Write PHP Like a Pro: Build a PHP MVC Framework From Scratch
Learn to write faster, easier to maintain, more secure PHP websites by using a model-view-controller framework. Learn the basic concepts of using a model-view-controller framework that will make your PHP projects faster, easier to write and maintain, and more secure.
Read more about the course
Learn to Structure your PHP Code Like a Professional by Building a PHP MVC Framework from Scratch.
- Model-view-controller (MVC) pattern concepts
- Build an MVC framework in PHP from scratch
- Separate application code from presentation code
- Use namespaces and an autoloader to load classes automatically
Take your PHP Projects to the Next Level
Learning how to use an MVC framework puts a very powerful tool at your fingertips. Most commercial websites and web applications written in PHP use some sort of framework, and the MVC pattern is the most popular type of framework in use.
The gap between knowing PHP and using a framework can be huge. This course bridges that gap. By writing your own framework from scratch, you'll gain an understanding of just how each component works. Frameworks like Laravel, Symfony and CodeIgniter all use the MVC pattern, so understanding how an MVC framework is put together will give you a strong background to more easily learn frameworks such as these.
Content and Overview
I designed this course to be easily understood by programmers who know PHP but don't know how to use a framework. Are you putting database code and HTML in the same PHP script? Want to know why this is a bad idea? Want to know how to do it better?
Starting with the basic concepts of MVC frameworks, this course will take you through all the steps needed to build a complete MVC framework, a piece at a time.
Beginning with a single PHP script, each lecture explains what you're going to add to the code and why, building up the framework step by step. At the end of this course, you'll have built a complete MVC framework in PHP, ready to use in your own projects.
Complete with working source code at every stage, you'll be able to work alongside the instructor and will receive a verifiable certificate of completion upon finishing the course.
- You should already be familiar with PHP and HTML.
- You should be comfortable installing software on your computer - in the first section we'll be installing a web server.
- This PHP MVC course is meant for those who already know PHP but want to know how they can improve their code by using a framework. This course is not for you if you don't already know PHP.
- The gap between learning PHP and using a framework can be large - if you've just learnt PHP and you're wondering what the next step might be, this course is for you.
What you'll learn:
- Separate application code from presentation code
- Organise your PHP code into models, views and controllers in an MVC framework
- Use namespaces and an autoloader to load PHP classes automatically
- Use the Composer tool to manage third-party package dependencies and autoloading
- Handle errors and exceptions in PHP and display more or less error detail based on the environment
- Understand how MVC frameworks work, making it easier to learn an existing framework like Laravel or CodeIgniter
Watch Online Write PHP Like a Pro: Build a PHP MVC Framework From Scratch
# | Title | Duration |
---|---|---|
1 | Introduction | 03:18 |
2 | The problem with writing web applications: how NOT to structure your code | 03:52 |
3 | The MVC pattern: What it is and how it can help you write better code | 03:09 |
4 | Install a web server, database server and PHP on your computer | 03:21 |
5 | Start writing the framework: Create the folders and configure the web server | 03:50 |
6 | Create a central entry point to the framework: the front controller | 02:59 |
7 | Configure the web server to have pretty URLs | 02:47 |
8 | Create and require (not include) the router class | 02:37 |
9 | Create the routing table in the router, and add some routes | 02:13 |
10 | Match the requested route to the list of routes in the routing table | 03:06 |
11 | Introduction to advanced routing using route variables | 01:35 |
12 | How to do complex string comparisons: an introduction to regular expressions | 04:48 |
13 | Using special characters in regular expressions: advanced pattern matching | 03:58 |
14 | Write even more powerful regular expressions: use character sets and ranges | 02:34 |
15 | Extract parts of strings using regular expression capture groups | 02:58 |
16 | Get the controller and action from a URL with a fixed structure | 05:37 |
17 | Replace parts of strings using regular expressions | 02:50 |
18 | Get the controller and action from a URL with a variable structure | 05:31 |
19 | Add custom variables of any format to the URL | 02:52 |
20 | Controllers and actions: an introduction | 01:30 |
21 | How to create objects and run methods dynamically | 01:49 |
22 | Dispatch the route: create the controller object and run the action method | 04:35 |
23 | How to better organise your classes by using namespaces | 03:48 |
24 | Class autoloading: load classes automatically without having to require them | 03:12 |
25 | Load classes automatically: add namespaces and an autoload function | 02:55 |
26 | Remove query string variables from the URL before matching to a route | 02:26 |
27 | Pass route parameters from the route to all controllers | 02:33 |
28 | The __call magic method: how to call inaccessible methods in a class | 02:25 |
29 | Action filters: call a method before and after every action in a controller | 04:49 |
30 | Organise controllers in subdirectories: add a route namespace option | 02:28 |
31 | Views: an introduction | 01:01 |
32 | Display a view: create a class to render views and use it in a controller | 02:16 |
33 | Output escaping: what it is, why do it, and how and when to do it | 04:31 |
34 | Pass data from the controller to the view | 01:56 |
35 | Templating engines: what they are and how they can improve your PHP code | 03:28 |
36 | Make views easier to create and maintain: add a template engine | 02:23 |
37 | Remove repetition in the view templates: add a base template to inherit from | 02:21 |
38 | Install third-party PHP code libraries automatically using Composer | 03:00 |
39 | Installing and using Composer | 03:35 |
40 | Install the template engine library using Composer | 02:00 |
41 | Include all package classes automatically using the Composer autoloader | 01:47 |
42 | Use the Composer autoloader to load the template engine library | 01:04 |
43 | Add your own classes to the Composer autoloader | 02:52 |
44 | Replace the autoload function with the Composer autoloader | 02:58 |
45 | Models: an introduction | 01:44 |
46 | Create a database and check you can connect to it from PHP | 02:59 |
47 | An introduction to PDO: why it makes working with databases in PHP easier | 03:28 |
48 | Add a model, get data from the database and display it in a view | 02:17 |
49 | Optimise the database connection: connect only on demand and reuse it | 02:52 |
50 | Put application configuration settings in a separate file | 02:16 |
51 | How PHP reports problems: errors, exceptions, and how to handle them | 03:16 |
52 | Handle errors: convert errors to exceptions and add an exception handler | 02:54 |
53 | PHP configuration settings: where to find them and how to change them | 02:03 |
54 | Configure PHP to display error messages | 01:58 |
55 | Show detailed error messages to developers, friendly error messages to users | 03:12 |
56 | Categorise different types of error using HTTP status codes | 03:15 |
57 | Add views to make error pages look nicer in production | 01:57 |
58 | A brief introduction to some popular frameworks | 03:14 |
59 | Conclusion | 02:55 |
Similar courses to Write PHP Like a Pro: Build a PHP MVC Framework From Scratch
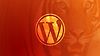
Become a WordPress Developer: Unlocking Power With Codeudemy
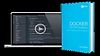
Docker for PHP Developersudemy
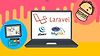
PHP with Laravel - Create a Restaurant Management Systemudemy
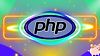
PHP Development Bootcamp: Zero to Masteryzerotomastery.io
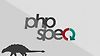
phpspec: Testing... *Designing* with a Bitesymfonycasts
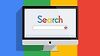
Make a Google search engine clone: JavaScript PHP and MySQLudemy
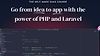
Self-Made SaaS CourseAndrew Schmelyun
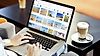
Make a Google search engine clone: JavaScript PHP and MySQLudemy
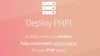
Deploy PHP!serversforhackers.com
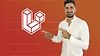