Let's Build a Go version of Laravel
Laravel is one of the most popular web web application frameworks in the PHP world, and with good reason. It's easy to use, well designed, and lets developers work on their applications without worrying about re-inventing the wheel every time they start a project. Go, often referred to as Golang, is one of the most popular programming languages in the world, and has been used to create systems at Netflix, American Express, and many other well known companies.
Read more about the course
It's extremely fast, type safe, and designed from the ground up to be used on the web.
This course is all about taking some of the most useful features found in Laravel, and implement similar functionality in Go. Since Go is compiled and type safe, web applications written in this language are typically much, much faster, and far less error prone that a similar application written in Laravel/PHP.
The key features we'll work on in this course include:
Implementing an Object Relation Mapper (ORM) that is database agnostic, and offers much of the functionality found in Laravel's Eloquent ORM.
A fully functional Database Migration system
Building a fully featured user authentication system that can be installed with a single command, which includes:
A password reset system
Session based authentication (for web based applications)
Token based authentication (for APIs and systems built with front ends like React and Vue)
A fully featured templating system (using both Go templates and Jet templates)
A complete caching system that supports Redis and Badger
Easy session management, with cookie, database (MySQL and Postgres), Redis stores
Simple response types for HTML, XML, JSON, and file downloads
Form validation
JSON validation
A complete mailing system which supports SMTP servers, and third party APIs including MailGun, SparkPost, and SendGrid
A command line application which allows for easy generation of emails, handlers, database models
Finally, the command line application will allow us to create a ready-to-go web application by tying a single command: celeritas new <myproject>
The only requirements for this course are:
A basic understanding of Go
A basic understanding of SQL databases
A Windows, Mac, or Linux computer
An internet connection
Docker
Visual Studio Code (or the IDE of your choice)
Watch Online Let's Build a Go version of Laravel
# | Title | Duration |
---|---|---|
1 | Introduction | 08:16 |
2 | A bit about me | 01:02 |
3 | How to ask for help | 01:15 |
4 | Install Go | 01:40 |
5 | Install VS Code | 01:18 |
6 | Install Make | 01:28 |
7 | Setting up project structure | 08:23 |
8 | Keeping our application and package in sync with Make | 04:19 |
9 | Starting work on Celeritas | 09:06 |
10 | Creating application folders | 05:45 |
11 | Creating and reading the .env file | 08:52 |
12 | Creating logs | 07:13 |
13 | Setting up Celeritas configuration | 03:30 |
14 | Getting a simple web server up and running | 11:16 |
15 | Implementing a page renderer in the Celeritas package (for Go templates) | 13:13 |
16 | Trying out the Go render functionality | 12:39 |
17 | Cleaning up Celeritas | 01:27 |
18 | Adding Jet support to our page rendering package | 09:35 |
19 | Rendering a Jet Template | 02:23 |
20 | Working with Jet Templates | 07:08 |
21 | Testing the render package | 09:10 |
22 | Writing more tests for the render package | 09:10 |
23 | Simplifying our tests using Table Tests | 08:27 |
24 | Implementing Sessions in Celeritas | 05:52 |
25 | Choosing and Installing a session package | 18:04 |
26 | Adding session middleware | 03:00 |
27 | Verifying that sessions work with myapp | 05:41 |
28 | Reading data from the session and passing it to the Jet template | 02:53 |
29 | Writing tests for the session package | 08:23 |
30 | Checking our Coverage | 02:06 |
31 | Installing Docker | 01:24 |
32 | Bringing up and tearing down a development environment using docker-compose | 06:49 |
33 | Getting started with Postgres | 06:44 |
34 | Building a Postgres connection string and connecting to the database | 10:47 |
35 | Trying out our database connection | 07:40 |
36 | Adding ORM like functionality to our application with upper/db | 07:57 |
37 | Creating a real users table and a user model | 20:38 |
38 | Additional database functions for the User type | 17:14 |
39 | Finishing up the database functions for the User model | 02:50 |
40 | Inserting a user | 06:13 |
41 | Testing other database functions on the User model | 13:24 |
42 | Creating a login page and handler | 13:58 |
43 | Creating the post handler for logging in | 18:41 |
44 | Adding functions to the Tokens model | 26:34 |
45 | Writing tests for models.go | 11:25 |
46 | Getting started with our integration tests | 17:55 |
47 | Creating tables in our test docker image, and running some tests | 18:51 |
48 | Continuing to write integration tests | 22:01 |
49 | Finishing up our integration tests | 34:28 |
50 | Cleaning up our tests | 03:59 |
51 | Setting up a simple CLI package in Celeritas | 15:48 |
52 | Adding support for migrations to the Celeritas package | 10:23 |
53 | Starting work on "make migration" in our CLI application | 07:58 |
54 | Using templates in our CLI | 09:23 |
55 | Trying out our make migration functionality | 05:08 |
