Working with Microservices in Go (Golang)
For a long time, web applications were usually a single application that handled everything—in other words, a monolithic application. This monolith handled user authentication, logging, sending email, and everything else. While this is still a popular (and useful) approach, today, many larger scale applications tend to break things up into microservices. Today, most large organizations are focused on building web applications using this approach, and with good reason.
More
Microservices, also known as the microservice architecture, are an architectural style which structures an application as a loosely coupled collection of smaller applications. The microservice architecture allows for the rapid and reliable delivery of large, complex applications. Some of the most common features for a microservice are:
it is maintainable and testable;
it is loosely coupled with other parts of the application;
it can deployed by itself;
it is organized around business capabilities;
it is often owned by a small team.
In this course, we'll develop a number of small, self-contained, loosely coupled microservices that will will communicate with one another and a simple front-end application with a REST API, with RPC, over gRPC, and by sending and consuming messages using AMQP, the Advanced Message Queuing Protocol. The microservices we build will include the following functionality:
A Front End service, that just displays web pages;
An Authentication service, with a Postgres database;
A Logging service, with a MongoDB database;
A Listener service, which receives messages from RabbitMQ and acts upon them;
A Broker service, which is an optional single point of entry into the microservice cluster;
A Mail service, which takes a JSON payload, converts into a formatted email, and send it out.
All of these services will be written in Go, commonly referred to as Golang, a language which is particularly well suited to building distributed web applications.
We'll also learn how to deploy our distributed application to a Docker Swarm and Kubernetes, and how to scale up and down, as necessary, and to update individual microservices with little or no downtime.
Watch Online Working with Microservices in Go (Golang)
# | Title | Duration |
---|---|---|
1 | Introduction | 09:24 |
2 | About me | 01:02 |
3 | Installing Go | 00:52 |
4 | Installing Visual Studio Code | 01:29 |
5 | Installing Make | 01:28 |
6 | Installing Docker | 00:52 |
7 | Asking for help | 01:15 |
8 | Mistakes. We all make them. | 01:07 |
9 | What we'll cover in this section | 00:42 |
10 | Setting up the front end | 01:37 |
11 | Reviewing the front end code | 03:37 |
12 | Our first service: the Broker | 14:57 |
13 | Building a docker image for the Broker service | 09:00 |
14 | Adding a button and JavaScript to the front end | 08:39 |
15 | Creating some helper functions to deal with JSON and such | 09:05 |
16 | Simplifying things with a Makefile (Mac & Linux) | 04:19 |
17 | Simplifying things with a Makefile (Windows) | 03:27 |
18 | What we'll cover in this section | 01:53 |
19 | Setting up a stub Authentication service | 11:14 |
20 | Creating and connecting to Postgres from the Authentication service | 08:56 |
21 | Updating our docker-compose.yml for Postgres and the Authentication service | 14:14 |
22 | Populating the Postgres database | 02:28 |
23 | Adding a route and handler to accept JSON | 08:41 |
24 | Update the Broker for a standard JSON format, and conect to our Auth service | 15:07 |
25 | Updating the front end to authenticate thorough the Broker and trying things out | 07:10 |
26 | What we'll cover in this section | 01:01 |
27 | Getting started with the Logger service | 10:08 |
28 | Setting up the Logger data models | 15:06 |
29 | Finishing up the Logger data models | 08:02 |
30 | Setting up routes, handlers, helpers, and a web server in our logger-service | 08:42 |
31 | Adding MongoDB to our docker-compose.yml file | 06:33 |
32 | Add the logger-service to docker-compose.yml and the Makefile | 04:24 |
33 | Adding a route and handler on the Broker to communicate with the logger service | 06:32 |
34 | Update the front end to post to the logger, via the broker | 04:13 |
35 | Add basic logging to the Authentication service | 04:25 |
36 | Trying things out | 04:24 |
37 | What we'll cover in this section | 01:11 |
38 | Adding Mailhog to our docker-compose.yml | 02:08 |
39 | Setting up a stub Mail microservice | 05:10 |
40 | Building the logic to send email | 23:19 |
41 | Building the routes, handlers, and email templates | 12:31 |
42 | Challenge: Adding the Mail service to docker-compose.yml and the Makefile | 00:46 |
43 | Solution to challenge | 03:48 |
44 | Modifying the Broker service to handle mail | 08:01 |
45 | Updating the front end to send mail | 09:50 |
46 | A note about mail and security | 01:19 |
47 | What we'll cover in this section | 02:30 |
