Web Development with Google’s Go (golang) Programming Language
The Go programming language was created by Google to do what Google does: performant web applications at scale. Open-sourced in 2009 and reaching version one in 2012, the Go programming language is the best choice for web development programming today. Ruby on Rails, Python, Django, Node.js, PHP, and ASP all fall short. Go is the most powerful, performant, and scalable programming language today for creating web applications, web API’s, microservices, and other distributed services.
Read more about the course
In this course, you will gain a solid foundation in web development. You will learn all of the following and more:
Architecture
- networking architecture
- the client / server architecture
- the request / response pattern
- the RFC standards defined by the IETF
- the format of requests from clients and responses from servers
Templates
- the role that templates play in server-side programming
- how to work with templates from Go’s standard library
- modifying data structures to work well with templates
Servers
- the relationship between TCP and HTTP
- how to build a TCP server which responds to HTTP requests
- how to create a TCP server which acts as an in-memory database
- how to create a restful TCP server that handles various routes and methods
- the difference between a web server, a servemux, a multiplexer, and a mux
- how to use a third-party server such as julien schmidt’s router
- the importance of HTTP methods and status codes
The net/http package
- streamlining your web development with the net/http package
- the nuances of the net/http package
- the handler interface
- http.ListenAndServe
- creating your own servemux
- using the default servemux
- http.Handle & http.Handler
- http.Handlefunc, func(ResponseWriter, *Request), & http.HandlerFunc
- http.ServeContent, http.ServeFile, & http.FileServer
- http.StripPrefix
- http.NotFoundHandler
State & Sessions
- how to create state: UUID’s, cookies, values in URL’s, security
- how to create sessions: login, permissions, logout
- how to expire a session
Deployment
- how to purchase a domain
- how to deploy an application to Google Cloud
Amazon Web Services
- how to use Amazon Web Services (AWS)
- how to create a virtual linux machine on AWS EC2 (Elastic Cloud Compute)
- how to use secure shell (SSH) to manage a virtual machine
- how to use secure copy (SCP) to transfer files to a virtual machine
- what load balancers are and how to use them on AWS
MySQL
- how to use MySQL on AWS
- how to connect a MySQL workbench to AWS
MongoDB
- understanding CRUD
- how to use MongoDB & Go
MVC (Model View Controller) Design Pattern
- understanding the MVC design pattern
- using the MVC design pattern
Docker
- virtual machines vs containers
- understanding the benefits of using Docker
- Docker images, Docker containers, and Docker registries
- implementing Docker and Go
- deploying Docker and Go
Google Cloud
- Google Cloud Storage
- Google Cloud no-sql datastore
- Google Cloud memcache
- Google Cloud PAAS App Engine
Web Dev Toolkit
- AJAX
- JSON
- json.Marhsal & json.Unmarshal
- json.Encode & json.Decode
- Hash message authentication code (HMAC)
- Base64 encoding
- Web storage
- Context
- TLS & HTTPS
- JSON with Go using Tags
Building Applications
- a photo blog
- a twitter clone
By the end of this course, you will have mastered the fundamentals of web development.
My name is Todd McLeod. I am tenured faculty in Computer Information Technology at Fresno City College and adjunct faculty in Computer Science at California State University Fresno. I have taught enough students over 20 years to know that by the end of this course, you will be an outstanding web developer.
You will have the best skills available today.
You will know the best way to do web development today.
You will have the hottest, most demanded, and highest paid skills in the marketplace.
Join me in this outstanding course. Come learn best practices for web development. Sign up for this course now and open doors to a great future.
Who is the target audience?
- This is a university level introduction to web programming course.
- This course is for individuals who know how to use the Go programming language.
- This course is perfect for programmers wanting a thorough introduction to web development using the Go programming language.
- This course is perfect for developers wanting to fill in gaps in their knowledge.
