Learn to Create Web Applications using Go
Why can't anyone point me to a concrete example of how to hash and store user passwords? Or how to build the rest of an authentication system? Why is it so frustrating simply trying to figure out how to share my database connection with my handlers, or how to email users without slowing down every web request? Can anyone just tell me how to organize my code? Why are there so many varying opinions on this? Which one is right? Should I be using MVC? What is this domain driven design? Ugh! I want to give up!
More
You have heard that Go is great for web apps, but getting started leads to so many questions that it can feel overwhelming. But what if you could build a web application this week, starting today? What if you had someone to guide you through the standard libraries and help you understand how they all work together?
Watch Online Learn to Create Web Applications using Go
# | Title | Duration |
---|---|---|
1 | 2.0 - Creating a code directory and a git repo | 12:10 |
2 | 2.1 - A basic web application | 13:13 |
3 | 2.1 [ASIDE] - What is a web request? | 10:03 |
4 | 2.2 - Explaining our web application in detail | 09:15 |
5 | 2.3 - Dynamic reloading | 10:33 |
6 | 3.1 - Setting the Content-Type header | 08:03 |
7 | 3.2 - Adding a contact page | 08:27 |
8 | 3.3 - Adding a 404 page | 07:35 |
9 | 3.4 - net/http's ServeMux | 15:20 |
10 | 3.5 - github.com/julienschmidt's httprouter | 07:48 |
11 | 3.6 - Gorilla Web Toolkit's mux package | 07:07 |
12 | 3.7 - Implementing the gorilla/mux router | 09:57 |
13 | 3.Ex0 - Exercises overview | 07:11 |
14 | 3.Ex1 - Adding an FAQ page | 05:35 |
15 | 3.Ex2 - Adding a 404 page | 05:25 |
16 | 3.Ex3 - Using httprouter | 07:44 |
17 | 4.0 - What are templates? | 07:22 |
18 | 4.1 - Our first HTML template | 14:51 |
19 | 4.2 - Code injection and contextual encoding | 07:24 |
20 | 4.Ex0 - Exercises overview | 03:34 |
21 | 4.Ex1 - Rendering a custom field | 03:12 |
22 | 4.Ex2 - Rendering additional data attributes | 06:03 |
23 | 4.Ex3 - Rendering with nested structures | 04:09 |
24 | 5.0 - Intro to the MVC videos | 02:58 |
25 | 5.1 - What is MVC? | 13:17 |
26 | 5.2 - Walking through a web request with MVC | 05:59 |
27 | 5.Ex0 - Exercises | 06:36 |
28 | 6.0 - Creating our first view | 11:58 |
29 | 6.1 - Creating the contact view | 05:49 |
30 | 6.2 - Named and nested templates | 08:44 |
31 | 6.3 - Creating the View type | 08:39 |
32 | 6.4 - Using the View type | 12:31 |
33 | 6.5 - Creating a Bootstrap layout | 25:13 |
34 | 6.6 - Adding a navigation bar | 12:46 |
35 | 6.7 - Cleaning up our code by globbing template files | 17:04 |
36 | 6.8 - Simplifying our view rendering logic | 09:51 |
37 | 6.9 - Moving our footer to the bootstrap layout | 03:50 |
38 | 6.10 - Summary | 04:43 |
39 | 7.0 - Creating the signup page | 13:37 |
40 | 7.1 - Wrapping the signup form in a bootstrap panel | 13:56 |
41 | 7.2 - Adding a signup link to the navbar | 03:30 |
42 | 7.3 - An introduction to REST | 14:19 |
43 | 7.4 - Creating our first controller - the users controller | 29:05 |
44 | 7.5 - CRUD, HTTP verbs with Gorilla mux, and the create action | 22:39 |
45 | 7.6 - Parsing the signup form (parsing POST forms) | 13:05 |
46 | 7.7 - Parsing forms with gorilla schema | 19:56 |
47 | 7.8 - DRYing up our form parsing code | 14:27 |
48 | 7.9 - Creating a controller for our mostly static pages | 15:24 |
49 | 7.10 - Making views easier to create | 13:31 |
50 | 8.0 - What does it mean to persist data? | 05:05 |
51 | 8.1 - Web applications use databases to persist data | 07:50 |
52 | 8.2 - We will be using PostgreSQL | 03:08 |
53 | 8.3 - SQL has many great educational resources | 06:09 |
54 | 8.4 - Postgres is scalable and relatively easy to use | 04:38 |
55 | 8.5 - Setting up and connecting to Postgres | 07:37 |
56 | 8.6 - SQL basics and creating tables to interact with | 07:16 |
