TypeScript Pro Essentials
You've tested the waters with TypeScript, but deep down you know that you're missing something. You've heard that TypeScript is the answer to all the problems that come with JavaScript– things like clunky autocompletion, runtime errors, and scaling for large projects. So why do you still feel like you're not getting the most out of TypeScript? You keep hitting type-related roadblocks, struggle with configuration, and feel limited by your understanding of the more advanced features. You know it's time to push yourself further, but you're not sure where to start. What you've heard about TypeScript is true. It will help you catch errors before they happen, write cleaner code, and refactor with confidence. But to get there, you need a guide.
Read more about the course
Total TypeScript Pro Essentials is what you've been looking for. This workshop is your map to becoming a TypeScript Wizard.
With over 200 lessons in 17 sections, this workshop will help you build a deep understanding of TypeScript at every level.
From configuration and IDE setup, to advanced type manipulation and best practices for application development, Total TypeScript Pro Essentials has it all. Whether you're brand new to TypeScript or have been using it for years, you are sure to take away valuable knowledge and skills from this workshop.
Here’s what’s included:
Introduction to TypeScript
Kickstart your TypeScript setup with a refresher on the core benefits and concepts of TypeScript: initial configuration, the TypeScript compiler, and type inference.
Follow the recommended setup and extensions for your editor, with a focus on Visual Studio Code, and become familiar with features like navigation, autocompletion, refactoring, and writing self-documenting code.
Understand TypeScript's role in the build process, and why browsers need help understanding the syntax. Structure your project to work nicely with the compiler, and configure for working with modern build tools like Vite and hot module replacement (HMR). Practice setting up type checking in a CI/CD pipeline, preventing errors from reaching production.
TypeScript Fundamentals
Reinforce your foundational skills by working with TypeScript's essential types and annotations. You'll work with function parameters and return types, and model data with object literal types, arrays, and tuples. Write reusable type aliases, correctly type async code, and handle data that comes from external sources. Create more flexible types by using unions, intersections, and interfaces.
Write more expressive code that refines types through several narrowing techniques, and recognize the role of any, never, and unknown in your codebase.
Objects, Classes, and Mutability
Types, interfaces, and classes all have their place in TypeScript. Learn the differences between them, and how to choose the right structure for your needs. Work with dynamic data in objects, and and overcome index signature limitations by using mapped types. Practice using essential type helpers like Pick, Omit, and Partial, and how to build your own helper when you need to.
Develop the mental model for how mutability affects type inference and safety. Recognize the benefits of immutable data structures, and how to work around their quirks.
Apply object-oriented programming principles to your TypeScript code. Understand the role of classes, constructors, and access modifiers, and how to use inheritance and composition to build complex systems that follow real-world design patterns.
Working with the Compiler
Harness the power of the TypeScript compiler to derive types from values, while furthering your understanding of type inference. Create new kinds of type aliases, and use indexed access types to target nested properties.
Recognize when TypeScript needs your help through type annotations and assertions, and the purpose of as, asserts, and satisfies. Apply these techniques to real-world scenarios like working with DOM APIs, parsing JSON, and creating configuration objects.
Understanding the Environment
Distinguish between TypeScript modules and scripts, and prevent global scope pollution. Provide types for JavaScript files and third-party libraries, and understand the role of ambient declarations.
Safely modify global interfaces like Window through declaration merging, work with namespaces like JSX, and understand when to use .d.ts type definition files vs. modules.
Learn best practices for configuring TypeScript's behavior based on the type of project you're working on. Adjust strictness levels, module resolution, and generate type declaration files. Use TypeScript as a linter, and optimize build performance for large projects.
Advanced Application Development
Understand the core differences between CommonJS (CJS) and ES Modules (ESM), and how TypeScript handles module syntax. Learn to use file extensions for guiding module resolution, and configure TypeScript settings for more consistent behavior. Make informed decisions about when to adopt ESM into your projects, and build libraries that support both CJS and ESM consumers for maximum compatibility.
