Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog App
11h 52m 40s
English
Paid
In this course, you will learn how to build a real-time complete blog application step by step using Spring Boot 3, Spring MVC, Thymeleaf, Spring Security 6, Spring Data JPA, and MySQL database.
In this course, we will be using Spring Boot 3, Spring Framework 6, Spring Security 6 (no deprecated APIs/classes), and Thymeleaf 3 - The latest versions as of now.
I have added a Thymeleaf crash course with 20+ lectures so if you are new to Thymeleaf then first watch the Thymeleaf crash course and then build a Blog application.
Note: This is the project development course so you need to have a basic understanding of Spring Boot, Spring Security, Spring Data JPA, and MySQL database
Watch Online Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog App
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Course Introduction - What We'll Build In This Course | 06:52 |
2 | Understanding Project High Level Requirements | 02:20 |
3 | What is Spring MVC? | 03:33 |
4 | What is DispatcherServlet? | 03:23 |
5 | Spring MVC Components | 08:31 |
6 | How Spring MVC Works Internally | 03:18 |
7 | Spring Boot Web MVC Architecture (Three - Layer Architecture) | 01:48 |
8 | What is Thymeleaf? | 04:05 |
9 | How Thymeleaf Engine Work? | 02:34 |
10 | Create Spring Boot Project and Integrate Thymeleaf | 05:34 |
11 | Spring Boot Auto Configuration for Thymeleaf | 03:48 |
12 | Thymeleaf Spring Boot Hello World Example | 08:02 |
13 | Thymeleaf Variable Expressions | 12:03 |
14 | Thymeleaf Selection Expressions | 08:20 |
15 | Thymeleaf Message Expressions | 06:58 |
16 | Thymeleaf Link (URL) Expressions | 10:21 |
17 | Thymeleaf Fragment Expressions | 11:45 |
18 | Thymeleaf Attribute - th:text | 02:55 |
19 | Thymeleaf Looping or Iteration - th:each Attribute | 11:49 |
20 | Thymeleaf Looping or Iteration - th:each Attribute Status Variable | 05:21 |
21 | Thymeleaf Attribute - th:if and th:unless | 09:56 |
22 | Thymeleaf Attribute - th:switch and th:case | 08:37 |
23 | Form Handling in Thymeleaf - Overview | 03:49 |
24 | Form Handling in Thymeleaf - Create Handler Method to Return Register Page | 06:35 |
25 | Form Handling in Thymeleaf - Design User Registration Form | 15:48 |
26 | Form Handling in Thymeleaf - Display User Registration Form Data | 08:37 |
27 | Create and Setup Spring Boot Project in IntelliJ | 06:46 |
28 | Understanding spring-boot-starter-thymeleaf Dependency | 04:58 |
29 | Configure MySQL Database in Spring Boot App | 07:21 |
30 | Create Standard Packaging Structure | 03:04 |
31 | Create Post JPA Entity | 10:55 |
32 | Create PostRepository Interface | 07:58 |
33 | Create PostDto | 03:07 |
34 | Create PostMapper | 05:58 |
35 | Implement List Posts Feature - Overview | 02:01 |
36 | Create Service Layer for List Posts Feature | 06:42 |
37 | Create Controller Layer for List Posts Feature | 06:15 |
38 | Create Thymeleaf Template to Display List of Posts | 19:53 |
39 | Add Header and Footer to Thymeleaf Template HTML Page | 08:55 |
40 | Include Header, Navbar, and Footer as Fragments | 10:37 |
41 | Implement Create Post Feature - Overview | 02:53 |
42 | Create Handler Method to Handle Create Post Request | 05:50 |
43 | Create Post Form Handling | 18:10 |
44 | Create Handler Method to Save Post | 09:23 |
45 | Adding Validation to Create Post Form | 13:53 |
46 | Adding jQuery CKEditor to Crete Post Form | 05:56 |
47 | Implement Update Post Feature -Overview | 02:02 |
48 | Create Handler Method for Edit Post Request | 07:57 |
49 | Create Thymeleaf Template for Edit Post | 03:18 |
50 | Create Handler Method to Update Edited Post Data | 10:20 |
51 | Implement Delete Post Feature Step by Step | 06:17 |
52 | Implement View Post Feature - Overview | 00:37 |
53 | Create Handler Method to Handle View Post Request | 06:15 |
54 | Create Thymeleaf Template for View Post | 09:03 |
55 | Search Blog Posts Feature Overview | 01:12 |
56 | Implement Backend for Search Blog Posts Feature | 09:59 |
57 | Implement Frontend for Search Blog Post Feature | 05:52 |
58 | Quickly Refactor UI Code | 02:02 |
