Professional TypeScript Training by Matt Pocock | Total TypeScript
Learn how to use TypeScript to level-up your applications as a web developer through exercise driven self-paced workshops and tutorials hosted by TypeScript wizard Matt Pocock.
Read more about the course
Your time is precious. You have bigger fish to fry.
You could spend that time building new features or bugfixes. You could be providing value. Instead, you're fighting TypeScript.
These days, TypeScript is an industry default. If you’re starting an enterprise app today, you’ll need a good reason NOT to use TypeScript.
So you keep on pushing - all the time feeling like you’re working against TypeScript, not with it.
The truth is that you never truly learned TypeScript, at least not in depth. That's the source of your pain.
You never developed a systematic approach to solving type errors.
You never developed a mental model for understanding when to use any.
You never understood the power of generics.
Ultimately…
You never learned the underlying principles and patterns of being an effective TypeScript engineer.
An exercise-driven approach for learning TypeScript
Total TypeScript's approach is different than what you'll find in other online courses.
Instead of sitting through hours of lectures, you'll be presented with problematic code and a concise explanation of what needs to be done.
Then using your existing skills along helpful links to relevant docs and other resources, you'll have as much time as you need to complete the challenge either in your local editor or using the editor embedded into each lesson.
When you're ready, you can watch how a true TypeScript Wizard approaches solving the challenge.
The exercises build upon another, demonstrating the most important TypeScript concepts in a variety different contexts so you can build understanding of when, where, and how to best apply them in your own application and library code.
Make TypeScript work for you, not against you
What’s the thing that separates TypeScript wizards from the rest?
Understanding.
To them, TypeScript doesn’t feel like magic. It feels simple, predictable and malleable.
They can bend it to their will, and use its power for amazing DX.
Total TypeScript Core Volume is all about building that understanding at a deep level.
When you’ve completed the exercises, you’ll reach a point of mastery where very little surprises you any more.
You’ll have less fear when approaching nasty TypeScript errors, more confidence when typing dynamic signatures, gain velocity by cutting lines of code, and so much more.
You deserve so much better
You deserve a practical, systematic approach to learning TypeScript in depth.
Total TypeScript shows you the techniques used by the most complex OSS libraries.
Become your company’s resident TypeScript Wizard.
- You will become a better contributor and reviewer.
- You will be the one to unblock others and raise their velocity.
- You will be the one who knows instinctively what TypeScript is doing.
You are indispensable.
Who is Total TypeScript for?
Total TypeScript Core Volume is suitable for any developer who is ready to work at becoming a wizard, though some basic familiarity with TypeScript is recommended.
The Type Transformations workshop begins by warming you up with exercises on basic inference and essential types.
Then nearly 150 exercises later, the Advanced TypeScript Patterns workshop will have you creating chainable method abstractions with generics and the builder pattern.
Check out the free Beginner's Tutorial to set a foundation and get an idea for the workshop's teaching approach.
Total TypeScript Core Volume
The Total TypeScript Core Volume contains three professional-grade workshops for you to achieve TypeScript Wizardry:
- Type Transformations (7 sections, 50+ exercises)
- TypeScript Generics (6 sections, 40+ exercises)
- Advanced TypeScript Patterns (7 sections, 40+ exercises)
Each workshop contains dozens of exercises designed to put your problem solving skills to the test.
Professional TypeScript Workshops
Type Transformations
The Type Transformations workshop is your guide to TypeScript features and patterns that allow you to manipulate types to produce exactly what you want. As you begin to see how the pieces fit together, you’ll find yourself writing less code that is easier to maintain.
There are over 50 exercises in this workshop, each with a challenge designed to help you learn to wield the power of transforming strings into objects, objects into unions, and everything in between.
TypeScript Generics
The Generics workshop is your guide to mastering one of TypeScript's most complex features.
Through dozens of exercises, you'll get to grips with generics from the lowest level, up through examples of how your favorite TS libraries use them.
