Java from Zero to First Job, Practical Guide, 2000+ examples
80h 4m 49s
English
Paid
Welcome to the fundamental and the most complete course 'Java from Zero to First Job'. As it's declared in the course name, it's created for people who want to learn programming from scratch. You need to know how to turn on your computer, no other knowledge is required for this course :) The rest will be covered in lessons.
Watch Online Java from Zero to First Job, Practical Guide, 2000+ examples
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Why Java and why this course? What is JDK, JRE and JVM? | 08:18 |
2 | Communication plan | 04:11 |
3 | Unlimited access to 'Learn IT' application for students | 03:18 |
4 | JDK Installation | 08:19 |
5 | First application: Hello World App | 12:47 |
6 | Homework Solution: .bat file creation | 02:06 |
7 | Integrated Development Environment, Eclipse Overview | 12:24 |
8 | Comments in Java source code | 07:00 |
9 | Primitive types and variables | 15:20 |
10 | Number Systems | 06:30 |
11 | Arrays | 13:26 |
12 | Packages: creation and package presentation in eclipse | 01:54 |
13 | Plugins: how to install free plugins, eclipse marketplace, workspace styles | 02:09 |
14 | Code Refactoring in Eclipse | 06:58 |
15 | Operators in Java | 20:00 |
16 | Operations with integers and floating-point numbers, BigDecimal type | 04:38 |
17 | Math class, NaN, Infinity | 05:13 |
18 | Type of the expression in Java | 04:14 |
19 | Compare primitive and reference types & Java Memory Model | 09:25 |
20 | Read user input from console | 02:04 |
21 | Homework review: Operators and Operations | 09:26 |
22 | String: object creation and main methods overview | 05:46 |
23 | String comparison: How to compare Strings and what is String Pool | 03:51 |
24 | Escape Sequences in Java | 02:49 |
25 | String Formatting | 02:53 |
26 | Regular expressions in Java | 05:36 |
27 | Homework review: String | 02:04 |
28 | 'if - else' construction | 03:21 |
29 | Homework review: 'if' statement | 01:58 |
30 | 'switch' construction | 04:02 |
31 | Homework review: 'switch' statement | 00:44 |
32 | Random numbers generation | 01:55 |
33 | 'while' loop | 01:58 |
34 | 'do-while' loop | 01:39 |
35 | 'for' with condition loop | 02:46 |
36 | 'for each' loop | 01:46 |
37 | Nested loops | 00:55 |
38 | Jump Statements - Break and Continue | 02:13 |
39 | Labels in Java | 02:37 |
40 | Homework review: Iteration Statements (Loops) | 01:16 |
41 | Methods in Java: Overview | 04:47 |
42 | Parameter Passing Mechanism in Java | 05:18 |
43 | Recursive methods | 02:50 |
44 | Variable Length Arguments | 02:59 |
45 | Homework review: Methods | 01:11 |
46 | Enumerations in Java | 07:09 |
47 | How to debug Java programs | 06:55 |
48 | Object-oriented programming: Basics | 14:16 |
49 | Classes & Objects | 13:58 |
50 | Different types of Classes, Abstract keyword and Abstract classes | 10:17 |
51 | Interfaces | 12:04 |
52 | Inheritance | 10:46 |
53 | Polymorphism and 'final' keyword | 09:19 |
54 | 'static' keyword | 07:09 |
55 | Encapsulation | 06:38 |
56 | Object, JNI and Object class overview | 09:36 |
57 | SOLID principles overview & Single Responsibility Principle | 07:29 |
58 | Open / Closed Principle | 07:29 |
59 | Liskov Substitution Principle | 05:09 |
60 | Interface Segregation Principle | 04:48 |
