Recursion, Backtracking and Dynamic Programming in Java
This course is about the fundamental concepts of algorithmic problems focusing on recursion, backtracking, dynamic programming and divide and conquer approaches. As far as I am concerned, these techniques are very important nowadays, algorithms can be used (and have several applications) in several fields from software engineering to investment banking or R&D.
Read more about the course
Section 1 - RECURSION
what are recursion and recursive methods
stack memory and heap memory overview
what is stack overflow?
Fibonacci numbers
factorial function
tower of Hanoi problem
Section 2 - SEARCH ALGORITHMS
linear search approach
binary search algorithm
Section 3 - SELECTION ALGORITHMS
what are selection algorithms?
how to find the k-th order statistics in O(N) linear running time?
quickselect algorithm
median of medians algorithm
the secretary problem
Section 4 - BACKTRACKING
what is backtracking?
n-queens problem
Hamiltonian cycle problem
coloring problem
knight's tour problem
Sudoku game
Section 5 - DYNAMIC PROGRAMMING
what is dynamic programming?
knapsack problem
rod cutting problem
subset sum problem
Section 6 - OPTIMAL PACKING
what is optimal packing?
bin packing problem
Section 7 - DIVIDE AND CONQUER APPROACHES
what is the divide and conquer approach?
dynamic programming and divide and conquer method
how to achieve sorting in O(NlogN) with merge sort?
the closest pair of points problem
Section 8 - COMMON INTERVIEW QUESTIONS
top interview questions (Google, Facebook and Amazon)
In each section we will talk about the theoretical background for all of these algorithms then we are going to implement these problems together from scratch in Java.
Finally, YOU CAN LEARN ABOUT THE MOST COMMON INTERVIEW QUESTIONS (Google, MicroSoft, Amazon etc.)
Watch Online Recursion, Backtracking and Dynamic Programming in Java
# | Title | Duration |
---|---|---|
1 | Introduction | 02:09 |
2 | What are stack and heap memory? | 03:45 |
3 | Stack memory and heap memory simulation | 06:15 |
4 | Recursion introduction | 08:44 |
5 | Adding numbers: iteration vs recursion | 05:05 |
6 | Recursion and stack memory (stack overflow) | 10:10 |
7 | Head recursion and tail recursion implementation | 06:39 |
8 | Factorial function - head recursion | 06:07 |
9 | Factorial problem - visualizing the stack | 04:15 |
10 | Factorial function - tail recursion | 05:48 |
11 | Fibonacci numbers - head recursion | 04:58 |
12 | Towers of Hanoi problem introduction | 06:01 |
13 | Tower of Hanoi problem implementation | 05:39 |
14 | Towers of Hanoi - visualizing the stack | 07:09 |
15 | Iteration and recursion revisited | 01:43 |
16 | What is linear search? | 01:39 |
17 | Linear search implementation | 03:17 |
18 | What is binary (logarithmic) search? | 03:49 |
19 | Binary search implementation | 08:54 |
20 | Selection algorithms introduction | 06:44 |
21 | Quickselect introduction - Hoare algorithm | 09:49 |
22 | Quickselect visualization | 08:52 |
23 | Quickselect implementation | 11:57 |
24 | What the problem with pivots? | 05:50 |
25 | Advanced selection - median of medians algorithm | 07:31 |
26 | Combining algorithms - introselect algorithm | 01:23 |
27 | Online selection - the secretary problem | 08:02 |
28 | Backtracking introduction | 05:51 |
29 | Brute-force search and backtracking | 04:03 |
30 | N-queens problem introduction | 08:07 |
31 | What is the search tree? | 03:10 |
32 | N-queens problem implementation I | 09:22 |
33 | N-queens problem implementation II | 06:17 |
34 | N-queens problem and stack memory visualization | 06:22 |
35 | Hamiltonian paths (and cycles) introduction | 08:03 |
36 | Hamiltonian cycle illustration | 04:42 |
37 | Hamiltonian cycle implementation I | 10:17 |
38 | Hamiltonian cycle implementation II | 07:02 |
39 | Coloring problem introduction | 08:49 |
40 | Coloring problem visualization | 04:33 |
41 | Coloring problem implementation I | 07:18 |
42 | Coloring problem implementation II | 05:25 |
43 | Knight's tour introduction | 03:55 |
44 | Knight's tour implementation I | 10:33 |
45 | Knight's tour implementation II | 04:49 |
46 | Maze problem introduction | 04:59 |
47 | Maze problem implementation I | 07:22 |
48 | Maze problem implementation II | 06:53 |
49 | Sudoku introduction | 05:52 |
50 | Sudoku implementation I | 08:38 |
51 | Sudoku implementation II | 03:28 |
52 | What is the issue with NP-complete problems? | 05:05 |
53 | Dynamic programming introduction | 08:24 |
54 | Fibonacci numbers introduction | 03:31 |
55 | Fibonacci numbers implementation | 08:36 |
56 | Knapsack problem introduction | 18:08 |
57 | Knapsack problem example | 12:59 |
58 | Knapsack problem implementation I | 08:08 |
59 | Knapsack problem implementation II | 04:27 |
60 | Rod cutting problem introduction | 08:04 |
61 | Rod cutting problem example | 11:07 |
62 | Rod cutting problem implementation | 08:46 |
63 | Subset sum problem introduction | 10:12 |
64 | Subset sum problem example | 09:09 |
65 | Subset sum problem implementation | 09:05 |
66 | Bin packing problem introduction | 08:10 |
67 | Bin packing problem implementation | 06:53 |
68 | What are divide-and-conquer approaches? | 09:24 |
69 | Binary search revisited | 01:46 |
70 | Merge sort theory | 08:18 |
71 | Merge sort implementation | 06:27 |
72 | Closest pair of points problem introduction I | 15:21 |
73 | Closes pair of points problem introduction II | 04:15 |
74 | Closest pair of points implementation | 23:54 |
75 | Palindrome problem solution | 08:01 |
76 | Integer reversion solution | 07:01 |
77 | Two eggs problem solution I | 08:02 |
78 | Two eggs problem solution II | 11:40 |
79 | Duplicates in an array problem solution | 07:57 |
80 | Anagram problem solution | 03:52 |
81 | Largest sum subarray problem solution | 10:49 |
82 | What is Algorhyme? | 00:42 |
Similar courses to Recursion, Backtracking and Dynamic Programming in Java
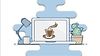
The complete guide to running Java in Docker and Kubernetesudemy
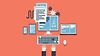
Hibernate and Java Persistence API (JPA) Fundamentalsudemy
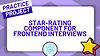
Crack the Frontend Interview with Reactzerotomastery.io
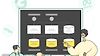
System Design InterviewByteByteGo
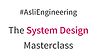
The System Design MasterclassArpit Bhayani
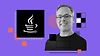
Java Data Structures & Algorithms + LEETCODE Exercisesudemy
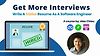
Get More Interviews: Write A Stellar Resume As A Software EngineerAlex Chiou
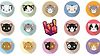
Catsrockthejvm.com
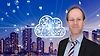
Software Architecture & Design of Modern Large Scale Systemsudemy
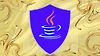