59 | Display List of Blog Posts Backend Implementation | 05:57 |
60 | Display List of Blog Posts Frontend Implementation | 17:07 |
61 | View Blog Post Implementation | 07:53 |
62 | Blog Search Feature Backend Implementation | 03:41 |
63 | Blog Search Feature Frontend Implementation | 07:36 |
64 | Blog Comments Management - Section Introduction | 02:19 |
65 | Create Comment JPA Entity | 09:44 |
66 | Create CommentRepository | 03:09 |
67 | Create CommentDto | 02:39 |
68 | Create CommentMapper | 04:39 |
69 | Create Comment Form Handling | 15:10 |
70 | Create Handler Method to Save Comment | 09:53 |
71 | Adding Validation to Create Comment Form | 09:33 |
72 | Display List of Comments for Blog Post | 08:54 |
73 | List Comments Feature - Backend | 07:17 |
74 | List Comments Feature - Frontend | 11:20 |
75 | Add Delete Comment Feature | 07:00 |
76 | Registration Feature - Section Introduction | 02:03 |
77 | Create User and Role Entities (Many to Many Mapping) | 11:32 |
78 | Create UserRepository and RoleRepository | 02:44 |
79 | Create Handler Method to Handle Registration Form Request | 05:06 |
80 | User Registration Form Handling | 13:33 |
81 | Create Handler Method to Save User Registered Data | 13:07 |
82 | Adding Validation to User Registration Form | 10:22 |
83 | Login and Logout Features - Section Introduction | 02:42 |
84 | Add Spring Security and Spring Security’s Default Login and Logout Features | 10:43 |
85 | Create Custom Login Form | 10:41 |
86 | Configure Spring Security | 08:41 |
87 | Configure Spring Security for Roles | 05:15 |
88 | Logout Feature Implementation | 06:49 |
89 | Database Authentication Implementation | 14:20 |
90 | Configure Spring Security for Client Side | 04:49 |
91 | Define Many to One Relationship Between Post and User | 04:19 |
92 | Refactor Create Post Feature for LoggedIn User | 06:24 |
93 | Refactor Update Post Feature for LoggedIn User | 03:25 |
94 | Refactor List Posts Feature to List Only LoggedIn User Posts | 07:52 |
95 | Refactor Admin Side List Comments Feature | 10:28 |
96 | Refactor List Posts and Comments Feature for ADMIN User | 08:30 |
97 | Adding Custom Error Page | 05:38 |
98 | Adding Specific Error Pages for 404,403 and 500 Error Codes | 07:35 |
Similar courses to Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog App
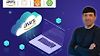
Deploy Spring Boot Microservices on AWS ECS with Fargateudemy
Category: AWS, Spring Boot
Duration 7 hours 1 minute 39 seconds
Course
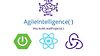
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Reduxudemy
Category: React.js, Spring Boot, Redux, Spring, Spring HATEOAS
Duration 5 hours 51 minutes 37 seconds
Course
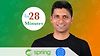
Master Java Unit Testing with Spring Boot & Mockitoudemy
Category: Spring Boot, Other (QA)
Duration 3 hours 56 minutes 12 seconds
Course
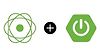
Build Reactive RESTFUL APIs using Spring Boot/WebFluxudemy
Category: Spring Boot
Duration 9 hours 34 minutes 2 seconds
Course
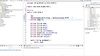
Building Your First App with Spring Boot and Angularpluralsight
Category: Angular, Spring Boot, Java
Duration 2 hours 22 minutes 15 seconds
Course
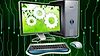
Java Spring Tutorial Masterclass - Learn Spring Framework 5udemy
Category: Spring Boot, Spring, Spring MVC
Duration 45 hours 18 minutes 33 seconds
Course
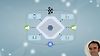
Microservices: Clean Architecture, DDD, SAGA, Outbox & Kafkaudemy
Category: Spring Boot, Others, Java
Duration 18 hours 2 minutes 34 seconds
Course
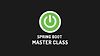
Spring Boot Master Classamigoscode (Nelson Djalo)
Category: Spring Boot
Duration 9 hours 28 minutes 30 seconds
Course
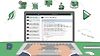
Learn Spring 5 and Spring Boot 2baeldung
Category: Spring Boot, Spring, Spring Security
Duration 4 hours 57 minutes 32 seconds
Course
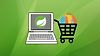
Spring Boot E-Commerce Ultimate Courseudemy
Category: Spring Boot
Duration 83 hours 14 minutes 29 seconds
Course