Watch Online Web Development with Google’s Go (golang) Programming Language
# | Title | Duration |
---|---|---|
1 | Why Go For Web Dev | 07:40 |
2 | Prerequisites | 01:36 |
3 | Resources | 02:33 |
4 | Language review | 18:31 |
5 | How to succeed | 08:13 |
6 | Understanding templates | 03:28 |
7 | Templating with concatenation | 06:26 |
8 | Understanding package text/template: parsing & executing templates | 16:53 |
9 | Passing data into templates | 03:58 |
10 | Variables in templates | 02:29 |
11 | Passing composite data structures into templates | 14:58 |
12 | Functions in templates | 15:12 |
13 | Pipelines in templates | 09:55 |
14 | Predefined global functions in templates | 06:11 |
15 | Nesting templates - modularizing your code | 07:52 |
16 | Passing data into templates & composition | 05:39 |
17 | Using methods in templates | 03:38 |
18 | Hands-on exercises | 01:59 |
19 | Using package html/template, character escaping, & cross-site scripting | 03:37 |
20 | Understanding servers | 10:09 |
21 | TCP server - write to connection | 06:56 |
22 | TCP server - read from connection using bufio.Scanner | 10:34 |
23 | TCP server - read from & write to connection | 04:26 |
24 | TCP server - code a client | 04:48 |
25 | TCP server - rot13 & in-memory database | 09:57 |
26 | TCP server - HTTP request / response foundation hands-on exercise | 06:57 |
27 | TCP server - HTTP method & URI retrieval hands-on exercise | 05:04 |
28 | TCP server - HTTP multiplexer | 05:04 |
29 | net/http package - an overview | 09:22 |
30 | Understanding & using ListenAndServe | 05:23 |
31 | Foundation of net/http: Handler, ListenAndServe, Request, ResponseWriter | 08:27 |
32 | Retrieving form values - exploring *http.Request | 15:08 |
33 | Retrieving other request values - exploring *http.Request | 08:11 |
34 | Exploring http.ResponseWriter - writing headers to the response | 07:36 |
35 | Review | 07:14 |
36 | Understanding ServeMux | 16:27 |
37 | Disambiguation: func(ResponseWriter, *Request) vs. HandlerFunc | 08:03 |
38 | Third-party servemux - Julien Schmidt’s router | 08:47 |
39 | Hands-on exercises | 01:15 |
40 | Hands-on exercises - solutions #1 | 04:21 |
41 | Hands-on exercises - solutions #2 | 04:21 |
42 | Serving a file with io.Copy | 19:57 |
43 | Serving a file with http.ServeContent & http.ServeFile | 05:03 |
44 | Serving a file with http.FileServer | 03:54 |
45 | Serving a file with http.FileServer & http.StripPrefix | 03:50 |
46 | Creating a static file server with http.FileServer | 06:01 |
47 | log.Fatal & http.Error | 03:11 |
48 | Hands-on exercises | 04:01 |
49 | Hands-on exercises - solutions | 01:00 |
50 | The http.NotFoundHandler | 05:52 |
51 | Buying a domain - Google domains | 05:48 |
52 | Deploying to Google Cloud | 02:17 |
53 | State overview | 07:53 |
54 | Passing values through the URL | 01:20 |
55 | Passing values from forms | 06:23 |
56 | Uploading a file, reading the file, creating a file on the server | 05:30 |
57 | Enctype | 11:55 |
58 | Redirects - overview | 01:01 |
59 | Redirects - diagrams & documentation | 06:33 |
60 | Redirects - in practice | 07:56 |
61 | Cookies - overview | 07:44 |
62 | Cookies - writing and reading | 03:32 |
63 | Writing multiple cookies & hands-on exercise | 05:21 |
64 | Hands-on exercise solution: creating a counter with cookies | 02:12 |
65 | Deleting a cookie | 03:20 |
66 | Sessions | 06:38 |
67 | Universally unique identifier - UUID | 07:27 |
68 | Your first session | 08:32 |
69 | Sign-up | 12:00 |
70 | Encrypt password with bcrypt | 06:01 |
71 | Login | 05:21 |
72 | Logout | 07:08 |
73 | Permissions | 04:05 |
74 | Expire session | 03:21 |
75 | Overview | 08:26 |
76 | Creating a virtual server instance on AWS EC2 | 07:21 |
77 | Hello world on AWS | 10:51 |
78 | Persisting an application | 12:02 |
79 | Hands-on Exercise | 04:43 |
80 | Hands-on Solution | 02:14 |
81 | Terminating AWS services | 16:00 |
82 | Overview | 01:32 |
83 | Installing MySQL - Locally | 06:25 |
84 | Installing MySQL - AWS | 02:45 |
