Spring Boot Microservices and Spring Cloud
This video course is for Beginners who have never build RESTful Web Services and Microservices before. It will guide you step-by-step through basics and will help you create and run RESTful Microservices from scratch. You will learn how to run Microservices on your own developer's machine as well as in Docker Containers on AWS EC2 Linux machines.
Read more about the course
By the end of this course you will have your own RESTful Spring Boot Microservices built and running in Spring Cloud.
You will learn how to create and run your own:
RESTful Microservices,
Eureka Discovery Service,
Zuul API Gateway,
Load Balancer,
Spring Cloud Config Server,
You will learn to use:
Spring Cloud Bus and Rabbit MQ,
Spring Boot Actuator
You will also learn how to implement for your REST API features like:
User Authentication(Login) and,
User Authorization(Registration)
You will learn to use:
Spring Security and JWT
You will learn how to use:
Spring Data JPA to store user details in a database,
H2 in-memory database and a database console,
MySQL database server,
Postman HTTP Client,
Spring Tool Suite,
Spring Initializer
This course also covers how to:
Trace HTTP Requests with Spring Cloud Sleuth and Zipkin(Distributed tracing)
Aggregate log files in one place(Centralized logging) with ELK stack(Logstash, Elasticsearch, Kibana).
You will also learn how to:
Start up AWS EC2 Linux machine,
Install Docker,
Create Docker images,
Run Microservices in Docker containers on multiple EC2 Linux machines in Amazon AWS Cloud.
- Know Java
- Mac computer
- Beginner Java developers
- Java developers interested in learning how to build Spring Boot Microservices
What you'll learn:
- Build and run RESTful Microservices
- Implement User Authentication
- Eureka Discovery Service
- Implement User Authorization with Spring Security and JWT
- Zuul API Gateway and a Load Balancer
- Learn to use JPA to persist data into a Database
- Cloud Cloud Config Server
- Learn to install MySQL Server and persist data into MySQL
- Spring Cloud Bus and Rabbit MQ
- H2 in-memory database and H2 Console
- Spring Boot Actuator
- Learn to use HTTP Postman
- Use Spring Security
- Learn to use Spring Initializer
- Distributed Tracing with Sleuth and Zipkin
- Learn to use Spring Tool Suite
- Centralized Logging with ELK Stack(Logstash, Elasticsearch, Kibana)
- Run Microservices in Docker Containers
Watch Online Spring Boot Microservices and Spring Cloud
# | Title | Duration |
---|---|---|
1 | Course Overview | 07:24 |
2 | A few suggestions | 03:30 |
3 | What is a Microservice? | 07:12 |
4 | Sample Microservices Architecture | 07:21 |
5 | Download and Install Postman HTTP Client | 02:10 |
6 | Postman Overview | 10:42 |
7 | Resource and Collection URIs | 11:13 |
8 | HTTP Methods: GET, POST, DELETE and PUT | 03:45 |
9 | HTTP Headers: Accept and Content Type | 04:13 |
10 | Install Java Platform(JDK) | 05:49 |
11 | Download and Install Spring Tool Suite(STS) | 01:56 |
12 | Introduction | 00:42 |
13 | Creating a New Project | 06:13 |
14 | Creating a new Spring project using Spring Boot Initializr | 04:43 |
15 | Create Users Rest Controller class | 02:46 |
16 | Adding Methods to Handle POST, GET, PUT, DELETE HTTP requests | 03:14 |
17 | Running Web Service Application | 04:37 |
18 | Reading Path Variables with @PathVariable annotaion | 03:32 |
19 | Reading Query String Request Parameters | 04:52 |
20 | Making Parameters Optional or Required | 04:36 |
21 | Returning Java Object as Return Value | 04:12 |
22 | Returning Object as JSON or XML Representation | 05:36 |
23 | Set Response Status Code | 04:59 |
24 | Reading HTTP POST Request Body. The @RequestBody annotation. | 08:14 |
25 | Validating HTTP POST Request Body | 08:57 |
26 | Store Users Temporary | 04:51 |