61 | Dependency Inversion Principle | 05:52 |
62 | Exam and homework for OOP topic | 09:27 |
63 | Version Control Systems & Git - Overview | 07:37 |
64 | How to Install Git and Basic Git Configuration | 09:41 |
65 | Git repo init, First commit and main bracnh | 09:12 |
66 | Git ignoring & Git log | 10:19 |
67 | Git undoing things & Vi text editor | 08:58 |
68 | Git remote repositories | 10:21 |
69 | SSH Connection | 08:39 |
70 | Git Branching | 16:55 |
71 | Pull Requests & Merge Requests | 11:13 |
72 | Updating local repository (fetch, merge, pull) & Team development demo | 12:59 |
73 | Merge Conflicts | 13:50 |
74 | Git Rebasing & Force Update of remote repository | 16:49 |
75 | Git Interactive Rebase | 09:02 |
76 | Git reset | 11:11 |
77 | Git stash | 12:12 |
78 | Restoring lost snapshots in Git: git reflog | 06:53 |
79 | Git cherry-pick: moving commits between branches | 11:00 |
80 | Git and Eclipse integration | 14:47 |
81 | Exception Handling in Java | 26:25 |
82 | Java Collections Framework: Overview | 09:17 |
83 | Collection Interface | 10:23 |
84 | Interfaces List, Set and Queue: Overview | 08:21 |
85 | List implementations - ArrayList, Vector, CopyOnWriteArrayList & Stack | 19:15 |
86 | LinkedList VS ArrayList, Big O Notation & Homework | 22:49 |
87 | Comparator and Comparable | 16:39 |
88 | Iterable, Iterator & ListIterator | 19:24 |
89 | Queue and Deque | 19:26 |
90 | Map Hierarchy | 15:37 |
91 | Hash Tables & HashMap | 22:27 |
92 | LinkedHashMap | 08:28 |
93 | SortedMap, NavigableMap & TreeMap | 13:48 |
94 | Set Implementations (HashSet practice) & java.util.Colelctions class | 16:05 |
95 | Generics in Java | 24:28 |
96 | Functional Programming in Java: Overview | 13:31 |
97 | Functional Interface, Lambda Functions & Method References | 14:34 |
98 | Function & BiFunction: Theory & Practice | 17:18 |
99 | Consumer, BiConsumer, Predicate & BiPredicate | 16:25 |
100 | Stream API with Practical Exercises | 24:26 |
101 | Input and Output Streams in Java | 33:40 |
102 | Serialization and Cloning | 19:21 |
103 | Multithreading Overview | 13:42 |
104 | First Multithreading Program: Thread & Runnable | 25:33 |
105 | Thread Scheduler, Race Condition, Daemon Threads & Thread Groups | 19:27 |
106 | Synchronization Basics | 28:13 |
107 | Memory Management in Multithreading Programs | 21:24 |
108 | Deadlock & Livelock | 12:01 |
109 | Executor Services, Callable & Future | 17:31 |
110 | Executor Service Implementations & ThreadFactory | 25:47 |
111 | Fork/Join Framework | 17:27 |
112 | CompletableFuture | 25:25 |
113 | Lock API - Lock, ReadWriteLock, ReentrantLock, ReentrantReadWriteLock, StampedLo | 35:04 |
114 | Synchronizers - CyclicBarrier, CountDownLatch, Semaphore, Exchanger & Phaser | 30:50 |
115 | Homework: Multithreading | 06:06 |
116 | Time Standards (GMT, UTC), Date and Calendar from java.util | 24:53 |
117 | java.time package (Instant, LocalDateTime, ZonedDateTime, OffsetDateTime etc) | 33:26 |
118 | Java Reflection API | 24:02 |
119 | Annotations | 20:09 |
120 | StringBuilder & StringBuffer | 14:31 |
121 | java.util.Optional - Optional in Java | 25:57 |
122 | Modules in Java: Modular Application Example | 35:28 |
123 | Migration of Java Apps: Example of migration to a modular application | 30:21 |
124 | Testing for software engineers: Overview | 25:06 |
