Spring Boot: Mastering REST API Development
Spring Boot is one of the most in-demand frameworks for modern backend development. This course will take you beyond the basics and help you confidently develop, secure, and deploy real-world applications.
This is the second part of an extensive course on Spring Boot. In the first part, you mastered the basics, and now it's time to apply your knowledge in practice by creating and deploying a backend for a full-fledged e-commerce application.
You will learn how to create clean and secure REST APIs, implement authentication and role-based access control, integrate with Stripe for payment processing, and deploy the application in the cloud.
Read more about the course
This course goes beyond the standard CRUD. We will focus on clean code, modular architecture, and best practices applied in real-world projects.
Who is this course for?
Those preparing for interviews, planning to create their own application, or wanting to elevate their backend development skills to a professional level.
You will learn how to:
- Develop REST API using Spring Boot
- Validate requests and handle errors with custom logic
- Secure the API with Spring Security and JWT
- Implement role-based access control
- Structure the application according to clean architecture principles
- Create a shopping cart system and a full checkout process
- Integrate Stripe for accepting real payments
- Configure environments using Spring Profiles
- Deploy the application and database to the cloud
- Write clean, testable, and production-ready code
What you will build:
A full-fledged backend for an e-commerce platform: including authentication, roles, shopping cart, checkout process, Stripe integration, and cloud deployment.
This is not just an educational CRUD project - it's a real application that is close to professional development.
Watch Online Spring Boot: Mastering REST API Development
# | Title | Duration |
---|---|---|
1 | 1 - Welcome | 01:33 |
2 | 2- What You'll Learn | 02:11 |
3 | 3- Prerequisites | 01:18 |
4 | 4- Starter Project | 04:09 |
5 | 5- How to Take This Course | 01:22 |
6 | 1- Introduction | 00:51 |
7 | 2- How the Web Works | 05:12 |
8 | 3- What is Spring MVC | 01:38 |
9 | 4- Creating a Controller | 04:15 |
10 | 5- View Templates | 03:27 |
11 | 6- Passing Data to Views | 02:00 |
12 | 7- Building an API | 04:29 |
13 | 1- Introduction | 00:58 |
14 | 2- Creating Your First RESTful API | 04:01 |
15 | 3- Calling APIs with Postman | 02:36 |
16 | 4- Creating Dynamic Routes | 03:29 |
17 | 5- Setting HTTP Status Codes | 03:03 |
18 | 6- Data Transfer Objects | 04:51 |
19 | 7- Mapping Objects Using MapStruct | 04:56 |
20 | 8- Customizing Reponse Data | 05:02 |
21 | 9- Extracting Query Parameters | 08:14 |
22 | 10- Exercise- Building Product Endpoints | 10:20 |
23 | 11- Extracting Request Headers | 02:51 |
24 | 12- Extracting Request Body | 03:18 |
25 | 13- Creating Resources | 09:27 |
26 | 14- Updating Resources | 05:47 |
27 | 15- Deleting Resources | 01:54 |
28 | 16- Handling Action-Based Updates | 04:55 |
29 | 17- Exercise- Building a CRUD API | 11:15 |
30 | 1- Introduction | 00:49 |
31 | 2- Introduction to Jakarta Validation | 03:52 |
32 | 3- Handling Validation Errors | 05:13 |
33 | 4- Global Error Handling | 01:44 |
34 | 5- Implementing Custom Validation | 04:39 |
35 | 6- Validating Business Rules | 03:16 |
36 | 1- Introduction | 01:01 |
37 | 2- Creating Database Tables | 08:06 |
38 | 3- Creating Entities | 06:32 |
39 | 4- Creating a Cart | 06:04 |
40 | 5- Adding a Product to the Cart | 12:52 |
41 | 6- Getting a Cart | 10:24 |
42 | 7- Updating a Cart Item | 08:40 |
43 | 8- Refactoring Towards an Object-Oriented Design | 06:17 |
44 | 9- Removing a Product from the Cart | 04:48 |
45 | 10- Clearing a Cart | 01:54 |
46 | 11- Extracting a Service | 12:30 |
47 | 12- Documenting APIs with Swagger | 04:35 |
48 | 1- Introduction | 00:53 |
