Spring Boot E-Commerce Ultimate Course
I'm very glad that you have found the most sophisticated and comprehensive course ever on web development with Java and Spring Boot. In this course, you will learn how to program a real-life shopping application using the latest technologies such as Java, Spring Boot, Hibernate, Thymeleaf, Bootstrap, jQuery, HTML, RESTful Webservices... putting all these pieces together for building professional applications beloved by the end users, and bring revenue to businesses.
Read more about the course
Modules you will learn to build in the Admin application: Users, Categories, Brands, Products, Customers, Shipping, Orders, Sales Report, Reviews, Questions, Settings.
Modules you will learn to build in the Shopping application: categories listing, product details, search products, shopping cart, checkout, manage orders, customer registration.
You also learn how to deploy the ecommerce webapp on Heroku cloud platform, with user's uploaded files stored on Amazon S3 server.
You will be a master of the following technologies by the end of this course:
- Spring framework development with Spring Boot
- Spring Data JPA and Hibernate framework
- Spring Security for authentication and authorization
- Spring OAuth for social login with Facebook and Google
- Spring Mail for sending customer registration confirmation and order confirmation
- PayPal Checkout API for capturing payment from customer
- Google Chart API for drawing charts of sales report
- JUnit, AssertJ and Mockito in unit testing
- Spring RESTful Webservices
- Bootstrap 4, HTML5 and jQuery 3
- Amazon S3 APIs
- Deployment on Heroku (AWS)
Watch Online Spring Boot E-Commerce Ultimate Course
# | Title | Duration |
---|---|---|
1 | Understand Functional Requirements | 02:29 |
2 | Understand Technical Requirements | 01:19 |
3 | Understand User Interface Requirements | 02:38 |
4 | Overview of Database Design | 01:30 |
5 | Overview of System Architecture | 01:27 |
6 | Setup Java Development Kit (JDK) | 05:58 |
7 | Setup Spring Tool Suite IDE | 04:38 |
8 | Setup MySQL Community Server | 09:53 |
9 | Install Git | 03:08 |
10 | Install Maven | 02:48 |
11 | Notes about Java Version for Project | 00:43 |
12 | Create a Multi-Module Project | 36:09 |
13 | Referential Project on GitHub | 00:55 |
14 | Clone Project from GitHub in Command Line | 08:15 |
15 | Clone Project from GitHub in Eclipse | 06:07 |
16 | Check Out a Specific Commit in Command Line | 04:31 |
17 | Understand Application Architecture | 02:18 |
18 | Understand Requirement for Admin Home Page | 01:32 |
19 | Using jQuery and Bootstrap | 16:01 |
20 | Add Logo and Create Header Menu | 13:36 |
21 | Key Notes #1 | 01:17 |
22 | How to Test on Your Mobile Phone | 02:11 |
23 | Understand Requirement of User Management Module | 04:14 |
24 | Understand Technical Design for User Module | 02:36 |
25 | Create Database and Configure Data Source | 05:52 |
26 | Code Role Entity, Role Repository and Unit Test | 26:21 |
27 | Code User Entity, User Repository and Unit Tests | 41:47 |
28 | Code Users Listing Function | 16:50 |
29 | Code New User Function | 35:01 |
30 | Encode User Password | 13:24 |
31 | Check Uniqueness of User Email | 36:37 |
32 | Using Fontawesome for Icons | 14:53 |
33 | Code Update User Function | 30:20 |
34 | Code Delete User Function | 19:27 |
35 | Code Update User Enabled Status | 11:57 |
36 | Code User Photos Upload Function | 55:56 |
37 | Fix Images Not Displayed | 07:22 |
38 | Import Sample Users Data | 09:28 |
39 | Code Pagination for Users List | 39:07 |
40 | Code Sorting for Users List | 22:43 |
41 | Code Filter Function for Users List | 32:49 |
