NodeJS - The Complete Guide (incl. MVC, REST APIs, GraphQL)
Join the most comprehensive Node.js course on Udemy and learn Node in both a practical as well as theory-based way! Node.js is probably THE most popular and modern server-side programming language you can dive into these days! Node.js developers are in high demand and the language is used for everything from traditional web apps with server-side rendered views over REST APIs all the way up to GraphQL APIs and real-time web services. Not to mention its applications in build workflows for projects of all sizes.
Read more about the course
This course will teach you all of that! From scratch with zero prior knowledge assumed. Though if you do bring some knowledge, you'll of course be able to quickly jump into the course modules that are most interesting to you.
Here's what you'll learn in this course:
Node.js Basics & Basic Core Modules
Parsing Requests & Sending Responses
Rendering HTML Dynamically (on the Server)
Using Express.js
Working with Files and generating PDFs on the Server (on-the-fly)
File Up- and Download
Using the Model-View-Controller (MVC) Pattern
Using Node.js with SQL (MySQL) and Sequelize
Using Node.js with NoSQL (MongoDB) and Mongoose
Working with Sessions & Cookies
User Authentication and Authorization
Sending E-Mails
Validating User Input
Data Pagination
Handling Payments with Stripe.js
Building REST APIs
Authentication in REST APIs
File Upload in REST APIs
Building GraphQL APIs
Authentication in GraphQL APIs
File Upload in GraphQL APIs
Building a Realtime Node.js App with Websockets
Automated Testing (Unit Tests)
Deploying a Node.js Application
And Way More!
Does this look like a lot of content? It certainly is!
This is not a short course but it is the "Complete Guide" on Node.js after all. We'll dive into a lot of topics and we'll not just scratch the surface.
We'll also not just walk through boring theory and some slides. Instead, we'll build two major projects: An online shop (including checkout + payments) and a blog.
All topics and features of the course will be shown and used in these projects and you'll therefore learn about them in a realistic environment.
Is this course for you?
If you got no Node.js experience, you'll love this course because it starts with zero knowledge assumed. It's the perfect course to become a Node.js developer.
If you got basic Node.js experience, this course is also a perfect match because you can go through the basic modules quickly and you'll benefit from all the deep dives and advanced topics the course covers.
Are you an advanced Node.js user? Check the curriculum then. Maybe you found no other course that shows how to use SQL with Node.js. Or you're interested in GraphQL. Chances are, that you'll get a lot of value out of this course, too!
Prerequisites
NO Node.js knowledge is required at all!
NO other programming language knowledge (besides JavaScript, see next point) is required
Basic JavaScript knowledge is assumed though - you should at least be willing to pick it up whilst going through the course. A JS refresher module exists to bring you up to the latest syntax quickly
Basic HTML + CSS knowledge helps but is NOT required
- General knowledge of how the web works is recommended but not a must-have
- Basic JavaScript knowledge is strongly recommended but could be picked up whilst going through the course
- NO NodeJS knowledge is required!
- Beginner or advanced web developers who want to dive into backend (server-side) development with NodeJS
- Everyone who's interested in building modern, scalable and high-performing web applications
