Fullstack React with Typescript
10h 16m 46s
English
Paid
May 18, 2023
Fullstack React with TypeScript is the complete guide to using TypeScript with React. Learn TypeScript patterns with React additional ecosystem advice (testing, redux, SSR) by building several apps including a Trello clone, a Medium-like website, testing with a digital-item e-comm app, and more!
Watch Online Fullstack React with Typescript
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Course introduction | 03:47 |
2 | How to get the most out of this course | 01:58 |
3 | What is TypeScript | 03:08 |
4 | Why use TypeScript with React | 03:22 |
5 | Introduction | 00:55 |
6 | What we are building and prerequisites | 02:14 |
7 | How to bootstrap React TypeScript app automatically | 15:10 |
8 | Clean up the code and define the global styles | 01:30 |
9 | How to style React elements | 02:11 |
10 | Prepare the styled components | 04:23 |
11 | Create column components | 04:43 |
12 | Create card components | 00:54 |
13 | Component for adding new items | 05:17 |
14 | The NewItemForm component | 03:23 |
15 | Auto focus on inputs | 03:41 |
16 | Submit on enter | 01:51 |
17 | Add global state and business logic | 04:45 |
18 | Implement the global state | 08:18 |
19 | Define the business logic | 11:37 |
20 | Moving the items | 03:38 |
21 | Add drag and drop (install React DnD) | 01:25 |
22 | Store the dragged item in the state | 01:42 |
23 | Define the useItemDrag hook | 01:44 |
24 | Drag the columns | 02:21 |
25 | Hide the dragged item | 03:04 |
26 | Implement the custom dragging preview | 08:41 |
27 | Drag the cards | 05:18 |
28 | Update the reducer | 02:32 |
29 | Drag the card to an empty column | 01:34 |
30 | Saving the state on the backend | 07:52 |
31 | Loading the data | 11:11 |
32 | How to test your applications: testing a digital goods store introduction | 00:35 |
33 | Get familiar with the application | 06:01 |
34 | Initial setup | 03:39 |
35 | Testing the App component | 03:06 |
36 | Mocking components | 02:33 |
37 | Jest helper to test navigation | 04:04 |
38 | Testing navigation | 02:28 |
39 | Shared components | 08:39 |
40 | The home page | 08:01 |
41 | The ProductCard Component | 05:03 |
42 | The Cart component | 08:57 |
43 | The CartItem component | 02:47 |
44 | The CheckoutList component | 01:36 |
45 | The CheckoutForm component | 06:43 |
46 | The FormField component | 03:24 |
47 | The order summary page | 04:04 |
48 | Testing the useProducts hook | 07:27 |
49 | Testing the useCart hook | 10:40 |
50 | Patterns in React TypeScript applications: making music with React introduction | 00:24 |
51 | What we're going to build | 00:54 |
52 | First steps and basic application layout | 06:18 |
53 | A bit of music theory | 09:03 |
54 | Third party API and browser API | 04:10 |
55 | The main app screen | 03:24 |
56 | Creating the keyboard | 08:27 |
57 | Adapter hook | 08:14 |
58 | Connecting to a keyboard | 04:33 |
59 | Mapping the real keys to virtual | 06:18 |
60 | The instruments list | 02:20 |
61 | The instrument selector | 04:06 |
62 | Loading the instruments | 01:23 |
63 | Render Props | 02:16 |
64 | Creating render props with functional components | 04:58 |
65 | Creating render props with classes | 10:05 |
66 | Higher-Order Components | 05:24 |
67 | Instrument adapter as a Higher-Order Component | 08:05 |
68 | Passing refs through | 02:37 |
69 | Static composition | 03:04 |
70 | Using the hooks with HOCs | 02:26 |
71 | Using Redux and TypeScript introduction | 00:49 |
72 | Using Redux and TypeScript preview the final result | 02:57 |
73 | What is Redux? | 05:45 |
74 | Using Redux and TypeScript initial setup | 03:01 |
75 | Redux logger | 01:37 |
76 | Prepare The Styles | 01:25 |
77 | Working with the canvas API | 04:59 |
78 | Handling the canvas events | 00:54 |
79 | Define the store types | 01:01 |
80 | Add actions | 02:02 |
81 | Dispatch actions | 01:51 |
82 | Draw the current stroke | 02:21 |
83 | Implement selecting colors | 03:55 |