Advanced TypeScript Patterns
The Advanced Patterns workshop is a primer on several patterns that emerge from TypeScript's primitives. We'll build on the generics and type transformations work we've done so far - extracting methods for architecting them into novel solutions.
Most of these patterns aren't documented by TypeScript - they've been discovered and iterated on by the TypeScript community. Each section focuses on a different pattern via interactive exercises.
This workshop is not appropriate for beginners.
Watch Online Professional TypeScript Training by Matt Pocock | Total TypeScript
# | Title | Duration |
---|---|---|
1 | Type Transformations Workshop Welcome: Explainer | 01:09 |
2 | Get the Return Type of a Function | 00:40 |
3 | Use a Utility Type to Extract a Function’s Return Type | 01:31 |
4 | Typeof Keyword, and Type Level | 02:02 |
5 | Extract Function Parameters Into A Type | 00:25 |
6 | Use a Utility Type to Extract Function Parameters | 00:46 |
7 | Extract The Awaited Result of a Promise | 00:38 |
8 | Use Utility Types To Extract a Promise's Result | 00:59 |
9 | Create a Union Type From an Object’s Keys | 00:34 |
10 | Create Unions from Objects Using Two Operators | 01:31 |
11 | Understand The Terminology Around Unions | 00:23 |
12 | Union Terminology Examples | 01:41 |
13 | Extracting Members of a Discriminated Union | 00:46 |
14 | Extract From A Union Using a Utility Type | 01:42 |
15 | Excluding Parts of a Discriminated Union | 00:20 |
16 | Use a Utility Type to Remove a Single Member of a Union | 00:32 |
17 | The Power of Union Types in TypeScript: Explainer | 02:47 |
18 | Extract Object Properties into Individual Types | 00:40 |
19 | Use Indexed Access Types to Extract Object Properties | 01:06 |
20 | Extract the Discriminator from a Discriminated Union | 00:25 |
21 | Simple Syntax Used to Access Parts of a Discriminated Union | 00:57 |
22 | Resolve an Object’s Values as Literal Types | 01:02 |
23 | The Annotation Used to Infer an Object's Values as Literal Types | 02:23 |
24 | Create a Union From an Object's Values | 00:53 |
25 | Extract Specific Members From A Union with Indexed Access | 01:12 |
26 | Get All of an Object’s Values | 00:27 |
27 | Use Two Operators With Indexed Access to Get All of an Object's Values | 00:56 |
28 | Create Unions out of Array Values | 00:29 |
29 | Methods Used to Create Unions out of Array Values | 01:24 |
30 | Only Allow Specified String Patterns | 00:43 |
31 | Template Literal with Strings | 01:09 |
32 | Extract Union Strings Matching a Pattern | 01:01 |
33 | Extracting String Pattern Matches with Template Literals | 01:02 |
34 | Create a Union of Strings with All Possible Permutations of Two Unions | 00:36 |
35 | Passing Unions Into Template Literals | 01:08 |
36 | Splitting A String into a Tuple | 00:45 |
37 | Using S From ts-toolbelt to Split a String Into a Tuple | 01:01 |
38 | Create an Object Whose Keys Are Derived From a Union | 00:35 |
39 | Use a Utility Type to Create An Object From A Union | 00:58 |
40 | Transform String Literals To Uppercase | 00:33 |
41 | Manipulate String Literals Using Type Helpers | 01:12 |
42 | Template Literals in Mattermost: Explainer | 01:55 |
43 | Introducing Type Helpers | 00:42 |
44 | Create Functions that Return Types | 02:46 |
45 | Creating a Maybe Type Helper | 00:23 |
46 | The Unconstrained Maybe Type Helper | 01:17 |
47 | Ensure Type Safety in a Type Helper | 01:06 |
48 | Use Constraints to Limit Type Parameters | 03:01 |
49 | Create a Reusable Type Helper | 00:27 |
50 | Add Support for Multiple Types in a Type Helper | 00:36 |