- Experienced NodeJS developers who want to dive into specific features like using GraphQL with NodeJS
What you'll learn:
- Work with one of the most in-demand web development programming languages
- Build modern, fast and scalable server-side web applications with NodeJS, databases like SQL or MongoDB and more
- Understand the NodeJS ecosystem and build server-side rendered apps, REST APIs and GraphQL APIs
Watch Online NodeJS - The Complete Guide (incl. MVC, REST APIs, GraphQL)
# | Title | Duration |
---|---|---|
1 | Introduction | 01:57 |
2 | What is Node.js? | 04:43 |
3 | Installing Node.js and Creating our First App | 09:40 |
4 | Understanding the Role & Usage of Node.js | 07:33 |
5 | Course Outline | 08:04 |
6 | How To Get The Most Out Of The Course | 03:55 |
7 | Working with the REPL vs Using Files | 02:59 |
8 | Module Introduction | 01:39 |
9 | JavaScript in a Nutshell | 02:39 |
10 | Refreshing the Core Syntax | 04:38 |
11 | let & const | 02:30 |
12 | Understanding Arrow Functions | 05:22 |
13 | Working with Objects, Properties & Methods | 03:24 |
14 | Arrays & Array Methods | 04:20 |
15 | Arrays, Objects & Reference Types | 02:17 |
16 | Understanding Spread & Rest Operators | 06:47 |
17 | Destructuring | 05:41 |
18 | Async Code & Promises | 10:34 |
19 | Wrap Up | 00:52 |
20 | Module Introduction | 01:51 |
21 | How The Web Works | 04:12 |
22 | Creating a Node Server | 13:23 |
23 | The Node Lifecycle & Event Loop | 04:54 |
24 | Understanding Requests | 03:11 |
25 | Sending Responses | 05:38 |
26 | Routing Requests | 05:49 |
27 | Redirecting Requests | 04:12 |
28 | Parsing Request Bodies | 11:13 |
29 | Understanding Event Driven Code Execution | 06:01 |
30 | Blocking and Non-Blocking Code | 05:05 |
31 | Node.js - Looking Behind the Scenes | 12:02 |
32 | Using the Node Modules System | 10:06 |
33 | Wrap Up | 05:16 |
34 | Module Introduction | 00:41 |
35 | Understanding NPM Scripts | 07:04 |
36 | Installing 3rd Party Packages | 08:21 |
37 | Using Nodemon for Autorestarts | 01:37 |
38 | Understanding different Error Types | 01:50 |
39 | Finding & Fixing Syntax Errors | 03:11 |
40 | Dealing with Runtime Errors | 02:48 |
41 | Logical Errors | 06:33 |
42 | Using the Debugger | 03:11 |
43 | Restarting the Debugger Automatically After Editing our App | 06:22 |
44 | Changing Variables in the Debug Console | 01:27 |
45 | Wrap Up | 03:15 |
46 | Module Introduction | 02:16 |
47 | What is Express.js? | 03:44 |
48 | Installing Express.js | 03:48 |
49 | Adding Middleware | 05:14 |
50 | How Middleware Works | 02:59 |
51 | Express.js - Looking Behind the Scenes | 03:43 |
52 | Handling Different Routes | 05:00 |
53 | Parsing Incoming Requests | 08:01 |
54 | Limiting Middleware Execution to POST Requests | 01:49 |
55 | Using Express Router | 08:05 |
56 | Adding a 404 Error Page | 02:31 |
57 | Filtering Paths | 03:39 |
58 | Creating HTML Pages | 05:10 |
59 | Serving HTML Pages | 07:20 |
60 | Returning a 404 Page | 02:01 |
61 | Using a Helper Function for Navigation | 03:38 |
62 | Styling our Pages | 13:59 |
63 | Serving Files Statically | 07:50 |
64 | Wrap Up | 03:37 |
65 | Module Introduction | 02:32 |
66 | Sharing Data Across Requests & Users | 08:06 |
67 | Templating Engines - An Overview | 04:41 |
68 | Installing & Implementing Pug | 11:05 |
69 | Outputting Dynamic Content | 11:03 |
70 | Converting HTML Files to Pug | 05:56 |
71 | Adding a Layout | 05:36 |
72 | Finishing the Pug Template | 05:51 |
73 | Working with Handlebars | 05:09 |
74 | Converting our Project to Handlebars | 07:42 |
75 | Adding the Layout to Handlebars | 08:42 |
