Let's Build a Go version of Laravel: Part Two
This is the follow up to "Let's Build a Go Version of Laravel," and is intended for students who have already taken that course! In the first part of this series, we built a re-usable Go module that gave us a lot of functionality, including html, json, and xml response types, support for Go templates and Jet templates to render pages, multiple database support, sessions, and more. This time around, we'll improve our Celeritas package and add the following functionality:
Read more about the course
Add support for remote file systems, including Amazon S3 buckets, Minio, sFTP, and WebDAV
Add support for Social Authentication using GitHub and Google (and you can add as many more as you like)
Add support for improved testing, including a Go version of Laravel's Dusk package, which takes a browser screen shot when testing functionality that renders a web page
Add support for "maintenance mode" using Remote Procedure Calls (RPC)
Improve our database migrations to support both raw SQL and soda's Fizz file format
Implement file upload functionality (with support for local and remote file systems)
Separate logic and routes for web and API
Make it easy for users to create tests by pre-populating stub test files and the appropriate setup_test.go files for their projects
By the time that you have completed this course, you will not only have a solid understanding of each of the things listed above, but also a reusable code base that will help you jump start your next project.
Watch Online Let's Build a Go version of Laravel: Part Two
# | Title | Duration |
---|---|---|
1 | Introduction | 03:02 |
2 | About me | 01:02 |
3 | Asking for help | 01:15 |
4 | Installing Go | 01:40 |
5 | Installing an IDE | 01:18 |
6 | Setting up the project | 05:57 |
7 | Making sure everything worked | 03:26 |
8 | What we're going to create | 02:26 |
9 | Setting up our remote file systems using Docker | 04:37 |
10 | Configuring Minio | 02:32 |
11 | Configuring sFTP | 03:09 |
12 | Setting up a type for file systems | 06:45 |
13 | Getting started with Minio: connecting and the Put function | 10:35 |
14 | Implementing the List function in Minio | 06:36 |
15 | Implementing the Delete function in Minio | 03:13 |
16 | Implementing the Get function in Minio | 03:23 |
17 | Creating stub filesystems for the other three types | 05:40 |
18 | Adding filesystems to Celeritas | 07:05 |
19 | Trying out our Minio filesystem | 04:14 |
20 | Creating a handler to list the remote file system | 10:52 |
21 | Connecting the handler to a route and trying things out | 01:39 |
22 | Creating handlers to display the upload form | 03:28 |
23 | Creating the handler to process the file upload | 11:13 |
24 | Creating the delete handler | 06:03 |
25 | Implementing the Put function for sFTP | 10:31 |
26 | Implementing the List function for sFTP | 04:05 |
27 | Implementing the Delete function for sFTP | 02:07 |
28 | Implementing the Get function for sFTP | 04:58 |
29 | Connecting Celeritas to our sFTP file system | 02:42 |
30 | Updating our ListFS handler to support sFTP | 02:12 |
31 | Updating our PostUploadToFS handler to support sFTP | 03:34 |
32 | Updating our DeleteFromFS handler to support sFTP | 01:20 |
33 | Cleaning up the Get function to avoid resource leaks | 03:56 |
34 | Implementing the Put function for WebDAV | 06:12 |
35 | Implementing the List function for WebDAV | 03:20 |
36 | Implementing the Delete function for WebDAV | 02:15 |
37 | Implementing the Get function for WebDAV | 03:55 |
38 | Testing things out | 06:25 |
39 | Implementing the List function for S3 file systems | 11:14 |
40 | Implementing the Put function for S3 file systems | 07:18 |
41 | Implementing the Delete function for S3 file systems | 05:28 |
42 | Implementing the Get function for S3 buckets | 03:52 |
43 | Connecting Celeritas to our S3 file system | 02:13 |
44 | Creating an S3 compatible bucket on Linode | 04:36 |
45 | Updating our handlers for S3 buckets | 01:40 |
