The Modern GraphQL Bootcamp (with Node.js and Apollo)
GraphQL is quickly changing how Node.js developers write their APIs and backend applications. It offers a much-needed replacement to the traditional REST HTTP API. It’s by far the most exciting change we’ve seen to Node.js development in a long time. Goodbye Express. Hello GraphQL. Who should learn GraphQL? Anyone building backend applications with Node.js!
Read more about the course
In this class, you’ll learn how to use GraphQL to replace the standard Express HTTP API, as well as support features like authentication, real-time applications, and more. GraphQL can be used with any client that has internet access, whether it’s a web app, mobile app, or server-side application.
Big and small companies, from Twitter and Facebook to Yelp and Twitch, are switching from frameworks like Express to GraphQL.
I designed this class to give you the skills and real-world experience needed to build and launch your own GraphQL apps.
To kick the class off, we’ll answer a few important questions. What is GraphQL? Why is it useful? How is GraphQL going to help me build better applications with Node.js?
Next, you’ll master the fundamentals of GraphQL by building out a blogging application. You’ll learn about GraphQL schemas, data types, queries, mutations, subscriptions, and many other fantastic GraphQL features.
From there, you’ll set up advanced features like authentication, database storage, production deployment, automated test suites, and more.
Throughout the course you’ll learn how to:
Model your application data using schemas
Create queries and subscriptions allowing clients to access data in the database
Create mutations allowing clients to create and change data in the database
Query and change your data from the browser by making requests with Apollo Client
Work with the Prisma ORM (v1) to communicate with your database via a GraphQL API
Deploy your GraphQL applications to production
Secure your application data with an authentication system
Write an automated test suite for your application
Learn GraphQL by Writing Code
This course was designed to be interactive, with more than 80 challenges along the way to get you writing code and solving problems on your own. This will give you the real-world skills and experience needed to write GraphQL applications once you’re done with the class.
Everything you need comes in one easy-to-use package.
There’s no need to worry whether you’re learning the right skills to land that GraphQL job or launch that GraphQL app. I’ve mapped out everything you need to know in an interactive, easy-to-follow package designed to get you up and running in a couple of weeks.
Get access to fast support if you get stuck.
There’s nothing worse than getting stuck ten hours into a course and not getting the help you need to continue. Getting stuck is part of the learning process. That’s why I’m here to answer every single question.
I guarantee this is the most up-to-date and engaging GraphQL course available, and it comes with a Udemy 30-day money-back guarantee.
The first videos are available as a free preview, so I’ll see you inside the class!
- Andrew Mead
Requirements:
Development: A basic understanding of Node and JavaScript is required.
Hardware: A computer with an internet connection (Windows, macOS, or Ubuntu).
- This course is for anyone who wants to learn how to build advanced Node applications.
- This course is for anyone interested in keeping their Node skills up-to-date.
What you'll learn:
- Learn and master GraphQL by building real-world Node applications.
- Use Prisma v1 to store and access data from a production database.
- Use Apollo Client to communicate with GraphQL from your web app.
- Learn how to deploy and test your GraphQL applications.
- Test your skills and gain confidence by completing more than 80 coding challenges.
- Get access to a free 110-page PDF guide with lecture notes, code samples, and documentation links.
Watch Online The Modern GraphQL Bootcamp (with Node.js and Apollo)
# | Title | Duration |
---|---|---|
1 | Welcome to the Class! | 05:28 |
2 | Grab the PDF Guide | 01:08 |
3 | Why GraphQL? | 13:09 |
4 | Installing Node.js and VSC | 03:16 |
5 | Section Intro: GraphQL Basics: Schemas and Queries | 00:31 |
6 | What is a Graph? | 06:11 |
7 | GraphQL Queries | 13:30 |
8 | Nested GraphQL Queries | 12:19 |
9 | Setting up Babel | 11:29 |
10 | ES6 Import/Export | 16:21 |
11 | Creating Your Own GraphQL API | 17:33 |
12 | GraphQL Scalar Types | 13:16 |
13 | Live Reload for GraphQL-Yoga | 04:44 |
14 | Creating Custom Types | 14:51 |
15 | Operation Arguments | 13:41 |
16 | Working with Arrays: Part I | 10:50 |
17 | Working with Arrays: Part II | 22:19 |
18 | Relational Data: Basics | 14:58 |
19 | Relational Data: Arrays | 06:24 |
20 | Comment Challenge: Part I | 06:28 |
21 | Comment Challenge: Part II | 08:42 |
22 | Comment Challenge: Part III | 12:44 |
23 | Section Intro: GraphQL Basics: Mutations | 00:40 |
24 | Creating Data with Mutations: Part I | 17:34 |
25 | Creating Data with Mutations: Part II | 20:29 |
26 | The Object Spread Operator with Node.js | 06:45 |