76 | Working with EJS | 08:20 |
77 | Working on the Layout with Partials | 09:34 |
78 | Wrap Up | 03:47 |
79 | [OPTIONAL] Assignment Solution | 25:03 |
80 | Module Introduction | 00:53 |
81 | What is the MVC? | 02:49 |
82 | Adding Controllers | 09:21 |
83 | Finishing the Controllers | 02:38 |
84 | Adding a Product Model | 08:06 |
85 | Storing Data in Files Via the Model | 09:40 |
86 | Fetching Data from Files Via the Model | 03:56 |
87 | Refactoring the File Storage Code | 04:26 |
88 | Wrap Up | 02:16 |
89 | Module Introduction | 01:22 |
90 | Creating the Shop Structure | 05:35 |
91 | Working on the Navigation | 02:50 |
92 | Registering the Routes | 11:05 |
93 | Storing Product Data | 05:16 |
94 | Displaying Product Data | 03:03 |
95 | Editing & Deleting Products | 04:48 |
96 | Adding Another Item | 02:09 |
97 | Module Introduction | 01:08 |
98 | Preparations | 01:24 |
99 | Adding the Product ID to the Path | 04:35 |
100 | Extracting Dynamic Params | 04:33 |
101 | Loading Product Detail Data | 04:53 |
102 | Rendering the Product Detail View | 05:27 |
103 | Passing Data with POST Requests | 07:20 |
104 | Adding a Cart Model | 12:30 |
105 | Using Query Params | 07:55 |
106 | Pre-Populating the Edit Product Page with Data | 06:47 |
107 | Linking to the Edit Page | 02:21 |
108 | Editing the Product Data | 08:59 |
109 | Adding the Product-Delete Functionality | 05:35 |
110 | Deleting Cart Items | 08:12 |
111 | Displaying Cart Items on the Cart Page | 08:46 |
112 | Deleting Cart Items | 05:25 |
113 | Fixing a Delete Product Bug | 01:32 |
114 | Wrap Up | 01:47 |
115 | Module Introduction | 01:34 |
116 | Choosing a Database | 04:18 |
117 | NoSQL Introduction | 04:21 |
118 | Comparing SQL and NoSQL | 05:07 |
119 | Setting Up MySQL | 07:17 |
120 | Connecting our App to the SQL Database | 06:51 |
121 | Basic SQL & Creating a Table | 04:08 |
122 | Retrieving Data | 03:01 |
123 | Fetching Products | 06:31 |
124 | Fetching Products - Time to Practice | 01:05 |
125 | Inserting Data Into the Database | 04:13 |
126 | Fetching a Single Product with the "where" Condition | 02:58 |
127 | Wrap Up | 01:25 |
128 | Module Introduction | 01:27 |
129 | What is Sequelize? | 02:36 |
130 | Connecting to the Database | 03:58 |
131 | Defining a Model | 05:48 |
132 | Syncing JS Definitions to the Database | 04:30 |
133 | Inserting Data & Creating a Product | 04:50 |
134 | Retrieving Data & Finding Products | 03:02 |
135 | Getting a Single Product with the "where" Condition | 04:31 |
136 | Fetching Admin Products | 01:26 |
137 | Updating Products | 05:19 |
138 | Deleting Products | 02:49 |
139 | Creating a User Model | 02:49 |
140 | Adding a One-To-Many Relationship | 05:55 |
141 | Creating & Managing a Dummy User | 06:02 |
142 | Using Magic Association Methods | 03:43 |
143 | Fetching Related Products | 02:47 |
144 | One-To-Many & Many-To-Many Relations | 06:04 |
145 | Creating & Fetching a Cart | 05:46 |
146 | Adding New Products to the Cart | 06:43 |
147 | Adding Existing Products & Retrieving Cart Items | 04:56 |
148 | Deleting Related Items & Deleting Cart Products | 02:25 |
149 | Adding an Order Model | 04:20 |
150 | Storing Cartitems as Orderitems | 08:20 |
151 | Resetting the Cart & Fetching and Outputting Orders | 09:54 |
152 | Wrap Up | 01:50 |
153 | Module Introduction | 01:18 |
154 | What is MongoDB? | 03:58 |
155 | Relations in NoSQL | 03:59 |
156 | Setting Up MongoDB | 04:49 |
157 | Installing the MongoDB Driver | 07:02 |
158 | Creating the Database Connection | 03:26 |
159 | Finishing the Database Connection | 04:22 |
