Node.js: The Complete Guide to Build RESTful APIs
Node.js, or Node, is a runtime environment for executing JavaScript code outside of a browser. It is ideal for building highly-scalable, data-intensive backend services (APIs) that power your client’s apps (web or mobile apps).
Read more about the course
Why learn Node?
Node is great for prototyping and agile development as well as building super fast and highly scalable apps; Companies like Uber and PayPal use Node in production to build applications because it requires fewer people and less code. Plus, Node has the largest ecosystem of open-source library, so you don’t have to build everything from scratch.
A step-by-step, A to Z course
What you’ll get when you sign up for this course:
15 hours of HD videos, complete with exercises and solutions
A real-world project: you'll build the back-end for a video rental application, not a dummy to-do app!
No more wasted time on lengthy courses or out-of-date tutorials
Up-to-date and practical information and solutions (no fluff!)
The opportunity to learn at your own pace - lifetime access - so take your time if you prefer
Expert tips to become a Node rockstar
The best practices and common pitfalls to avoid
Watch on any device, online or offline - mobile friendly and downloadable lessons
Certificate of completion to present to your employer
You’ll learn to:
Confidently build RESTful services (APIs) using Node.js, Express.js, and MongoDB
Employ the best practices for Node.js
Avoid common mistakes
What we’ll cover:
Node module system
Node Package Manager (NPM)
Asynchronous JavaScript
Useful ES6+ features
Implementing CRUD operations
Storing complex data in MongoDB
Data Validation
Authentication and authorization
Handling and logging errors the right way
Unit and integration testing
Test-driven development (TDD)
Deployment
This course is for you if:
You’re a back-end developer who is used to working with frameworks like ASP.NET, Rails, Django, etc. You want to add Node.js to your toolbox.
You’re a front-end developer and want to transition to full-stack development.
You’ve tried other Node.js tutorials and found them to be too slow, out-of-date, and boring!
Having Node.js on your resume helps you find more jobs and make more money.
And here is what other students say:
"Absolutely the best! Highly recommended if you want to get started on Node.js from zero. I have learned what Node is and what it can do. Truly comprehensive. Perfect rating! Mosh knows his stuff and he deserves your support. On a side note, my current company uses Mosh's courses as a huge resource and reference when training / refreshing knowledge. I just want to say thank you! Please don't stop teaching. You were born with the talent to teach." -Eugene John Arellano
"Mosh is one of the top 3 instructors for modern web development. He explains difficult concepts with ease. I recommend all of his courses because of the amount of detail and his style of teaching." -Warren Isaac
"What I like most about the course is Mosh's methodology and the way how he explains the things. Very well structured course with high quality of presentation as well." -Omar Amrani
Requirements:
- Basic familiarity with JavaScript
- You’re a back-end developer familiar with frameworks like ASPNET, Rails, Django, etc. Now, you want to add Node to your toolbox.
- You’re a front-end developer and want to transition to full-stack development.
- Having Node on your resume helps you find more jobs and make more money.
What you'll learn:
- Build the backend for your web and mobile apps
- Use modern JavaScript features (ES6, ES7)
- Implement CRUD operations
- Handle and log errors, the right way
- Write unit and integration tests
- Practice test-driven development (TDD)
- Store and retrieve complex data in MongoDB
- Implement authentication and authorization
- Deploy your Node apps to production
- Apply the best practices for building fast, scalable and secure apps
Watch Online Node.js: The Complete Guide to Build RESTful APIs
# | Title | Duration |
---|---|---|
1 | Welcome | 00:31 |
2 | What is Node | 03:00 |
3 | Node Architecture | 03:02 |
4 | How Node Works | 04:24 |
5 | Installing Node | 02:32 |
6 | Your First Node Program | 02:20 |
7 | Course Structure | 02:14 |
