TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL
Learn advanced React, Node, TypeScript, and GraphQL by creating a ready-to-use application in this online course. Explore each of these technologies and find out how to create a production application that combines them.
Read more about the course
10 things you will learn:
How to create GraphQL API in Node.js using Apollo Server, from scratch.
Get confidence in building robust, off-the-shelf applications.
Build massive React apps using 2020 methods (like hooks).
Use the React Apollo and Apollo CLI to process React's GraphQL queries.
Avoid mistakes by using TypeScript to write strongly typed code.
Build robust code with advanced development tools.
Process payments with Stripe
Learn how to easily deploy apps with Heroku
Solve authentication complexity with industry standard OAuth 2.0 (Google Sign-In)
Enable location searches with the powerful Google Geocode API
Watch Online TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL
# | Title | Duration |
---|---|---|
1 | TinyHouse Welcome | 02:40 |
2 | Syllabus | 18:37 |
3 | How To Go Through The Course | 10:47 |
4 | Environment Setup | 02:54 |
5 | Part One Welcome | 03:09 |
6 | Module 1 Introduction | 01:04 |
7 | What is Node? | 05:06 |
8 | Running JavaScript with Node | 02:59 |
9 | Creating a minimal Node:Express Server | 08:08 |
10 | Automatic Reloading Using Nodemon | 03:50 |
11 | Introducing TypeScript | 05:38 |
12 | Adding TypeScript to our Server | 16:11 |
13 | Compiling our TypeScript project | 03:06 |
14 | Linting with ESLint | 13:18 |
15 | Introducing mock listings | 07:04 |
16 | Creating GET and POST Express routes | 10:46 |
17 | Module 1 Summary | 04:04 |
18 | Module 2 Introduction | 00:43 |
19 | What is GraphQL? | 05:41 |
20 | Comparing Github's REST and GraphQL APIs | 09:48 |
21 | GraphQL Concepts | 11:23 |
22 | Module 3 Introduction | 01:01 |
23 | Installing Apollo Server and GraphQL | 02:06 |
24 | Creating a GraphQL Schema with the GraphQL JS Library | 09:29 |
25 | Querying and mutating listings data with GraphQL | 11:34 |
26 | Using the GraphQL Schema Language | 10:52 |
27 | Module 3 Summary | 02:07 |
28 | Module 4 Introduction | 00:55 |
29 | Introduction to MongoDB | 03:45 |
30 | Setting up a new MongoDB Cluster with Atlas | 06:52 |
31 | Connecting the server with MongoDB | 11:19 |
32 | TypeScript Generics and adding types to our Collections | 12:34 |
33 | Setting Up Env Variables | 08:02 |
34 | Seeding mock data to the database | 08:41 |
35 | Processing Data from MongoDB | 14:34 |
36 | Modularizing Resolvers | 07:18 |
37 | Module 4 Summary | 02:15 |
38 | Module 5 Introduction | 00:47 |
39 | React | 06:59 |
40 | Create React App | 04:22 |
41 | Create React App - The Rundown | 15:58 |
42 | Module 6 Introduction | 00:46 |
43 | The Listings Component | 03:47 |
44 | Props & TypeScript | 03:27 |
45 | Functional Components & TypeScript | 06:48 |
46 | Querying Listings | 14:17 |
47 | Abstracting the type of data from server fetch | 04:54 |
48 | Deleting a listing | 08:43 |
49 | Module 6 Summary | 02:05 |
50 | Module 7 Introduction | 01:23 |
51 | React Hooks | 08:00 |
52 | The useState Hook | 07:50 |
53 | The useEffect Hook | 11:46 |
54 | Custom useQuery Hook | 08:52 |
55 | Custom useQuery and refetch | 06:58 |
56 | Custom useQuery and loading/error states | 11:54 |
57 | Custom useMutation Hook | 16:22 |
58 | The useReducer Hook | 13:26 |
59 | Module 7 Summary | 05:08 |
60 | Module 8 Introduction | 00:55 |
61 | Creating our Apollo Client | 04:22 |
62 | React Apollo Hooks | 04:09 |
63 | Autogenerated types with Apollo CLI | 10:25 |
64 | Module 8 Summary | 02:39 |
65 | Module 9 Introduction | 00:27 |
66 | UI Frameworks and Ant Design | 04:35 |
67 | Styling with Ant Design | 20:31 |
68 | Part One Conclusion | 00:33 |
69 | Part Two Welcome | 01:56 |
70 | The TinyHouse App | 15:11 |
71 | Walkthrough of TinyHouse Code | 12:18 |
72 | Code Patterns & Behavior | 10:55 |
73 | How To Go Through The Course | 16:40 |
74 | Set-up For Part II | 02:52 |
75 | Module 2 Introduction | 00:34 |
76 | Routing in TinyHouse | 06:15 |
77 | React Router | 07:27 |
78 | Module 3 Introduction | 01:06 |
79 | Database Collection Structure | 05:17 |
80 | Database Document Structure | 15:18 |
81 | Seed & Clear Data from MongoDB | 08:28 |
82 | Module 4 Introduction | 00:53 |
83 | OAuth 2.0 | 04:36 |
84 | Google Sign-In (OAuth) | 04:50 |
85 | Google Sign-In GraphQL Fields | 04:51 |
86 | Using Google Sign-In & People API | 08:31 |
87 | Building the Authentication Resolvers | 18:56 |
88 | Building the UI for Login | 11:36 |
89 | Executing Login | 22:36 |
90 | Building the AppHeader & Logout | 18:06 |