Practice domain modeling by representing real-world concepts and encoding business logic within your types. Establish single sources of truth for type definitions, and create reusable generic type utilities. Enforce stricter type checking with well-placed constraints.
Combine generics and function overloads for maximum flexibility, and create custom type guards and predicates to enhance type inference. Create type-safe APIs with template literal types, mapped types, and custom error types.
Watch Online TypeScript Pro Essentials
# | Title | Duration |
---|---|---|
1 | 001 TypeScript's Relationship with JavaScript | 04:25 |
2 | 002 JavaScript vs. TypeScript in the Build Process | 02:55 |
3 | 003 Essential Tools for TypeScript Development | 01:43 |
4 | 004 Set up Node.js and VS Code | 01:33 |
5 | 005 Installing pnpm for Package Management | 03:01 |
6 | 006 Installing t | 01:19 |
7 | 007 Unexpected Token Error in the Browser | 00:48 |
8 | 008 Browsers Can't Understand TypeScript Syntax | 01:20 |
9 | 009 Convert a TypeScript File into a JavaScript File | 01:06 |
10 | 010 Compile TypeScript to JavaScript with the TypeScript CLI | 04:05 |
11 | 011 Automating TypeScript Compilation | 00:48 |
12 | 012 Use tsc's Watch Mode to Automatically Compile TypeScript Files | 01:24 |
13 | 013 Compiling TypeScript Files to a Directory | 01:09 |
14 | 014 Configure the Output Directory for Compiled Files | 01:11 |
15 | 015 Use Vite for a Frontend App with TypeScript | 01:47 |
16 | 016 Configure Vite for a Vanilla TypeScript Project | 02:56 |
17 | 017 Comparing Vite with tsc | 01:03 |
18 | 018 TypeScript as a Linter | 03:33 |
19 | 019 TypeScript in a CI-CD System | 03:41 |
20 | 020 Should TypeScript Block Your Dev Server_ | 04:47 |
21 | 021 Quickly Create Scripts with tsx | 01:44 |
22 | 022 Understanding Function Errors | 00:51 |
23 | 023 Fixing Errors in the | 01:05 |
24 | 024 Annotating Empty Parameters | 00:37 |
25 | 025 Annotating Function Parameters | 01:15 |
26 | 026 Basic Types in TypeScript | 00:56 |
27 | 027 TypeScript Basic Types | 01:37 |
28 | 028 Optional Function Parameters | 00:51 |
29 | 029 Optional Function Parameters in TypeScript | 00:57 |
30 | 030 Default Parameters in JavaScript Functions | 00:45 |
31 | 031 Add a Default Parameter | 01:27 |
32 | 032 Typing Object Literals | 00:43 |
33 | 033 Annotating with {} | 01:47 |
34 | 034 Making Object Property Types Optional | 00:52 |
35 | 035 Specifying Optional Properties | 00:55 |
36 | 036 A Single Source of Truth for Type Definitions | 00:53 |
37 | 037 Creating | 01:30 |
38 | 038 Add an Array to an Object | 00:53 |
39 | 039 Two Syntaxes for Arrays | 01:46 |
40 | 040 Arrays of Objects in TypeScript | 00:48 |
41 | 041 Representing an Array of Objects | 02:12 |
42 | 042 Rest Parameters in TypeScript | 00:37 |
43 | 043 Using Rest Parameters in TypeScript | 01:01 |
44 | 044 Tuples for Precise Array Structures in TypeScript | 01:20 |
45 | 045 Using Tuple Syntax in TypeScript | 01:22 |
46 | 046 Using Optional Tuple Members in TypeScript | 00:50 |
47 | 047 Working with Optional Tuple Members in TypeScript | 01:34 |
48 | 048 The Controversial | 01:07 |
49 | 049 The Dangers of Using _any_ | 02:06 |
50 | 050 Function Types | 01:25 |
51 | 051 Function Types for Type Aliases in TypeScript | 01:45 |