160 | Using the Database Connection | 05:15 |
161 | Creating Products | 02:09 |
162 | Understanding the MongoDB Compass | 02:39 |
163 | Fetching All Products | 04:35 |
164 | Fetching a Single Product | 07:46 |
165 | Making the "Edit" & "Delete" Buttons Work Again | 02:22 |
166 | Working on the Product Model to Edit our Product | 07:15 |
167 | Finishing the "Update Product" Code | 03:58 |
168 | One Note About Updating Products | 01:48 |
169 | Deleting Products | 03:31 |
170 | Fixing the "Add Product" Functionality | 01:29 |
171 | Creating New Users | 07:01 |
172 | Storing the User in our Database | 05:41 |
173 | Working on Cart Items & Orders | 07:14 |
174 | Adding the "Add to Cart" Functionality | 06:15 |
175 | Storing Multiple Products in the Cart | 07:02 |
176 | Displaying the Cart Items | 09:21 |
177 | Fixing a Bug | 01:03 |
178 | Deleting Cart Items | 04:03 |
179 | Adding an Order | 04:37 |
180 | Adding Relational Order Data | 06:22 |
181 | Getting Orders | 03:21 |
182 | Removing Deleted Items From the Cart | 02:59 |
183 | Wrap Up | 02:20 |
184 | Module Introduction | 01:24 |
185 | What is Mongoose? | 02:05 |
186 | Connecting to the MongoDB Server with Mongoose | 04:48 |
187 | Creating the Product Schema | 06:02 |
188 | Saving Data Through Mongoose | 06:11 |
189 | Fetching All Products | 02:28 |
190 | Fetching a Single Product | 01:25 |
191 | Updating Products | 04:15 |
192 | Deleting Products | 01:20 |
193 | Adding and Using a User Model | 06:37 |
194 | Using Relations in Mongoose | 03:45 |
195 | One Important Thing About Fetching Relations | 03:55 |
196 | Working on the Shopping Cart | 05:26 |
197 | Loading the Cart | 05:13 |
198 | Deleting Cart Items | 02:46 |
199 | Creating & Getting Orders | 09:57 |
200 | Storing All Order Related Data | 01:53 |
201 | Clearing the Cart After Storing an Order | 02:00 |
202 | Getting & Displaying the Orders | 03:41 |
203 | Wrap Up | 01:38 |
204 | Module Introduction | 00:46 |
205 | What is a Cookie? | 01:59 |
206 | The Current Project Status | 02:19 |
207 | Optional: Creating the Login Form | 06:23 |
208 | Adding the Request Driven Login Solution | 07:41 |
209 | Setting a Cookie | 05:56 |
210 | Manipulating Cookies | 01:59 |
211 | Configuring Cookies | 05:44 |
212 | What is a Session? | 02:59 |
213 | Initializing the Session Middleware | 02:44 |
214 | Using the Session Middleware | 04:15 |
215 | Using MongoDB to Store Sessions | 06:00 |
216 | Sessions & Cookies - A Short Summary | 01:54 |
217 | Deleting a Cookie | 04:36 |
218 | Fixing Some Minor Bugs | 04:28 |
219 | Making "Add to Cart" Work Again | 06:46 |
220 | Two Tiny Improvements | 03:12 |
221 | Wrap Up | 03:44 |
222 | Module Introduction | 01:23 |
223 | What is Authentication? | 02:26 |
224 | How is Authentication Implemented? | 02:06 |
225 | Our Updated Project Status | 02:26 |
226 | Implementing an Authentication Flow | 07:35 |
227 | Encrypting Passwords | 04:37 |
228 | Adding a Tiny Code Improvement | 01:22 |
229 | Adding the Signin Functionality | 06:22 |
230 | Working on Route Protection | 02:13 |
231 | Using Middleware to Protect Routes | 04:39 |
232 | Understanding CSRF Attacks | 03:24 |
233 | Using a CSRF Token | 06:57 |
234 | Adding CSRF Protection | 04:24 |
235 | Fixing the Order Button | 01:17 |
236 | Providing User Feedback | 07:11 |
237 | Optional: Styling Error Messages | 03:33 |
238 | Finishing the Flash Messages | 02:21 |
239 | Adding Additional Flash Messages | 02:21 |
240 | Wrap Up | 02:33 |
241 | Module Introduction | 00:47 |
242 | How Does Sending Emails Work? | 01:35 |
243 | Using SendGrid | 01:25 |
244 | Using Nodemailer to Send an Email | 05:10 |
