Go Programming (Golang): The Complete Developer's Guide
13h 10m 35s
English
Paid
Learn Golang from scratch, from an industry expert. Build real-world apps. You'll learn the fundamentals all the way to advanced concurrency so that you go from beginner to being able to get hired as a Go developer!
Read more about the course
- Write clean, bug free Go code using best practices
- Learn the entire ecosystem of a Go programmer
- Build massively concurrent programs that scale with Goroutines and Channels
- Master Go programming from fundamentals all the way to advanced concurrency using goroutines, channels, mutexes + more
- Learn one of the best programming languages for building performant distributed Microservices
- Build a Pixl Art cross platform desktop app (+ add it to your portfolio) that will wow employers
- Create a blazing fast GREP tool that uses multiple goroutines to search for a string within files
- Have the skills and understanding of Go to confidently apply for Go (Golang) programming jobs
Watch Online Go Programming (Golang): The Complete Developer's Guide
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Go Programming (Golang): The Complete Developer's Guide | 02:33 |
2 | So You Want To Learn Go? | 02:21 |
3 | Keiko Corp | 00:59 |
4 | Install Golang | 02:38 |
5 | Packages & Modules | 03:36 |
6 | Data Types | 05:09 |
7 | Strings / Runes | 07:37 |
8 | Go CLI | 01:47 |
9 | Variables | 10:32 |
10 | Demo: Variables | 09:56 |
11 | Exercise: Variables | 04:09 |
12 | Basic Functions | 05:53 |
13 | Demo: Functions | 04:04 |
14 | Exercise: Functions | 04:31 |
15 | Operators | 05:00 |
16 | if..else | 08:23 |
17 | Demo: if..else | 02:54 |
18 | Exercise: if..else | 05:44 |
19 | switch | 04:11 |
20 | Demo: switch | 03:43 |
21 | Exercise: switch | 03:39 |
22 | Looping | 05:05 |
23 | Demo: Looping | 03:56 |
24 | Exercise: Looping | 03:58 |
25 | Section Review: Dice Roller | 09:53 |
26 | Structures | 06:08 |
27 | Demo: Structures | 05:00 |
28 | Exercise: Structures | 05:23 |
29 | Arrays | 09:01 |
30 | Demo: Arrays | 04:57 |
31 | Exercise: Arrays | 05:12 |
32 | Slices | 12:03 |
33 | Demo: Slices | 04:07 |
34 | Exercise: Slices | 03:42 |
35 | Ranges | 04:47 |
36 | Maps | 06:02 |
37 | Demo: Maps | 07:01 |
38 | Exercise: Maps | 07:45 |
39 | Pointers | 05:53 |
40 | Demo: Pointers | 06:42 |
41 | Exercise: Pointers | 05:07 |
42 | Section Review: Library | 17:14 |
43 | Exercise: Imposter Syndrome | 02:56 |
44 | Receiver Functions | 05:31 |
45 | Demo: Receiver Functions | 04:28 |
46 | Exercise: Receiver Functions | 06:09 |
47 | iota | 06:17 |
48 | Exercise: iota | 04:38 |
49 | Variadics | 04:06 |
50 | Text Formatting: fmt | 06:12 |
51 | Packages | 09:16 |
52 | Init Function | 02:20 |
53 | Testing | 07:47 |
54 | Demo: Testing | 08:30 |
55 | Exercise: Testing | 06:56 |
56 | Interfaces | 09:38 |
57 | Demo: Interfaces | 04:03 |
58 | Exercise: Interfaces | 06:53 |
59 | Error Handling | 07:21 |
60 | Demo: Error Handling | 02:27 |
61 | Exercise: Error Handling | 08:10 |
62 | Readers & Writers | 09:04 |
63 | Demo Readers | 05:06 |
64 | Exercise: Readers | 04:43 |
65 | Type Embedding | 05:15 |
66 | Demo: Type Embedding | 05:48 |
67 | Exercise: Type Embedding | 05:20 |
68 | Generics | 08:27 |
69 | Demo: Generics | 09:43 |
70 | Exercise: Generics | 03:24 |
71 | Function Literals | 06:19 |
72 | Demo: Function Literals | 05:04 |