46 | Trying things out | 03:14 |
47 | What we'll build | 01:38 |
48 | Adding file systems to the Celeritas type | 02:10 |
49 | Creating the file uploader | 06:52 |
50 | Limiting upload by mime type | 06:20 |
51 | Adding the mime type and file size limitations to the Celeritas config type | 06:02 |
52 | Setting up handlers and routes to try things out | 06:02 |
53 | Trying things out | 03:26 |
54 | Pop vs. SQL | 04:32 |
55 | Getting started with Pop functions for our migrations code in Celeritas | 03:39 |
56 | Implementing the CreatePopMigration() function to create up and down migrations | 02:37 |
57 | Implementing the RunPopMigrations() function | 02:21 |
58 | Implementing the PopMigrateDown() function | 03:26 |
59 | Implementing the PopMigrateReset() function | 01:22 |
60 | Making changes in the Celeritas CLI for our pop migrations | 10:25 |
61 | Trying out our new make migration command | 03:20 |
62 | Ensuring the database is connected before allowing people to make migrations | 03:42 |
63 | Creating a database.yml file and running migrations | 05:54 |
64 | Trying out the migrate command | 02:35 |
65 | Updating the "make auth" command for our Pop integration | 06:40 |
66 | Trying out make auth | 03:00 |
67 | Social Authentication or Single Sign On: an Overview | 03:38 |
68 | Getting started with Goth and Social Authentication | 03:38 |
69 | Setting up authentication routes | 04:10 |
70 | Initializing social sign on | 07:24 |
71 | Implementing the SocialLogin handler | 05:18 |
72 | Implementing the SocialCallback handler | 11:37 |
73 | Connecting our social authentication handlers to routes | 01:48 |
74 | Setting up GitHub for social authentication | 03:30 |
75 | Trying out the GitHub login functionality | 05:52 |
76 | Logging out | 05:22 |
77 | Really logging out | 09:51 |
78 | Trying the socialLogout function | 01:53 |
79 | Adding support for Google login | 04:01 |
80 | Updating the auth-handlers.go file for Google to enable login | 02:28 |
81 | Trying out login with Google | 02:01 |
82 | Adding the case for logging out of Google in socialLogout() | 01:59 |
83 | Trying things out | 01:30 |
84 | Separating Web and API routes | 05:07 |
85 | Getting started with "Maintenance Mode" functionality using RPC | 09:21 |
86 | Starting RPC | 00:58 |
87 | Adding maintenance mode middleware | 06:28 |
88 | Updating the CLI for maintenance mode | 05:12 |
89 | Testing the maintenance mode functionality | 03:39 |
90 | Graceful Shutdown | 08:01 |
91 | Adding a simple setup_test.go file to handlers | 09:34 |
92 | Adding two functions to our setup_test.go file | 02:53 |
93 | Adding and running a sample test | 04:44 |
94 | Adding some additional tests | 05:05 |
95 | Implementing Laravel Dusk like screen captures | 02:08 |
96 | Writing the screen capture function | 07:58 |
97 | Trying out the screen capture function | 04:45 |
98 | Writing additional helper functions for testing | 14:31 |
99 | Updating our templates in the CLI, and making some changes to the myapp | 08:15 |
100 | Creating our skeleton app | 04:33 |
101 | Additional updates to the skeleton application and the celeritas project | 04:31 |
102 | Trying out the "celeritas new <project>" command | 02:18 |
103 | Trying things out | 05:21 |
Similar courses to Let's Build a Go version of Laravel: Part Two
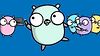
Web Authentication, Encryption, JWT, HMAC, & OAuth With Goudemy
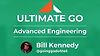
Ultimate Go: Advanced Engineering 2.0ardanlabs.com
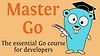
Master Goappliedgo.com (Christoph Berger)
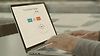
Advanced Branching and Looping in GOpluralsight
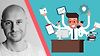
Mastering Multithreading Programming with Go (Golang)udemy
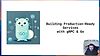
Building Production-Ready Services with gRPC and GoByteSizeGo
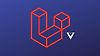
Master Laravel, Vue 3 & Inertia Full Stack 2023udemy
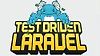