245 | Potential Limitation for Large Scale Apps | 01:30 |
246 | Module Introduction | 00:43 |
247 | Resetting Passwords | 03:04 |
248 | Implementing the Token Logic | 07:42 |
249 | Creating the Token | 01:28 |
250 | Creating the Reset Password Form | 05:28 |
251 | Adding Logic to Update the Password | 06:36 |
252 | Why we Need Authorization | 01:59 |
253 | Adding Authorization | 02:08 |
254 | Adding Protection to Post Actions | 03:44 |
255 | Why Editing Fails | 01:07 |
256 | Wrap Up | 01:46 |
257 | Module Introduction | 01:04 |
258 | Why Should We Use Validation? | 02:29 |
259 | How to Validate Input? | 04:05 |
260 | Setup & Basic Validation | 10:45 |
261 | Using Validation Error Messages | 02:12 |
262 | Built-In & Custom Validators | 03:56 |
263 | More Validators | 04:27 |
264 | Checking For Field Equality | 03:15 |
265 | Adding Async Validation | 04:47 |
266 | Keeping User Input | 04:12 |
267 | Adding Conditional CSS Classes | 05:30 |
268 | Adding Validation to Login | 05:37 |
269 | Sanitizing Data | 03:42 |
270 | Validating Product Addition | 11:41 |
271 | Validating Product Editing | 06:58 |
272 | Wrap Up | 01:24 |
273 | Module Introduction | 00:46 |
274 | Types of Errors & Error Handling | 05:15 |
275 | Analyzing the Error Handling in the Current Project | 02:23 |
276 | Errors - Some Theory | 06:44 |
277 | Throwing Errors in Code | 02:43 |
278 | Returning Error Pages | 06:55 |
279 | Using the Express.js Error Handling Middleware | 05:48 |
280 | Updating the App | 03:10 |
281 | Using the Error Handling Middleware Correctly | 04:55 |
282 | Status Codes | 05:58 |
283 | Wrap Up | 02:22 |
284 | Module Introduction | 00:55 |
285 | Adding a File Picker to the Frontend | 03:07 |
286 | Handling Multipart Form Data | 05:16 |
287 | Handling File Uploads with Multer | 04:52 |
288 | Configuring Multer to Adjust Filename & Filepath | 04:30 |
289 | Filtering Files by Mimetype | 02:29 |
290 | Storing File Data in the Database | 07:43 |
291 | Serving Images Statically | 04:26 |
292 | Downloading Files with Authentication | 07:34 |
293 | Setting File Type Headers | 02:36 |
294 | Restricting File Access | 03:20 |
295 | Streaming Data vs Preloading Data | 03:21 |
296 | Using PDFKit for .pdf Generation | 05:33 |
297 | Generating .pdf Files with Order Data | 06:51 |
298 | Deleting Files | 05:58 |
299 | Fixing Invoice Links | 00:36 |
300 | Wrap Up | 01:32 |
301 | Module Introduction | 00:49 |
302 | Adding Pagination Links | 04:09 |
303 | Retrieving a Chunk of Data | 03:48 |
304 | Preparing Pagination Data on the Server | 03:53 |
305 | Adding Dynamic Pagination Buttons | 08:08 |
306 | Re-Using the Pagination Logic & Controls | 03:42 |
307 | Wrap Up | 01:03 |
308 | Module Introduction | 00:50 |
309 | What are Async Requests? | 02:08 |
310 | Adding Client Side JS Code | 07:25 |
311 | Sending & Handling Background Requests | 09:27 |
312 | Manipulating the DOM | 03:02 |
313 | Module Introduction | 00:59 |
314 | How Payments Work | 02:05 |
315 | Adding a Checkout Page | 06:01 |
316 | Using Stripe in Your App | 19:24 |
317 | Module Introduction | 01:26 |
318 | What are REST APIs and why do we use Them? | 07:02 |
319 | Accessing Data with REST APIs | 05:42 |
320 | Understanding Routing & HTTP Methods | 05:26 |
321 | REST APIs - The Core Principles | 04:10 |
322 | Creating our REST API Project & Implementing the Route Setup | 06:42 |
323 | Sending Requests & Responses and Working with Postman | 13:29 |
324 | REST APIs, Clients & CORS Errors | 10:34 |
325 | Sending POST Requests | 06:33 |
326 | Wrap Up | 02:15 |
327 | Module Introduction | 01:11 |