52 | 052 Typing an Event Listener | 00:46 |
53 | 053 The | 01:52 |
54 | 054 Restricting | 00:50 |
55 | 055 Creating Typed Sets | 02:16 |
56 | 056 Type Checking Maps | 00:52 |
57 | 057 Adding Types to Maps | 01:48 |
58 | 058 Debugging JSON Parsing | 00:39 |
59 | 059 Decoding the JSON.parse Error | 01:20 |
60 | 060 Typing Fetch API Responses in Async Functions | 01:11 |
61 | 061 Adding Types to an Async Function | 02:27 |
62 | 062 Understanding How TypeScript Works in Your IDE | 01:16 |
63 | 063 Introspecting Variables and Declarations in TypeScript | 01:19 |
64 | 064 Hovering Over a Function Call | 00:28 |
65 | 065 Fixing Errors with Hovering | 01:32 |
66 | 066 Adding Documentation for Hovers | 00:33 |
67 | 067 TSDoc Comments | 01:41 |
68 | 068 Manually Triggering Autocomplete | 01:19 |
69 | 069 The Control + Space Shortcut | 01:32 |
70 | 070 TypeScript's Approach to Errors | 03:43 |
71 | 071 Quick Renaming in VS Code | 01:51 |
72 | 072 Navigating Code with _Go to Definition_ | 02:29 |
73 | 073 Fast Imports in VS Code | 00:53 |
74 | 074 Using Autocomplete and Quick Fix to Import Variables | 02:12 |
75 | 075 Organizing Imports in Large Files | 00:39 |
76 | 076 The Organize Imports Hotkey in VS Code | 01:12 |
77 | 077 Quick Fix Refactoring | 00:54 |
78 | 078 Refactoring with Quick Fixes | 01:44 |
79 | 079 Automatic Code Formatting with Prettier | 02:08 |
80 | 080 Restarting the TypeScript Server in VS Code | 01:17 |
81 | 081 Handling Null Values in TypeScript | 01:01 |
82 | 082 Introducing Union Types in TypeScript | 01:14 |
83 | 083 Diving Deeper into Unions and Assignability | 00:49 |
84 | 084 Restricting Function Parameters | 00:55 |
85 | 085 Combining Literal & Union Types | 01:57 |
86 | 086 Literal Type Assignability | 01:06 |
87 | 087 Combining Union Types in TypeScript | 01:29 |
88 | 088 Create New Types By Combining Unions | 01:08 |
89 | 089 How Big Can a Union Be_ | 01:11 |
90 | 090 Resolving Literal Types to Wider Types | 02:21 |
91 | 091 Narrowing Unions with | 02:12 |
92 | 092 Conditional Narrowing in TypeScript | 01:09 |
93 | 093 Different Approaches for Narrowing Inputs | 02:50 |
94 | 094 Narrowing with Boolean Won't Work | 01:05 |
95 | 095 Gotchas When Narrowing a Map in TypeScript | 01:00 |
96 | 096 Correctly Narrowing a Map in TypeScript | 01:41 |
97 | 097 Narrowing by Throwing Errors | 00:59 |
98 | 098 Throwing Errors to Narrow | 01:43 |
99 | 099 Narrowing with | 01:30 |
100 | 100 Dynamically Handling Different API Responses in TypeScript | 01:41 |
101 | 101 Introducing the Unknown Type in TypeScript | 02:08 |
102 | 102 Dealing with Unknown Errors in TypeScript | 01:40 |
103 | 103 Narrowing with | 02:15 |
104 | 104 Narrowing Unknown in a Large Conditional Statement | 01:16 |
105 | 105 Narrowing Unknown Types in TypeScript | 02:56 |
106 | 106 Introducing the | 02:24 |
107 | 107 Solving the Never Type in TypeScript | 00:28 |
108 | 108 Empty Arrays and the Never Type in TypeScript | 01:46 |
109 | 109 Narrowing Return Types with TypeScript | 01:31 |
110 | 110 Returning | 02:39 |
111 | 111 Narrowing in Different Scopes | 01:29 |
112 | 112 Narrowing Behavior Across Scopes | 04:08 |
113 | 113 Handling Separate But Related Types | 01:42 |
114 | 114 Improving Type Safety with Discriminated Unions in TypeScript | 04:17 |