125 | Unit Testing & JUnit Overview | 24:07 |
126 | JUnit 5 examples VS JUnit 4 + Equivalence class partitioning | 20:07 |
127 | JUnit 5 API (Advanced) & Test Code Coverage | 19:22 |
128 | JUnit 5 API (Advanced p. 2) & Hamcrest Library | 20:04 |
129 | Integration Testing in Java | 13:36 |
130 | Mockito: Part 1 | 20:47 |
131 | Mockito: Part 2 | 18:18 |
132 | PowerMock | 14:30 |
133 | Test-driven development: Theory | 23:56 |
134 | BDD & ATTD | 15:55 |
135 | TDD, BDD & ATTD - Practice | 13:52 |
136 | Clean Code Architecture, Coupling & Cohesion | 22:11 |
137 | Tell, Don’t Ask Pricniple & Data Structures | 20:19 |
138 | Law of Demeter | 08:53 |
139 | Packaging Pricniples p.1: Cohesion Principles | 21:31 |
140 | Packaging Pricniples p.2: Coupling Principles and Others | 24:56 |
141 | GoF Patterns: Overview | 13:58 |
142 | Creational Patterns | 30:40 |
143 | Structural Patterns, p.1 | 31:08 |
144 | Structural Patterns, p.2 | 21:11 |
145 | Behevioral Patterns, p.1 | 33:59 |
146 | Behevioral Patterns, p.2 | 31:52 |
147 | Behevioral Patterns, p.3 | 20:53 |
148 | [Part 1] Localization and Internationalization | 14:24 |
149 | [Part 2] Localization and Internationalization | 18:46 |
150 | Databases: Overview - Part 1 | 24:57 |
151 | Databases: Overview - Part 2 | 22:59 |
152 | MySQL: Overview & Installation (including Workbench Installation) | 20:43 |
153 | PostgreSQL: Overview & Installation (including pgAdmin installation) | 16:47 |
154 | Relational Databases: Basic Concepts | 27:25 |
155 | Create Schema & Table: Naming, Collation, Engines, Types, Column Properties | 36:11 |
156 | Referential Integrity: Foreign Key Constraint & Cascading Operations | 21:35 |
157 | Indexes in Databases | 20:34 |
158 | Database Normalization & Denormalization | 32:39 |
159 | SQL: General Overview & DDL | 20:11 |
160 | SQL: DML - CRUD Operations (SELECT, INSERT, UPDATE, DELETE) | 29:09 |
161 | JOIN Queries, UNION & Subqueries | 15:01 |
162 | Views, Triggers, Stored Procedures & Functions | 30:37 |
163 | MySQL Workbench: Administration | 10:23 |
164 | Database Modelling & Design: Conceptual, Logical and Physical Data Models | 25:36 |
165 | SQL Homework: Task and Solution Review | 07:53 |
166 | JDBC Overview: Establish connection with DB from Java App | 20:23 |
167 | Statement, PreparedStatement & CallableStatement | 23:05 |
168 | Transactions, Batch Updates and MetaData | 20:54 |
169 | DAO (Data Access Object) Design Pattern | 19:30 |
170 | Exam task review and solution | 19:36 |
171 | OSI Model, HTTP, TCP/IP | 29:00 |
172 | Client-Server Architecture. URI, URN, URL | 18:27 |
173 | Web Servers: Overview | 19:31 |
174 | HTTP: Part 1 | 17:39 |
175 | HTTP: Part 2 | 20:41 |
176 | Apache Tomcat: Overview, Installation and First Run | 24:32 |
177 | Build Automation Tools: Overview | 14:51 |
178 | Apache Maven - Basics | 20:59 |
179 | The First Maven Project. Archetypes. | 26:46 |
180 | Working with Maven Project | 21:32 |
181 | Execute Maven Goals & Advanced Dependency Management | 20:01 |
182 | Maven Web Project | 19:12 |
183 | Maven Multi-Module Project | 28:03 |
184 | Intro to Servlets: Hello World Servlet | 27:32 |
185 | Servlet API | 31:23 |
186 | web.xml - Deployment Descriptor | 18:43 |
187 | Web Filters | 19:32 |
188 | Session & Cookies | 25:29 |