49 | 2- Authentication Methods | 05:21 |
50 | 3- Adding Spring Security | 01:14 |
51 | 4- Configuring Security Settings | 07:35 |
52 | 5- Hashing Passwords | 02:51 |
53 | 6- Exercise- Building the Login API | 04:52 |
54 | 7- Working with AuthenticationManager | 11:18 |
55 | 8- Generating JSON Web Tokens | 06:19 |
56 | 9- Managing Secrets | 08:04 |
57 | 10- Validating JSON Web Tokens | 07:16 |
58 | 11- Understanding Filters | 03:07 |
59 | 12- Implementing a Custom Filter to Validate JWTs | 08:56 |
60 | 13- Accessing the Current User | 03:40 |
61 | 14- Exercise- Enhancing the JWT Payload | 06:21 |
62 | 15- Understanding Refresh Tokens | 01:52 |
63 | 16- Issuing Refresh Tokens | 04:57 |
64 | 17- Externalizing JWT Configuration | 04:56 |
65 | 18- Refreshing Access Tokens | 07:58 |
66 | 19- Adding Role to Users | 05:45 |
67 | 20- Role-based Authorization | 08:34 |
68 | 21- Exercise- Refactoring the JwtService | 12:41 |
69 | 22 - Logging Out Users | 01:37 |
70 | 23- Using Auth Providers | 02:11 |
71 | 1- Introduction | 00:55 |
72 | 2- Creating Database Tables | 04:52 |
73 | 3- Creating Entities | 05:52 |
74 | 4- Checking Out | 14:25 |
75 | 5- Organizing API Tests with Postman Collections | 12:04 |
76 | 6- Improving Error Handling | 05:01 |
77 | 7- Refactoring Towards an Object-Oriented Design | 03:56 |
78 | 8- Extracting a Service | 05:57 |
79 | 9- Getting Orders | 10:25 |
80 | 10- Getting a Single Order | 10:30 |
81 | 1- Introduction | 00:51 |
82 | 2- Overview of the Checkout Process | 03:09 |
83 | 3- Adding Stripe to the Project | 03:30 |
84 | 4- Creating a Checkout Session | 09:45 |
85 | 5- Handling Stripe Erorrs | 07:12 |
86 | 6- Decoupling from Stripe | 10:41 |
87 | 7- Building a Webhook Endpoint | 06:49 |
88 | 8- Testing the Webhook Endpoint | 05:08 |
89 | 9- Updating Order Status | 04:59 |
90 | 10- Refactoring the Webhook Logic | 17:48 |
91 | 11- Organizing Code by Feature | 03:22 |
92 | 1- Introduction | 00:49 |
93 | 2- Deploying the Database | 02:35 |
94 | 3- Managing Environments with Spring Profiles | 03:57 |
95 | 4- Packaging the App for Production | 05:23 |
96 | 5- Pushing Your Code to GitHub | 01:46 |
97 | 6- Deploying the App | 01:16 |
98 | 7- Configuring Production Environment Variables | 04:38 |
99 | 8- Testing with Postman Environments | 02:14 |
100 | 9- Populating the Database | 02:53 |
101 | 10- Revisiting the Security Rules | 02:29 |
102 | 11- Modularizing Security Rules | 05:53 |
103 | 12- Registering the Webhook Endpoint with Stripe | 07:01 |
104 | 1- Thank You | 00:25 |
Read Book Spring Boot: Mastering REST API Development
# | Title |
---|---|
1 | 1.6- Questions and Support |
2 | 1.7- Connect with Me |
3 | 2.8- Summary - Introduction to Spring MVC |
4 | 3.18- Buliding RESTful APIs |
5 | 4.7- Summary - Validating API Requests |
6 | 6.24- Summary - Securing APIs with Spring Security |
7 | 8.12- Final Refactorings |
8 | 9.13- What-s Next |
Similar courses to Spring Boot: Mastering REST API Development
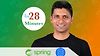
Master Java Unit Testing with Spring Boot & Mockitoudemy
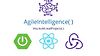
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Reduxudemy
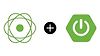
Build Reactive RESTFUL APIs using Spring Boot/WebFluxudemy
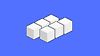
Microservices and Distributed Systemsamigoscode (Nelson Djalo)
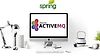
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
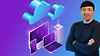
Spring Boot Microservices and Spring Cloud. Build & Deploy.udemy
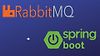
RabbitMQ : Messaging with Java, Spring Boot And Spring MVCudemy
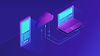
Spring Boot Microservices and Spring Cloududemy
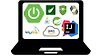
Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog Appudemy
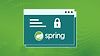