48 | Creating a stub Listener service | 03:21 |
49 | Adding RabbitMQ to our docker-compose.yml | 04:22 |
50 | Connecting to RabbitMQ | 07:52 |
51 | Writing functions to interact with RabbitMQ | 21:16 |
52 | Adding a logEvent function to our Listener microservice | 02:41 |
53 | Updating main.go to start the Listener function | 02:45 |
54 | Change the RabbitMQ server URL to the Docker address | 00:41 |
55 | Creating a Docker image and updating the Makefile | 06:40 |
56 | Updating the broker to interact with RabbitMQ | 04:30 |
57 | Writing logic to Emit events to RabbitMQ | 05:59 |
58 | Adding a new function in the Broker to log items via RabbitMQ | 06:38 |
59 | Trying things out | 03:56 |
60 | What we'll cover in this section | 02:15 |
61 | Setting up an RPC server in the Logger microservice | 05:39 |
62 | Listening for RPC calls in the Logger microservice | 04:46 |
63 | Calling the Logger from the Broker using RPC | 05:42 |
64 | Trying things out | 02:47 |
65 | What we'll cover in this section | 02:29 |
66 | Installing the necessary tools for gRPC | 02:47 |
67 | Defining a Protocol for gRPC: the .proto file | 04:23 |
68 | Generating the gRPC code from the command line | 06:19 |
69 | Getting started with the gRPC server | 07:01 |
70 | Listening for gRPC connections in the Logger microservice | 04:08 |
71 | Writing the client code | 10:49 |
72 | Updating the front end code | 02:12 |
73 | Trying things out | 02:43 |
74 | What we'll cover in this section | 03:19 |
75 | Building images for our microservices | 03:53 |
76 | Creating a Docker swarm deployment file | 11:51 |
77 | Initalizing and starting Docker Swarm | 04:40 |
78 | Starting the front end and hitting our swarm | 02:13 |
79 | Scaling services | 03:20 |
80 | Updating services | 04:31 |
81 | Stopping Docker swarm | 01:51 |
82 | Updating the Broker service, and creating a Dockerfile for the front end | 05:55 |
83 | Solution to the Challenge | 02:27 |
84 | Adding the Front end to our swarm.yml deployment file | 01:26 |
85 | Adding Caddy to the mix as a Proxy to our front end and the broker | 10:16 |
86 | Modifying our hosts file to add a "backend" entry and bringing up our swarm | 06:32 |
87 | Challenge: correcting the URL to the broker service in the front end | 06:44 |
88 | Solution to challenge | 02:21 |
89 | Updating Postgres to 14.2 - why monitoring is important! | 01:54 |
90 | Spinning up two new servers on Linode | 04:39 |
91 | Setting up a non-root account and putting a firewall in place. | 05:33 |
92 | Installing Docker on the servers | 03:20 |
93 | Setting the hostname for our server | 03:33 |
94 | Adding DNS entries for our servers | 05:45 |
95 | Adding a DNS entry for the Broker service | 01:19 |
96 | Initializing a manager, and adding a worker | 02:27 |
97 | Updating our swarm.yml and Caddy dockerfile for production | 07:06 |
98 | Trying things out, and correcting some mistakes | 10:49 |
99 | Populating the remote database using an SSH tunnel | 02:34 |
100 | Enabling SSL certificates on the Caddy microservice | 11:13 |
101 | What we'll cover in this section | 01:59 |
102 | Installing minikube | 01:55 |
103 | Installing kubectl | 02:39 |
104 | Initializing a cluster | 03:07 |
105 | Bringing up the k8s dashboard | 02:47 |
106 | Creating a deployment file for Mongo | 14:08 |
107 | Creating a deployment file for RabbitMQ | 04:53 |
108 | Creating a deployment file for the Broker service | 03:22 |
109 | When things go wrong... | 07:54 |
110 | Creating a deployment file for MailHog | 02:21 |
111 | Creating a deployment file for the Mail microservice | 04:19 |
112 | Creating a deployment file for the Logger service | 04:28 |
113 | Creating a deployment file for the Listener service | 02:03 |
114 | Running Postgres on the host machine, so we can connect to it from k8s | 02:48 |
115 | Creating a deployment file for the Authentication service | 03:51 |
116 | Trying things out by adding a LoadBalancer service | 06:29 |
117 | Creating a deployment file for the Front End microservice | 04:42 |
118 | Adding an nginx Ingress to our cluster | 05:34 |
119 | Trying out our Ingress | 04:25 |
120 | Scaling services | 03:33 |
121 | Updating services | 02:04 |
122 | Deploying to cloud services | 05:21 |
123 | Just some final thoughts and observations | 03:52 |
Similar courses to Working with Microservices in Go (Golang)
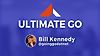
Ultimate Go
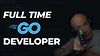
Full Time Go Dev
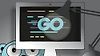
Go Bootcamp: Master Golang with 1000+ Exercises and Projects
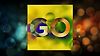
Design Patterns in Go
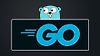
Go - The Complete Guide
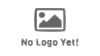
Go: The Complete Developer's Guide (Golang)
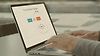
Advanced Branching and Looping in GO
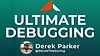
Ultimate Debugging
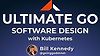