8 | Introduction | 00:29 |
9 | Global Object | 03:22 |
10 | Modules | 03:36 |
11 | Creating a Module | 04:44 |
12 | Loading a Module | 05:23 |
13 | Module Wrapper Function | 04:41 |
14 | Path Module | 04:09 |
15 | OS Module | 04:19 |
16 | File System Module | 04:51 |
17 | Events Module | 06:18 |
18 | Event Arguments | 03:09 |
19 | Extending Event Emitter | 08:02 |
20 | HTTP Module | 07:25 |
21 | Introduction | 03:42 |
22 | Package.json | 02:55 |
23 | Installing a Node Package | 03:34 |
24 | Using a Package | 03:21 |
25 | Package Dependencies | 03:19 |
26 | NPM Packages and Source Control | 03:52 |
27 | Semantic Versioning | 04:30 |
28 | Listing the Installed Packages | 02:11 |
29 | Viewing Registry Info for a Package | 02:46 |
30 | Installing a Specific Version of a Package | 01:06 |
31 | Updating Local Packages | 05:03 |
32 | DevDependencies | 01:52 |
33 | Uninstalling a Package | 00:41 |
34 | Working with Global Packages | 02:34 |
35 | Publishing a Package | 04:33 |
36 | Updating a Published Package | 01:56 |
37 | Introduction | 00:48 |
38 | RESTful Services | 05:58 |
39 | Introducing Express | 02:20 |
40 | Building Your First Web Server | 05:46 |
41 | Nodemon | 01:31 |
42 | Environment Variables | 03:14 |
43 | Route Parameters | 03:23 |
44 | Handling HTTP GET Requests | 04:46 |
45 | Handling HTTP POST Requests | 03:43 |
46 | Calling Endpoints Using Postman | 02:08 |
47 | Input Validation | 07:59 |
48 | Handling HTTP PUT Requests | 08:31 |
49 | Handling HTTP Delete Requests | 04:45 |
50 | Project- Build the Genres API | 01:19 |
51 | Introducion | 00:23 |
52 | MIddleware | 02:49 |
53 | Creating Custom Middleware | 04:24 |
54 | Built-In Middleware | 03:58 |
55 | Third-party Middleware | 03:56 |
56 | Environments | 04:07 |
57 | Configuration | 09:46 |
58 | Debugging | 06:55 |
59 | Templating Engines | 04:56 |
60 | Database Integration | 01:37 |
61 | Authentication | 00:30 |
62 | Structuring Express Applications | 07:45 |
63 | Project- Restructure the App | 03:06 |
64 | Synchronous Vs. Asynchronous Code | 05:52 |
65 | Patterns For Dealing With Asynchronous Code | 03:08 |
66 | Callbacks | 06:00 |
67 | Callback Hell | 02:29 |
68 | Named Functions to Rescue | 04:42 |
69 | Promises | 08:38 |
70 | Replacing Callbacks with Promises | 03:32 |
71 | Consuming Promises | 05:32 |
72 | Creating Settled Promises | 02:50 |
73 | Running Parallel Promises | 06:15 |
74 | Async and Await | 06:56 |
75 | Exercise | 05:57 |
76 | Introducing MongoDB | 02:01 |
77 | Installing MongoDB on Mac | 03:59 |
78 | Installing MongoDB on Windows | 05:40 |
79 | Connecting to MongoDB | 03:53 |
80 | Schemas | 03:49 |
81 | Models | 04:19 |
82 | Saving a Document | 03:34 |
83 | Querying Documents | 04:19 |
84 | Comparison Query Operators | 05:00 |
85 | Logical Query Operators | 02:02 |
86 | Regular Expressions | 03:21 |
87 | Counting | 00:50 |
88 | Pagination | 01:37 |
89 | Exercise 1 | 07:58 |
90 | Exercise 2 | 04:35 |
91 | Exercise 3 | 03:20 |
92 | Updating Documents- Query First | 03:36 |
93 | Updating a Document- Update First | 06:15 |
94 | Removing Documents | 02:39 |
95 | Validation | 07:00 |
96 | Built-In Validators | 04:56 |
97 | Custom Validators | 03:17 |
98 | Async Validators | 02:36 |
99 | Validation Errors | 03:38 |
100 | SchemaType Options | 05:57 |
101 | Project- Add Persistence to Genres API | 14:06 |
102 | Project- Build the Customers API | 07:00 |
103 | Restructuring the Project | 06:15 |
104 | Modelling Relationships | 07:46 |
105 | Referencing Documents | 03:52 |
106 | Population | 04:17 |
107 | Embedding Documents | 06:55 |
108 | Using an Array of Sub-documents | 04:32 |
109 | Project- Build the Movies API | 07:06 |
110 | Project- Build the Rentals API | 08:02 |
111 | Transactions | 08:46 |
112 | ObjectID | 07:04 |
113 | Validating Object ID's | 06:14 |
114 | A Better Implementation | 02:24 |
115 | Introduction | 04:02 |
116 | Creating the User Model | 03:41 |
117 | Registering Users | 07:54 |
118 | Using Lodash | 05:22 |
119 | Hashing Passwords | 06:55 |
120 | Authenticating Users | 04:55 |
121 | Testing the Authentication | 02:43 |
122 | JSON Web Tokens | 05:05 |
123 | Generating Authentication Tokens | 03:19 |
124 | Storing Secrets in Environment Variables | 06:13 |
125 | Setting Response Headers | 03:47 |
