Building Modern Web Applications with Go (Golang)
Learn to write modern, fast, and secure web applications in Google's Go programming language, and learn it from an award winning University professor with 20 years of teaching experience, and 20 years of experience working in the industry as an entrepreneur. Go is a modern, type safe, compiled, and extremely fast programming language. It it is ideally suited for building safe, scalable, incredibly fast web applications.
Read more about the course
This course is well-suited for both absolute beginners, and for developers who already know something about web development, but want to add Go to their toolbox.
We start with an overview of the Go language, and then cover everything you need to get started writing web applications, including an overview of HTML5, a survey of JavaScript and JavaScript modules, how to work with Cascading Style Sheets to make our application look the way we want, and much more.
The major project in this course is building a bookings and reservation system for a Bed & Breakfast. Visitors to our site will be able to search for accommodations by date and make an online reservation, and the site owner will be able to manage reservations from a secure back end.
By the time you finish this course, you will have a solid grasp of what it takes to build a completely functional, secure, and fast web application from the ground up, and you will have a solid understanding of the Go programming language.
Watch Online Building Modern Web Applications with Go (Golang)
# | Title | Duration |
---|---|---|
1 | Introduction: who I am, and what we're going to do | 05:21 |
2 | Why Go? Why not PHP, or Python, or Node.js, or whatever? | 04:21 |
3 | Why Go? - System Resources | 03:09 |
4 | Installing Go, an IDE, and writing a simple program | 14:23 |
5 | Getting help: How to ask questions | 01:43 |
6 | A note about the terminal on Windows: Git Bash | 01:35 |
7 | Variables & Functions | 19:23 |
8 | Pointers | 06:54 |
9 | Types and Structs | 15:12 |
10 | Receivers: Structs with functions | 04:14 |
11 | Other data structures: Maps and Slices | 17:08 |
12 | Decision Structures | 13:18 |
13 | Loops and ranging over data | 09:23 |
14 | Interfaces | 08:34 |
15 | Packages | 05:04 |
16 | Channels | 11:40 |
17 | Reading and Writing JSON | 10:31 |
18 | Writing Tests | 15:17 |
19 | How web applications work: the request/response lifecycle | 04:55 |
20 | Making a "Hello, World" web application | 13:09 |
21 | Functions and handlers | 13:11 |
22 | Error checking | 06:11 |
23 | Serving HTML Templates | 09:14 |
24 | Reorganizing our code, and adding some basic styling to pages | 07:32 |
25 | Enabling Go Modules and refactoring our code to use packages | 09:38 |
26 | Working with Layouts, and building a template cache (part one) | 24:29 |
27 | Building a template cache (part two) | 08:23 |
28 | Setting application wide configuration | 06:42 |
29 | Why the application wide config is so useful | 01:30 |
30 | Optimizing our template cache by using an application config | 15:25 |
31 | Sharing data with templates | 15:00 |
32 | Using pat for routing | 06:50 |
33 | Using chi for routing | 07:00 |
34 | Developing our own middleware | 09:40 |
35 | Installing and setting up a sessions package | 14:53 |
36 | Experimenting with sessions | 06:45 |
37 | What are we going to build? | 04:10 |
38 | Setting up our project | 19:57 |
39 | Enabling static files | 08:02 |
40 | Creating pages as HTML | 14:08 |
41 | Creating a landing page | 45:17 |
42 | Creating a page for each room | 17:19 |
43 | Adding a form to search for availability | 11:52 |
44 | Improving our form | 06:37 |
45 | Creating the reservation page | 04:35 |
46 | What is Javascript, and why should I care? | 21:15 |
47 | Making a better date picker | 12:28 |
48 | Custom alerts using Notie | 08:30 |
