Working with Design Patterns in Go (Golang)
Go is a powerful language for building efficient and scalable applications. But as your projects grow, you'll encounter common problems that can be elegantly solved with design patterns.
This course will equip you with the knowledge and skills to leverage these design patterns effectively in your Go code. We'll explore various categories of patterns, including:
- Creational Patterns: Learn techniques for object creation that promote flexibility and decoupling.
- Structural Patterns: Discover ways to compose classes and objects to achieve desired functionality.
- Behavioral Patterns: Explore patterns that define communication and interaction between objects.
By understanding these patterns, you'll gain the ability to:
- Write cleaner, more maintainable, and reusable code.
- Improve the design and architecture of your Go applications.
- Solve common programming challenges with proven solutions.
- Communicate design concepts more effectively with other developers.
We'll approach design patterns with a practical lens, focusing on real-world Go scenarios. In fact, we'll build a simple web application that allows us to see how, why, and when you can use a particular design pattern to make your code more efficient, maintainable, and easy to understand. My goal is ensure that you'll gain hands-on experience implementing these patterns so that you can use them in your own projects.
So, whether you're a seasoned Go developer or just getting started with the language, this course will provide you with valuable tools to take your Go development skills to the next level.
Watch Online Working with Design Patterns in Go (Golang)
# | Title | Duration |
---|---|---|
1 | Introduction | 05:57 |
2 | A bit about me | 01:02 |
3 | Installing Go | 01:28 |
4 | Installing an Integrated Development Environment | 03:15 |
5 | Installing Docker | 00:52 |
6 | Asking for Help | 01:15 |
7 | Mistakes. We all make them. | 01:07 |
8 | What we'll build in this section | 00:59 |
9 | How web applications work | 02:13 |
10 | Setting up our main application | 09:53 |
11 | Installing a routing package | 02:31 |
12 | Setting up routes | 11:18 |
13 | Setting up a simple HTML template | 08:49 |
14 | Creating a render function | 16:37 |
15 | Rendering our first page | 04:33 |
16 | Adding navigation | 04:51 |
17 | Creating templates for all site pages | 05:25 |
18 | Setting up a route & handler for site pages | 05:49 |
19 | Trying out our template cache | 02:14 |
20 | Adding WebP and jpeg images to the home page | 22:48 |
21 | What we'll build in this section | 01:03 |
22 | The Factory Pattern | 06:24 |
23 | Setting up some types | 13:39 |
24 | Creating a simple Factory | 02:51 |
25 | Creating handlers for the simple Factory | 03:57 |
26 | Setting up the front end | 09:25 |
27 | Adding routes for our Factory handlers | 02:37 |
28 | Trying our Factory pattern out | 01:24 |
29 | The Abstract Factory pattern | 13:45 |
30 | Creating an Abstract Factory | 08:36 |
31 | Creating a route and handler for the Abstract Factory | 03:04 |
32 | Updating the front end | 05:09 |
33 | Trying out our Abstract Factory | 02:10 |
34 | What we'll build in this section | 00:36 |
35 | Adding a module to support MySQL/MariaDB | 01:26 |
36 | Setting up a local MariaDB instance with Docker | 08:23 |
37 | Connecting our application to MariaDB | 11:44 |
38 | What we'll build in this section | 01:28 |
39 | Writing our first database function | 12:15 |
40 | Creating a handler to list dog breeds | 07:33 |
41 | Updating the front end to fetch the list of dog breeds | 02:52 |
42 | Updating the front end to display the list of dog breeds | 14:19 |
43 | Writing a test for our handler | 10:23 |
44 | Implementing the Repository pattern I | 03:50 |
45 | Implementing the Repository pattern II | 03:37 |
46 | Implementing the Repository pattern III | 01:19 |
47 | Trying things out | 01:31 |
48 | Implementing a test database repository | 03:33 |
49 | Updating our handler test | 04:03 |
50 | What we'll build in this section | 00:52 |
51 | Creating a simple Singleton | 06:56 |
52 | Updating our project to use our configuration package (singleton) | 04:15 |
53 | What we'll build in this section | 01:14 |