126 | Encapsulating Logic in Mongoose Models | 07:12 |
127 | Authorization Middleware | 06:51 |
128 | Protecting Routes | 03:07 |
129 | Getting the Current User | 04:04 |
130 | Logging Out Users | 02:05 |
131 | Role Based Authorization | 05:44 |
132 | Testing the Authorization | 04:11 |
133 | Introduction | 03:03 |
134 | Handling Rejected Promises | 03:08 |
135 | Express Error Middleware | 04:41 |
136 | Removing Try_Catch docs | 08:30 |
137 | Express Async Errors | 03:19 |
138 | Logging Errors | 06:37 |
139 | Logging to MongoDB | 04:12 |
140 | Uncaught Exceptions | 03:36 |
141 | Unhandled Promise Rejections | 07:24 |
142 | Error Handling Recap | 02:27 |
143 | Refactoring Index.js- Extracting Routes | 04:55 |
144 | Extracting the DB Logic | 03:23 |
145 | Logging | 02:12 |
146 | Extracting the Config Logic | 02:31 |
147 | Extracting the Validation Logic | 01:54 |
148 | Showing Unhandled Exceptions on the Console | 01:30 |
149 | What is Automated Testing? | 02:41 |
150 | Benefits of Automated Testing | 02:38 |
151 | Types of Tests | 04:01 |
152 | Test Pyramid | 02:56 |
153 | Tooling | 02:16 |
154 | Writing Your First Unit Test | 05:01 |
155 | Testing Numbers | 06:37 |
156 | Grouping Tests | 01:52 |
157 | Refactoring with Confidence | 02:44 |
158 | Testing Strings | 03:25 |
159 | Testing Arrays | 05:51 |
160 | Testing Objects | 05:31 |
161 | Testing Exceptions | 07:26 |
162 | Continually Running Tests | 01:44 |
163 | Exercise- Testing the FizzBuzz | 05:29 |
164 | Creating Simple Mock Functions | 05:38 |
165 | Interaction Testing | 05:01 |
166 | Jest Mock Functions | 07:17 |
167 | What to Unit Test | 03:07 |
168 | Exercise | 07:40 |
169 | Introduction | 01:10 |
170 | Preparing the App | 02:19 |
171 | Setting Up the Test DB | 03:02 |
172 | Your First Integration Test | 05:45 |
173 | Populating the Test DB | 06:18 |
174 | Testing Routes with Parameters | 03:20 |
175 | Validating Object ID's | 03:32 |
176 | Refactoring with Confidence | 02:05 |
177 | Testing the Authorization | 02:40 |
178 | Testing Invalid Inputs | 05:33 |
179 | Testing the Happy Paths | 02:36 |
180 | Writing Clean Tests | 08:41 |
181 | Testing the Auth Middleware | 09:46 |
182 | Unit Testing the Auth Middleware | 06:15 |
183 | Code Coverage | 07:10 |
184 | Exercise | 00:27 |
185 | What is Test-driven Development? | 02:55 |
186 | Implementing the Returns | 02:34 |
187 | Test Cases | 02:54 |
188 | Populating the Database | 07:15 |
189 | Testing the Authorization | 07:23 |
190 | Testing the Input | 03:43 |
191 | Refactoring Tests | 04:19 |
192 | Looking Up an Object | 03:05 |
193 | Testing if Rental Processed | 02:14 |
194 | Testing the Valid Request | 02:24 |
195 | Testing the Return Date | 04:16 |
196 | Testing the Rental Fee | 04:51 |
197 | Testing the Movie Stock | 05:38 |
198 | Testing the Response | 03:37 |
199 | Refactoring the Validation Logic | 06:45 |
200 | Mongoose Static Methods | 06:13 |
201 | Refactoring the Domain Logic | 04:05 |
202 | Introduction | 01:22 |
203 | Preparing the App for Production | 02:29 |
204 | Getting Started With Heroku | 02:16 |
205 | Preparing the App for Deployment | 01:56 |
206 | Adding the Code to a Git Repository | 02:45 |
207 | Deploying to Heroku | 03:45 |
208 | Viewing Logs | 02:47 |
209 | Setting Environment Variables | 02:45 |
210 | MongoDB in the Cloud | 08:24 |
Similar courses to Node.js: The Complete Guide to Build RESTful APIs
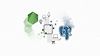
Node, SQL, & PostgreSQL - Mastering Backend Web Developmentudemy
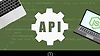
Node.js API Masterclass With Express & MongoDBudemyBrad Traversy
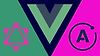
Full-Stack Vue with GraphQL - The Ultimate Guideudemy
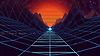
MERN STACK 2022 - Build Ultimate CMS (WordPress Clone)udemy
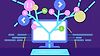
100 Days Of Code: The Complete Web Development Bootcamp 2024Academind Pro
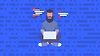
Full-Stack Web Developer Bootcamp with Real Projectsudemy
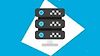
Mastering REST APIs in Node.js: Zero To Heroudemy
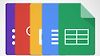
Build Collaborative editor and Real-time video call with NodeJSCode4Startup (coderealprojects)
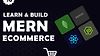