49 | Creating modals with SweetAlert | 08:37 |
50 | Implementing a Javascript module | 06:07 |
51 | Adding custom alerts in our Javascript module | 08:50 |
52 | Using our Javascript module on the "Book Now" button | 16:34 |
53 | What is CSS, and how does it work? | 14:27 |
54 | Converting our pages to Go templates | 23:15 |
55 | Creating handlers for our forms & adding CSRF Protection | 15:36 |
56 | Creating a handler that return JSON | 12:09 |
57 | Sending and processing an AJAX request | 11:08 |
58 | Sending AJAX post and generalizing our custom function | 09:15 |
59 | Refactoring to use internal packages | 03:56 |
60 | Server-side form validation | 17:28 |
61 | Server side form validation II | 16:35 |
62 | Server side form validation III | 19:27 |
63 | Server Side form validation IV | 05:19 |
64 | Displaying a response to user after posting form data | 16:11 |
65 | Finishing up our response to user, and adding alerts | 09:31 |
66 | An aside: Alternate Templating Engines | 08:48 |
67 | Writing tests for our main package | 16:51 |
68 | Writing tests for our GET handlers | 22:37 |
69 | Writing tests for our POST handlers | 08:11 |
70 | Writing tests for our Render package I | 12:08 |
71 | Writing tests for our Render package II | 13:45 |
72 | Getting test coverage | 05:47 |
73 | Exercise: Writing tests for the Forms package | 04:55 |
74 | Solution to writing tests for the Forms package | 22:35 |
75 | Making running our application easier | 02:47 |
76 | Centralizing our error handling to a helpers package | 13:09 |
77 | Using our ClientError and ServerError helper functions | 05:25 |
78 | Updating our tests | 02:11 |
79 | Installing PostgreSQL | 03:58 |
80 | Connecting to the database with DBeaver on a Mac | 03:48 |
81 | Connecting to the database with DBeaver on Windows | 06:06 |
82 | Basic SQL syntax | 12:31 |
83 | More complex queries | 14:45 |
84 | Identifying database structure, and Entity Relationship Diagarams | 17:44 |
85 | Install Soda | 01:43 |
86 | Creating the users table using migrations | 11:57 |
87 | Creating the rest of our database using migrations | 07:17 |
88 | Setting up a foreign key | 04:10 |
89 | Setting up the rest of our foreign keys | 05:21 |
90 | Adding Indices & Exercise | 06:02 |
91 | Solution to Exercise | 06:38 |
92 | How to connect a Go application to a database | 33:17 |
93 | Creating a Driver package | 11:25 |
94 | Connecting to the database and adding the SQL connection to our Repository | 14:11 |
95 | Setting up models | 06:33 |
96 | Cleaning up our code | 05:54 |
97 | A word about ORMs | 02:45 |
98 | Setting up database functions: inserting a reservation | 17:08 |
99 | Testing our insert reservation function | 05:26 |
100 | Inserting Room Restrictions | 10:57 |
101 | Searching for availability by room | 15:56 |
102 | Searching for availablity for all rooms | 15:44 |
103 | Connecting our handlers to our new database functions | 18:21 |
104 | Connecting search availablity to the make reservation page | 11:03 |
105 | Cleaning up our make reservation page and testing everything | 16:40 |
106 | Cleaning up the reservation summary page and improving validation | 14:21 |
107 | Searching for availability by Room | 08:26 |
108 | Providing feedback when searching by room, and connecting to the reservation pag | 11:31 |
109 | Connecting the rooms page to the make reservation page | 09:36 |
110 | Connecting the Major's Suite page, and extracting our javascript module | 04:44 |
111 | Adding a migration for seeding rooms | 01:26 |
112 | Adding a migration for seeding restrictions | 01:28 |
113 | Creating a test database repository | 10:05 |
114 | Updating our existing tests to handle sessions | 15:02 |
115 | Improving our tests by handling multiple cases | 12:10 |
116 | Testing Post handlers | 28:21 |
117 | Testing AvailabilityJSON | 08:22 |