91 | Module 4 Summary | 06:03 |
92 | Module 5 Introduction | 00:56 |
93 | Cookies & Login Sessions | 02:40 |
94 | localStorage vs. sessionStorage vs. cookies | 05:33 |
95 | Adding the Viewer Cookie on the Server | 11:06 |
96 | Adding the Viewer Cookie on the Client | 08:20 |
97 | X-CSRF Token | 08:51 |
98 | Module 5 Summary | 04:10 |
99 | Module 6 Introduction | 00:54 |
100 | User GraphQL Fields | 02:24 |
101 | Modifying the User, Listing, and Booking GraphQL TypeDefs | 10:48 |
102 | Building the User Resolvers | 21:39 |
103 | The UserProfile React Component | 23:02 |
104 | The UserListings & UserBookings React Components | 42:51 |
105 | Module 6 Summary | 07:21 |
106 | Module 7 Introduction | 00:34 |
107 | Listing GraphQL Fields | 02:21 |
108 | Building the Listing Resolvers | 16:07 |
109 | Querying for listing data | 11:21 |
110 | ListingDetails & ListingBookings | 25:39 |
111 | The ListingCreateBooking React Component | 34:31 |
112 | Module 7 Summary | 04:48 |
113 | Module 8 Introduction | 00:46 |
114 | Listings GraphQL Fields | 03:00 |
115 | Building the Listings Resolvers | 13:53 |
116 | Building the UI of the Homepage | 23:10 |
117 | Displaying the highest-priced listings in the Homepage | 19:59 |
118 | Module 8 Summary | 03:14 |
119 | Module 9 Introduction | 01:05 |
120 | Google's Geocoding API | 08:16 |
121 | Location-based searching for listings | 07:49 |
122 | Updating the listings resolver | 19:50 |
123 | Building the Listings page | 17:34 |
124 | Pagination & Filtering in the Listings page | 30:34 |
125 | Searching for listings from the App Header | 26:57 |
126 | Index location-based data | 09:25 |
127 | Module 9 Summary | 03:29 |
128 | Module 10 Introduction | 00:57 |
129 | Stripe & Stripe Connect | 07:59 |
130 | Stripe Connect OAuth | 11:34 |
131 | Stripe Connect GraphQL Fields | 09:12 |
132 | Building the Stripe Connect Resolvers | 13:52 |
133 | Connecting with Stripe on the Client | 28:00 |
134 | Disconnecting from Stripe on the Client | 13:31 |
135 | Module 10 Summary | 04:18 |
136 | Module 11 Introduction | 00:37 |
137 | HostListing GraphQL Fields | 02:44 |
138 | Building the HostListing Resolver | 15:28 |
139 | Building the UI of the Host page | 38:22 |
140 | Executing the HostListing Mutation | 28:21 |
141 | Module 11 Summary | 05:06 |
142 | Module 12 Introduction | 00:42 |
143 | Cloudinary & Image Storage | 07:14 |
144 | Image uploads with Cloudinary | 08:47 |
145 | Module 13 Introduction | 01:05 |
146 | CreateBooking GraphQL Fields | 02:52 |
147 | Building the CreateBooking Resolver | 25:58 |
148 | Resolving the BookingsIndex of a Listing | 23:03 |
149 | Disabling booked dates on the client | 25:21 |
150 | Creating the Booking Confirmation Modal | 21:13 |
151 | Displaying the Payment Form with React Stripe Elements | 17:22 |
152 | Executing the CreateBooking Mutation | 28:11 |
153 | Module 13 Summary | 09:02 |
154 | Module 14 Introduction | 00:29 |
155 | Cloud Computing | 06:41 |
156 | Deploying with Heroku | 45:14 |
157 | Walkthrough of Deployed App | 10:26 |
158 | Module 15 Introduction | 00:52 |
159 | The NotFound Page | 05:33 |
160 | Apollo Client & FetchPolicy | 16:33 |
161 | useLayoutEffect & Window Scroll | 10:27 |
162 | React Router Hooks | 10:26 |
163 | Disconnecting from Stripe & Revoking Access | 10:33 |
164 | Additional Listing DatePicker Changes | 10:07 |
165 | Part Two Conclusion | 00:41 |
166 | MODULE 17 INTRODUCTION | 00:49 |
167 | SQL VS. NOSQL | 05:07 |
168 | POSTGRESQL | 06:05 |
169 | TYPEORM | 16:04 |
170 | TINYHOUSE - POSTGRESQL & TYPEORM | 33:11 |
171 | Module 18 Introduction | 03:39 |
172 | Unit Testing | 11:45 |
173 | Jest & React Testing Library | 30:38 |
174 | Apollo React Testing | 08:36 |
175 | Getting Started | 08:23 |
176 | Testing the Home Component I | 23:21 |
177 | Testing the Home Component II | 40:34 |
178 | Testing the Login Component I | 26:17 |
179 | Testing the Login Component II | 29:00 |
180 | Pushing code & creating PRs | 14:40 |
Similar courses to TinyHouse: A Fullstack React Masterclass with TypeScript and GraphQL
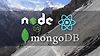
MERN Stack - React Node from Scratch Building Social Networkudemy
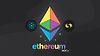
Solidity & Ethereum in React (Next JS): The Complete Guideudemy
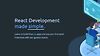
React Formula - Learn Frontend DevelopmentAlvin Zablan
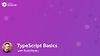
TypeScript Fundamentalsultimatecourses.com
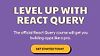
React Queryui.dev (ex. Tyler McGinnis)
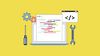
Laravel with React JS - Build Twitter Like Real Time Web Appudemy
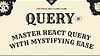