42 | Code Refactor #1 | 16:47 |
43 | Code Export Users to CSV | 17:55 |
44 | Code Export Users to Excel | 29:29 |
45 | Code Export Users to PDF | 21:04 |
46 | Update Users Listing for Mobile | 30:30 |
47 | Test Users Listing on iPhone | 03:05 |
48 | Key Notes #2 | 04:42 |
49 | Understand Requirement for User Authentication | 01:34 |
50 | Code User Login Page | 14:42 |
51 | Code User Authentication | 17:07 |
52 | Show User's Full Name and Logout Link | 09:04 |
53 | Code Logout Function | 08:02 |
54 | Code Refactor #2 | 11:41 |
55 | Enable Remember Me for Login | 11:42 |
56 | Code Update Account Details | 37:18 |
57 | Test User Authentication on iPhone | 03:00 |
58 | Key Notes #3 | 02:30 |
59 | Understand Requirement for User Authorization | 02:54 |
60 | Code User Authorization Basics | 15:39 |
61 | Code Refactor #3 | 12:42 |
62 | Test User Authorization on Smartphone | 03:11 |
63 | Code Custom Error Pages | 09:49 |
64 | Configure Logging for Admin application | 21:48 |
65 | Understand Requirement of Category Module | 03:12 |
66 | Understand Technical Design for Category Module | 01:52 |
67 | Code Category Entity and Repository | 08:21 |
68 | Code Unit Tests for Category Repository | 27:40 |
69 | Exercise: Write Initial Code for Listing Categories | 02:56 |
70 | Code Category Form | 19:29 |
71 | Code Create Category Function | 21:32 |
72 | List Categories in Hierarchical Form | 24:25 |
73 | Exercise: Code Update Category Function | 06:14 |
74 | Check Uniqueness of Category | 45:15 |
75 | Code Sort Function for Categories List | 22:39 |
76 | Exercise: Code Update Category Enabled Status | 02:19 |
77 | Exercise: Code Delete Category Function | 07:03 |
78 | Code Pagination for Categories List | 19:31 |
79 | Code Filter Function for Categories List | 15:16 |
80 | Import Sample Categories Data | 05:01 |
81 | Exercise: Code Export Categories to CSV | 02:15 |
82 | Exercise: Make Categories Listing Page Fully Responsive | 02:35 |
83 | Code Refactor #4 | 19:06 |
84 | Key Notes #4 | 01:04 |
85 | Understand Requirement of Brand Module | 01:44 |
86 | Understand Technical Design for Brand Module | 01:54 |
87 | Code Brand Entity and Repository | 08:48 |
88 | Exercise: Code Unit Tests for Brand Repository | 03:05 |
89 | Exercise: Code Listing Brands | 04:21 |
90 | Exercise: Code Brand Form | 04:34 |
91 | Code Category Selection in Brand Form | 10:11 |
92 | Exercise: Code Create, Update and Delete Brand Function | 08:35 |
93 | Exercise: Check Uniqueness of Brand | 05:24 |
94 | Import Sample Brands Data | 06:03 |
95 | Exercise: Code Pagination, Sort and Filter for Brands Listing | 03:50 |
96 | Exercise: Make Brands Page Fully Responsive | 02:28 |
97 | Code Refactor #5 | 06:43 |
98 | Key Notes #5 | 00:53 |
99 | Understand Requirement of Product Module | 03:48 |
100 | Understand Technical Design for Product Module | 02:33 |
101 | Code Product Entity and Repository | 12:34 |
102 | Code Unit Tests for Product Repository | 20:17 |
103 | Code Products Listing Page | 14:17 |
104 | Code Product Overview Form | 43:15 |
105 | Code Product Form Description and Shipping | 18:15 |
106 | Code Save Product Function | 09:40 |
107 | Exercise: Check Uniqueness of Product | 04:09 |
108 | Exercise: Update Product Enabled Status | 03:40 |
109 | Exercise: Code Delete Product | 03:33 |
110 | Start Coding Upload Product Images | 14:32 |
111 | Code Upload Product's Main Image | 18:40 |
112 | Code Add Extra Images on Product Form | 39:08 |
113 | Code Upload Product's Extra Images | 15:17 |
114 | Exercise: Code Product Detail Entity Class | 02:55 |
115 | Code Add Details (Specs) on Product Form | 24:49 |