85 | Connect Workbench to MySQL on AWS | 06:29 |
86 | Go & SQL - Setup | 04:40 |
87 | Go & SQL - In Practice | 04:38 |
88 | Overview of load balancers | 07:44 |
89 | Create EC2 security groups | 03:39 |
90 | Create an ELB load balancer | 06:32 |
91 | Implementing the load balancer | 08:00 |
92 | Connecting to your MySQL server using MySQL workbench | 13:49 |
93 | Hands-on exercise | 05:12 |
94 | Hands-on solution | 01:06 |
95 | Autoscaling & CloudFront | 18:52 |
96 | Starting files | 12:43 |
97 | User data | 04:33 |
98 | Storing Multiple Values | 05:45 |
99 | Uploading pictures | 03:37 |
100 | Displaying pictures | 08:52 |
101 | Hash message authentication code (HMAC) | 01:26 |
102 | Base64 encoding | 04:29 |
103 | Web storage | 03:07 |
104 | Context | 05:31 |
105 | TLS & HTTPS | 13:19 |
106 | JSON - JavaScript Object Notation | 09:46 |
107 | Go & JSON - Marshal & Encode | 04:31 |
108 | Unmarshal JSON with Go | 09:48 |
109 | Unmarshal JSON with Go using Tags | 10:28 |
110 | Hands-on exercise solution | 10:01 |
111 | AJAX introduction | 02:09 |
112 | AJAX server side | 11:50 |
113 | Organizing code into packages | 12:13 |
114 | Create user & delete user | 10:13 |
115 | MVC design pattern - model view controller | 04:29 |
116 | Install mongodb | 06:37 |
117 | Connect to mongodb | 04:58 |
118 | CRUD with Go & mongodb | 05:29 |
119 | Hands on exercise & solution | 08:52 |
120 | Hands on exercise & solution | 05:24 |
121 | Hands on exercise & solution | 06:20 |
122 | Introduction to Docker | 02:39 |
123 | Virtual machines & containers | 02:40 |
124 | Installing docker | 09:54 |
125 | Docker whalesay example | 05:19 |
126 | Using a Dockerfile to build an image | 08:05 |
127 | Launching a container running curl | 12:48 |
128 | Running a Go web app in a Docker container | 04:50 |
129 | Pushing & pulling to docker hub | 05:23 |
130 | Go, Docker & Amazon Web Services (AWS) | 08:56 |
131 | Installing Postgres | 09:13 |
132 | Create database | 07:33 |
133 | Create table | 05:35 |
134 | Insert records | 03:23 |
135 | Auto increment primary key | 00:49 |
136 | Hands-on exercise | 02:32 |
137 | Hands-on exercise - solution | 05:43 |
138 | Relational databases | 07:07 |
139 | Query - cross join | 06:29 |
140 | Query - inner join | 09:57 |
141 | Query - three table inner join | 05:03 |
142 | Query - outer joins | 01:08 |
143 | Clauses | 00:43 |
144 | Update a record | 04:46 |
145 | Delete a record | 07:38 |
146 | Users - create, grant, alter, remove | 08:59 |
147 | Go & Postgres | 04:57 |
148 | Select query | 04:56 |
149 | Web app | 07:29 |
150 | Query Row | 04:06 |
151 | Insert record | 01:30 |
152 | Update record | 11:24 |
153 | Delete record | 11:33 |
154 | Code organization | 10:11 |
155 | NoSQL | 10:53 |
156 | MongoDB | 06:58 |
157 | Installing mongo | 03:53 |
158 | Database | 06:58 |
159 | Collection | 03:53 |
160 | Document | 03:50 |
161 | Find (aka, query) | 06:15 |
162 | Update | 06:59 |
163 | Remove | 02:00 |
164 | Projection | 02:45 |
165 | Limit | 01:05 |
166 | Sort | 02:52 |
167 | Index | 01:24 |
168 | Aggregation | 04:33 |
169 | Users | 09:20 |
170 | JSON | 04:41 |
171 | Create Read Update Delete (CRUD) | 16:59 |
Similar courses to Web Development with Google’s Go (golang) Programming Language
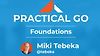
Practical Go Foundationsardanlabs.com
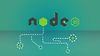
NodeJS - The Complete Guide (incl. MVC, REST APIs, GraphQL)udemyAcademind Pro
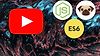
Youtube cloneNomad Coders
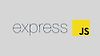
Just Express (with a bunch of node and http). In detail.udemy
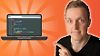
Build Fullstack Trello clone: WebSocket, Socket IOudemy
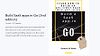
Build SaaS apps in Go (2nd edition)Dominic St-Pierre
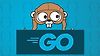
Getting Started With GolangAcademind Pro
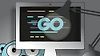
Go Bootcamp: Master Golang with 1000+ Exercises and Projectsudemy
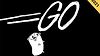