Design Patterns in TypeScript
Learn All of the 23 GoF (Gang of Four) Design Patterns and Implemented them in TypeScript. Design Patterns are descriptions or templates that can be repeatedly applied to commonly recurring problems during in software design. A familiarity of Design Patterns is very useful when planning, discussing, managing and documenting your applications from now and into the future.
More
Also, throughout the course, as each design pattern is discussed and demonstrated using example code, I introduce new TypeScript coding concepts along with each new design pattern. So that as you progress through the course and try out the examples, you will also get experience and familiarity with some of the finer details of programming with TypeScript.
In this course, you will learn about these 23 Design Patterns,
Creational
Factory
Abstract Factory
Builder
Prototype
Singleton
Structural
Decorator
Adapter
Facade
Bridge
Composite
Flyweight
Proxy
Behavioral
Command
Chain of Responsibility
Observer Pattern
Interpreter
Iterator
Mediator
Memento
State
Strategy
Template
Visitor
In the list of patterns above, there are Creational, Structural and Behavioral patterns.
Creational : Abstracts the instantiation process so that there is a logical separation between how objects are composed and finally represented.
Structural : Focuses more on how classes and objects are composed using the different structural techniques, and to form structures with more or altered flexibility.
Behavioral : Are concerned with the inner algorithms, process flow, the assignment of responsibilities and the intercommunication between objects.
Design patterns will give you a useful and common vocabulary for when designing, documenting, analyzing, restructuring new and existing software development projects from now and into the future.
Watch Online Design Patterns in TypeScript
# | Title | Duration |
---|---|---|
1 | Development Environment Setup | 05:21 |
2 | Course Code | 08:07 |
3 | Getting Started | 08:46 |
4 | Adding tsconfig.json | 08:47 |
5 | TSC Watch | 03:13 |
6 | Let/Const | 02:40 |
7 | Common Types | 02:10 |
8 | Strings | 01:55 |
9 | Boolean | 02:29 |
10 | Number | 02:08 |
11 | Array | 06:59 |
12 | Dictionary | 07:24 |
13 | Tuple | 02:31 |
14 | Set | 03:50 |
15 | Classes | 05:17 |
16 | Interfaces | 05:42 |
17 | Abstract Classes | 07:02 |
18 | Access Modifiers | 06:14 |
19 | Static Members | 06:22 |
20 | ES6 Imports/Exports | 05:38 |
21 | UML Diagrams | 05:15 |
22 | Factory Pattern | 05:25 |
23 | Factory Use Case | 05:04 |
24 | Abstract Factory Pattern | 03:52 |
25 | Abstract Factory Use Case | 03:09 |
26 | Builder Pattern | 03:03 |
27 | Builder Use Case | 04:20 |
28 | Prototype Pattern | 05:38 |
29 | Prototype Use Case | 06:31 |
30 | Singleton Pattern | 03:49 |
31 | Singleton Use Case | 03:27 |
32 | Decorator Pattern | 02:51 |
33 | Decorator Use Case | 05:07 |
34 | Adapter Pattern | 04:26 |
35 | Adapter Use Case | 06:10 |
36 | Facade Pattern | 02:14 |
37 | Facade Use Case | 03:57 |
38 | Bridge Pattern | 05:05 |
39 | Bridge Use Case | 02:01 |
40 | Composite Pattern | 06:05 |
41 | Composite Use Case | 02:57 |
42 | Flyweight Pattern | 05:23 |
43 | Flyweight Use Case | 04:03 |
44 | Proxy Pattern | 03:51 |
45 | Proxy Use Case | 05:49 |
46 | Command Pattern | 04:38 |
47 | Command Use Case | 04:16 |
48 | Chain of Responsibility Pattern | 04:11 |
49 | Chain of Responsibility Use Case | 04:01 |
50 | Observer Pattern | 04:55 |
51 | Observer Use Case | 04:53 |
52 | Interpreter Pattern | 10:12 |
53 | Interpreter Use Case | 05:00 |
54 | Iterator Pattern | 03:07 |
55 | Iterator Use Case | 03:12 |
56 | Mediator Pattern | 02:45 |
57 | Mediator Use Case | 03:34 |
58 | Memento Pattern | 04:19 |
59 | Memento Use Case | 03:43 |
60 | State Pattern | 03:02 |
61 | State Use Case | 02:57 |
62 | Strategy Pattern | 04:03 |
63 | Strategy Use Case | 02:37 |
64 | Template Method | 04:24 |
65 | Template Method Use Case | 03:53 |
66 | Visitor Pattern | 05:44 |
67 | Visitor Use Case | 02:59 |
68 | Summary | 01:31 |
Similar courses to Design Patterns in TypeScript
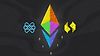
NFT Marketplace in React, Typescript & Solidity - Full Guideudemy
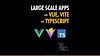
Course: Large Scale Apps with Vue, Vite and TypeScriptDamiano Fusco
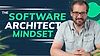
The Software Architect Mindset (COMPLETE)ArjanCodes
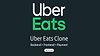
Uber Eats Clone Nomad Coders
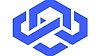
Loopback 4: Modern ways to Build APIs in Typescript & NodeJsudemy
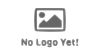
Mastering Next.js 13 with TypeScriptcodewithmosh (Mosh Hamedani)
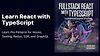
Fullstack React with Typescriptfullstack.io
![React & TypeScript Chrome Extension Development [2021]](https://cdn.courseflix.net/courses/100x56/react-typescript-chrome-extension-development-2021.jpg?d=1735914804811)
React & TypeScript Chrome Extension Development [2021]udemy
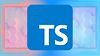
TypeScript Bootcamp: Zero to Masteryzerotomastery.io
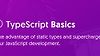