 07:20 |
232 | Create Integration Test for New Order to Allocate | 01:07 |
233 | Testing with WireMock | 08:46 |
234 | WireMock for Beer Service | 17:55 |
235 | Validation JMS Test Component | 14:37 |
236 | Using Awaitility | 22:27 |
237 | Resolving Locking Failure | 06:03 |
238 | Refactor Beer Order Service to use Saga | 04:18 |
239 | Spring Cloud Contract - Interview with Marcin Grzejszczak | 06:38 |
240 | Introduction | 24:37 |
241 | Testing Failed Validation | 00:58 |
242 | Failed Validation Compensating Transaction | 07:40 |
243 | Cancel Order Requirements | 05:16 |
244 | Cancel Order Configuration | 03:28 |
245 | Cancel Order Compensating Transaction | 05:01 |
246 | Cancel Order Consumer | 05:49 |
247 | Refactoring for Persistence of Status Change | 03:28 |
248 | Introduction | 05:33 |
249 | The API Gateway Pattern | 00:50 |
250 | Developer Oriented API Gateways | 11:53 |
251 | Spring Cloud Gateway Service Creation | 09:12 |
252 | Spring Cloud Gateway Route Configuration | 06:06 |
253 | Review Running Services Locally | 11:33 |
254 | Beer Service Route Configuration | 06:10 |
255 | Inventory Service Route Configuration | 09:28 |
256 | Introduction | 05:14 |
257 | Introduction to Eureka | 00:57 |
258 | Eureka Service Creation | 05:35 |
259 | Eureka Client Configuration for Beer Service | 06:05 |
260 | Introduction | 05:03 |
261 | Open Feign Client | 01:25 |
262 | Configure Gateway for Service Discovery | 11:20 |
263 | Introduction | 08:45 |
264 | Circuit Breaker Pattern Overview | 01:46 |
265 | Resilience4j Failover for Spring Cloud Gateway | 03:16 |
266 | Using Hystrix Circuit Breaker with Feign Client | 09:20 |
267 | Using Resilience4j with Feign Client | 09:46 |
268 | Introduction | 01:52 |
269 | Overview of Spring Cloud Config | 01:21 |
270 | Create Spring Cloud Config Server | 03:14 |
271 | Spring Cloud Config Server Server Configuration | 04:56 |
272 | Server Side Application Configuration | 09:47 |
273 | Spring Cloud Client Configuration | 12:41 |
274 | Introduction | 14:07 |
275 | Introduction to Distributed Tracing | 01:30 |
276 | Zipkin Server | 10:11 |
277 | Setup Spring Cloud Sleuth | 02:32 |
278 | Logging Config for JSON | 07:48 |
279 | Refactor Zipkin Configuration | 06:43 |
280 | Introduction | 06:30 |
281 | Security 101 | 01:50 |
282 | Property Encryption / Decryption | 18:34 |
283 | Encrypt Beer Service Passwords | 08:22 |
284 | Secure Spring Cloud Config Server | 06:52 |
285 | Use Spring Security to Secure Eureka Server | 08:41 |
286 | Secure Inventory Service with Spring Security | 10:28 |
287 | Configure RESTTemplate for HTTP Basic Authentication | 04:13 |
288 | Configure Feign Client for HTTP Basic Authentication | 05:23 |
289 | Security Retrospective | 06:15 |
290 | Introduction | 06:30 |
291 | Requirements and Docker Hello World | 01:18 |
292 | Considerations for the JVM in a Docker Container | 03:09 |
293 | Overview of Building Docker Containers with Maven | 06:16 |
294 | Docker File Configuration | 07:03 |
295 | Troubleshoot Spring Boot Bug | 10:44 |
296 | Docker Image for Gateway Service | 09:23 |
297 | Push Images to Docker Hub | 08:32 |
298 | Docker Image Release Demo | 04:39 |
299 | Spring Boot Validation Update | 07:50 |
300 | Interview with James Labocki of RedHat | 03:39 |
301 | Introduction | 14:44 |
302 | Docker Compose for JMS Broker | 01:34 |
303 | Docker Compose for Zipkin | 07:55 |
304 | Docker Compose for Eureka Service & Spring Cloud Config | 02:49 |
305 | Docker Compose for Inventory Service | 05:24 |
306 | Configure API Gateway | 11:17 |
307 | Service Restart Policy | 07:14 |
308 | Introduction | 05:30 |
309 | Overview | 01:23 |
310 | Logging Configuration Update | 05:28 |
311 | Add Elasticsearch | 03:02 |
312 | Add Kibana | 02:58 |
313 | Add Filebeat | 01:41 |
314 | View Logs in Kibana | 06:12 |
315 | Introduction | 07:24 |
316 | Deployment Design | 03:37 |
317 | Provision Database Servers | 09:28 |
318 | Configure Database | 06:24 |
319 | Configure Java Truststore | 04:28 |
320 | Add Truststore file to Docker Image | 09:10 |
321 | Docker Image Release Process | 03:06 |
322 | Provision Service VMs | 06:42 |
323 | Configure JMS Server | 04:20 |
324 | Configure Elasticsearch Server | 03:13 |
325 | Configure Kibana Server | 02:35 |
326 | Configure Zipkin Server | 06:05 |
327 | Configure Eureka Server | 02:13 |
328 | Configure Spring Cloud Config Server | 02:55 |
329 | Spring Cloud Config Server IP Address Update | 08:36 |
330 | Provision Docker Swarm Cluster | 07:10 |
331 | Initialize Docker Swarm Cluster | 02:12 |
332 | Filebeat Swarm Configuration | 08:55 |
333 | Eureka Swarm Configuration | 05:23 |
334 | Spring Cloud Configuration | 09:23 |
335 | Digital Ocean Profile | 05:31 |
336 | Running Microservices with Docker Swarm | 03:33 |
337 | Tracing Requests for Troubleshooting | 04:39 |
338 | Zipkin Tracing | 05:52 |
339 | Tasting Room Service Challenge | 04:19 |
340 | Retrospective | 03:04 |
341 | unnamed 341 | 04:34 |
342 | unnamed 342 | 12:21 |
Similar courses to Spring Boot Microservices with Spring Cloud Beginner to Guru
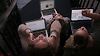
Angular 7 + Spring Boot and Cloud Microservices(Inc. Docker)
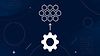
Event-Driven Microservices, CQRS, SAGA, Axon, Spring Boot
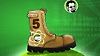
Spring Framework 5: Beginner to Guru
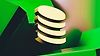
RESTful Web Services, Java, Spring Boot, Spring MVC and JPA
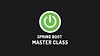
Spring Boot Master Class
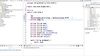
Building Your First App with Spring Boot and Angular
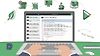
Learn Spring 5 and Spring Boot 2
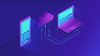