27 | Handle HTTP PUT Request | 09:07 |
28 | Handle HTTP Delete Request | 04:53 |
29 | Handle an Exception | 07:45 |
30 | Return Custom Error Message Object | 05:36 |
31 | Handle a Specific Exception | 03:07 |
32 | Throw and Handle You Own Custom Exception | 04:14 |
33 | Catch More Than One Exception with One Method | 02:55 |
34 | Dependency Injection: Create and Autowire a Service Layer Class | 10:55 |
35 | Constructor Based Dependency Injection | 06:25 |
36 | Run Web Service as a Standalone Application | 04:53 |
37 | Introduction to Eureka Discovery Service | 04:18 |
38 | Startup Eureka Service Discovery | 05:40 |
39 | Introduction to Building a Users Microservice | 02:11 |
40 | Users Microservice - Create new Spring Boot Project | 03:41 |
41 | Enable Spring Discovery Cloud Client | 03:02 |
42 | Create Users Rest Controller | 02:05 |
43 | Access Users Web Service Endpoint via Eureka Discovery Service | 03:00 |
44 | Introduction to Building an Account Management Microservice | 01:35 |
45 | Password Reset - Create a new Spring Boot Project | 04:00 |
46 | Access Account Management Microservice via Eureka Discovery Service | 02:57 |
47 | Important note | 01:49 |
48 | Introduction to Zuul API Gateway | 03:25 |
49 | Create a ZUUL API Gateway Project | 03:38 |
50 | Access Microservices via API Gateway | 04:15 |
51 | Load Balancer - Introduction | 00:51 |
52 | Starting Up More Microservices | 08:59 |
53 | Trying How Load Balancer Works | 04:48 |
54 | Important Note | 01:44 |
55 | Introduction | 06:28 |
56 | Creating API Gateway Project | 05:05 |
57 | Automatic Mapping of Gateway Routes | 06:50 |
58 | Manually Configuring API Gateway Routes | 07:50 |
59 | Trying how it works | 04:09 |
60 | Rewriting URL Path | 05:07 |
61 | Automatic & Manual Routing | 01:58 |
62 | Starting Up More Microservices | 08:59 |
63 | Trying How Load Balancer Works | 03:54 |
64 | H2 In-memory Database. Introduction. | 03:20 |
65 | H2 Database Console Overview | 07:41 |
66 | Adding Support for the H2 Database | 04:41 |
67 | Introduction | 00:42 |
68 | Adding method to handle HTTP Post Request | 01:15 |
69 | Implementing the Create User Request Model class | 03:18 |
70 | Validating HTTP Request Body | 04:21 |
71 | Application Layers | 02:49 |
72 | Implementing Service Layer Class | 02:11 |
73 | Create a Shared DTO Class | 03:16 |
74 | Generate Unique Public User Id | 02:17 |
75 | Adding Support for Spring Data JPA | 02:02 |
76 | Implementing User Entity Class | 06:08 |
77 | Implementing Spring Data JPA CRUD Repository | 02:35 |
78 | Save User Details in Database | 11:16 |
79 | Return Http Status Code | 02:30 |
80 | Implementing Create User Response Model | 04:31 |
81 | Add Spring Security to Users Microservice | 01:16 |
82 | Add WebSecurity Configuration | 04:13 |
83 | Encrypt User Password | 04:51 |
84 | Allow only IP address of Zuul API Gateway | 02:16 |
85 | Adding Support to Return JSON or XML | 05:00 |
86 | Introduction | 01:11 |
87 | Implementing LoginRequestModel | 01:22 |
88 | AuthenticationFilter. Implementing attemptAuthentication() | 06:04 |
89 | Implementing loadUserByUserName() | 11:38 |
90 | AuthenticationFilter. Implementing successfulAuthentication(). | 13:13 |
91 | Trying How User Login Works | 05:46 |
92 | Customize User Authentication URL | 03:31 |
93 | Introduction to Spring Security on API Gateway | 01:54 |
94 | Adding Support for Spring Security and JWT Tokens | 01:18 |
95 | Enable Web Security in Zuul | 04:30 |
96 | Allow Access to Registration and Login Urls | 05:07 |
97 | Implementing Authorization Filter | 08:44 |
98 | Accessing Protected Microservices with Access Token | 05:23 |
99 | Introduction | 03:11 |
100 | Using Header Predicate | 04:05 |
101 | Adding Support for JWT Token Validation | 01:52 |
102 | Creating AuthorizationFilter class | 03:50 |