54 | The Builder Pattern (& Fluent Interface) | 06:25 |
55 | Getting started with the Builder pattern in our project | 05:19 |
56 | Setting up our Builder code | 08:32 |
57 | Fixing three problems with our Builder code | 02:01 |
58 | Setting up a handler to use our Builder pattern | 05:33 |
59 | Setting up a route to our handler | 01:02 |
60 | Modifying the front end to call our Builder route | 06:12 |
61 | Challenge | 01:14 |
62 | Solution to challenge | 02:22 |
63 | What we'll cover in this section | 01:29 |
64 | The Adapter Pattern - Overview | 22:43 |
65 | Installing an application to serve JSON and XML | 03:34 |
66 | Setting up the Adapter type & the Adaptee | 07:28 |
67 | Adding the Adapter to our application config | 02:34 |
68 | Setting up a handler | 02:35 |
69 | Adding a route | 02:25 |
70 | Updating the Cat Breeds template | 02:03 |
71 | Switching adapters from JSON to XML | 09:24 |
72 | Adding a test for the Cat Breeds handler, using our adapter pattern | 05:00 |
73 | What we'll cover in this section | 01:14 |
74 | Creating a stub AnimalFromAbstractFactory handler | 02:55 |
75 | Creating a New factory function for pet with embedded breed | 04:53 |
76 | Creating stub newPetWithBreed method for Cats and Dogs | 08:07 |
77 | Adding a GetDogBreedByName method in the database Repository | 08:18 |
78 | Refactoring adapters.go to new package | 02:12 |
79 | Adding a GetCatBreedByName method on our JSON adapter | 04:05 |
80 | Adding a GetCatBreedByName method on our XML adapter | 03:15 |
81 | Finishing up the new Adapter and Abstract Factory code | 08:48 |
82 | Finishing up the changes in our Abstract Factory to use the new adapters | 02:58 |
83 | Setting up a route to our stub handler | 04:43 |
84 | Finishing off the handler code | 04:11 |
85 | Updating the front end to try things out | 07:04 |
86 | Writing the necessary javascript to call our back end | 04:22 |
87 | What we'll cover in this section | 01:30 |
88 | Setting up a route and a stub handler | 02:04 |
89 | Setting up the database table | 01:41 |
90 | Adding database methods for Dog of Month | 06:25 |
91 | Completing our DogOfMonth handler | 07:53 |
92 | Creating the Dog of Month template and trying things out | 07:08 |
93 | What we'll cover in this section | 02:57 |
94 | Worker Pool Overview | 08:08 |
95 | Setting up a Go Workspace to develop our new module | 02:31 |
96 | Getting started with the App and Streamer projects | 06:12 |
97 | Creating a Factory function for our Worker Pool | 07:56 |
98 | Getting started on the worker pool functionality | 03:06 |
99 | Setting up the Processor type | 03:11 |
100 | Setting up the Pool functionality | 12:18 |
101 | Creating Videos to send to the worker pool | 08:34 |
102 | Sending videos to the worker pool | 04:43 |
103 | Filling in the encode() function's logic | 13:10 |
104 | Trying things out and tracing through program execution | 12:57 |
105 | Installing ffmpeg | 02:20 |
106 | Adding logic to encode to MP4 | 06:06 |
107 | Trying out the EncodeToMP4 method | 02:49 |
108 | Adding logic to encode to HLS: Part One | 05:30 |
109 | Adding logic to encode to HLS: Part Two | 16:44 |
110 | Trying out our HLS encoder | 03:22 |
111 | Adding random filename generation to our videos | 02:46 |
112 | Trying out our worker pool with four videos | 07:01 |
113 | Adding streamer to our Breeders project | 06:57 |
Similar courses to Working with Design Patterns in Go (Golang)
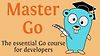
Master Goappliedgo.com (Christoph Berger)
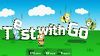
Testing with Gousegolang.com
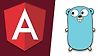
Angular and Golang: A Practical Guideudemy
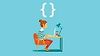
Go: The Complete Developer's Guide (Golang)udemyStephen Grider
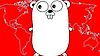
Web Development with Google’s Go (golang) Programming Languagegreatercommons.com
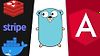
Angular and Golang: A Rapid Guide - Advancedudemy
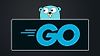
Go - The Complete GuideAcademind Pro
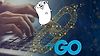
Golang: How to Build a Blockchain in Go Guideudemy
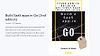
Build SaaS apps in Go (2nd edition)Dominic St-Pierre
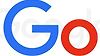