57 | 8.7 - Connecting to our database with Go's sql package | 18:24 |
58 | 8.8 - Writing records to our database with Go's sql package | 15:52 |
59 | 8.9 - Querying for records with Go's sql package | 12:59 |
60 | 8.10 - Handling relational data with Go's sql package | 15:31 |
61 | 8.11 - Setting up GORM | 08:27 |
62 | 8.12 - Creating our first model with GORM | 14:19 |
63 | 8.13 - Creating records and logging with GORM | 13:35 |
64 | 8.14 - Querying records with GORM | 16:42 |
65 | 8.15 - Error handling with GORM | 12:18 |
66 | 8.16 - Relational data with GORM | 11:15 |
67 | 9.1 - Creating the User model | 09:27 |
68 | 9.2 - Creating the UserService | 19:10 |
69 | 9.3 - The create user method | 07:22 |
70 | 9.4 - What does a model test look like? | 16:12 |
71 | 9.5 - Finishing the UserService implementation | 26:11 |
72 | 9.6 - Connecting models and controllers | 25:02 |
73 | 10.0 - An intro to building an auth system | 14:20 |
74 | 10.1 - Store hashes, not passwords | 13:37 |
75 | 10.2 - Implementing bcrypt hashing | 10:40 |
76 | 10.3 - Using passwords from the signup form | 05:27 |
77 | 10.4 - Salt and pepper | 14:28 |
78 | 10.5 - Creating the login form | 14:00 |
79 | 10.6 - Authenticating users | 17:55 |
80 | 11.0 - Remembering users and creating our first cookie | 19:41 |
81 | 11.1 - Viewing cookies via code | 05:44 |
82 | 11.2 - Creating cookies on login and signup | 09:04 |
83 | 11.3 - Securing our cookies from tampering | 19:42 |
84 | 11.4 - Generating remember tokens | 13:09 |
85 | 11.4 [ASIDE] - Why 32 bytes? | 07:29 |
86 | 11.5 - Writing a remember token hasher | 16:28 |
87 | 11.6 - Hashing remember tokens on user creation and update | 19:29 |
88 | 11.7 - Storing remember tokens in cookies | 15:10 |
89 | 11.8 - Securing our cookies from XSS | 07:33 |
90 | 11.9 - Securing our cookies from theft and CSRF | 10:50 |
91 | 12.0 - Validating and normalizing | 14:03 |
92 | 12.1 - Static types vs interfaces | 08:41 |
93 | 12.1 [ASIDE] - Emebedding, interfaces, and chaining | 11:43 |
94 | 12.2 - The UserDB interface | 18:11 |
95 | 12.3 - The UserService interface | 17:55 |
96 | 12.4.0 - Organizing validation code | 21:46 |
97 | 12.4.1 - Remember token normalizer | 07:04 |
98 | 12.4.2 - Ensuring remember tokens are set on create | 04:31 |
99 | 12.4.3 - Ensuring a valid ID on delete | 06:59 |
100 | 12.5.0 - Converting emails to lowercase and trimming whitespace | 09:45 |
101 | 12.5.1 - Requiring email addresses | 04:11 |
102 | 12.5.2 - Verifying emails match a pattern | 16:28 |
103 | 12.5.3 - Verifying an email address isn't taken | 10:09 |
104 | 12.6 - Validating and normalizing passwords | 15:12 |
105 | 12.7 - Validating and normalizing remember tokens | 12:41 |
106 | 13.1 - Bootstrap alerts | 06:08 |
107 | 13.2 - Dynamic alerts | 05:19 |
108 | 13.3 - Only show alerts when necessary | 05:09 |
109 | 13.4 - Creating the views.Data type | 10:25 |
110 | 13.5 - Handling signup errors | 13:11 |
111 | 13.6 - Only display public errors | 18:02 |
112 | 13.7 - Handling login errors | 09:45 |
113 | 13.8 - Handling rendering errors | 11:42 |
114 | 14.0 - Intro to the gallery chapter | 04:36 |
115 | 14.1 - The gallery model | 07:31 |
116 | 14.2 - Sharing a GORM connection and the GalleryService | 07:49 |
117 | 14.3 - Utilizing our shared GORM connection (the Services type) | 06:12 |
118 | 14.4 - Moving close to the Services type | 07:47 |
119 | 14.5 - Implementing the GalleryService | 05:55 |
120 | 14.6 - Creating the galleries controller | 09:35 |
121 | 14.7 - Implementing the Gallery create action | 06:26 |
122 | 14.8 - Gallery validators and normalizers | 15:23 |
123 | 14.9 - Requiring users to be logged in to view specific pages | 20:13 |
124 | 14.10 - Adding users to the request context | 15:57 |
125 | 14.11 - Rendering individual galleries | 35:59 |
126 | 14.12 - Galleries edit action | 13:39 |