51 | Optional Type Parameters in Type Helpers | 00:43 |
52 | Set a Default Type Value in a Type Helper | 01:53 |
53 | Functions as Constraints for Type Helpers | 00:59 |
54 | Support Function Type Constraints with Variable Arguments | 02:42 |
55 | Constraining Types for Anything but null or undefined | 00:43 |
56 | Exclude null and undefined from the Maybe Type | 03:35 |
57 | Constraining Type Helpers to Non-Empty Arrays | 00:33 |
58 | Enforce a Minimum Array Length in a Type Helper | 01:58 |
59 | Explainer: Type Helpers in Redux | 04:59 |
60 | Add Conditional Logic to a Type Helper | 00:39 |
61 | Compare and Return Values with Extends and the Ternary Operator | 02:27 |
62 | Refine Conditional Logic in a Type Helper | 01:00 |
63 | Prevent Unwanted Type Scenarios from Happening | 02:22 |
64 | How TypeScript Added Conditional Types: Explainer | 01:45 |
65 | Introducing infer for Conditional Logic | 01:09 |
66 | Infer Elements Inside a Conditional with Infer | 02:43 |
67 | Extract Type Arguments to Another Type Helper | 01:00 |
68 | Use infer with Generics to Extract Types from Arguments | 02:36 |
69 | Extract Parts of a String with a Template Literal | 00:31 |
70 | Pattern Matching on Template Literals with Infer | 01:27 |
71 | Template Literal Types Were Nearly Regexes: Explainer | 02:51 |
72 | Extract the Result of an Async Function | 00:52 |
73 | Optionally Infer the Return Type of a Function | 01:23 |
74 | Extract the Result From Several Possible Function Shapes | 00:52 |
75 | Two Methods for Extracting the Result of Multiple Possible Functions | 01:17 |
76 | Distributivity in Conditional Types | 00:56 |
77 | Using Generic Context to Avoid Distributive Conditional Types | 03:03 |
78 | Map Over a Union to Create an Object | 00:40 |
79 | Use Mapped Types to Create an Object from a Union | 02:03 |
80 | Mapped Types with Objects | 00:36 |
81 | Map Over the Keys of an Object | 02:25 |
82 | Transforming Object Keys in Mapped Types | 00:54 |
83 | Remapping Object Keys in a Mapped Type | 02:10 |
84 | How Excalidraw uses Mapped Types to Save Lines of Code: Explainer | 01:45 |
85 | Conditionally Extract Properties from Object | 00:53 |
86 | Selective Remapping with Conditional Types and Template Literals | 03:15 |
87 | Map a Discriminated Union to an Object | 00:49 |
88 | Two Techniques for Mapping a Discriminated Union to an Object | 03:29 |
89 | Map an Object to a Union of Tuples | 00:52 |
90 | Create a Union of Tuples by Reindexing a Mapped Type | 02:05 |
91 | Transform an Object into a Union of Template Literals | 00:27 |
92 | Map an Object to a Union of Template Literals | 01:57 |
93 | Transform a Discriminated Union into a Union | 00:33 |
94 | Iteratively Map and Remap to Transform Types | 02:15 |
95 | Transform Path Parameters from Strings to Objects | 01:01 |
96 | Extract from String with Mapped Types, Template Literals, and infer. | 03:32 |
97 | Transform an Object into a Discriminated Union | 00:36 |
98 | Create a Discriminated Union through Intermediary Transformations | 02:40 |
99 | Transform a Discriminated Union with Unique Values to an Object | 01:06 |
100 | Create an Object using Mapped Types, Conditional Types, and TypeScript Keywords | 02:14 |
101 | Construct a Deep Partial of an Object | 01:02 |
102 | Use Recursion and Mapped Types to Create a Type Helper | 05:17 |
103 | TypeScript Generics Workshop Welcome | 01:17 |
104 | Typing Functions with Generics | 00:46 |
105 | Replace the unknown Type with a Generic | 01:54 |
106 | Restricting Generic Argument Types | 00:40 |