116 | Code Save Product's Details (Specs) | 08:01 |
117 | Code Edit Product Function | 50:25 |
118 | Code Update Product Function | 53:55 |
119 | Code View Product Details in Modal Dialog | 30:51 |
120 | Import Sample Products Data | 09:43 |
121 | Code Pagination, Sort and Filter for Products Page | 16:04 |
122 | Update Category Module for Product Search | 10:01 |
123 | Code Filter Products by Category | 35:47 |
124 | Code Authorization for Product Module | 38:13 |
125 | Exercise: Make Products Listing Page Fully Responsive | 03:47 |
126 | Code Refactor #6 | 12:12 |
127 | Key Notes #6 | 01:32 |
128 | Understand Requirement and Design of Products Listing | 03:27 |
129 | Code Home Page for Shopping Application | 19:49 |
130 | Code Listing Categories in Home Page | 14:37 |
131 | Code Listing Products in Category | 56:04 |
132 | Code Product Details Page | 42:33 |
133 | Code Product Images Slideshow | 34:08 |
134 | Code Search Products Function | 36:51 |
135 | Key Notes #7 | 01:35 |
136 | Understand Requirement and Design of Settings Module | 02:38 |
137 | Code Setting Entity, Repository and Unit Tests | 15:46 |
138 | Code Currency Entity, Repository and Unit Tests | 04:09 |
139 | Code Manage General Settings | 01:06:56 |
140 | Apply General Settings for Shopping Application | 42:42 |
141 | Exercise: Code Repository Layer for Country and State | 04:22 |
142 | Code Controller Layer for Country | 42:04 |
143 | Code Manage Countries | 01:03:49 |
144 | Exercise: Code Controller Layer for State/Province | 04:03 |
145 | Exercise: Code Manage States/Provinces | 06:35 |
146 | Import Sample Data for Countries and States | 06:19 |
147 | Code Refactor #7 | 15:23 |
148 | Key Notes #8 | 03:14 |
149 | Understand Requirement and Design of Customer Registration | 03:58 |
150 | Exercise: Code Repository Layer for Customer | 04:56 |
151 | Code Customer Registration Form | 46:29 |
152 | Code Manage E-mail Settings | 35:42 |
153 | Code Send Verification Email | 37:20 |
154 | Code Verify Customer Account | 11:18 |
155 | Test Customer Registration on Smartphone | 04:24 |
156 | Code Refactor #8 | 11:52 |
157 | Key Notes #9 | 01:24 |
158 | Understand Requirement and Design of Customer Module | 03:17 |
159 | Exercise: Code Customer Management Module | 11:56 |
160 | Import Sample Customers Data | 05:27 |
161 | Code Refactor #9 (Part 1) | 37:35 |
162 | Code Refactor #9 (Part 2) | 40:18 |
163 | Understand Requirement and Design of Customer Authentication | 02:04 |
164 | Exercise: Code Customer Login and Logout | 12:28 |
165 | Update Authentication Type of Customer | 12:20 |
166 | Understand OAuth 2.0 | 06:01 |
167 | Code Login with Google | 51:23 |
168 | Code Login with Facebook | 17:11 |
169 | Code Update Customer's Details | 54:09 |
170 | Code Forgot Password Function | 59:14 |
171 | Test Customer Authentication on Smartphone | 05:20 |
172 | Code Refactor #10 | 10:56 |
173 | Key Notes #10 | 00:50 |
174 | Understand Requirement and Design of Shopping Cart Module | 03:33 |
175 | Code Repository Layer for Shopping Cart | 29:50 |
176 | Code Quantity Control Component | 20:55 |
177 | Code Add Product to Shopping Cart | 36:11 |
178 | Code View Shopping Cart | 40:13 |
179 | Code Update Product Quantity | 34:32 |
180 | Code Remove Product from Shopping Cart | 31:55 |
181 | Format Currency in Shopping Cart | 17:56 |
182 | Test Shopping Cart on Smartphone | 06:37 |
183 | Code Refactor #11 | 09:27 |
184 | Key Notes #11 | 07:38 |
185 | Understand Requirement and Design of Shipping Rates Module | 03:06 |
186 | Exercise: Code Shipping Rates Management Module | 12:47 |
187 | Import Sample Data for Shipping Rates | 03:14 |