127 | 14.13 - Galleries update action | 06:35 |
128 | 14.14 - GalleryService update method | 05:21 |
129 | 14.15 - Gallery delete action | 13:01 |
130 | 14.16 - Gallery index action | 22:26 |
131 | 14.17 - Navbar updates | 35:12 |
132 | 15.1 - File upload HTML form | 25:23 |
133 | 15.2 - File upload handler | 24:49 |
134 | 15.3 - The ImageService and create method | 18:50 |
135 | 15.4 - Finding images by Gallery ID | 16:04 |
136 | 15.5 - Rendering images | 21:58 |
137 | 15.6 - Deleting images | 32:52 |
138 | 16.1 - Error cleanup | 19:51 |
139 | 16.2 - Serving static assets | 07:59 |
140 | 16.3 - CSRF protection | 26:13 |
141 | 16.4 - Don't lookup the user when serving static assets | 06:36 |
142 | 16.5 - URL encoding image path bug | 07:46 |
143 | 16.6 - Starting with config variables | 23:06 |
144 | 16.7 - Functional options for services | 21:55 |
145 | 16.8 - Loading a JSON config | 15:54 |
146 | 16.9 - Setting up a droplet (server) on Digital Ocean | 06:29 |
147 | 16.10 - Installing postgres on our prod server | 07:53 |
148 | 16.11 - Caddy server | 13:36 |
149 | 16.12 - Deploy script | 32:28 |
150 | 17.1 - Deleting cookies and logging out users | 13:40 |
151 | 17.2 - Redirecting with alerts | 18:10 |
152 | 17.3 - Emailing users | 34:29 |
153 | 17.4 - Persisting form data | 12:26 |
154 | 17.5.1 - Resetting passwords - Creating the pwReset DB table | 29:17 |
155 | 17.5.2 - Resetting passwords - Implementing UserService methods | 24:30 |
156 | 17.5.3 - Resetting passwords - Form HTML templates | 05:08 |
157 | 17.5.4 - Resetting passwords - Controller updates | 20:59 |
158 | 17.5.5 - Resetting passwords - Sending emails and building links | 21:04 |
159 | 18.1 - Intro to OAuth | 06:34 |
160 | 18.2 - Setting up a Dropbox Application | 13:07 |
161 | 18.3 - Go's OAuth2 Package | 09:16 |
162 | 18.4 - Dropbox OAuth Spike (Part 1) | 16:28 |
163 | 18.5 - Dropbox OAuth Spike (Part 2) | 11:59 |
164 | 18.6.1 - Persisting OAuth Tokens - Explaining the Token | 09:22 |
165 | 18.6.2 - Persisting OAuth Tokens - OAuth Model | 35:46 |
166 | 18.6.3 - Persisting OAuth Tokens - Connecting It All | 13:17 |
167 | 18.7 - Making Dropbox API Calls | 17:51 |
168 | 18.8 - Refactoring to Controllers | 09:29 |
169 | 18.9 - Generic OAuth Controller | 12:23 |
170 | 19.1 - Intro to the Dropbox SDK | 20:08 |
171 | 19.2 - Custom Dropbox Package | 15:20 |
172 | 19.3 - Dropbox Chooser | 54:28 |
173 | Mark Bates - Go Buffalo | 33:53 |
174 | Joe Fitzgerald & Zac Bergquist - Atom + Go | 35:15 |
175 | Matt Holt - Caddy & Securing your Server | 27:18 |
176 | Michael Hartl - Learning Just Enough | 35:24 |
177 | Ryan Patterson - Technical Interviews | 28:35 |
178 | Mattan Griffel - Learning to Code | 42:36 |
179 | Charlie Guo - Doing the Unscalable | 41:20 |
180 | Ryan Jackson - Starting a Tech Company | 42:58 |
181 | Adam Wathan - Testing and More | 40:05 |
Similar courses to Learn to Create Web Applications using Go
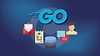
Working with Microservices in Go (Golang)
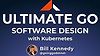
Ultimate Go: Software Design with Kubernetes
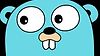
How to design & develop REST microservices in Golang (Go)
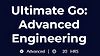
Ultimate Go: Advanced Engineering
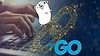
Golang: How to Build a Blockchain in Go Guide
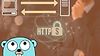
How to develop a productive HTTP client in Golang (Go)
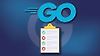
Introduction to Testing in Go (Golang)
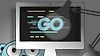
Go Bootcamp: Master Golang with 1000+ Exercises and Projects
![gRPC [Golang] Master Class: Build Modern API & Microservices](https://cdn.courseflix.net/courses/100x56/grpc-golang-master-class-build-modern-api-microservices.jpg)
gRPC [Golang] Master Class: Build Modern API & Microservices
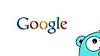