107 | Add Constraints to a Generic | 00:56 |
108 | Typing Independent Parameters | 00:31 |
109 | Use Multiple Generics with a Function | 01:20 |
110 | Approaches for Typing Object Parameters | 00:28 |
111 | Approaches for Typing Object Parameters: Solution | 01:51 |
112 | Generic Functions in Excalidraw | 02:20 |
113 | Generics in Classes | 00:47 |
114 | Add Types to a Class | 01:11 |
115 | Generic Mapper Function | 01:04 |
116 | Add Object Property Constraints to a Generic | 02:15 |
117 | The Importance of Generics in TypeScript | 01:29 |
118 | Add Type Parameters to a Function | 00:45 |
119 | Pass Type Arguments to a Function | 02:51 |
120 | Defaults in Type Parameters | 00:33 |
121 | Specify a Default Value | 00:45 |
122 | Infer Types from Type Arguments | 01:08 |
123 | Infer from the Type Arguments of a Class | 02:12 |
124 | Strongly Type a Reduce Function | 01:12 |
125 | Pass Type Arguments to a Reduce Function | 03:06 |
126 | Avoid any Types with Generics | 00:36 |
127 | Use Generics to Type a Fetch Request | 03:47 |
128 | Passing Type Arguments in cal.com | 02:03 |
129 | Improving Code Maintainability | 03:38 |
130 | Generics at Different Levels | 01:26 |
131 | Represent Generics at the Lowest Level | 03:04 |
132 | Typed Object Keys | 00:47 |
133 | Two Approaches for Typing Object Keys | 03:45 |
134 | Make a Generic Wrapper for a Function | 01:51 |
135 | Constrain a Type Argument to a Function | 04:49 |
136 | Understand Literal Inference in Generics | 03:54 |
137 | Understand Generic Inference When Using Objects as Arguments | 03:33 |
138 | Inferring Literal Types from any Basic Type | 00:55 |
139 | Accepting Multiple Literal Types | 00:57 |
140 | Infer the Type of an Array Member | 00:57 |
141 | Constrain to the Array Member, Not the Array | 02:14 |
142 | Generics in a Class Names Creator | 01:33 |
143 | Two Approaches to Working with Class Names | 01:44 |
144 | Generics in React Query | 04:50 |
145 | Generics with Conditional Types | 01:00 |
146 | Ensure Runtime Level & Type Level Safety with Conditional Types | 03:25 |
147 | Fixing Errors in Generic Functions | 01:22 |
148 | Fixing the "Not Assignable" Error | 02:30 |
149 | Generic Function Currying | 01:08 |
150 | Fix Type Inference in Curried Functions | 03:21 |
151 | Generic Interfaces with Functions | 02:28 |
152 | Understanding Generics at Different Levels of Functions | 04:32 |
153 | Spotting Useless Generics | 00:58 |
154 | Refactoring Functions with Unnecessary Type Arguments | 01:32 |
155 | Spotting Missing Generics | 01:32 |
156 | Improving Type Inference with Additional Generics | 02:53 |
157 | How tRPC Handles Inheritable Generics | 03:33 |
158 | Refactoring Generics for a Cleaner API | 01:37 |
159 | Create a Factory Function to Apply Type Arguments to All Child Functions | 02:11 |
160 | The Partial Inference Problem | 01:53 |
161 | A Workaround for The Lack of Partial Inference | 03:57 |
162 | What is a Function Overload? | 00:42 |
163 | Understanding Function Overloads | 01:08 |
164 | Function Overloads vs. Conditional Types | 00:32 |
165 | Match Return Types with Function Overloads | 03:29 |
166 | Debugging Overloaded Functions | 01:18 |
167 | Specifying Types for an Overloaded Function | 03:53 |
168 | Function Overloads vs. Union Types | 00:46 |
169 | When to Use Overloads and Unions | 02:03 |
170 | Generics in Function Overloads | 00:43 |
171 | Typing Different Function Use Cases | 02:50 |
172 | Solving an Inference Mystery | 01:00 |
173 | The Inference Mystery Solved | 06:10 |
174 | Use Function Overloads to Infer Initial Data | 01:17 |