189 | Events Handling in Java Web Application | 23:42 |
190 | HTML Basics | 25:56 |
191 | РЎSS Basics | 28:36 |
192 | HTML Forms | 28:59 |
193 | Exam: HTML, CSS, Servlets, Web Filters & Web Server | 25:16 |
194 | JSP Overview | 24:12 |
195 | First JSP Page & Practical Examples | 19:10 |
196 | JSTL: Part 1 | 16:38 |
197 | JSTL: Part 2 | 20:02 |
198 | Custom Tags in JSP | 31:41 |
199 | MVC Design Pattern | 15:49 |
200 | Layered Architecture | 25:59 |
201 | Task Overview | 23:09 |
202 | Solution Overview: Part 1 | 27:15 |
203 | Solution Overview: Part 2 | 18:32 |
204 | Solution Overview: Part 3 | 11:02 |
205 | Connection Pooling: DBCP, C3P0, HikariCP & Tomcat Connection Pool with JNDI | 28:01 |
206 | OWASP Top 10: Overview | 18:48 |
207 | Broken Access Control | 35:22 |
208 | Cryptography Failures (Theory, Sensitive Data, Data Breach, Types of Failures) | 12:59 |
209 | Cryptography Failures (Practical Examples, SQL Injections, TLS/SSL, HTTPS) | 19:17 |
210 | Cryptography Failures (Examples, Password Encryption, Hashing, Salting) | 17:37 |
211 | Injection (Overview, Fuzzing, CWEs, Impact, Injection Types, Command Injection) | 15:22 |
212 | Injection (Cross Site Scripting, Types of XSS, SQL, JPA, NoSQL Injections) | 16:31 |
213 | Injection (XPath Injection, Log Injection, Input Validation) | 16:03 |
214 | Insecure Design (Overivew, CWEs, Shift Left Security, Threat Modeling Manifesto) | 19:43 |
215 | Insecure Design (Secure Design Process, Security Controls, Metrics, Examples) | 22:59 |
216 | Security Misconfiguration (Overview, CWEs, Types, Real-life attacks) | 20:18 |
217 | Security Misconfiguration (Hardening, Zero Trust, Defense in Depth, Practice) | 29:03 |
218 | Vulnerable & Outdated Components | 23:05 |
219 | Identification & Authentication Failures | 33:55 |
220 | Software & Data Integrity Failures | 17:34 |
221 | Security Logging & Monitoring Failures | 22:55 |
222 | Server-Side Request Forgery (SSRF) | 24:33 |
223 | Logging in Java: Part 1 (Logging theory, Logging Levels, Java Logging Framework) | 31:16 |
224 | Logging in Java: Part 2 (Log4J, Logback, SLF4J) | 32:02 |
225 | Introduction to JPA & ORM | 14:04 |
226 | First JPA Project: Entity, ID GenerationType, Composite Primary Keys, etc) | 20:42 |
227 | First JPA Project - Part 2: EntityManager, persistence.xml, Transactions in JPA | 21:40 |
228 | Operations with Entity (Create, Read, Update, Delete) & JPA Entity Lifecycle | 19:26 |
229 | Locking JPA: Optimistic & Pessimistic Locking | 30:34 |
230 | Relationships Between Entities | 22:25 |
231 | JPA Queries (Query, TypedQuery, NativeQuery, JPQL, Criteria API) | 25:41 |
232 | Caching in JPA/Hibernate | 32:24 |
233 | N+1: Problem and Solution | 16:35 |
234 | ===== EXAM TASK: JPA & Hibernate ===== | 21:07 |
235 | Spring Framework: General Overview | 15:49 |
236 | Spring Architecture, Inversion of Control, Dependency Injection & Spring Beans | 14:35 |
237 | IoC, Beans Configuration, XML and Annotations - Practice | 08:56 |
238 | Spring Dependency Injection: Practice | 26:19 |
239 | Spring MVC: Overview & First Project | 34:02 |
240 | Model, RequestParam & PathVariable | 20:35 |
241 | Spring MVC Forms, @ModelAttribute, Bean Validation | 25:52 |
242 | Exception Handling in Spring MVC | 25:25 |
243 | Serving Static Resources in Spring MVC | 13:12 |
