Learn Spring 5, Boot 2, JPA, Thymeleaf, AOP, Web MVC, REST
Read more about the course
Why Spring?
Spring is, by far, the most popular framework for application development in the Java ecosystem. Nothing else even comes close. Why? Because it makes software development so much easier in Java. You can build webapps, mobile apps, desktop apps, batch and big data apps and services in a record time using Spring. Spring does a lot of things right, and it's getting better and better with each release. There aren't a lot of technologies with the kind of wide reach, stability and maturity of Spring, that still move fast and innovate. That's a really tough balance to hit, and Spring has been a leader in that space for over a decade.
Today, Spring, along with Boot, is a polished, modern and highly expressive framework that makes building apps like twitter almost trivial (easily passes the twitter test). So, the popularity of the Spring ecosystem is well deserved. If you're working in Java, you're very likely doing work with Spring because 90% of all Java projects use Spring!
This course will prepare you to become an expert on the Spring Framework. Together, we will build an industry standard Spring Web Application which will transition you from a beginner to an experienced and employable Spring Developer. We will build a real world application in which we will cover topics and practices that are used throughout the industry. You will type every line of code along with me, as I explain, to ensure that you fully comprehend the subject matter. I will be available to answer any questions that you may have, so you will never be stuck on a section, or have confused on any topic.
Topics covered in this course include:
Spring Framework 5
Spring Boot 2
Spring MVC
Maven
Spring Data CRUD Repositories
Tymeleaf
Hibernate
Spring Annotations
Using the Command Line to run Spring Boot Apps
Core Spring Concepts
Property Configurations
PostgreSQL Database
Integration Testing
Deploying our Spring Application to the AWS EC2 Server
Containerization using Docker
Spring AOP (Aspect Oriented Programming)
Spring Security
- RESTFul Web Services API
- Student needs to know how to write basic applications in Java
- Basics of SQL Databases
Who this course is for:
- Students that want real-world experience in enterprise software development
- Beginner level Java developers
- Students that are sick of learning from building 20 little Twitter clones or todo list apps and want to actually master something at a deeper level!
What you'll learn:
- BUILD a REAL-WORLD INDUSTRY STANDARD full-stack Spring web application with Production Deployment
- MASTER Industry Relevant practices for development in Spring 5
- LEARN Thymeleaf and how to involve Spring data with Javascript
- CODE along with me to PRACTICE and IMPLEMENT everything you learn in real-time
- LEARN to use Spring MVC & Spring Data CRUD Repositories, Hibernate using a real database like PostgreSQL, as well as H2
- PRACTICE Production Deployment in AWS, as well as Containerizing your Spring apps using Docker
- LEARN Property File Configurations for Integration Testing vs Live App
- TRANSITION from a beginner to a SKILLED Spring Developer
- NOT WASTE TIME building 20 Twitter or Todo list app clones, but build something more SIGNIFICANT and dive deeper as you learn and build an INDUSTRY GRADE app
- OBTAIN and DEMONSTRATE your skills with the Spring Framework to LAND A JOB as a Spring Developer
Watch Online Learn Spring 5, Boot 2, JPA, Thymeleaf, AOP, Web MVC, REST
# | Title | Duration |
---|---|---|
1 | IMPORTANT: Source Code and Files [Watch Entire Video First] | 03:44 |
2 | What and Why of Spring | 07:18 |
3 | Setting up the Development Environment | 19:36 |
4 | Understanding Post Form, Query Params and Path Variables | 20:56 |
5 | Path Variables and Request Params Continued | 21:08 |
6 | Overview of Spring MVC | 04:49 |
7 | Form Submission in Views | 27:49 |
8 | Redirects and Data | 05:53 |
9 | Setting Up a New Project (The PMA Web App) | 06:39 |
10 | Turn a Class into an Entity | 06:06 |