115 | 115 Destructuring a Discriminated Union in TypeScript | 00:53 |
116 | 116 Limitations of Destructuring Discriminated Unions | 02:59 |
117 | 117 Narrowing a Discriminated Union with a Switch | 01:05 |
118 | 118 Refactoring to a Switch Statement | 02:20 |
119 | 119 The switch (true) Pattern in TypeScript | 01:21 |
120 | 120 Refining Types with Discriminated Unions of Tuples | 01:50 |
121 | 121 Handle Error and Success States by Narrowing | 02:42 |
122 | 122 Discriminated Booleans | 00:55 |
123 | 123 Discriminated Unions with Boolean Discriminators | 01:09 |
124 | 124 Adding Defaults to a Discriminated Union | 00:59 |
125 | 125 Handling Optional Parameters in Discriminated Unions | 03:52 |
126 | 126 Should You Provide Function Return Types_ | 04:51 |
127 | 127 Extending Objects in TypeScript | 02:09 |
128 | 128 Use the Intersection Operator to Extend an Object Type in TypeScript | 00:49 |
129 | 129 Extend an Object Using Interfaces in TypeScript | 00:54 |
130 | 130 Extending Interfaces to Create Hierarchies in TypeScript | 01:50 |
131 | 131 Extending Incompatible Properties | 00:53 |
132 | 132 Comparing Intersections and Interface Extends | 03:20 |
133 | 133 Comparing Intersection and Interface Extends in TypeScript | 02:11 |
134 | 134 Allow Dynamic Keys in TypeScript Types | 01:00 |
135 | 135 Dynamic Keys with Index Signatures and Record Types | 03:42 |
136 | 136 Allow Any String Key while Supporting Default Properties | 00:41 |
137 | 137 Add an Index Signature to an Interface | 01:33 |
138 | 138 Supporting Different Types of Keys in TypeScript | 01:46 |
139 | 139 Using the PropertyKey Type in TypeScript | 00:51 |
140 | 140 Restricting Object Keys in TypeScript | 01:09 |
141 | 141 Records and Mapped Types in TypeScript | 02:52 |
142 | 142 An Issue with Duplicate Interfaces | 00:51 |
143 | 143 Declaration Merging in TypeScript Interfaces | 03:09 |
144 | 144 Working with Partial Data from a Type | 01:37 |
145 | 145 Manage Subtypes with the Pick Utility Type | 02:56 |
146 | 146 Exclude a Property from an Interface | 00:50 |
147 | 147 The Omit Utility Type in TypeScript | 01:29 |
148 | 148 A Quirk of Omit in TypeScript | 01:49 |
149 | 149 Understanding Distributive Omit and Pick in TypeScript | 03:06 |
150 | 150 Excluding Fields from a TypeScript Type | 00:55 |
151 | 151 The Partial Utility Type in TypeScript | 01:08 |
152 | 152 Making Type Properties Required in TypeScript | 00:48 |
153 | 153 Specifying a Type with Shared Properties in TypeScript | 01:24 |
154 | 154 Common Keys of Unions of Objects in TypeScript | 01:28 |
155 | 155 Fixing a Type Assignment Inference Error | 01:02 |
156 | 156 Inference differences between let and const | 02:10 |
157 | 157 Object Property Inference | 00:49 |
158 | 158 Understanding Object Properties and Assignability in TypeScript | 02:44 |
159 | 159 Creating Read-only Properties | 00:28 |
160 | 160 Specifying Read-only Object Properties | 01:03 |
161 | 161 Using a Type Helper to Create Read-only Properties | 00:52 |
162 | 162 Use the Readonly Type Helper to Create Read-Only Objects in TypeScript | 00:50 |
163 | 163 Deeply Apply Read-only Properties to an Object in TypeScript | 01:23 |
164 | 164 Using as const to Deeply Apply Read-only Properties to an Object in TypeScript | 01:18 |
