Spring Boot: Mastering the Fundamentals
Spring Boot is the primary framework for modern Java development. Whether you are creating web applications, microservices, or enterprise systems, Spring Boot simplifies development by automating configuration, managing dependencies, and providing embedded servers, allowing for quick creation and deployment of applications.
Mastering Spring Boot is not only an opportunity to write higher quality code but also a significant career step. According to Glassdoor, Spring Boot specialists are in demand in the market, with salaries ranging from $164K to $306K per year, with a median of $219K.
Read more about the course
This course is the first part of a series aimed at guiding you from basics to full mastery in Spring Boot. In this part of the course, we focus on fundamental concepts to ensure a solid understanding of basic principles before moving on to building web applications and APIs in the next module.
This is not just another Spring Boot course - it's a clear, concise, and practical program where each lesson is designed to expand your knowledge through real-world examples, best practices, and useful tips rarely found in other training programs.
If you are looking for a structured, practical course with minimal theory that will equip you with the skills to create real applications, then this course is for you!
What you will learn:
- Understand how Spring Boot manages objects and dependencies.
- Connect applications to relational databases.
- Configure applications using application.properties and YAML files.
- Use Spring Data repositories for database operations.
- Distinguish between "database-first" and "model-first" approaches and understand when to apply each.
- Track entities through Hibernate and ensure data integrity with transactions.
- Optimize data fetching strategies with eager and lazy loading.
- Create custom queries using @Query, JPQL, and native SQL.
- Apply projections to extract only necessary data and reduce load.
- Build dynamic queries using Query by Example, Criteria API, and Specifications API.
- Implement sorting and pagination for efficient handling of large data volumes.
- Apply Lombok to reduce boilerplate code and simplify entity classes.
- Use tools for quickly generating entities, repositories, and queries.
- Apply best practices for application structuring and performance optimization.
Watch Online Spring Boot: Mastering the Fundamentals
# | Title | Duration |
---|---|---|
1 | 1- Welcome | 01:13 |
2 | 2- Prerequisites | 00:51 |
3 | 3- Course Structure | 01:44 |
4 | 4- Source Code | 01:17 |
5 | 1- Introduction | 00:51 |
6 | 2- Introduction to Spring Boot | 02:42 |
7 | 3- Setting up the Development Environment | 03:26 |
8 | 4- Creating a Spring Boot Project | 03:44 |
9 | 5- Understanding the Project Structure | 03:59 |
10 | 6- Dependency Management | 07:52 |
11 | 7- Building Your First Spring Controller | 04:14 |
12 | 8- Running Spring Boot Applications | 02:25 |
13 | 9- Debugging Spring Boot Applications | 05:55 |
14 | 10- Automatic Restarts with Spring Boot DevTools | 03:38 |
15 | 11- Configuring Application Properties | 02:38 |
16 | 1- Introduction | 01:02 |
17 | 2- What is Dependency Injection? | 07:43 |
18 | 3- Constructor Injection | 04:55 |
19 | 4- Setter Injection | 02:40 |
20 | 5- The Spring IoC Container | 02:53 |
21 | 6- Configuring Beans Using Annotations | 05:02 |
22 | 7- Controlling Bean Selection | 02:57 |
23 | 8- Exerise- Implementing a Notification Service | 04:27 |
24 | 9- Externalizing Configuration | 07:31 |
25 | 10- Configuring Beans Using Code | 06:32 |
26 | 11- Lazy Initialization | 03:13 |
27 | 12- Bean Scopes | 02:31 |
28 | 13- Bean Lifecycle Hooks | 03:59 |
29 | 14- Exercise- Implementing a User Registration Service | 05:48 |
30 | 1- Introduction | 02:04 |
31 | 2- JDBC, JPA, Spring Data JPA | 04:09 |
32 | 3- Setting Up MySQL | 01:53 |
33 | 4- Setting Up Spring Data JPA | 03:24 |
34 | 5- Designing the Application Model | 02:50 |
35 | 6- Creating the Database | 00:57 |