103 | Assign Custom Filter to a Gateway Route | 01:13 |
104 | Signup and Login Routes | 03:06 |
105 | Reading Authorization HTTP Header | 07:43 |
106 | Validating JWT Access Token | 07:28 |
107 | Accessing Protected Microservices with Access Token | 06:40 |
108 | Introduction to Spring Cloud Config Server | 04:04 |
109 | Create Your Own Config Server | 02:54 |
110 | Create Private GitHub Repository | 02:25 |
111 | Naming Property Files Served by Config Server | 02:33 |
112 | Configure Config Server to Access Private GitHub Repository | 02:47 |
113 | Adding Properties File to Git Repository | 03:17 |
114 | Configure Users Microservice to be a Client of Config Server | 07:23 |
115 | Make Zuul Gateway a Client of Config Server | 02:19 |
116 | Introduction to Spring Cloud Bus | 03:53 |
117 | Add Spring Cloud Bus & Actuator Dependencies | 02:28 |
118 | Enable the /bus-refresh URL Endpoint | 01:48 |
119 | Download and Run Rabbit MQ | 03:21 |
120 | Rabbit MQ Default Connection Details | 02:16 |
121 | Trying how Spring Cloud Bus Works | 08:38 |
122 | Change default Rabbit MQ Password | 03:30 |
123 | Introduction to Spring Cloud Config File System as a Backend | 00:46 |
124 | Setting up File System Backend | 04:52 |
125 | Previewing Values Returned by Config Server | 01:42 |
126 | Trying how Microservices work | 02:39 |
127 | Introduction | 02:32 |
128 | Shared and a Microservice specific properties | 07:02 |
129 | Introduction to Spring Boot Actuator | 02:16 |
130 | Add Spring Boot Actuator to API Gateway | 05:00 |
131 | Trying How It Works | 05:03 |
132 | Enable Actuator for Users Microservice | 05:52 |
133 | Introduction | 01:01 |
134 | Download and Install MySQL | 03:17 |
135 | Start MySQL Server and Login | 06:15 |
136 | Create MySQL Database And a New User | 06:26 |
137 | Downloading and Installing MySQL Workbench | 03:03 |
138 | Connect to MySQL Database using MySQL WorkBench | 05:27 |
139 | MySQL WorkBench brief overview | 04:16 |
140 | Configure MySQL Database Access Details | 09:49 |
141 | Use H2 Console to Access MySQL Database | 02:49 |
142 | Copy MySQL properties to a Config Server | 01:41 |
143 | Introduction to Encryption and Decryption of Configuration Properties | 02:44 |
144 | Add Java Cryptography Extension | 06:15 |
145 | Symmetric Encryption of Properties | 08:51 |
146 | Creating a Keystore for Asymmetric Encryption | 05:06 |
147 | Asymmetric Encryption of Properties | 05:26 |
148 | Introduction to Microservices Communication | 03:49 |
149 | Clone Source Code of Albums Microservice | 02:12 |
150 | A walk through an Albums Microservice | 05:38 |
151 | Implementing Get User Details | 05:44 |
152 | Make Users Microservice call Albums Microservice | 10:26 |
153 | Trying how it works | 04:00 |
154 | Feign Web Service Client - Introduction | 01:33 |
155 | Enable Feign in Spring Boot Project | 01:55 |
156 | Create Feign Client | 05:08 |
157 | Using Feign Client | 01:48 |
158 | Trying How Feign Client Works | 03:12 |
159 | Enable HTTP Requests Logging in Feign Client | 05:42 |
160 | Handle FeignException | 04:37 |
161 | Handle Response Errors with Feign Error Decoder | 12:58 |
162 | Hystrix Circuit Breaker & Feign. Introduction. | 02:56 |
163 | Configure Project to use Hystrix Circuit Breaker | 04:29 |
164 | Trying How Hystrix Circuit Breaker & Feign work | 03:11 |
165 | Error Handling with Feign Hystrix FallbackFactory | 11:06 |
166 | Introduction to Distributed Tracing with Sleuth and Zipkin | 03:08 |
167 | Add Spring Cloud Sleuth to Users Microservice | 04:13 |
168 | Checking Trace ID and Span ID in a Console | 05:27 |
169 | Startup Zipkin Server | 02:19 |
170 | View Traces in Zipkin | 05:15 |
171 | Introduction to Aggregating Log Files with ELK Stack | 02:05 |
172 | Configure Microservices to Log into a File | 02:56 |