84 | Implement undo and redo | 04:23 |
85 | Splitting the root reducer and using combineReducers | 06:58 |
86 | Exporting an image | 12:50 |
87 | Using Redux Toolkit | 06:49 |
88 | Using createAction | 03:02 |
89 | Using createReducer | 05:42 |
90 | Using slices | 04:21 |
91 | Add the modal windows slice | 07:20 |
92 | Add the modal manager component | 02:01 |
93 | Prepare the server | 03:27 |
94 | Save the project using thunks | 01:35 |
95 | Load the project | 08:05 |
96 | Static Site Generation and Server-Side Rendering using Next.js introduction | 08:37 |
97 | Generating pages on the backend using Next.js | 00:46 |
98 | Creating the first page | 02:57 |
99 | Basic application layout | 02:00 |
100 | Custom document component | 04:49 |
101 | Application theme | 02:47 |
102 | Custom App component | 02:29 |
103 | Front page | 02:02 |
104 | The 404 page | 05:25 |
105 | Post page template | 01:51 |
106 | Backend API server | 01:26 |
107 | Frontend API client | 05:49 |
108 | Updating the main page | 01:37 |
109 | Pre-Render the post page | 03:47 |
110 | The category page | 08:03 |
111 | Adding breadcrumbs | 07:15 |
112 | Comments and Server-Side Rendering | 02:00 |
113 | Components to render comments | 03:31 |
114 | API for adding comments | 05:47 |
115 | Adding comments to a page | 02:15 |
116 | Connecting Redux | 01:38 |
117 | Optimizing images | 11:29 |
118 | Building the project | 05:29 |
119 | Remaking the API | 01:31 |
120 | Creating client requests | 08:51 |
121 | Updating pages | 04:17 |
122 | Deploying with serverless functions | 02:16 |
123 | GraphQL, React, and TypeScript introduction | 02:54 |
124 | GraphQL, React, and TypeScript. What we are building | 01:51 |
125 | Authenticate in GitHub and preview the final result | 02:01 |
126 | Setting up the project | 01:40 |
127 | Running the application | 02:43 |
128 | Get the auth code | 02:35 |
129 | Auth flow link | 05:12 |
130 | Authentication context | 04:45 |
131 | GraphQL queries - getting the user data | 00:50 |
132 | Adding helper components | 02:37 |
133 | Defining the WelcomeWindow layout | 12:13 |
134 | Getting GitHub GraphQL schema | 01:18 |
135 | Generating the types | 01:16 |
136 | Adding routing | 01:19 |
137 | Implement navigation | 02:24 |
138 | Repositories main component | 05:29 |
139 | Getting the list of repositories | 03:12 |
140 | GraphQL mutations. Creating repositories | 04:45 |
141 | Getting the repository ID | 06:45 |
142 | Working with GitHub issues | 01:41 |
143 | Getting the list of issues | 03:26 |
144 | Creating an issue | 02:22 |
145 | Working with GitHub pull requests | 07:07 |
146 | Getting the list of pull requests | 01:47 |
147 | Creating a new pull request | 02:15 |
Read Book Fullstack React with Typescript
# | Title |
---|---|
1 | asdasdasdasdasdasd |
Similar courses to Fullstack React with Typescript
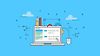
Node with React: Fullstack Web Development
Duration 25 hours 36 minutes 19 seconds
Course
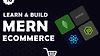
MERN Stack From Scratch
Duration 13 hours 32 minutes 38 seconds
Course
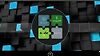
MERN Stack Front To Back: Full Stack React, Redux & Node.js.
Duration 11 hours 52 minutes 29 seconds
Course
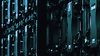
Data fetching with React Server Components
Duration 1 hour 15 minutes 56 seconds
Course
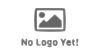
Instagram Clone Coding 3.0
Duration 20 hours 48 minutes 39 seconds
Course
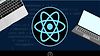
React Front To Back 2022
Duration 19 hours 57 minutes 45 seconds
Course
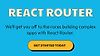
REACT ROUTER (v6)
Duration 3 hours 15 minutes 27 seconds
Course
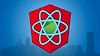
React, Angular, Node In-Depth Guide: Beginner to Pro
Duration 80 hours 1 minute 57 seconds
Course
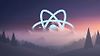
The Modern React 18 Bootcamp - A Complete Developer Guide
Duration 20 hours 35 minutes 57 seconds
Course