175 | Split Functions Into Two Different Call Signatures | 02:11 |
176 | The "Instantiated with Subtype" Error | 01:48 |
177 | Handling Default Arguments with Function Overloads | 03:52 |
178 | Make An Infinite Scroll Function Generic with Correct Type Inference | 01:17 |
179 | Introduce a Type Parameter to Ensure Type Consistency | 05:03 |
180 | Create a Function with a Dynamic Number of Arguments | 01:34 |
181 | Use a Tuple to Represent a Dynamic Number of Arguments | 03:44 |
182 | Create a Pick Function | 01:10 |
183 | Extracting Object Properties with Reduce and Generics | 07:54 |
184 | Create a Form Validation Library | 02:02 |
185 | Add Strong Typing and Proper Error Handling to a Form Validator | 06:34 |
186 | Improve a Fetch Function to Handle Missing Type Arguments | 01:20 |
187 | Modify a Generic Type Default for Improved Error Messages | 01:55 |
188 | Typing a Function Composition with Overloads and Generics | 02:22 |
189 | Using Overloads and Generics to Type Function Composition | 05:53 |
190 | Build an Internationalization Library | 02:32 |
191 | Extract Types from Strings for an Internationalization Library | 07:46 |
192 | Advanced Workshop Welcome | 01:14 |
193 | What is a Branded Type? | 03:23 |
194 | Form Validation with Branded Types | 01:39 |
195 | Assigning Branded Types to Values | 01:38 |
196 | Using Branded Types as Entity Id’s | 00:43 |
197 | Add Branded Types to Functions and Models | 02:54 |
198 | Creating Reusable Validity Checks with Branded Types and Type Helpers | 01:10 |
199 | Combine Type Helpers with Branded Types | 01:52 |
200 | Creating Validation Boundaries with Branded Types | 01:54 |
201 | Using Branded Types to Validate Code Logic | 03:45 |
202 | Using Index Signatures with Branded Types | 01:14 |
203 | Indexing an Object with Branded Types | 02:20 |
204 | TypeScript's Global Scope | 01:02 |
205 | Add a Function to the Global Scope | 04:37 |
206 | Add Functionality to Existing Global Interfaces | 01:03 |
207 | Use Declaration Merging to Add Functionality to the Global Window | 04:43 |
208 | Add Types to Properties of Global Namespaced Interfaces | 01:03 |
209 | Typing process.env in the NodeJS Namespace | 03:26 |
210 | Colocating Types for Global Interfaces | 02:00 |
211 | Solving the Colocation Problem with Globals | 02:56 |
212 | Filtering with Type Predicates | 00:49 |
213 | Use a Type Predicate to Filter Types | 02:56 |
214 | Checking Types with Assertion Functions | 01:13 |
215 | Ensure Valid Types with an Assertion Function | 02:46 |
216 | Avoiding TypeScript's Most Confusing Error | 00:48 |
217 | Declare Assertion Functions Properly to Avoid Confusing Errors | 00:37 |
218 | Combining Type Predicates with Generics | 01:45 |
219 | Filtering with Type Predicates and Generics | 02:35 |
220 | Combining Brands and Type Predicates | 00:50 |
221 | Checking for Validity with Brands and Type Predicates | 00:44 |
222 | Combining Brands with Assertion Functions | 00:24 |
223 | Validate Types with Brands and Assertions | 01:05 |
224 | Classes as Types and Values | 01:02 |
225 | Using Classes in TypeScript | 00:54 |
226 | Dive into Classes with Type Predicates | 02:13 |
227 | Simplifying TypeScript with Type Predicates | 02:41 |
228 | Assertion Functions and Classes | 01:25 |
229 | Leverage Assertion Functions for Better Inference in Classes | 01:27 |
230 | Class Implementation Following the Builder Pattern | 07:44 |
231 | TRPC's Creator on the Builder Pattern | 04:08 |
232 | Create a Type Safe Map with the Builder Pattern | 01:09 |
233 | Getters and Setters in the Builder Pattern | 02:19 |