27 | The Input Type | 13:18 |
28 | Deleting Data with Mutations: Part I | 16:35 |
29 | Deleting Data with Mutations: Part II | 13:23 |
30 | A Pro GraphQL Project Structure: Part I | 17:22 |
31 | A Pro GraphQL Project Structure: Part II | 08:34 |
32 | Updating Data with Mutations: Part I | 11:52 |
33 | Updating Data with Mutations: Part II | 15:51 |
34 | Section Intro: GraphQL Basics: Subscriptions | 01:14 |
35 | GraphQL Subscription Basics | 15:25 |
36 | Setting up a Comments Subscription | 10:33 |
37 | Setting up a Posts Subscription | 07:48 |
38 | Expanding the Posts Subscription for Edits and Deletions | 19:39 |
39 | Expanding the Comments Subscription for Edits and Deletions | 09:56 |
40 | Enums | 08:51 |
41 | Section Intro: Database Storage with Prisma | 01:15 |
42 | What is Prisma? | 08:44 |
43 | Prisma Mac Setup | 13:05 |
44 | Prisma Windows Setup | 15:29 |
45 | Prisma Ubuntu Setup | 16:50 |
46 | Prisma 101 | 17:17 |
47 | Exploring the Prisma GraphQL API | 12:55 |
48 | Add Post type to Prisma | 17:55 |
49 | Adding Comment Type to Prisma | 11:40 |
50 | Integrating Prisma into a Node.js Project | 17:13 |
51 | Using Prisma Bindings | 13:21 |
52 | Mutations with Prisma Bindings | 15:05 |
53 | Using Async/Await with Prisma Bindings | 17:35 |
54 | Checking If Data Exists Using Prisma Bindings | 15:20 |
55 | Customizing Type Relationships | 13:09 |
56 | Modeling a Review System with Prisma: Set Up | 10:34 |
57 | Modeling a Review System with Prisma: Solution | 16:50 |
58 | Section Intro: Authentication with GraphQL | 01:16 |
59 | Adding Prisma into GraphQL Queries | 15:44 |
60 | Integrating Operation Arguments | 14:18 |
61 | Refactoring Custom Type Resolvers | 09:14 |
62 | Adding Prisma into GraphQL Mutations | 14:08 |
63 | Adding Prisma into GraphQL Update Mutations: Part I | 13:27 |
64 | Adding Prisma into GraphQL Update Mutations: Part II | 16:26 |
65 | Adding Prisma into GraphQL Subscriptions | 19:13 |
66 | Closing Prisma to the Outside World | 08:07 |
67 | Allowing for Generated Schemas | 08:31 |
68 | Storing Passwords | 11:27 |
69 | Creating Auth Tokens with JSON Web Tokens | 20:20 |
70 | Logging in Existing Users | 16:13 |
71 | Validating Auth Tokens | 16:29 |
72 | Locking Down Mutations (Users) | 13:23 |
73 | Locking Down Mutations (Posts and Comments) | 15:42 |
74 | Locking Down Queries: Part I | 19:20 |
75 | Locking Down Queries: Part II | 09:34 |
76 | Locking Down Individual Type Fields | 11:27 |
77 | Fragments | 18:48 |
78 | Cleaning up Some Edge Cases | 10:48 |
79 | Locking Down Subscriptions | 10:14 |
80 | Token Expiration | 11:20 |
81 | Password Updates | 08:35 |
82 | Section Intro: Pagination and Sorting with GraphQL | 01:19 |
83 | Pagination | 11:06 |
84 | Pagination Using Cursors | 09:46 |
85 | Working with createdAt and updatedAt | 10:20 |
86 | Sorting Data | 16:28 |
87 | Section Intro: Production Deployment | 00:45 |
88 | Creating a Prisma Service | 14:24 |
89 | Prisma Configuration and Deployment | 11:10 |
90 | Exploring the Production Prisma Instance | 06:07 |
91 | Node.js Production App Deployment: Part I | 13:39 |
92 | Node.js Production App Deployment: Part II | 18:42 |
93 | Node.js Production Environment Variables | 18:15 |
94 | Section Intro: Apollo Client and Testing GraphQL | 01:01 |
95 | Setting up a Test Environment | 03:54 |
96 | Installing and Exploring Jest | 11:21 |
97 | Testing and Assertions | 20:49 |
98 | Apollo Client in the Browser: Part I | 06:59 |
99 | Apollo Client in the Browser: Part II | 17:04 |
100 | Configuring Jest to Start the GraphQL Server | 14:50 |
101 | Testing Mutations | 13:07 |
102 | Seeding the Database with Test Data | 11:35 |
103 | Testing Queries | 08:47 |
104 | Expecting GraphQL Operations to Fail | 12:12 |
105 | Supporting Multiple Test Suites and Authentication | 13:04 |
106 | Testing with Authentication: Part I | 17:33 |
107 | Testing with Authentication: Part II | 17:41 |
108 | GraphQL Variables: Part I | 18:34 |
109 | GraphQL Variables: Part II | 09:28 |
110 | Testing Comments | 19:06 |
111 | Testing Subscriptions | 22:52 |
112 | Test Case Ideas | 03:09 |
113 | Section Intro: Creating a Boilerplate Project | 01:13 |
114 | Creating a Boilerplate Project | 16:47 |
115 | Using the Boilerplate Project | 10:27 |
116 | Section Intro: Wrapping Up | 00:47 |
117 | A New App Idea | 05:05 |
118 | Bonus: Where Do I Go from Here? | 03:14 |
Similar courses to The Modern GraphQL Bootcamp (with Node.js and Apollo)
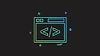
Learn Web Scraping with NodeJs in 2020 - The Crash Courseudemy
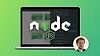
Node.js, Express, MongoDB & More The Complete Bootcamp 2023udemy
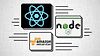
React Node AWS - Build infinitely Scaling MERN Stack Appudemy
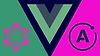
Full-Stack Vue with GraphQL - The Ultimate Guideudemy
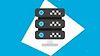
Mastering REST APIs in Node.js: Zero To Heroudemy
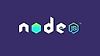
Node.js: The Complete Guide to Build RESTful APIscodewithmosh (Mosh Hamedani)
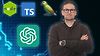