328 | REST APIs & The Rest Of The Course | 04:00 |
329 | Understanding the Frontend Setup | 04:19 |
330 | Planning the API | 03:03 |
331 | Fetching Lists of Posts | 06:20 |
332 | Adding a Create Post Endpoint | 07:37 |
333 | Adding Server Side Validation | 06:20 |
334 | Setting Up a Post Model | 05:15 |
335 | Storing Posts in the Database | 03:33 |
336 | Static Images & Error Handling | 06:54 |
337 | Fetching a Single Post | 07:49 |
338 | Uploading Images | 08:57 |
339 | Updating Posts | 14:03 |
340 | Deleting Posts | 04:18 |
341 | Adding Pagination | 06:21 |
342 | Adding a User Model | 04:09 |
343 | Adding User Signup Validation | 06:30 |
344 | Signing Users Up | 07:25 |
345 | How Does Authentication Work? | 03:11 |
346 | Starting with User Login | 03:52 |
347 | Logging In & Creating JSON Web Tokens (JWTs) | 07:54 |
348 | Using & Validating the Token | 09:44 |
349 | Adding Auth Middleware to All Routes | 01:53 |
350 | Connecting Posts & Users | 06:14 |
351 | Adding Authorization Checks | 03:51 |
352 | Clearing Post-User Relations | 02:55 |
353 | Wrap Up | 02:29 |
354 | Module Introduction | 00:59 |
355 | What is Async Await All About? | 04:09 |
356 | Transforming "Then Catch" to "Async Await" | 04:27 |
357 | Top-level "await" | 01:19 |
358 | Wrap Up | 03:04 |
359 | Module Introduction | 00:56 |
360 | What Are Websockets & Why Would You Use Them? | 04:18 |
361 | Websocket Solutions - An Overview | 01:36 |
362 | Setting Up Socket.io on the Server | 03:57 |
363 | Establishing a Connection From the Client | 02:28 |
364 | Identifying Realtime Potential | 02:43 |
365 | Sharing the IO Instance Across Files | 02:47 |
366 | Synchronizing POST Additions | 07:15 |
367 | Fixing a Bug - The Missing Username | 01:45 |
368 | Updating Posts On All Connected Clients | 03:59 |
369 | Sorting Correctly | 01:01 |
370 | Deleting Posts Across Clients | 02:32 |
371 | Wrap Up | 01:49 |
372 | Module Introduction | 00:58 |
373 | What is GraphQL? | 09:18 |
374 | Understanding the Setup & Writing our First Query | 11:16 |
375 | Defining a Mutation Schema | 05:43 |
376 | Adding a Mutation Resolver & GraphiQL | 09:43 |
377 | Adding Input Validation | 04:20 |
378 | Handling Errors | 03:46 |
379 | Connecting the Frontend to the GraphQL API | 06:50 |
380 | Adding a Login Query & a Resolver | 06:21 |
381 | Adding Login Functionality | 04:58 |
382 | Adding a Create Post Mutation | 07:24 |
383 | Extracting User Data From the Auth Token | 05:49 |
384 | Sending the "Create Post" Query | 04:47 |
385 | Fixing a Bug & Adding New Posts Correctly | 02:54 |
386 | Adding a "Get Post" Query & Resolver | 04:54 |
387 | Sending "Create Post" and "Get Post" Queries | 05:14 |
388 | Adding Pagination | 04:29 |
389 | Uploading Images | 10:27 |
390 | Viewing a Single Post | 06:08 |
391 | Updating Posts | 09:33 |
392 | Deleting Posts | 09:26 |
393 | Managing the User Status | 09:18 |
394 | Using Variables | 16:07 |
395 | Fixing a Pagination Bug | 02:49 |
396 | Wrap Up | 03:37 |
397 | Module Introduction | 01:17 |
398 | Deploying Different Kinds of Apps | 01:40 |
399 | Deployment Preparations | 04:53 |
400 | Using Environment Variables | 11:13 |
401 | Using Production API Keys | 01:20 |
402 | Setting Secure Response Headers with Helmet | 02:42 |
403 | Compressing Assets | 02:34 |
404 | Setting Up Request Logging | 03:35 |
405 | Setting Up a SSL Server | 09:56 |
406 | Using a Hosting Provider | 04:26 |
407 | Understanding the Project & the Git Setup | 04:04 |
408 | A Deployment Example with Heroku | 10:27 |
409 | Deploying APIs | 02:53 |
410 | Module Introduction | 00:44 |
411 | What is Testing? | 02:43 |