234 | Debugging the Builder Pattern | 00:41 |
235 | Default Generics in the Builder Pattern | 02:41 |
236 | Building Chainable Middleware with the Builder Pattern | 03:40 |
237 | The Power of Generics and the Builder Pattern | 06:37 |
238 | Subclassing in Zod | 03:18 |
239 | Where Do External Types Come From? | 05:24 |
240 | Extract Types to Extend an External Library | 00:59 |
241 | Retrieve Function Parameters from an External Library | 02:00 |
242 | Navigating Lodash's Type Definitions | 03:26 |
243 | Finding Proper Type Arguments and Generics with Lodash | 01:21 |
244 | Passing Type Arguments with Lodash | 05:15 |
245 | Navigating Express's Type Definitions | 09:06 |
246 | Add Query Params to an Express Request | 01:18 |
247 | Make an Express Request Function Generic | 03:44 |
248 | Browsing Zod's Types | 02:45 |
249 | Create a Runtime and Type Safe Function with Generics and Zod | 01:39 |
250 | Infer Runtime Arguments from a Zod Schema | 04:38 |
251 | Override External Library Types | 01:38 |
252 | Create a Declarations File to Override Types | 03:50 |
253 | Identity Functions as an Alternative to the `as const` | 01:31 |
254 | Using const type parameters For Better Inference | 02:05 |
255 | Add Constraints to an Identity Function | 00:47 |
256 | Constraining and Narrowing an Identity Function | 02:51 |
257 | Specifying Where Inference Should Not Happen | 01:38 |
258 | Fix Inference Issues with F.NoInfer | 02:17 |
259 | Find the Generic Flow of an Identity Function | 01:10 |
260 | Avoid Duplicate Code in an Identity Function with Generics | 02:39 |
261 | Reverse Mapped Types | 01:19 |
262 | Inference Inception in an Identity Function | 02:17 |
263 | Merge Dynamic Objects with Global Objects | 00:59 |
264 | Add Objects to the Global Scope Dynamically | 02:19 |
265 | Narrowing with an Array | 01:19 |
266 | Narrowing with Arrays and Generics | 08:30 |
267 | Create a Type-Safe Request Handler with Zod and Express | 01:59 |
268 | Type-Safe Request Handlers with Zod and Express | 07:45 |
269 | Building a Dynamic Reducer | 03:55 |
270 | Dynamic Reducer with Generic Types | 11:26 |
271 | Custom JSX Elements | 01:06 |
272 | Adding Custom Elements to JSX.IntrinsicElements | 04:01 |
273 | Tejas Kumar Discusses How to Build Bulletproof Apps with TypeScript | 52:27 |
274 | Adopting TypeScript at Netflix with Shaundai Person | 43:02 |
275 | Mark Erikson on Avoiding Breaking Changes and Improving Maintainability with TypeScript | 54:35 |
276 | The TypeScript Culture at Formidable with Kadi Kraman | 42:01 |
277 | Colin McDonnell Talks About The Design Choices Behind Zod | 01:01:20 |
278 | TypeScript's History and Growth with Daniel Rosenwasser | 56:41 |
279 | Priscila Oliveira on Sentry's TypeScript Migration | 43:52 |
280 | Gabriel Vergnaud on Type and Value Level Mapping in TypeScript | 48:45 |
281 | Building Familiarity with TypeScript's Syntax and Functionality with Orta Therox | 49:57 |
282 | A Look at tRPC with its Creator Alex "KATT" Johansson | 40:46 |
283 | Exploring Generics in React Query with Tanner Linsley | 52:39 |
284 | Type-Checking React Props With Discriminated Unions | 01:18 |
285 | Using Discriminated Unions to Create Flexible Component Props in React | 01:45 |
286 | Destructuring Discriminated Unions in React Props | 00:48 |
287 | Destructuring vs Accessing Discriminated Union Props | 02:32 |
288 | Adding a Prop Required Across Discriminated Union Variants | 05:15 |
289 | Resolving Discriminated Union Types with an Intersection | 03:37 |
290 | Differentiating Props With a Boolean Discriminator | 02:24 |