56 | Running migrations | 13:39 |
57 | Trying out our "make migrate" commands with the Celeritas CLI | 07:04 |
58 | Getting started with Implementing "make auth" functionality | 07:57 |
59 | Trying out the make auth functionality | 03:01 |
60 | Continuing with the "make auth" functionality in our command line program | 07:55 |
61 | Creating simple auth middleware, and adding it to the "make auth" command | 12:30 |
62 | Installing our auth middleware with the celeritas command line utility | 03:14 |
63 | Trying out our improved make auth functionality | 04:46 |
64 | Implementing "make handler" functionality | 09:19 |
65 | Implementing "make model" functionality | 10:13 |
66 | Adding database stores to our sessions package | 11:26 |
67 | Adding support for database session store to the celeritas project | 06:12 |
68 | Supporting MySQL/MariaDB with"make auth" | 01:38 |
69 | Creating a validation package | 13:11 |
70 | Trying out our validation | 04:02 |
71 | Adding validation to models | 02:40 |
72 | Trying out our model validation | 02:08 |
73 | Building a simple form and performing validation on it | 08:22 |
74 | Building our PostForm handler with validation | 07:03 |
75 | Helper functions for the routes file | 04:03 |
76 | Helper functions for handlers | 07:14 |
77 | JSON, XML, and other response types | 09:46 |
78 | Creating handlers for our response types | 06:32 |
79 | Creating the routes and links for our response types | 04:59 |
80 | Ecryption/Decryption | 12:25 |
81 | Generating and getting our encryption key | 04:48 |
82 | Trying out our encryption functionality | 07:56 |
83 | Installing the necessary package and getting started | 10:23 |
84 | Connecting to Redis | 13:53 |
85 | Completing the rest of the cache functions | 18:50 |
86 | Testing the cache package | 19:42 |
87 | Trying out the cache in myapp | 13:07 |
88 | Finishing up our cache page in myapp | 10:39 |
89 | Adding a Redis store to our sessions package | 07:32 |
90 | CSRF Protection | 16:09 |
91 | Speeding up templates | 09:57 |
92 | Installing the necessary package and implementing necessary functions | 26:22 |
93 | Updating setup_test.go to create a Badger database for our tests | 04:30 |
94 | Writing and running tests for our Badger cache | 12:03 |
95 | Connecting to Badger | 08:06 |
96 | Trying out the Badger cache | 05:40 |
97 | Getting started sending email using SMTP | 17:00 |
98 | Adding the necessary packages, and completing sending email via SMTP | 16:47 |
99 | Sending email using Mailgun, SparkPost and more | 14:27 |
100 | Connecting Celeritas to our mailer package | 07:39 |
101 | Trying out or mailer package | 10:51 |
102 | Sending mail using an API | 04:39 |
103 | Adding "make mail" to the CLI | 06:16 |
104 | Testing mail | 28:07 |
105 | Setting up models and middleware for "remember me" functionality | 24:26 |
106 | Updating the auth handlers for remember me functionality | 12:54 |
107 | Trying out the remember me functionality | 02:59 |
108 | Password resets | 05:15 |
109 | Handling a password reset request | 10:35 |
110 | Sending a password reset link via email | 19:04 |
111 | Validating our signed link, and displaying the password reset form | 09:06 |
112 | Resetting the user's password | 08:29 |
113 | Updating the "make auth" functionality in the Celeritas CLI | 09:13 |
114 | Starting work on "celeritas new" in the CLI | 05:29 |
115 | Sanitizing the project name | 03:10 |
116 | Cloning a (currently non-existent) repository right in Go | 05:23 |
117 | Creating a skeleton application | 06:16 |
118 | Pushing our skeleton application to GitHub | 03:37 |
119 | Trying out the code that clones a remote GitHub repository | 05:09 |
120 | Removing the .git directory and creating a .env file | 07:23 |
121 | Creating the correct Makefile | 04:20 |
122 | Update go.mod | 05:01 |
123 | Update imports in .go files | 07:37 |
124 | Running go mod tidy | 04:49 |
125 | Pushing our Celeritas project to GitHub | 02:24 |
126 | Trying out our make new functionality | 09:14 |
127 | Where to go from here | 02:55 |
Similar courses to Let's Build a Go version of Laravel
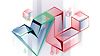
Laravel Backends for Vue.js 3vueschool.io
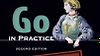
Go in Practice, Second EditionMatt ButcherMatt FarinaNathan Kozyra
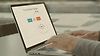
Advanced Branching and Looping in GOpluralsight
![Golang + Lambda Masterclass [EARLY-ACCESS]](https://cdn.courseflix.net/courses/100x56/golang-lambda-masterclass-early-access.jpg?d=1743457046281)
Golang + Lambda Masterclass [EARLY-ACCESS]Gourav Kumar
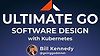
Ultimate Go: Software Design with Kubernetesardanlabs.com
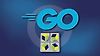
Working with Design Patterns in Go (Golang)udemy
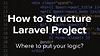
How to Structure Laravel Projectlaraveldaily.com
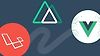
Vue 3, Nuxt.js and Laravel: A Practical Guideudemy
![gRPC [Golang] Master Class: Build Modern API & Microservices](https://cdn.courseflix.net/courses/100x56/grpc-golang-master-class-build-modern-api-microservices.jpg?d=1743457046281)
gRPC [Golang] Master Class: Build Modern API & Microservicesudemy
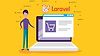