165 | 165 Comparing Object.freeze with as const | 00:55 |
166 | 166 Deeply Apply Read-only Properties with as const | 01:36 |
167 | 167 Prevent Array Mutation in TypeScript | 00:47 |
168 | 168 Two Ways to Create Read-Only Arrays in TypeScript | 01:37 |
169 | 169 Distinguishing Assignability Between Read-Only and Mutable Arrays | 02:02 |
170 | 170 Fixing Unsafe Tuples | 01:32 |
171 | 171 Specifying a readonly Tuple | 01:54 |
172 | 172 Use the ts-reset Library to Improve Readonly Array Handling in TypeScript | 02:11 |
173 | 173 Improve Type Inference for an Async Function | 01:20 |
174 | 174 Two Options for Improving Promise Return Types in TypeScript | 02:03 |
175 | 175 Infer Strings as Their Literal Types in Objects with as const | 01:55 |
176 | 176 Classes in TypeScript | 00:51 |
177 | 177 Creating a Class with Default Properties | 02:46 |
178 | 178 Implement Class Methods in TypeScript | 00:55 |
179 | 179 Declaring Methods in TypeScript Classes | 01:31 |
180 | 180 Creating Constructors for Classes in TypeScript | 00:36 |
181 | 181 Receive Arguments in Class Constructors | 01:42 |
182 | 182 Getter Methods in TypeScript | 01:01 |
183 | 183 Adding a Getter to a Class in TypeScript | 01:42 |
184 | 184 Public and Private Properties in TypeScript | 00:54 |
185 | 185 Prevent Access to Private Properties in Classes | 01:54 |
186 | 186 Setter Methods in TypeScript | 00:54 |
187 | 187 Add a Setter to a TypeScript Class | 01:26 |
188 | 188 Extending Classes in TypeScript | 01:32 |
189 | 189 Inheriting Properties from Other Classes in TypeScript | 04:12 |
190 | 190 Overriding Methods in TypeScript | 03:05 |
191 | 191 Ensure a Class Adheres to a Contract | 00:47 |
192 | 192 Implementing Interfaces in TypeScript with Classes | 02:15 |
193 | 193 Using | 01:10 |
194 | 194 Annotating | 02:54 |
195 | 195 Intro to TypeScript-only Features | 00:53 |
196 | 196 Parameter Properties in TypeScript | 01:43 |
197 | 197 Working with Enums in TypeScript | 01:46 |
198 | 198 Create an Enum in TypeScript | 02:51 |
199 | 199 String Enums in TypeScript | 01:15 |
200 | 200 Creating a String Enum in TypeScript | 02:28 |
201 | 201 Const Enums in TypeScript | 04:16 |
202 | 202 Namespaces in TypeScript | 02:52 |
203 | 203 Merging Namespaces in TypeScript | 01:31 |
204 | 204 Declaration Merging with Interfaces Inside of Namespaces | 01:15 |
205 | 205 When to Prefer ES Features to TS Features | 04:43 |
206 | 206 Introduction to Deriving Types in TypeScript | 00:44 |
207 | 207 The keyof Operator | 00:58 |
208 | 208 Flowing Types with the keyof Operator | 01:23 |
209 | 209 The typeof Operator | 01:34 |
210 | 210 Combine keyof and typeof to Derive Types | 02:21 |
211 | 211 No Creating Runtime Values from Types | 02:04 |
212 | 212 Deriving Types with Classes | 00:58 |
213 | 213 Using typeof with Classes in TypeScript | 02:16 |
214 | 214 Enums as Types and Values in TypeScript | 00:53 |
215 | 215 The this Keyword in TypeScript | 01:38 |
216 | 216 Using the Same Name for Values and Types in TypeScript | 02:10 |
217 | 217 Creating Types from Complex Function Parameters | 01:38 |
218 | 218 Use the Parameters Type to Extract Function Parameters | 01:39 |
219 | 219 Extracting Return Types from Functions | 00:34 |
220 | 220 Using the ReturnType Type Helper | 00:43 |