291 | Discriminated Unions for Conditional Props in TypeScript | 00:49 |
292 | Using the Record Type to Represent an Empty Object | 02:28 |
293 | Conditionally Require Props With Discriminated Unions | 01:19 |
294 | Allow Optional Props Using A Discriminated Union Branch With Undefined Types | 02:16 |
295 | Finding a Better Type Definition For A Mapped Component | 02:17 |
296 | What's The Difference Between React.ReactNode and React.FC? | 01:23 |
297 | Syncing Types without Manual Updates | 02:38 |
298 | The `keyof typeof` Pattern | 01:05 |
299 | The Partial Autocompletion Quirk | 04:17 |
300 | Solving Partial Autocompletion | 01:21 |
301 | Extracting Keys and Values from a Type | 01:43 |
302 | Using `as const` and Indexed Access Types to Extract Keys and Values from a Type | 02:19 |
303 | Ensuring Correct Inference for Prop Types | 01:25 |
304 | Comparing `as const`, `as`, and `satisfies` | 01:18 |
305 | Inference from a Single Source of Truth | 00:54 |
306 | Understanding and Implementing Dynamic Props Mapping in React | 03:29 |
307 | DRY out Code with Generic Type Helpers | 01:17 |
308 | Implement a Generic Type Helper | 01:27 |
309 | Refactoring to a Type Helper | 03:36 |
310 | Creating an "All or Nothing" Type Helper for React props | 04:30 |
311 | Constraining a Type Helper to Accept Specific Values | 01:04 |
312 | Add Generic Constraints to Type Helpers | 03:30 |
313 | Adding Type Arguments to a Hook | 01:50 |
314 | Adding Type Arguments to a Function | 02:56 |
315 | Wrapping a Generic Function Inside of Another | 01:19 |
316 | Type Inference with Generic Functions in TypeScript | 04:18 |
317 | Applying Generics to Components | 01:46 |
318 | Add a Generic Type Argument to a Props Interface | 00:50 |
319 | Generics in Class Components | 01:18 |
320 | Converting a Class Component to be Generic | 01:49 |
321 | Passing Type Arguments To Components | 02:18 |
322 | Use the Angle Brackets Syntax to Pass a type to a Component | 01:17 |
323 | Generic Inference through Multiple Type Helpers | 03:55 |
324 | Adding Generic Type Arguments to Type Helpers | 04:49 |
325 | Build a useMutation hook | 02:36 |
326 | Refactoring a Generic Hook for Best Inference | 01:59 |
327 | Generics vs Discriminated Unions | 02:12 |
328 | Refactoring from Generics to a Discriminated Union | 00:40 |
329 | Fixing Type Inference in a Custom React Hook | 02:12 |
330 | Use 'as const' to Infer a Tuple return type | 08:06 |
331 | Strongly Typing React Context | 01:56 |
332 | Using Type Arguments to Create A Strongly Typed Context | 01:54 |
333 | Using TypeScript to Manage Complex State | 04:18 |
334 | Handling Complex State Management with TypeScript Unions | 01:21 |
335 | Using Discriminated Unions in useState | 01:17 |
336 | Handling Different State Values with Discriminated Unions | 01:59 |
337 | Discriminated Tuples in Custom Hooks | 01:23 |
338 | Improved Type Safety with Discriminated Tuples in TypeScript | 02:17 |
339 | Use Function Overloads for Better Type Inference | 01:14 |
340 | Overloading Functions in TypeScript | 05:32 |
341 | Mimicking useState Behavior with Function Overloads | 00:59 |
342 | Wrapping useState Functionality with Function Overloads | 03:47 |
343 | Currying Hooks | 01:29 |
344 | Inferring Type Arguments in Curried Hooks | 03:41 |
345 | Exploring the React Namespace | 01:23 |
346 | Understanding the React Namespace | 03:46 |
347 | JSX.Element, React.ReactElement, and React.ReactNode | 01:31 |
348 | Understanding React's JSX Types | 06:06 |
349 | Strongly Typing Children in React | 01:45 |
