PHP From Scratch 2024 | Beginner To Advanced
Learn PHP fundamentals then build a job listing website from the ground up, using a Laravel-like infrastructure. This course is broken up into two parts. First, we have seven learning modules to learn the fundamentals of PHP programming, including:
Read more about the course
- Data Types & Variables
- Arrays & Iteration
- Control Structures & Conditionals
- Functions & Scope
- Object Oriented Programming
- Superglobals ($_GET, $_POST, $_SESSION, $_COOKIES, etc)
- Database Integration & PDO
After that, we go for a hands-on approach and build a job listing website. We build this from the ground up without any framework or libraries. These sections will teach you how to structure a vanilla PHP project and is a great precursor to learning Laravel or another framework.
Here is what we will do in the project videos:
- We will create a custom Laravel-like router. We will refactor this a bunch of times to keep adding features like accepting HTTP methods, params and middleware.
- We will structure our project into two main folders, which will be called Framework and App. Framework will be the core that includes classes like Router, Database, Validation, and Session. App will include our controllers and views.
- We will create CRUD operations for job listings.
- We will implement an authentication and authorization system as well as protect routes using custom middleware.
- We will add a search feature to search/filter listings by keywords and/or location
- We will learn to validate and sanitize data as well as use prepared statements with PDO to protect against SQL injection attacks.
If you are a beginner, I suggest taking the course from the beginning and go through all of the learning modules. If you are more experienced and know the fundamentals, you can jump right into the project.
Watch Online PHP From Scratch 2024 | Beginner To Advanced
# | Title | Duration |
---|---|---|
1 | Welcome To The Course | 04:16 |
2 | What Is PHP? | 11:06 |
3 | Setup PHP - MacOS | 04:39 |
4 | Setup PHP - Windows | 04:42 |
5 | Text Editor Setup | 04:16 |
6 | PHP Sandbox Setup | 03:48 |
7 | PHP Tags, Printing & Comments | 09:29 |
8 | Variables | 05:46 |
9 | Data Types | 10:22 |
10 | String Concatenation | 07:36 |
11 | Type Casting & Juggling | 10:12 |
12 | Variables Challenge | 04:41 |
13 | Arithmetic Operators & Functions | 12:29 |
14 | String Functions | 06:15 |
15 | Dates & Times | 07:52 |
16 | Intro To Arrays | 12:28 |
17 | Array Functions | 20:04 |
18 | Associative Arrays | 05:29 |
19 | Multi-Dimensional Arrays | 07:13 |
20 | Array Challenges | 14:47 |
21 | Basic Loops | 12:03 |
22 | Nested Loops | 07:22 |
23 | Looping Through Arrays | 08:33 |
24 | Multi-Dimensional Array Iteration | 04:58 |
25 | Array & Loop Challenges | 09:47 |
26 | If Statements | 06:13 |
27 | Conditional HTML Output | 05:33 |
28 | Comparison & Logical Operators | 06:44 |
29 | Conditionals In Loops - break & continue | 06:25 |
30 | Activity: Dynamic Job Listings | 05:20 |
31 | FizzBuzz Challenge | 05:27 |
32 | Switch Statements | 06:43 |
33 | Ternary Operator | 09:30 |
34 | Null Coalescing Operator | 04:18 |
35 | Names Challenge | 07:26 |
36 | Functions & Return Values | 05:04 |
37 | Parameters & Arguments | 06:23 |
38 | Global & Local Scope | 06:11 |
39 | Constants | 05:06 |
40 | Optional Type Declarations | 04:37 |
41 | Activity: Job Listings Helper Functions | 07:06 |
42 | Average Salary Challenge | 07:45 |
43 | Anonymous Functions & Closures | 06:29 |
44 | Callback Functions | 04:42 |
45 | Arrow Functions | 04:34 |
46 | Format Salary Refactor Challenge | 03:30 |
47 | More Function Challenges | 12:27 |
48 | OOP Overview | 05:35 |
49 | Creating a Class | 10:34 |
50 | Access Modifiers, Getters & Setters | 06:48 |
51 | Inheritence | 07:09 |
52 | Static Members & Methods | 03:22 |
53 | OOP Challenges | 10:55 |
54 | Abstract Classes | 10:28 |