221 | 221 Extract the Return Type from an Async Function | 00:47 |
222 | 222 Unwrap Promises with the Awaited Type Helper | 01:01 |
223 | 223 Access Specific Values in an as const Object | 01:22 |
224 | 224 Use an Indexed Access Type to Access a Property | 02:23 |
225 | 225 Pass a Union to an Indexed Access Type | 00:43 |
226 | 226 Unions and Indexed Access Types in TypeScript | 01:56 |
227 | 227 Extract a Union of All Values from an Object | 00:39 |
228 | 228 Pass keyof into an Indexed Access Type | 02:38 |
229 | 229 Create a Union from an as const Array | 01:23 |
230 | 230 Pass a Primitive to an Indexed Access Type | 02:04 |
231 | 231 Required vs Unnecessary Annotations | 00:42 |
232 | 232 Simplifying TypeScript Annotations | 04:18 |
233 | 233 Provide Additional Information to TypeScript | 01:12 |
234 | 234 The _as_ and _as any_ Keywords in TypeScript | 04:11 |
235 | 235 Global Typings use any | 01:08 |
236 | 236 Preventing 'any' Type Issues from Global Typings | 03:18 |
237 | 237 The Limits of as in TypeScript | 02:22 |
238 | 238 Non-null Assertions | 01:02 |
239 | 239 Adding a Non-null Assertion | 03:16 |
240 | 240 The @ts-ignore Directive in TypeScript | 03:16 |
241 | 241 The @ts-expect-error Directive in TypeScript | 03:34 |
242 | 242 The @ts-nocheck Directive in TypeScript | 01:47 |
243 | 243 Improve Type Annotations with the satisfies Operator | 01:33 |
244 | 244 The satisfies Operator in TypeScript | 02:29 |
245 | 245 Using satisfies with keyof and typeof in TypeScript | 00:37 |
246 | 246 Specify Type Constraints with the satisfies Operator | 01:31 |
247 | 247 Comparing as, satisfies, and Variable Annotations in TypeScript | 01:30 |
248 | 248 When to Use as, satisfies, and Variable Annotations in TypeScript | 04:15 |
249 | 249 The satisfies Keyword and Deeply Read-Only Objects in TypeScript | 01:04 |
250 | 250 Combine as const with satisfies for Read-Only Objects | 02:01 |
251 | 251 Accept Anything Except Null or Undefined | 00:52 |
252 | 252 The Empty Object Type | 03:03 |
253 | 253 Typing a Truly Empty Object | 01:00 |
254 | 254 Handling a Truly Empty Object in TypeScript | 02:45 |
255 | 255 Excess Property Warnings in TypeScript | 01:08 |
256 | 256 Techniques for Triggering Excess Property Warnings in TypeScript | 04:03 |
257 | 257 Excess Properties in Functions | 01:11 |
258 | 258 Understanding Unexpected Properties in TypeScript | 03:15 |
259 | 259 Object.keys and Object.entries in TypeScript | 03:47 |
260 | 260 Iterating Over Object Keys in TypeScript | 01:14 |
261 | 261 Techniques for Iterating Over Object Keys | 03:42 |
262 | 262 Evolving Types using the | 01:03 |
263 | 263 The Evolving | 01:44 |
264 | 264 The Evolving | 01:42 |
265 | 265 Function Parameter Comparisons in TypeScript | 00:53 |
266 | 266 Understanding TypeScript's Function Parameter Comparisons | 03:09 |
267 | 267 Typing Functions with Object Params | 00:53 |
268 | 268 Typing Arrays of Functions with Object Params | 01:40 |
269 | 269 Properly Typing an Object of Functions | 01:48 |
270 | 270 Handling Union of Functions with Incompatible Types in TypeScript | 02:13 |
271 | 271 Unions of Function Return Types | 01:41 |
272 | 272 Annotating the Errors Thrown by a Function | 01:13 |
273 | 273 Techniques for Annotating Errors in TypeScript | 02:15 |