11 | Bind Java Objects to Forms in Thymeleaf | 16:53 |
12 | Create Crud Repository for Project Entity | 17:53 |
13 | Create Crud Repository for Employee Entity | 17:42 |
14 | Using Crud Repositories | 19:07 |
15 | Organize Thymeleaf Views | 15:43 |
16 | HW for Thymeleaf Views and Navigation | 11:01 |
17 | @OneToMany and @ManyToOne Annotations for Relating Entities | 10:29 |
18 | @OneToMany Annotation with Thymeleaf Form Binding | 29:09 |
19 | @ManyToMany Annotation | 19:16 |
20 | Seeding the Database with CommandLineRunner | 06:49 |
21 | Seeding the Database Using SQL Files | 20:01 |
22 | Including Javascript and CSS Files in Your Project | 08:05 |
23 | Improving the Homepage | 12:51 |
24 | Custom Queries in Spring Data Repositories | 19:11 |
25 | Custom Query Continued: Project Status Query | 14:14 |
26 | Using Model Attributes with JavaScript in Spring and Thymeleaf | 19:11 |
27 | Spring Dependency Injection | 19:26 |
28 | Component Scanning @Service, @Component and @Repository Annotations | 12:13 |
29 | Constructor Injection, Field Injection and Setter Injection | 11:32 |
30 | @Primary and @Qualifier Annotations | 10:46 |
31 | Property Configurations and Reading Values | 08:09 |
32 | Reading Environment Variables in Property Configuration Files | 12:06 |
33 | Installing the PostgreSQL Database and Driver | 07:54 |
34 | Configure Properties for Postgres Database | 23:34 |
35 | Setting Up Configurations for Integration Testing | 24:04 |
36 | Using @SpringBootTest Annotation Correctly | 07:58 |
37 | Integration Tests for Controllers/Views | 10:22 |
38 | Deploy a Spring Application to AWS EC2 Server | 40:10 |
39 | Working with Docker to Containerize Your Apps | 33:46 |
40 | Building a Docker Image for a Spring Boot App and Running it in a Container | 24:59 |
41 | Setting up an AWS Cloud Hosted Postgres Database | 20:43 |
42 | Making a Production Ready Docker Image for Your Spring Boot App | 13:42 |
43 | Decoupling Repositories from Controllers | 02:35 |
44 | Working with Spring Profiles | 19:37 |
45 | Spring AOP Part 1: Pointcut and Advice (@Before and @After) | 18:43 |
46 | Spring AOP Part 2: JoinPoint and ProceedingJoinPoint with @Around | 15:48 |
47 | Best Practices for Logging | 06:22 |
48 | Spring Security Part 1: Basic In-Memory Authentication | 12:18 |
49 | Spring Security Part 2: Authorization in Memory | 08:48 |
50 | Spring Security Part 3: JDBC Backed Security | 08:26 |
51 | Spring Security Part 4: Postgres Database with JDBC Authorization/Authentication | 20:48 |
52 | Spring Security Part 5: User Registration and Password Encryption | 32:15 |
53 | Spring Security Part 6: Customize White Label Error Pages | 13:29 |
54 | The Anatomy of a REST API | 09:13 |
55 | CRUD REST Endpoints for Entities and HTTP Verbs | 33:02 |
56 | Setting Validation Rules for a REST API | 08:26 |
57 | Custom Clientside Validation Involving Data Repositories | 18:10 |
58 | Pagination in REST API | 11:07 |
59 | Spring Data Rest | 11:11 |
60 | Update and Delete Entities | 23:07 |
61 | Improving Forms and Validations | 17:56 |
62 | Timelines Project- Part 1 | 15:03 |
63 | Timelines Project - Part 2 | 11:21 |
64 | Timelines Project- Part 3 | 16:30 |
Similar courses to Learn Spring 5, Boot 2, JPA, Thymeleaf, AOP, Web MVC, REST
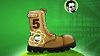
Spring Framework 5: Beginner to Guruudemy
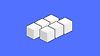
Microservices and Distributed Systemsamigoscode (Nelson Djalo)
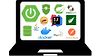
Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)udemy
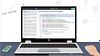
Build Your REST API with Spring 5baeldung
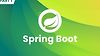
Spring Boot: Mastering the Fundamentalscodewithmosh (Mosh Hamedani)
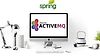
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
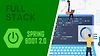
Full Stack Spring Boot & Reactamigoscode (Nelson Djalo)
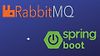
RabbitMQ : Messaging with Java, Spring Boot And Spring MVCudemy
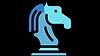