350 | Strongly Typing Children in React Doesn't Work | 03:08 |
351 | Exploring JSX.IntrinsicElements | 01:04 |
352 | Understand the Structure of React's JSX.IntrinsicElements | 02:55 |
353 | Understanding React's ElementType and ComponentType | 02:35 |
354 | Appending to React's Global Namespace | 00:31 |
355 | Declaration Merging and the Global Namespace | 01:04 |
356 | Modify Existing Interfaces in the Global React Namespace | 00:53 |
357 | Extend the Global React namespace with Declaration Merging in TypeScript | 00:53 |
358 | Add a New Global Element in TypeScript | 00:45 |
359 | Extend JSX.IntrinsicElements with Declaration Merging | 02:18 |
360 | Exploring HTML Attribute and Element Types | 01:17 |
361 | Navigating HTMLAttribute types | 04:40 |
362 | Add Attributes to All Elements with Declaration Merging | 00:37 |
363 | Updating the Global Namespace for an Additional Attribute | 02:28 |
364 | Strongly Typed Lazy Loading | 01:30 |
365 | Strongly Typing Lazy Loaded Components with Generics | 05:07 |
366 | Render Props | 01:40 |
367 | Typing the Children Prop for Render Props | 02:38 |
368 | Records of Components with the Same Props | 01:37 |
369 | Infer Shared Props for Multiple Components | 03:34 |
370 | The Problem With forwardRef | 06:43 |
371 | Fixing forwardRef Locally | 01:03 |
372 | Override forwardRef's Behavior Locally | 01:58 |
373 | Typing Higher Order Components | 01:39 |
374 | Implementing a Generic Higher Order Component | 04:45 |
375 | Using Higher Order Components with Generic Components | 01:34 |
376 | Add Generic Component Support to a Higher Order Component | 04:51 |
377 | The `as` Prop in React | 02:29 |
378 | Approaching the `as` Prop with IIMTs and Generics | 07:06 |
379 | The `as` Prop with Custom Components | 01:27 |
380 | Type Helpers for React Components | 03:17 |
381 | The `as` Prop with Defaults | 01:31 |
382 | Two Approaches to Defaults for the `as` Prop | 05:25 |
383 | The `as` Prop with `forwardRef` | 01:45 |
384 | Distributive Omit with the `as` Prop | 07:38 |
385 | React Hook Form's Types | 02:50 |
386 | Understanding useForm Type Declarations in React Hook Form | 05:43 |
387 | Wrapping the useForm Hook from React Hook Form | 01:28 |
388 | Creating a Generic Wrapper for useForm | 04:55 |
389 | React-Select's Generics | 01:58 |
390 | Capture and Extend React Select's Type Definitions | 06:07 |
391 | Understanding React Query's Overloads | 03:05 |
392 | Targeting Overloads with useQuery | 08:01 |
393 | Wrapping useQuery | 01:26 |
394 | Handling Type Arguments in a Custom Query Hook | 05:43 |
Similar courses to Professional TypeScript Training by Matt Pocock | Total TypeScript
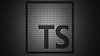
Design Patterns in TypeScriptudemy
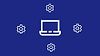
Microservices with NodeJS, React, Typescript and Kubernetesudemy
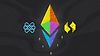
NFT Marketplace in React, Typescript & Solidity - Full Guideudemy
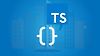
Understanding TypeScript - 2023 EditionudemyAcademind Pro
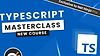
TypeScript MasterclassNet Ninja
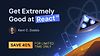
Learn React 19 with Epic React v2Kent C. Dodds
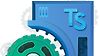
TypeScript with Vue.js 3vueschool.io
![React & TypeScript Chrome Extension Development [2021]](https://cdn.courseflix.net/courses/100x56/react-typescript-chrome-extension-development-2021.jpg?d=1753535125615)
React & TypeScript Chrome Extension Development [2021]udemy
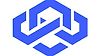