412 | Why & How? | 03:26 |
413 | Setup and Writing a First Test | 09:41 |
414 | Testing the Auth Middleware | 12:34 |
415 | Organizing Multiple Tests | 04:13 |
416 | What Not To Test! | 08:03 |
417 | Using Stubs | 08:39 |
418 | Testing Controllers | 08:04 |
419 | Testing Asynchronous Code | 05:54 |
420 | Setting up a Testing Database | 06:34 |
421 | Testing Code With An Active Database | 06:34 |
422 | Cleaning Up | 03:30 |
423 | Hooks | 04:35 |
424 | Testing Code That Requires Authentication | 12:38 |
425 | Wrap Up & Mastering Tests | 04:23 |
426 | Module Introduction | 01:02 |
427 | npm & Node.js | 01:43 |
428 | Using npm | 07:53 |
429 | What is a Build Tool? | 05:02 |
430 | Using Node.js in Build Processes | 05:51 |
431 | Module Introduction | 02:29 |
432 | What is this Module About? | 02:20 |
433 | Working with ES Modules & Node | 09:34 |
434 | More on ES Modules | 06:27 |
435 | Node Core Modules & Promises | 05:19 |
436 | Module Introduction | 01:23 |
437 | TypeScript: What & Why? | 06:03 |
438 | TypeScript Setup | 04:10 |
439 | Assigning Types | 03:30 |
440 | Type Inference & Type Casting | 06:11 |
441 | Configuring TypeScript | 05:02 |
442 | Working with Union Types | 04:24 |
443 | Using Object & Array Types | 06:18 |
444 | Working with Type Aliases & Interfaces | 03:23 |
445 | Understanding Generics | 05:10 |
446 | A First Summary | 00:35 |
447 | Node & TypeScript: Setup | 02:15 |
448 | Getting Started with Node and TypeScript | 09:32 |
449 | Writing TypeScript Express.js Code | 04:00 |
450 | Adding REST Routes with TypeScript | 06:43 |
451 | Finishing the REST Routes | 05:54 |
452 | Testing the API | 04:53 |
453 | Using Type Casting | 04:22 |
454 | Moving to a Better Project Structure | 03:45 |
455 | Wrap Up | 02:51 |
456 | Module Introduction | 01:36 |
457 | What is Deno? | 03:10 |
458 | Why Deno? | 01:56 |
459 | Deno Setup | 05:51 |
460 | Writing First Deno Code | 04:19 |
461 | The Deno Runtime (Namespace) API | 03:14 |
462 | Using the Runtime API | 05:27 |
463 | Working with Deno Permissions | 04:32 |
464 | Repeating the Example with Node | 04:17 |
465 | How Deno Features Are Organized | 04:29 |
466 | Using the Standard Library | 08:31 |
467 | Creating a Webserver | 02:55 |
468 | Using the Oak Framework with Deno | 09:49 |
469 | An Example Node REST API | 12:16 |
470 | Re-building the REST API with Deno | 16:19 |
471 | Should You Switch From Node to Deno? | 05:06 |
472 | Module Introduction | 01:01 |
473 | App Setup | 04:07 |
474 | Handling CORS Errors | 07:17 |
475 | Connecting Deno to MongoDB | 08:06 |
476 | Using the MongoDB Client Module | 10:35 |
477 | Finishing the Deno MongoDB CRUD Operations | 05:30 |
478 | Wrap Up | 01:11 |
479 | Course Roundup | 02:41 |
Similar courses to NodeJS - The Complete Guide (incl. MVC, REST APIs, GraphQL)
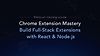
Chrome Extension Mastery: Build Full-Stack Extensions with React & Node.jsRyan Fitzgerald
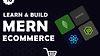
MERN Stack From ScratchBrad Traversy
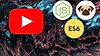
Youtube cloneNomad Coders
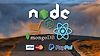
React Node FullStack - Ecommerce from Scratch to Deploymentudemy
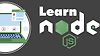
Learn Nodewesbos
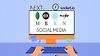
MERN Stack React, Socket io, Next.js Express,MongoDb, Nodejsudemy
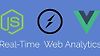
Real-time chat with Node.js, Socket.io and Vue.jsCodecourse
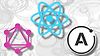
Full-Stack React with GraphQL and Apollo Boostudemy
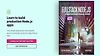