173 | Download Logstash | 01:40 |
174 | Configure Logstash to Read Log Files | 09:12 |
175 | Download and Run Elasticsearch | 03:17 |
176 | Run Search Query | 06:51 |
177 | Download, Install and Run Kibana | 03:45 |
178 | View Aggregated Logs in Kibana | 05:28 |
179 | Configure Spring Security to Eureka Server | 02:16 |
180 | Enable Web Security | 02:41 |
181 | Configure Eureka Clients to use Username and Password | 03:11 |
182 | Configure Eureka Service URL in Config Server | 04:20 |
183 | Move Username and Password to Config Server | 04:01 |
184 | Encrypting Username and Password | 06:04 |
185 | Introduction to Running Microservices in Docker Containers | 08:00 |
186 | Start up a new Linux Server on AWS EC2 | 14:57 |
187 | Connect to EC2 Instance | 04:47 |
188 | Install Docker on AWS EC2 | 01:52 |
189 | Docker Hub Introduction | 01:58 |
190 | Run RabbitMQ Docker Container | 09:13 |
191 | Basic Docker Commands: Run, Stop, Start, Remove Containers and Images | 06:50 |
192 | Create Config Server Docker Image | 09:49 |
193 | Publish Config Server Docker Image to Docker Hub | 04:28 |
194 | Run Config Server on EC2 from Docker Hub | 10:28 |
195 | Start New EC2 Instance for Eureka | 03:24 |
196 | Build Docker Image for Eureka Discovery Service | 04:02 |
197 | Run Eureka in Docker container | 11:21 |
198 | Elastic IP address for EC2 Instance | 04:42 |
199 | Create Zuul Api Gateway Docker Image | 04:42 |
200 | Run Zuul Api Gateway in Docker Container | 08:04 |
201 | Run Elastic Search in Docker container | 05:56 |
202 | Run Kibana in Docker Container | 06:35 |
203 | Run Kibana and Elastic Search on the same Network | 04:32 |
204 | Docker Image for Albums Microservice | 04:28 |
205 | Run Albums Microservice Docker Image on EC2 | 10:50 |
206 | Logstash Docker Image for Albums Microservice | 08:51 |
207 | Run Logstash in Docker container | 04:35 |
208 | Run MySQL in Docker Container | 08:38 |
209 | Make MySQL Docker Container Persist Data on EC2 | 03:20 |
210 | Build Users Microservice Docker Image | 04:23 |
211 | Run Users Microservice in Docker container | 10:00 |
212 | Run Logstash for Users Microservice | 07:52 |
213 | Introduction | 05:10 |
214 | Preparing Configuration for another environment | 08:32 |
215 | Creating Beans Based on Spring Boot @Profile used | 11:33 |
216 | Running Docker Container for Different Environments | 01:03 |
217 | Introduction | 06:31 |
218 | Pass Authorization Header to Downstream Microservice | 01:23 |
219 | Add AuthorizationFilter to Users Microservice | 06:02 |
220 | Configure HttpSecurity | 02:31 |
221 | Trying how it works | 03:12 |
222 | Introduction to Method-Level security | 04:56 |
223 | Enable Method-Level Security | 02:01 |
224 | @PreAuthorize annotation example | 03:31 |
225 | Trying how @PreAuthorize annotation works | 03:03 |
226 | @PostAuthorize annotation example | 07:12 |
Similar courses to Spring Boot Microservices and Spring Cloud
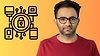
Spring Security 6 Zero to Master along with JWT,OAUTH2udemy
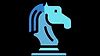
Master Spring 6 Spring Boot 3 REST JPA Hibernateudemy
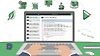
Learn Spring 5 and Spring Boot 2baeldung
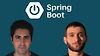
The Complete Spring Boot Development Bootcampudemy
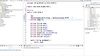
Building Your First App with Spring Boot and Angularpluralsight
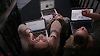
Angular 7 + Spring Boot and Cloud Microservices(Inc. Docker)udemy
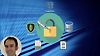
Web security: Injection Attacks with Java & Spring Bootudemy
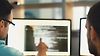
Go Full Stack with Spring Boot and Reactudemy
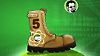
Spring Framework 5: Beginner to Guruudemy
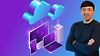