274 | 274 Understanding Modules and Scripts in TypeScript | 02:27 |
275 | 275 Enforcing Module Usage in TypeScript | 00:47 |
276 | 276 Update tsconfig for Modules | 01:12 |
277 | 277 Declaration Files in TypeScript | 01:40 |
278 | 278 Declaration Files Can Be Modules or Scripts | 02:27 |
279 | 279 Using Declaration Files with JavaScript in TypeScript | 00:57 |
280 | 280 Typing a JavaScript Module | 02:29 |
281 | 281 Type Variables Declared Elsewhere | 01:16 |
282 | 282 Ambient Context and the declare Keyword in TypeScript | 02:32 |
283 | 283 Global Scope Declarations in TypeScript | 00:58 |
284 | 284 Understanding Global Declarations in TypeScript | 02:39 |
285 | 285 Configuring the lib Compiler Option | 00:32 |
286 | 286 Setting a Compiler Target in tsconfig.json | 05:14 |
287 | 287 DOM Typing Configuration in TypeScript | 00:34 |
288 | 288 Update tsconfig.json to include DOM typings | 02:16 |
289 | 289 Update DOM Typing to Include Iterable | 00:39 |
290 | 290 Configuring TypeScript for DOM Iteration | 01:22 |
291 | 291 Modifying TypeScript Global Scope | 01:27 |
292 | 292 Adding Types to the Global Scope | 02:58 |
293 | 293 Adding Type Definitions for External Libraries | 00:38 |
294 | 294 Install Type Definitions from DefinitelyTyped | 03:46 |
295 | 295 Integrating TypeScript with Node | 01:11 |
296 | 296 Modifying process.env Typing in TypeScript | 02:13 |
297 | 297 Updating Node.ProcessEnv | 03:17 |
298 | 298 Types that Ship With Libraries | 01:43 |
299 | 299 Declaring Module Types in TypeScript | 01:01 |
300 | 300 The declare module Syntax in TypeScript | 01:42 |
301 | 301 Importing and Typing Non-Code Files in TypeScript | 00:59 |
302 | 302 Use Wildcards in TypeScript Module Declarations | 02:29 |
303 | 303 TSConfig Options and Declaration Files | 00:59 |
304 | 304 Understanding skipLibCheck in TypeScript | 04:04 |
305 | 305 Should You Use Declaration Files to Store Your Types_ | 02:30 |
306 | 306 Recommended Base Configuration | 03:26 |
307 | 307 Understanding Isolated Modules in TypeScript | 03:27 |
308 | 308 Index Access Settings | 00:51 |
309 | 309 Making Code Safer with No Unchecked Indexed Access | 01:30 |
310 | 310 Using TypeScript as a Linter | 01:11 |
311 | 311 The noEmit Option in tsconfig.json | 02:06 |
312 | 312 Module NodeNext with Extensions | 04:22 |
313 | 313 The moduleResolution Option in tsconfig.json | 05:08 |
314 | 314 Enforcing Import Syntax | 01:07 |
315 | 315 The Verbatim Module Syntax in TSConfig | 03:22 |
316 | 316 Verbatim Module Syntax Enforces Import Type | 02:52 |
317 | 317 Understanding lib and target in TypeScript Configuration | 03:46 |
318 | 318 Bundle Your Code for Library Use | 01:20 |
319 | 319 Emitting TypeScript Declaration Files | 01:05 |
320 | 320 Navigate to Source Files Instead of Declaration Files | 01:04 |
321 | 321 Utilizing Declaration Maps in TypeScript | 01:29 |
322 | 322 Configure JSX Support in TypeScript | 00:37 |
323 | 323 Update tsconfig.json to Support JSX | 02:31 |
324 | 324 Multiple TypeScript Configurations | 00:39 |
325 | 325 Managing Multiple tsconfig Files | 01:19 |
326 | 326 Globals are Tied to a Single Configuration | 00:51 |
327 | 327 Share Configuration Across Multiple tsconfig Files | 00:36 |
328 | 328 Extending a Base TypeScript Configuration | 01:45 |