36 | 6.1- Creating Database Tables | 05:50 |
37 | 6.2- Database Migrations with Flyway | 04:53 |
38 | 6.3- Chaning the Database Schema | 05:03 |
39 | 6.4- Running Migrations Using Maven | 05:18 |
40 | 6.5- Exercise- Creating the Profiles, and Tags Tables | 04:56 |
41 | 7- Creating the Domain Model | 00:50 |
42 | 7.1- Defining Entities | 05:59 |
43 | 7.2- Simplifying Code with Lombok | 05:55 |
44 | 7.3- Exercise- Defining the Address, Profile and Tag Entities | 02:40 |
45 | 7.4 - Defining One-To-Many Relationships | 09:23 |
46 | 7.5- Defining Many-to-Many Relationships | 07:37 |
47 | 7.6- Defining One-to-One Relationships | 04:49 |
48 | 7.7- Exercise- Creating the Category and Product Entities | 03:29 |
49 | 7.8- Generating Entities Using JPA Buddy | 05:38 |
50 | 7.9- Model First with JPA Buddy | 07:40 |
51 | 7.10- Exercise- Creating the Wishlist Table | 04:32 |
52 | 7.11- Generating Database Tables with Hibernate | 04:39 |
53 | 8- Creating Repositories | 01:07 |
54 | 8.1- Defining Repositories | 04:36 |
55 | 8.2- Using Repositories | 06:19 |
56 | 8.3- Understanding Entity States | 02:09 |
57 | 8.4- Managing Transactions | 07:04 |
58 | 8.5- Fetching Strategies- Eager and Lazy Loading | 08:25 |
59 | 8.6- Exercise- Understanding Fetching Strategies | 04:03 |
60 | 8.7- Persisting Related Entities | 03:18 |
61 | 8.8- Deleting Related Entities | 10:09 |
62 | 8.9- Exercise- Managing Products and Wishlists | 11:49 |
63 | 9- Writing Custom Queries | 00:58 |
64 | 9.1- Derived Query Methods | 05:25 |
65 | 9.2- Writing Custom Queries Using @Query | 08:54 |
66 | 9.3- Fetching Partial Data with Projections | 08:09 |
67 | 9.4- Efficiently Loading Entities with @EntityGraph | 06:39 |
68 | 9.5- Avoiding the N+1 Problem | 05:22 |
69 | 9.6- Calling Stored Procedures | 06:55 |
70 | 9.7- Exercise- Writing Custom Queries | 08:51 |
71 | 10- Writing Dynamic Queries | 01:10 |
72 | 10.1- Query by Example | 06:04 |
73 | 10.2- Dynamic Queries Using Criteria API | 10:00 |
74 | 10.3- Composable Queries Using Specifications API | 07:21 |
75 | 10.4- Sorting and Pagination | 06:19 |
76 | 1- What's Next | 01:28 |
Read Book Spring Boot: Mastering the Fundamentals
# | Title |
---|---|
1 | 1. Questions and Support |
2 | 2. Connect with Me |
3 | 3. Getting Started with Spring Boot |
4 | 4. Implementing a Notification Service |
5 | 5. Implementing a User Registration Service |
6 | 6. Dependency Injection |
7 | 7. Application Model |
8 | 8. Creating the Profiles and Tags Tables |
9 | 9. Creating the Address, Profile, and Tag Entities |
10 | 10. Creating the Category and Product Entities |
11 | 11. Creating the Wishlist Table |
12 | 12. Repositories |
13 | 13. Entity States |
14 | 14. Understanding Fetching Strategies |
15 | 15. Managing Products and Wishlists |
16 | 16. Writing Custom Queries |
17 | 17. Writing Dynamic Queries |
18 | 18. Database Integration with Spring Data JPA |
Similar courses to Spring Boot: Mastering the Fundamentals
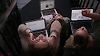
Angular 7 + Spring Boot and Cloud Microservices(Inc. Docker)udemy
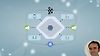
Microservices: Clean Architecture, DDD, SAGA, Outbox & Kafkaudemy
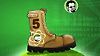
Spring Framework 5: Beginner to Guruudemy
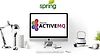
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
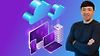
Spring Boot Microservices and Spring Cloud. Build & Deploy.udemy
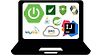
Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog Appudemy
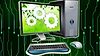
Java Spring Tutorial Masterclass - Learn Spring Framework 5udemy
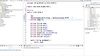
Building Your First App with Spring Boot and Angularpluralsight
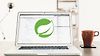
Learn Spring 5, Boot 2, JPA, Thymeleaf, AOP, Web MVC, RESTudemy
![[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners](https://cdn.courseflix.net/courses/100x56/new-spring-boot-3-spring-6-hibernate-for-beginners.jpg?d=1745020916372)