React Query: Server State Management in React
React Query has become the go-to solution for server state management in React apps, and for good reason! This smart, comprehensive solution makes it easy to keep your app up-to-date with data on the server. In fact, if you are using Redux simply to manage data from the server, React Query can replace Redux in your app. And, for server data management, React Query is much simpler and more powerful than Redux.
Read more about the course
For example, React Query:
tracks loading and error states for your server queries (no need to manage that yourself!)
makes cached server data available for display while you're fetching updated data
This course starts with a simple app to learn the basics of React Query:
queries
loading and error states
React Query dev tools
pagination and pre-fetching
mutations
Then we take a detour with the Star Wars API to learn about Infinite Queries (getting more data just as the user has gotten near the end of the current data).
Finally, we work on a large, multi-component app to learn about the above in more detail, plus
centralizing loading and error handling
filtering data
integrating React Query with auth
ways to keep data up to date after mutations, including optimistic updates
testing React Query
Other notable course features:
pre-written projects to use as a backdrop for React Query, so there's no time wasted writing code not relevant to the course
ample opportunities to practice with periodic "code quizzes" to make sure you're understanding the concepts
visual models for complicated concepts to help understand all of the moving pieces
the major project is written in TypeScript
supportive instructor who loves engaging with students in Q&A
Come see what the hype is about, and improve your apps with simpler, more powerful server state management!
Watch Online React Query: Server State Management in React
# | Title | Duration |
---|---|---|
1 | Introduction to React Query | 06:01 |
2 | Introduction to this Course | 03:03 |
3 | First project: Blog-em Ipsum | 05:39 |
4 | Creating Queries with useQuery | 07:47 |
5 | Handling Loading and Error States | 07:03 |
6 | React Query Dev Tools | 04:57 |
7 | staleTime vs cacheTime | 06:33 |
8 | Intro to Code Quizzes | 02:34 |
9 | Code Quiz! Create Query for Blog Comments | 07:03 |
10 | Query Keys | 06:23 |
11 | Pagination | 06:06 |
12 | Pre-fetching Data | 06:50 |
13 | isLoading vs isFetching | 04:55 |
14 | Intro to Mutations | 03:51 |
15 | Delete Post with useMutation | 06:49 |
16 | Code Quiz! Mutation to Update Post Title | 04:02 |
17 | Summary: React Query Basics | 03:01 |
18 | Introduction to Infinite Scroll | 04:32 |
19 | Code Quiz! Set up Infinite SWAPI for React Query | 05:27 |
20 | Intro to useInfiniteQuery | 06:20 |
21 | Infinite Scroll Diagram | 04:32 |
22 | Write useInfiniteQuery Call | 05:10 |
23 | InfiniteScroll Component | 06:18 |
24 | useInfiniteQuery Fetching and Error states | 04:08 |
25 | Code Quiz! Infinite Species | 07:09 |
26 | Summary: Infinite Scroll | 02:54 |
27 | Intro to Lazy Days Spa App | 06:22 |
28 | Lazy Days Spa App Code Orientation | 05:43 |
29 | Install and set up React Query | 07:01 |
30 | Custom Query Hook: useTreatments | 06:17 |
31 | Fallback Data | 02:13 |
32 | Centralized Fetching indicator with useIsFetching | 04:25 |
33 | onError Handler for useQuery | 06:45 |
34 | onError Default for Query Client | 06:40 |
35 | Code Quiz! Custom Hook for useStaff | 04:50 |
36 | Summary: Larger App Setup, Centralization, Custom Hooks | 03:00 |
37 | Adding Data to the Cache | 04:16 |
38 | Pre-Fetching Treatments (concepts) | 05:11 |
39 | Pre-Fetching Treatments (syntax) | 05:23 |
40 | Intro to useAppointments Custom Hook | 10:47 |
41 | useQuery for useAppointments | 03:53 |
42 | Query Keys as Dependency Arrays | 06:22 |
43 | Code Quiz! Pre-Fetch Appointments | 07:25 |
44 | Summary: Query Features I | 03:26 |
45 | Filtering Data with the useQuery select Option | 08:16 |
46 | Code Quiz! Selector for useStaff | 05:58 |
47 | Intro to Re-Fetch | 06:00 |
48 | Update Re-Fetch Options | 05:15 |
49 | Global Re-Fetch Options | 05:36 |
50 | Overriding Re-Fetch Defaults and Polling | 04:53 |
51 | Polling: Auto Re-Fetching at an Interval | 04:11 |
52 | Summary: Query Features II | 01:13 |
53 | Intro to React Query and Authentication | 08:31 |
54 | Intro to useAuth and useUser | 04:47 |
55 | Dependent Query in useUser | 07:13 |
56 | Code Quiz! Dependent Query in useUserAppointments | 09:13 |
57 | Adding User Data to Query Cache | 06:12 |
58 | Removing User Appointments Data on Signout | 04:55 |
59 | Summary: React Query and Authentication | 02:57 |
60 | Introduction to Mutations and Mutations Global Settings | 00:00 |
61 | Custom Mutation Hook: useReserveAppointments | 06:18 |
62 | OPTIONAL: TypeScript for `mutate` Function | 04:04 |
63 | Invalidating Query after Mutation | 05:29 |
64 | Query Key Prefixes | 05:44 |
65 | Code Quiz! Mutation to Cancel an Appointment | 07:03 |
66 | Update User and Query Cache with Mutation Response | 09:34 |
67 | Intro to Optimistic Updates in React Query | 06:42 |
68 | Making a Query "Cancel-able" | 07:28 |
69 | Writing Optimistic Update | 12:27 |
70 | Summary: Mutations | 01:25 |
71 | Intro to Testing React Query | 02:09 |
72 | Testing Setup, including Mock Service Worker | 04:33 |
73 | Query Client and Provider in Tests | 09:05 |
74 | Testing Rendered Query Data | 03:21 |
75 | Code Quiz! Test Rendered Staff Data | 03:43 |
76 | Testing Query Errors | 11:14 |
77 | Code Quiz! Staff Query Errors | 04:21 |
78 | Testing Mutations | 08:28 |
79 | Code Quiz! Test Cancel Appointment Mutation | 04:24 |
80 | Intro to Testing Custom Hooks | 03:36 |
81 | Test Appointments Filter | 08:08 |
82 | Code Quiz! Test Staff Filter | 03:24 |
83 | Summary: Testing React Query | 02:53 |
Similar courses to React Query: Server State Management in React
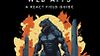
Building Large Scale Web Apps | A React Field GuideAddy OsmaniHassan Djirdeh
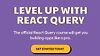
React Queryui.dev (ex. Tyler McGinnis)
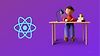
Advanced React For Enterprise: React for senior engineersudemy
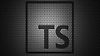
Design Patterns in TypeScriptudemy
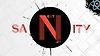
Next.JS with Sanity CMS - Serverless Blog App (w/ Vercel)udemy
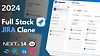
Build a Jira cloneCode With Antonio
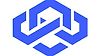
Loopback 4: Modern ways to Build APIs in Typescript & NodeJsudemy
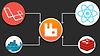
React and Laravel: Breaking a Monolith to Microservicesudemy
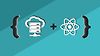
Server side rendering with Next + Reactudemy
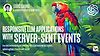