329 | 329 TypeScript Project References | 02:38 |
330 | 330 Organizing TypeScript Configurations with Project References | 06:00 |
331 | 331 Designing Your Types | 01:14 |
332 | 332 Introduction to Generic Types | 01:35 |
333 | 333 Implement a Generic Type | 03:59 |
334 | 334 Generics with Multiple Type Parameters | 00:51 |
335 | 335 Add Multiple Type Arguments to a Generic Type | 01:52 |
336 | 336 Handle Errors with a Generic Result Type | 01:57 |
337 | 337 Default Type Parameters in Generics | 01:10 |
338 | 338 Specify a Default Type Parameter | 01:42 |
339 | 339 Add Constraints to a Type Parameter | 01:11 |
340 | 340 Constraining Type Parameters | 02:46 |
341 | 341 Build a Stricter Omit Type | 01:06 |
342 | 342 Constrain Keys in a StrictOmit Type Helper | 01:38 |
343 | 343 Template Literal Types in TypeScript | 01:03 |
344 | 344 Constrain Strings to Match a Pattern with Template Literal Types | 02:10 |
345 | 345 Represent All Possible Type Combinations with Template Literals | 00:38 |
346 | 346 Passing Unions to Template Literal Types | 01:59 |
347 | 347 Derive Types with Mapped Types | 01:04 |
348 | 348 Use a Mapped Type to Create a New Object Shape | 03:21 |
349 | 349 Remapping Keys in TypeScript Map Types | 01:13 |
350 | 350 Combine the | 02:28 |
351 | 351 Introduction to the Utilities Folder | 01:55 |
352 | 352 Generic Functions Without Inference | 01:07 |
353 | 353 Make a Function Generic | 01:54 |
354 | 354 Default Parameter Types for Generic Functions | 00:51 |
355 | 355 Setting a Default Parameter Type for Generic Functions | 00:40 |
356 | 356 Inference in Generic Functions | 01:09 |
357 | 357 Enable Generic Functions to Better Infer Types | 03:39 |
358 | 358 Type Parameter Constraints with Generic Functions | 01:22 |
359 | 359 Add Constraints to Generic Functions with the extends Keyword | 02:45 |
360 | 360 Combining Generic Types with Generic Functions | 01:16 |
361 | 361 Refactoring for Generic Types and Functions | 02:15 |
362 | 362 Multiple Type Arguments in Generic Functions | 01:08 |
363 | 363 Type Inference in Function Parameters | 04:16 |
364 | 364 Using Type Predicates to Create Reusable Type Guards | 01:26 |
365 | 365 Annotate a Return Type with a Type Predicate | 03:23 |
366 | 366 Understanding Assertion Functions in TypeScript | 01:35 |
367 | 367 Asserting Types with Type Predicates | 02:09 |
368 | 368 Adapt a Function to Handle Different Types of Arguments | 01:22 |
369 | 369 Implement Function Overloads | 04:29 |
Similar courses to TypeScript Pro Essentials
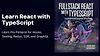
Fullstack React with Typescriptfullstack.io
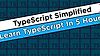
TypeScript Simplifiedwebdevsimplified.com
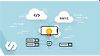
Typescript: The Complete Developer's GuideudemyStephen Grider
![React & TypeScript Chrome Extension Development [2021]](https://cdn.courseflix.net/courses/100x56/react-typescript-chrome-extension-development-2021.jpg?d=1743595988647)
React & TypeScript Chrome Extension Development [2021]udemy
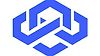
Loopback 4: Modern ways to Build APIs in Typescript & NodeJsudemy
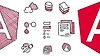
Angular Architecture. How to Build Scalable Web Applicationsudemy
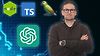