188 | Understand Requirement and Design of Address Book Module | 04:44 |
189 | Exercise: Code Repository Layer for Address Book | 08:47 |
190 | Code Address Book Listing | 34:32 |
191 | Exercise: Code Add, Edit and Delete Address | 16:00 |
192 | Code Set Default Address | 20:52 |
193 | Test Address Book on Smartphone | 04:10 |
194 | Import Sample Data for Address Book | 04:33 |
195 | Understand Requirement and Design of Order Module | 04:03 |
196 | Code Repository Layer for Order Module | 43:36 |
197 | Exercise: Code Orders Listing | 18:43 |
198 | Exercise: Code View Order Details | 10:33 |
199 | Exercise: Code Delete Order | 04:24 |
200 | Exercise: Code Fully-responsive Orders Page | 03:24 |
201 | Code Refactor #12 | 24:18 |
202 | Key Notes #12 | 01:47 |
203 | Understand Requirement and Design of Checkout Module | 05:24 |
204 | Update Shopping Cart Page | 45:06 |
205 | Code Checkout Page | 54:20 |
206 | Change Shipping Address from Checkout Page | 26:27 |
207 | Exercise: Update Settings Module | 06:18 |
208 | Code Place Order with COD | 28:43 |
209 | Code Send Order Confirmation E-mail | 39:08 |
210 | Create PayPal Accounts | 03:08 |
211 | Code Payment for Checkout Page | 47:31 |
212 | Code Validate PayPal Order | 51:27 |
213 | Review transactions of PayPal sandbox account | 01:27 |
214 | Test Checkout on Smartphone | 13:39 |
215 | Code Refactor #13 | 06:20 |
216 | Key Notes #13 | 01:58 |
217 | Understand Remaining Requirement and Design | 03:34 |
218 | Update Repository Layer for Order Module | 18:24 |
219 | Exercise: Display Order Tracking Information | 07:50 |
220 | Exercise: Code Edit Order Form (Overview and Shipping) | 11:49 |
221 | Code Edit Products in an Order | 01:01:53 |
222 | Calculate Shipping Cost of a Product | 19:11 |
223 | Code Add Products to an Order (Part 1) | 37:05 |
224 | Code Add Products to an Order (Part 2) | 46:50 |
225 | Code Remove Products from an Order | 18:31 |
226 | Exercise: Code Manage Track Records of an Order | 11:31 |
227 | Code Save Order into Database | 54:34 |
228 | Code Refactor #14 | 12:03 |
229 | Understand Requirement and Design of Order Management for Shipper | 04:03 |
230 | Code Orders Listing for Shipper | 51:52 |
231 | Code Service and Controller for Updating Order Status | 16:15 |
232 | Code View Layer for Updating Order Status | 39:23 |
233 | Test Shipper Role on Smartphone | 04:17 |
234 | Understand Requirement and Design of Order Management for Customer | 02:58 |
235 | Exercise: Code Orders Listing for Customer | 13:38 |
236 | Exercise: Code View Order Details for Customer | 09:25 |
237 | Code Return Order Function (Service and Controller) | 28:17 |
238 | Code Return Order Function (View Layer) | 43:54 |
239 | Code Refactor #15 | 05:32 |
240 | Test Managing Orders on Smartphone | 05:28 |
241 | Understand Key Concepts in Amazon S3 | 03:41 |
242 | Create AWS Account and IAM Users | 12:33 |
243 | Move Existing Image Files to Amazon S3 | 28:21 |
244 | Setup AWS SDK for Java | 06:01 |
245 | Code Amazon S3 Utility Class | 35:22 |
246 | Integrate Amazon S3 Functions | 28:48 |
247 | Key Notes #14 | 01:38 |
248 | Understand Key Concepts in Heroku | 05:13 |
249 | Create Heroku Account and Install Heroku CLI | 01:35 |
250 | Deploy a Simple App on to Heroku | 24:41 |
251 | Understand Deployment Approach for Shopme Project | 02:51 |
252 | Prepare for Deployment of Shopme Project | 11:52 |
253 | Deploy ShopmeBackEnd Project | 12:15 |
254 | Deploy MySQL Database | 25:36 |
255 | Deploy ShopmeFrontEnd Project | 17:25 |
256 | Add Custom Domain Names for Apps | 08:45 |
257 | Enable Secure Connection (https) | 07:21 |