244 | WebFilters & HandlerInterceptor in Spring MVC | 17:27 |
245 | L10N & I18N in Spring MVC | 09:38 |
246 | Redirect & Forward in Spring MVC | 07:53 |
247 | Spring API - Work with Cookies in Spring MVC & Work with Properties | 09:09 |
248 | EXAM: Spring Core & Spring MVC | 39:02 |
249 | Spring Security Introduction | 16:31 |
250 | First Login Form & First Security Filter Configuration | 34:13 |
251 | Login with Database Users, Roles & Privileges | 25:42 |
252 | Remember Me & Methods Security | 15:59 |
253 | Spring Security Architecture & Authentication Provider | 18:09 |
254 | EXAM: Spring Security - Online Shop | 25:31 |
255 | Spring Data & Spring Data JPA: Overview | 13:50 |
256 | Spring Data JPA: Practice | 21:30 |
257 | Spring JDBC: Overview | 13:40 |
258 | Spring JDBC: Practice | 28:56 |
259 | Aspect Oriented Programming | 17:50 |
260 | Spring AOP with AspectJ: Practice | 22:27 |
261 | REST Architecture: Overview and Key Principles | 21:47 |
262 | Naming Convention for RESTful Services | 17:53 |
263 | RESTful Service Implementation with Spring MVC | 23:40 |
264 | Review of tools for API testing & Postman Installation | 14:20 |
265 | API Testing with Postman | 23:15 |
266 | Spring Boot: Introduction | 22:43 |
267 | The First Spring Boot Project | 18:32 |
268 | Spring Boot Starters | 33:57 |
269 | Spring Boot Configurations & Application Properties | 29:42 |
270 | Spring Boot Actuator - Monitoring Tools | 26:33 |
271 | OpenAI: Basic Concepts | 27:26 |
272 | What programming language to choose | 43:18 |
273 | Q&A Online Stream with Students (June 19th, 2021) | 58:01 |
274 | Bonus Lesson | 02:52 |
Similar courses to Java from Zero to First Job, Practical Guide, 2000+ examples
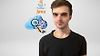
Java Test Automation Engineer - from Zero to Heroudemy
Category: Java, Other (QA)
Duration 18 hours 40 minutes 6 seconds
Course
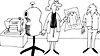
Java Design Patternsjavaspecialists.eu
Category: Java
Duration 16 hours 20 minutes 37 seconds
Course
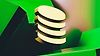
RESTful Web Services, Java, Spring Boot, Spring MVC and JPAudemy
Category: Spring Boot, Spring Data, Java, Spring, Spring MVC, Spring Security
Duration 25 hours 8 minutes 11 seconds
Course
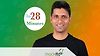
Learn Java Unit Testing with Junit & Mockito in 30 Stepsudemy
Category: Java
Duration 4 hours 44 minutes 35 seconds
Course
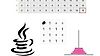
Algorithms in Java: Live problem solving & Design Techniquesudemy
Category: Java
Duration 19 hours 41 minutes 26 seconds
Course
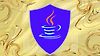
Java Programming Bootcamp: Zero to Masteryzerotomastery.io
Category: Java
Duration 9 hours 15 minutes 36 seconds
Course
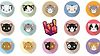
Catsrockthejvm.com
Category: Java
Duration 10 hours 39 minutes 36 seconds
Course
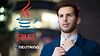
Java Foundations: The Complete Course with Java 21 Updatesudemy
Category: Java
Duration 88 hours 37 minutes 5 seconds
Course
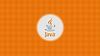
Experience Design Patterns In Javaudemy
Category: Java
Duration 7 hours 19 minutes 58 seconds
Course
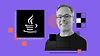
Java Data Structures & Algorithms + LEETCODE Exercisesudemy
Category: Java
Duration 9 hours 47 minutes 55 seconds
Course