118 | Simplifying adding post parameters | 03:07 |
119 | Sending email using the Standard Library | 04:27 |
120 | Installing a mailer package and setting up a mail channel | 20:25 |
121 | Installing Mailhog on a Mac for testing purposes | 02:57 |
122 | Installing Mailhog on Windows for testing purposes | 00:57 |
123 | Creating and sending mail notifications | 05:29 |
124 | Solution to sending notification to property owner | 01:23 |
125 | Sending nicely formatted email using Foundation | 12:17 |
126 | Updating our tests | 04:20 |
127 | Create the login screen | 04:07 |
128 | Creating the authentication handlers for the login screen | 02:59 |
129 | Creating the authentication and user database functions | 17:47 |
130 | Creating our handler to log in | 09:20 |
131 | Writing Authentication Middleware | 06:49 |
132 | Adding a user to the database | 03:04 |
133 | Testing Login | 01:21 |
134 | Checking to see if a user is logged in, and logging a user out | 07:29 |
135 | Protecting our routes with authentication middleware | 04:40 |
136 | Picking an admin template | 03:30 |
137 | Convert the admin template into a Go template | 03:51 |
138 | Solution to creating admin templates | 04:59 |
139 | Create stub handlers for admin functionality | 05:53 |
140 | Listing all reservations | 24:57 |
141 | Listing new reservations | 08:13 |
142 | Showing one reservation | 24:01 |
143 | Database functions for editing a reservation | 06:24 |
144 | Editing a reservation | 07:14 |
145 | Marking a reservation as processed | 14:27 |
146 | Deleting a reservation | 06:11 |
147 | Showing the reservation calendar | 14:21 |
148 | Reservation Calendar II | 23:36 |
149 | Reservation Calendar III | 22:41 |
150 | Reservation Calendar IV | 14:51 |
151 | Handling Calendar changes I | 03:21 |
152 | Handling Calendar changes II | 05:54 |
153 | Handling Calendar changes III | 13:53 |
154 | Handling Calendar changes IV | 07:40 |
155 | Fixing our redirects | 19:11 |
156 | Updating our tests | 31:26 |
157 | Fixing a bug missed by our tests | 02:55 |
158 | A word about the updated tests | 01:15 |
159 | Changing our app to use command line flags | 10:25 |
160 | An alternative: the .env file | 01:14 |
161 | Using vi to edit files on remote server | 05:40 |
162 | Choosing a server platform | 08:18 |
163 | Add admin user to migrations | 02:22 |
164 | Connecting the application to the web server | 13:15 |
165 | Setting up Supervisor | 04:05 |
166 | Writing an update script for the server | 02:57 |
167 | A note about sending mail from the live server | 01:28 |
168 | Updating to Bootstrap 5 | 07:34 |
169 | Updating the footer and page title | 05:28 |
170 | Finishing touches, and fixing a bug | 07:20 |
171 | Populating the rest of the pages | 01:36 |
172 | Additional resources | 02:43 |
Similar courses to Building Modern Web Applications with Go (Golang)
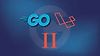
Let's Build a Go version of Laravel: Part Twoudemy
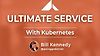
Ultimate Service 3.0ardanlabs.com
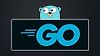
Go - The Complete GuideAcademind Pro
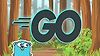
Go Programming (Golang): The Complete Developer's Guidezerotomastery.io
![Golang + Lambda Masterclass [EARLY-ACCESS]](https://cdn.courseflix.net/courses/100x56/golang-lambda-masterclass-early-access.jpg?d=1743580065165)
Golang + Lambda Masterclass [EARLY-ACCESS]Gourav Kumar
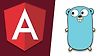
Angular and Golang: A Practical Guideudemy
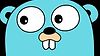
Introduction to industry REST microservices in Golang (Go)udemy
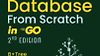
Build Your Own Database in Go From ScratchJames Smith
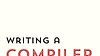
Writing A Compiler In GoThorsten Ball
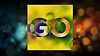