55 | Interfaces | 08:37 |
56 | Overview Of Superglobals | 03:39 |
57 | $_SERVER - Get Server Information | 11:54 |
58 | Environment Variables & $GLOBALS | 06:39 |
59 | $_GET - Data From Query Params | 15:46 |
60 | $_POST - Data From Forms | 12:26 |
61 | $_REQUEST - Superglobal | 02:41 |
62 | $_FILES - Uploading Files | 12:19 |
63 | Message Alert Challenge | 08:23 |
64 | $_SESSION - Creating a Session | 08:45 |
65 | $_COOKIE - Working With Cookies | 10:24 |
66 | An Intro To Databases | 07:35 |
67 | MySQL Setup - MacOS | 03:05 |
68 | MySQL Setup - Windows | 02:14 |
69 | MySQL Shell & Making Queries | 13:52 |
70 | MySQL Workbench & Database Setup | 10:54 |
71 | Database Users & Privileges | 06:00 |
72 | Connect With PDO | 07:21 |
73 | Fetch Multiple Records | 11:07 |
74 | Fetch Single Record | 08:57 |
75 | Create Form & Insert Record | 07:35 |
76 | Delete Records | 10:16 |
77 | Edit Form & Update Records | 11:15 |
78 | Project Intro | 07:16 |
79 | UI Theme Files | 05:42 |
80 | Folder Setup | 02:35 |
81 | Home View & Set Document Root | 11:58 |
82 | Git Setup & Commit | 04:32 |
83 | Split UI Into Partials | 15:37 |
84 | Inspect Helper Functions | 04:34 |
85 | Create a VERY Basic Router | 11:40 |
86 | Create Views | 06:44 |
87 | Separate Router Files | 04:32 |
88 | Router Refactor To Class | 22:16 |
89 | Section Intro | 00:52 |
90 | Project Database Setup - MySQL Workbench | 16:49 |
91 | Database Class & Connection | 10:07 |
92 | Query Method & Fetch Listings | 09:00 |
93 | Pass Data To View | 14:15 |
94 | Single Listing & Named Params | 10:59 |
95 | Single Listing Display | 04:26 |
96 | Section Intro | 01:37 |
97 | Folder Structure Refactor | 04:14 |
98 | Custom Autoloader | 03:56 |
99 | Composer & PSR-4 Autoloader | 05:12 |
100 | Namespaces | 05:03 |
101 | Router Refactor For Controller Classes | 11:31 |
102 | Controller Classes - Home & Listings | 08:25 |
103 | ErrorController Class | 08:01 |
104 | Handling Route Params | 22:42 |
105 | Section Wrap | 02:31 |
106 | Section Intro | 01:09 |
107 | Validation Class | 12:36 |
108 | Form Submission & Sanitizing Data | 10:51 |
109 | Implement Validation | 10:17 |
110 | Insert Listings Into Database | 15:07 |
111 | Delete Listings | 09:52 |
112 | Flash Messages | 08:56 |
113 | Edit Form | 07:49 |
114 | Update Listing | 19:37 |
115 | Section Intro | 01:27 |
116 | User Controller & Views | 09:55 |
117 | Register Validation & Error Partial | 12:39 |
118 | Register User | 11:34 |
119 | Session Class & Set User | 15:47 |
120 | Dynamic Navbar Links | 05:04 |
121 | Logout & Clear Session | 04:37 |
122 | Login Functionality | 13:38 |
123 | Authorize Middleware | 14:00 |
124 | Delete Authorization | 12:37 |
125 | Flash Message Methods | 12:01 |
126 | Update Authorization | 05:52 |
127 | Section Intro | 00:36 |
128 | Listing Search Functionality | 17:30 |
129 | Hostinger Intro | 02:41 |
130 | Hosting & Domain Setup | 03:31 |
131 | Database Export - Local | 05:08 |
132 | Upload Website & Configure Files | 09:00 |
133 | Course Wrap Up | 02:09 |
Similar courses to PHP From Scratch 2024 | Beginner To Advanced
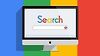
Make a Google search engine clone: JavaScript PHP and MySQLudemy
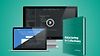
Refactoring to Collectionsadamwathan
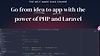
Self-Made SaaS CourseAndrew Schmelyun
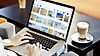
Make a Google search engine clone: JavaScript PHP and MySQLudemy
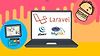
PHP with Laravel - Create a Restaurant Management Systemudemy
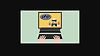
Write PHP Like a Pro: Build a PHP MVC Framework From Scratchudemy
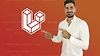
Mastering Laravel 10 Query Builder, Eloquent & Relationshipsudemy
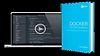
Docker for PHP Developersudemy
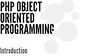