258 | Update Social Login with Google and Facebook | 08:21 |
259 | Use Unicode for MySQL Database | 07:00 |
260 | Key Notes #15 | 01:54 |
261 | Understand Requirement and Design of Sales Report Module | 04:57 |
262 | Import Sample Data for Sales Report | 08:25 |
263 | How to Produce Sales Report | 01:31 |
264 | Code Repository Layer for Sales Report | 34:54 |
265 | Understand Google Charts Library | 07:23 |
266 | Code Sales Report by Date - Part 1 | 49:14 |
267 | Code Sales Report by Date - Part 2 | 46:29 |
268 | Code Sales Report by Date - Part 3 | 14:24 |
269 | Code Sales Report by Date - Part 4 | 28:51 |
270 | Format Currency for Sales Report | 15:12 |
271 | Code Sales Report by Category - Part 1 | 37:17 |
272 | Code Sales Report by Category - Part 2 | 41:16 |
273 | Code Sales Report by Product | 14:58 |
274 | Test Sales Report on Smartphone | 08:49 |
275 | Key Notes #16 | 01:22 |
276 | Understand Requirement and Design of Product Review Module | 05:58 |
277 | Exercise: Code Repository Layer for Review | 07:41 |
278 | Exercise: Code Review Management for Admin | 17:40 |
279 | Exercise: Code Review Management for Customer | 10:40 |
280 | Update Review Count and Average Rating of a Product | 21:39 |
281 | Show Rating Stars for Products | 23:28 |
282 | Exercise: Show Reviews in Product Detail Page | 12:35 |
283 | Exercise: Code Review Listing of a Product | 08:32 |
284 | Show Review Buttons for Products in Order Details | 34:12 |
285 | Code Write Review Function - Part 1 | 14:22 |
286 | Code Write Review Function - Part 2 | 26:58 |
287 | Code Write Review Function - Part 3 | 16:23 |
288 | Code Refactor #16 | 13:03 |
289 | Import Sample Data for Product Reviews | 04:09 |
290 | Test Product Review on Smartphone | 12:52 |
291 | Key Notes #17 | 01:41 |
292 | Understand Requirement and Design of Review Voting Module | 04:20 |
293 | Exercise: Code Repository Layer for Review Voting Module | 14:07 |
294 | Code Service Layer for Review Voting Module | 22:18 |
295 | Code Controller Layer for Review Voting Module | 29:37 |
296 | Code View Layer for Review Voting Module | 40:11 |
297 | Update Voting Status for Signed-in Customer | 17:11 |
298 | Exercise: Sort Reviews | 05:32 |
299 | Test Voting Reviews on Smartphone | 06:31 |
300 | Key Notes #18 | 01:03 |
301 | Understand Requirements of Product Question Module | 12:31 |
302 | Test Question Module | 22:08 |
303 | Solution for Assignment 1 | 00:24 |
304 | Understand Requirements of Article Management Module | 04:06 |
305 | Test Article Management Module | 08:55 |
306 | Solution for Assignment 2 | 00:25 |
Similar courses to Spring Boot E-Commerce Ultimate Course
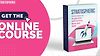
Stratospheric - From Zero to Production with Spring Boot and AWS + BOOKleanpub
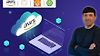
Deploy Spring Boot Microservices on AWS ECS with Fargateudemy
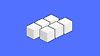
Microservices and Distributed Systemsamigoscode (Nelson Djalo)
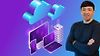
Spring Boot Microservices and Spring Cloud. Build & Deploy.udemy
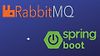
RabbitMQ : Messaging with Java, Spring Boot And Spring MVCudemy
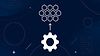
Event-Driven Microservices, CQRS, SAGA, Axon, Spring Bootudemy
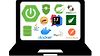
Spring 6 & Spring Boot 3 for Beginners (Includes 5 Projects)udemy
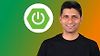
Master Spring Boot 3 & Spring Framework 6 with Javaudemy
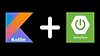
Build RESTFUL APIs using Kotlin and Spring Bootudemy
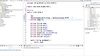