73 | Exercise: Function Literals | 05:31 |
74 | Defer | 03:15 |
75 | Concurrent Programming | 04:57 |
76 | Goroutines | 04:28 |
77 | Demo: Goroutines | 05:00 |
78 | Exercise: Goroutines | 05:56 |
79 | Channels | 09:33 |
80 | Demo: Channels | 09:57 |
81 | Exercise: Channels | 04:03 |
82 | Synchronization | 08:52 |
83 | Demo: WaitGroups | 04:38 |
84 | Demo: Mutexes | 05:42 |
85 | Exercise: Synchronization | 05:58 |
86 | Concurrency Patterns | 08:52 |
87 | Pattern: Pipelines | 14:43 |
88 | Pattern: Pipeline Cancellation/Quit | 04:09 |
89 | Pattern: Pipeline Fan-In | 06:04 |
90 | Pattern: Generator | 07:34 |
91 | Pattern: Context | 06:58 |
92 | Section Review: Multithreaded grep | 27:32 |
93 | gcc Installation | 04:43 |
94 | Intro & Project Setup | 03:09 |
95 | Canvas Overview & State | 05:00 |
96 | Creating a Swatch | 13:30 |
97 | Swatch Layout & First Run | 10:19 |
98 | Color Picker & App Layout | 04:07 |
99 | Pixel Canvas Structure | 08:37 |
100 | Pixel Canvas Renderer | 14:29 |
101 | Pixel Canvas Layout | 03:30 |
102 | Panning & Zooming | 10:05 |
103 | Painting Pixels | 09:52 |
104 | Cursor Display | 09:00 |
105 | Creating New Images | 11:18 |
106 | Saving Images | 06:48 |
107 | Loading Images | 05:39 |
108 | Project Setup | 01:47 |
109 | Creating Database Tables | 06:13 |
110 | Implementing CRUD Operations | 12:03 |
111 | JSON Data Processing Functions | 07:14 |
112 | JSON Endpoints | 10:23 |
113 | Server CLI | 06:36 |
114 | Testing JSON API | 05:16 |
115 | Protocol Buffers | 07:18 |
116 | gRPC Data Processing Functions | 04:46 |
117 | gRPC Endpoints | 14:29 |
118 | gRPC Client | 15:28 |
119 | Benchmarking | 04:06 |
120 | Thank You! | 01:02 |
Similar courses to Go Programming (Golang): The Complete Developer's Guide
![gRPC [Golang] Master Class: Build Modern API & Microservices](https://cdn.courseflix.net/courses/100x56/grpc-golang-master-class-build-modern-api-microservices.jpg?d=1743473633730)
gRPC [Golang] Master Class: Build Modern API & Microservicesudemy
Category: Golang (Google Go)
Duration 3 hours 46 minutes 30 seconds
Course
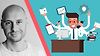
Mastering Multithreading Programming with Go (Golang)udemy
Category: Golang (Google Go)
Duration 5 hours 24 minutes 43 seconds
Course
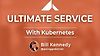
Ultimate Service 3.0ardanlabs.com
Category: Golang (Google Go), Kubernetes
Duration 13 hours 33 minutes 5 seconds
Course
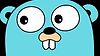
Introduction to industry REST microservices in Golang (Go)udemy
Category: Golang (Google Go)
Duration 15 hours 45 minutes 33 seconds
Course
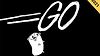
Go (Golang) Programming The Complete Go Bootcamp 2023udemy
Category: Golang (Google Go)
Duration 17 hours 49 minutes 29 seconds
Course
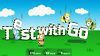
Testing with Gousegolang.com
Category: Golang (Google Go)
Duration 33 hours 16 minutes 48 seconds
Course
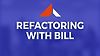
Refactoring With Billardanlabs.com
Category: Golang (Google Go)
Duration 7 hours 49 minutes 54 seconds
Course
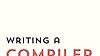
Writing A Compiler In GoThorsten Ball
Category: Golang (Google Go)
Duration
Book
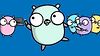
Web Authentication, Encryption, JWT, HMAC, & OAuth With Goudemy
Category: Golang (Google Go)
Duration 14 hours 3 minutes 23 seconds
Course